ETH transfer methods in solidity
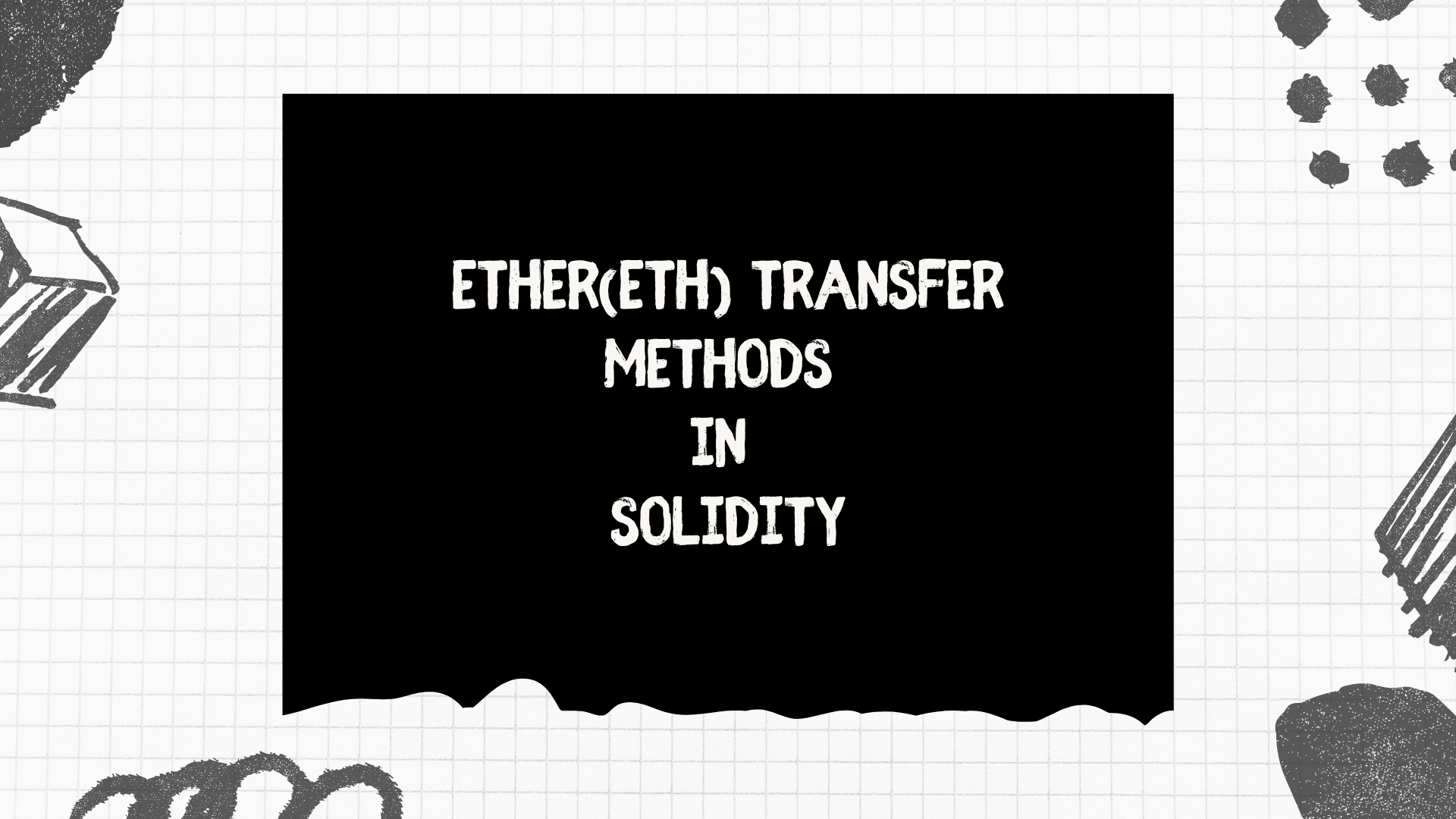
In Ethereum, transferring Ether between accounts is a fundamental operation. Whether you're building a decentralized application (dApp) or creating a complex financial system, understanding how to safely and efficiently send Ether is crucial.
Solidity, the primary programming language for Ethereum, offers several methods to achieve this, each with its own nuances and best-use scenarios.
We have 3 primary methods of sending ETH in solidity
send()
transfer()
call()
We will discuss their differences, their usecases, and the recommended practices for engineers.
Transfer
This method allows you to send Ether, consuming 2300
gas units. If the operation fails, it throws an error and reverts the transaction. It's gas is very low which is aimed at stopping re-entrancy attacks.
Characteristics:
Gas Limit: Automatically forwards a fixed amount of
2300
gas to the recipient. This is just enough to perform a basic operation, such as logging an event, but not enough to execute complex logic.Automatic Reversion: If the transfer fails for any reason (e.g., if the recipient's fallback function uses more than 2300 gas), the transaction will automatically revert, ensuring no Ether is lost.
Ease of Use: It is a straightforward method that requires minimal error handling.
Usecases
Use
transfer
for simple Ether transfers where you want to ensure the transaction will revert if the transfer fails.Ideal for cases where the recipient's fallback function is not expected to perform any complex operations.
Example:
contract EtherTransferExample {
function transferEther(address payable recipient) external payable {
recipient.transfer(msg.value); // Transfer all Ether sent to this function
}
}
Send
Similar to transfer
, send
also consumes 2300 gas units but returns a boolean value indicating the success or failure of the operation. Although Solidity's send function is not formally deprecated, its shortcomings with regard to security and gas management make it discouraged and essentially outdated.
Characteristics:
Gas Limit: Like
transfer
,send
also forwards2300
gas to the recipient.Boolean Return Value: Instead of automatically reverting the transaction on failure,
send
returnsfalse
. It is up to the developer to handle this return value and manage failure scenarios.Manual Error Handling: Because it doesn't automatically revert,
send
requires you to explicitly check the return value and handle errors accordingly.
Example:
contract EtherSendExample {
function sendEther(address payable recipient) external payable {
bool success = recipient.send(msg.value);
require(success, "Send failed"); // Handle failure manually
}
}
Call Method
The call
method is the most flexible and powerful of the three Ether transfer methods. It is a low-level function that can be used not only for sending Ether but also for calling functions on other contracts.
Characteristics:
Gas Limit: By default,
call
forwards all remaining gas to the recipient, allowing for more complex operations to be performed. However, you can also specify a custom gas limit if needed.Versatility:
call
can be used to invoke functions on other contracts or simply to send Ether. This makes it extremely versatile.Boolean Return Value: Like
send
,call
returns a boolean indicating success or failure. This allows you to handle errors manually.Security Considerations: Because it forwards all gas,
call
can be vulnerable to reentrancy attacks if not used carefully. It is essential to follow best practices, such as using the "checks-effects-interactions" pattern, to mitigate these risks.
Example:
contract EtherCallExample {
function callEther(address payable recipient) external payable {
(bool success, ) = recipient.call{value: msg.value}(""); // Send Ether using call
require(success, "Call failed"); // Handle failure manually
}
}
call
method is preferred in modern Solidity development because it overcomes the limitations of transfer
and send
, providing greater flexibility, versatility, and adaptability. While call
requires careful handling to avoid security issues like reentrancy, its ability to forward custom gas amounts, invoke external contract functions, and adapt to future changes in the Ethereum network makes it the most powerful and preferred option for sending Ether in Solidity.Conclusion
Choosing the right Ether sending method in Solidity depends on your specific use case and the complexity of the recipient's contract.
While transfer
and send
are simpler and were once widely used, call
has become the preferred method in modern Solidity development due to its flexibility, power, and adaptability to various scenarios. By understanding the characteristics and differences between these methods, you can make informed decisions that enhance the security and efficiency of your smart contracts.
Subscribe to my newsletter
Read articles from Akanimo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Akanimo
Akanimo
I am a Frontend Engineer who is passionate about tech and the web generally. I write about the best programming practices, concepts, and usage of tech products (documentations).