Mastering the Page Object Model in Selenium with Java: Best Practices with Examples
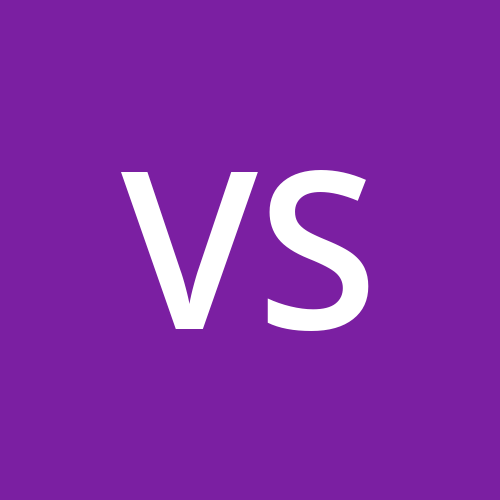
When it comes to test automation, maintaining a clean and scalable framework is crucial. The Page Object Model (POM) is a popular design pattern in Selenium that offers a structured way to handle web elements and interactions, leading to more maintainable and readable test scripts. In this blog, we'll dive into the best practices for implementing POM in Selenium with Java, along with some practical examples.
What is the Page Object Model?
The Page Object Model is a design pattern that encapsulates the elements and actions of a webpage within a dedicated class, often called a “Page Object.” Each class represents a specific page in the web application, and contains the locators for web elements (like buttons, text fields, etc.) along with methods to interact with these elements.
Key Benefits:
Separation of Key Components: Test logic is separated from the code that interacts with the UI, making your tests more modular and easier to maintain.
Reusability: Common page actions can be reused across different tests, reducing code duplication.
Readability: Tests become more readable as they describe the user’s interactions with the application in a clear and understandable way.
Best Practices for Implementing POM in Selenium with Java
1. Use Descriptive Names for Page Classes and Methods
Example: If you have a login page, your class should be named
LoginPage
and methods should be descriptive likeenterUsername()
,enterPassword()
, andclickLoginButton()
.Benefit: Descriptive names make the code self-explanatory, enhancing readability and maintainability.
javaCopy codepublic class LoginPage {
WebDriver driver;
@FindBy(id="username")
WebElement usernameField;
@FindBy(id="password")
WebElement passwordField;
@FindBy(id="loginButton")
WebElement loginButton;
public LoginPage(WebDriver driver) {
this.driver = driver;
PageFactory.initElements(driver, this);
}
public void enterUsername(String username) {
usernameField.sendKeys(username);
}
public void enterPassword(String password) {
passwordField.sendKeys(password);
}
public void clickLoginButton() {
loginButton.click();
}
}
2. Keep Page Object Classes Focused on One Page
Example: Your
HomePage
class should only contain elements and methods related to the home page. Avoid mixing elements from different pages.Benefit: This separation makes the code modular and easier to maintain as your application grows.
3. Utilize Inheritance for Common Page Elements
Example: If your web application has a common header or footer across pages, create a
BasePage
class that contains elements and methods for those components, and extend other page classes from this base class.Benefit: This reduces code duplication and centralizes changes to common components.
javaCopy codepublic class BasePage {
WebDriver driver;
@FindBy(id="headerLogo")
WebElement headerLogo;
public BasePage(WebDriver driver) {
this.driver = driver;
PageFactory.initElements(driver, this);
}
public void clickHeaderLogo() {
headerLogo.click();
}
}
public class HomePage extends BasePage {
public HomePage(WebDriver driver) {
super(driver);
}
// HomePage-specific methods here
}
4. Avoid Hard-Coding Locators
Example: Store locators in a separate file or use the
@FindBy
annotation to keep your code clean and centralized.Benefit: This approach makes it easier to update locators if the web application’s structure changes.
5. Implement a Consistent Strategy for Locators
Example: Choose the most stable and unique attributes (like
id
,name
, orclass
) to locate elements. If IDs are dynamic, consider using more robust strategies like CSS Selectors or XPath.Benefit: This reduces the chances of flaky tests due to changes in the UI.
javaCopy code@FindBy(css = "input[type='text']")
WebElement searchBox;
6. Use Method Chaining for Fluid Interfaces
- Example: Enable method chaining in your Page Object methods to create more readable and fluent test scripts.
javaCopy codepublic class LoginPage {
WebDriver driver;
@FindBy(id="username")
WebElement usernameField;
@FindBy(id="password")
WebElement passwordField;
@FindBy(id="loginButton")
WebElement loginButton;
public LoginPage(WebDriver driver) {
this.driver = driver;
PageFactory.initElements(driver, this);
}
public LoginPage enterUsername(String username) {
usernameField.sendKeys(username);
return this;
}
public LoginPage enterPassword(String password) {
passwordField.sendKeys(password);
return this;
}
public HomePage clickLoginButton() {
loginButton.click();
return new HomePage(driver);
}
}
7. Keep Tests Independent of Each Other
Example: Each test should prepare its own data and environment. Avoid dependencies between tests to ensure they can be run in isolation.
Benefit: This makes your test suite more reliable and easier to debug.
Conclusion
The Page Object Model is a powerful design pattern that can greatly enhance the maintainability and scalability of your Selenium test automation framework. By following these best practices, you can ensure that your tests are not only robust but also easy to read and maintain as your project grows.
Implementing POM effectively in your Selenium framework with Java is not just about writing better code; it’s about creating a foundation that will scale as your testing needs evolve.
Happy testing! 🚀
Subscribe to my newsletter
Read articles from Vanshika Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
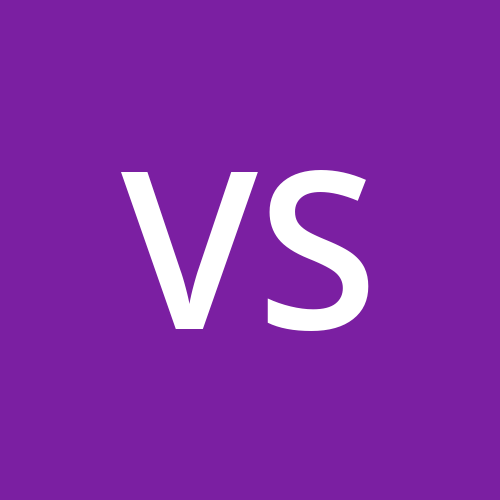