Discover Helo: Simplified Local Mail Development Testing

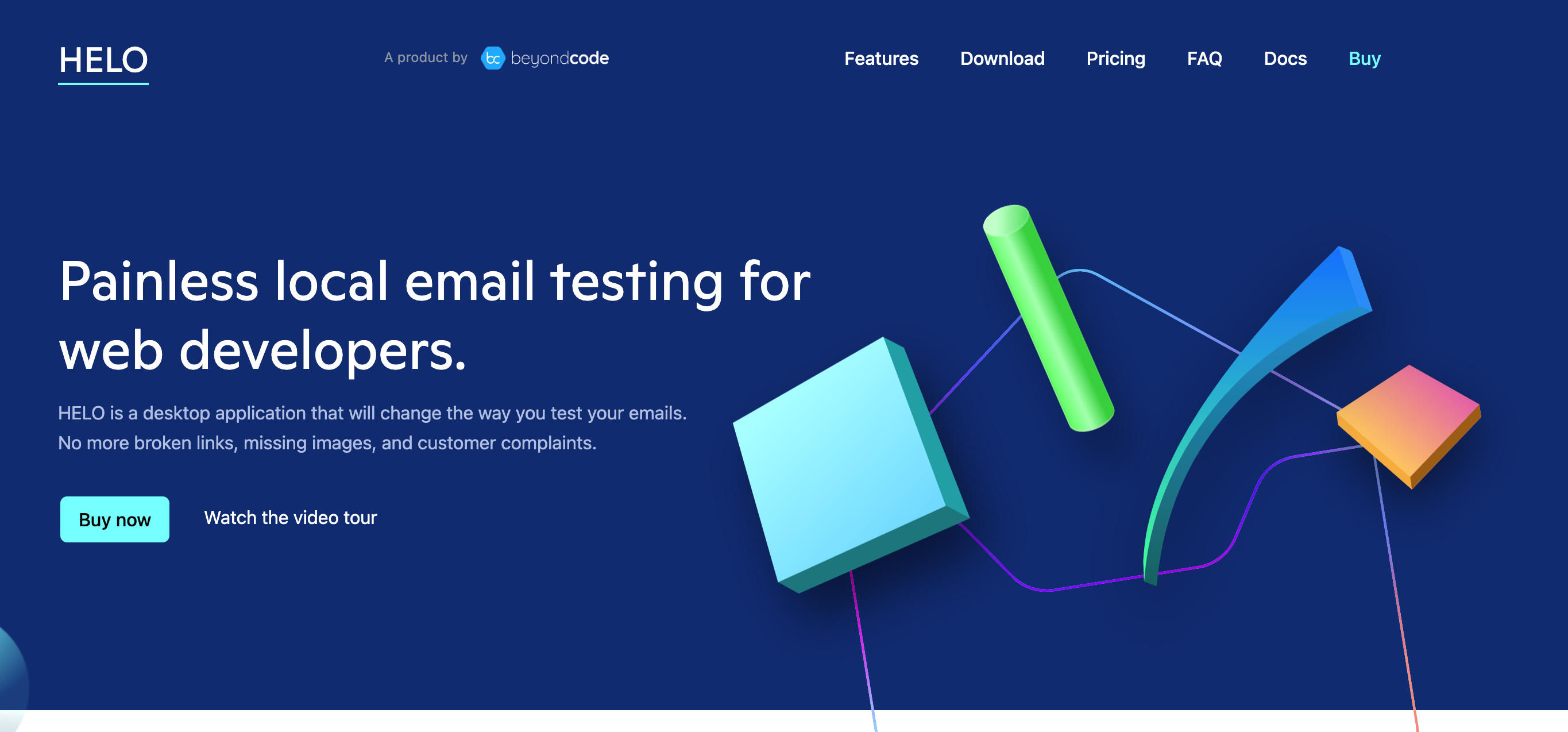
Introduction
In the realm of software development, testing email functionality is crucial to ensure that communications are sent correctly and received as intended. Helo is a powerful tool designed to facilitate local email testing by catching outgoing emails from your application and providing a suite of features to review and debug them.
Key Features of Helo
Local SMTP Server: Helo exposes an SMTP server that intercepts outgoing emails from your application, preventing them from reaching their actual recipients. This allows for safe and controlled testing environments.
Unlimited Inboxes: You can create dedicated inboxes for different applications or projects, with no limit on the number of inboxes. This helps in organizing and managing emails efficiently.
Comprehensive Content Review: Helo provides multiple views for email content, including HTML representation, text, source code, raw data, and headers. This ensures thorough inspection before deployment.
View Inspector: By installing the open-source helper package, developers can debug Laravel views or create custom helpers for other frameworks, enhancing the debugging process.
Link Checker: Helo automatically verifies that all links in an email are valid and return an HTTP 200 status code, ensuring no broken links are sent out.
Spam Report: With the spam report feature, Helo checks the Apache SpamAssassin score of incoming emails, helping to avoid emails being marked as spam.
Email Forwarding: Emails can be forwarded to teammates or clients for review, or to testing services to see how they appear in different email clients. This feature requires Helo Cloud.
Sharing Emails and Inboxes: Multiple emails and inboxes can be shared with clients and colleagues, facilitating collaborative testing and review processes.
Pricing
Helo costs a one-time fee of $49.
Configuration for Different Frameworks
Laravel
For Laravel applications, you need to adjust the mail server settings in your .env
file:
For Laravel 7+:
MAIL_MAILER=smtp
MAIL_HOST=127.0.0.1
MAIL_PORT=2525
MAIL_USERNAME=Inbox-Name
MAIL_PASSWORD=null
MAIL_ENCRYPTION=null
For Laravel 6 and below:
MAIL_DRIVER=smtp
MAIL_HOST=127.0.0.1
MAIL_PORT=2525
MAIL_USERNAME=Inbox-Name
MAIL_PASSWORD=null
MAIL_ENCRYPTION=null
Install the optional Helo helper package for additional debug information:
composer require --dev beyondcode/helo-laravel
Symfony
Modify the .env
file in your project directory:
MAILER_URL=smtp://127.0.0.1:2525?encryption=null&auth_mode=login&username=Mailbox-Name&password=
WordPress
Add the following code to configure your WordPress site:
function helo ($phpmailer) {
$phpmailer->isSMTP();
$phpmailer->Host = '127.0.0.1';
$phpmailer->SMTPAuth = true;
$phpmailer->Port = 2525;
$phpmailer->Username = 'Mailbox-Name';
$phpmailer->Password = '';
}
add_action('phpmailer_init', 'helo');
PHPMailer
Use this configuration:
$mail = new PHPMailer();
$mail->isSMTP();
$mail->Host = '127.0.0.1';
$mail->SMTPAuth = true;
$mail->Username = 'Mailbox-Name';
$mail->Password = '';
$mail->Port = 2525;
Yii Framework
Add the following to your config file:
'components' => [
...
'mail' => [
'class' => 'yii\swiftmailer\Mailer',
'transport' => [
'class' => 'Swift_SmtpTransport',
'host' => '127.0.0.1',
'username' => 'Mailbox-Name',
'password' => '',
'port' => '2525',
'encryption' => 'tls',
],
],
...
],
Nodemailer
Configure Nodemailer in Node.js:
let transport = nodemailer.createTransport({
host: "127.0.0.1",
port: 2525,
auth: {
user: "Mailbox-Name",
pass: ""
}
});
Ruby on Rails
Specify ActionMailer defaults in config/environments/*.rb
:
config.action_mailer.delivery_method = :smtp
config.action_mailer.smtp_settings = {
user_name: 'Mailbox-Name',
password: '',
address: '127.0.0.1',
domain: '127.0.0.1',
port: '2525',
authentication: :plain
}
Ruby (net/smtp)
Use the following code:
require 'net/smtp'
message = <<-END.split("\n").map!(&:strip).join("\n")
From: Private Person <from@127.0.0.1>
To: A Test User <to@127.0.0.1>
Subject: HELO!
This is a test e-mail message from HELO.
END
Net::SMTP.start('127.0.0.1', 2525, '127.0.0.1', 'Mailbox-Name', '', :plain) do |smtp|
smtp.send_message message, '[email protected]', '[email protected]'
end
Conclusion
Helo is an invaluable tool for developers looking to test email functionality locally. Its robust features and easy integration with various frameworks make it a go-to solution for ensuring that emails are sent correctly and efficiently before going live. By following the configuration guidelines, you can set up Helo with your application and start testing emails in no time.
Subscribe to my newsletter
Read articles from Tran Tuan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Tran Tuan
Tran Tuan
As a seasoned Senior FullStack Web and Mobile Developer, I bring over 10+ years of extensive experience in designing, developing, and deploying robust applications across various platforms. My expertise spans a diverse range of technologies, including PHP, NodeJS, Flutter, ReactNative, VueJS, AngularJS, and ReactJS, enabling me to deliver comprehensive solutions that meet the dynamic needs of modern businesses.