Redux in Next.js: A Comprehensive Guide

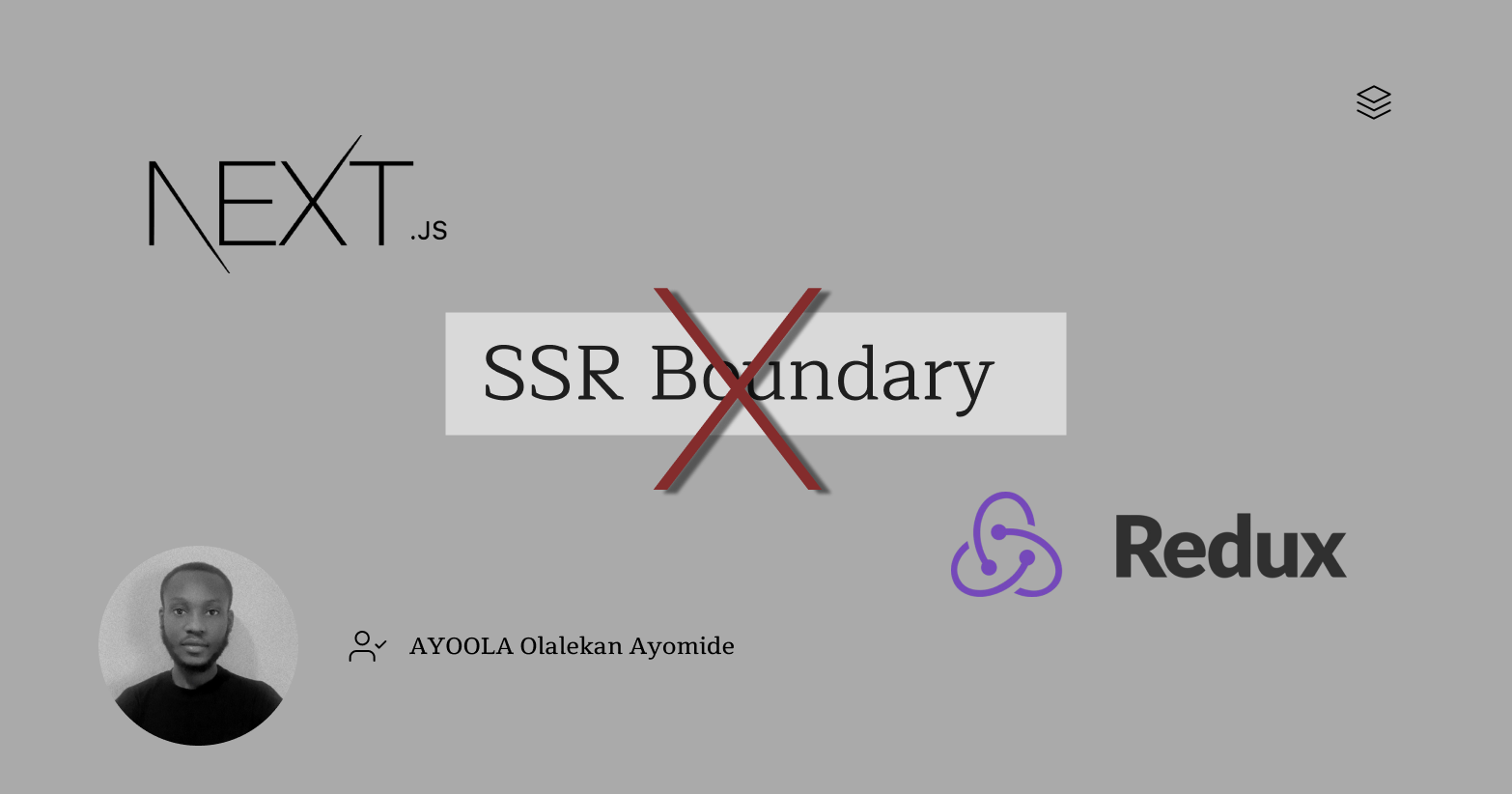
Next.js has rapidly gained popularity as a framework for building efficient and scalable web applications. As a React-based framework, it seamlessly integrates with many React tools, including Redux. However, integrating Redux into Next.js requires a thoughtful approach due to its server-side rendering (SSR) capabilities.
This article will guide you through the process of setting up a Next.js application, installing Redux and Redux Toolkit, and effectively integrating Redux into your project. By understanding the unique considerations of using Redux in Next.js, you can leverage its state management capabilities to build robust and maintainable web applications.
Introduction to Next.js and Redux
Next.js is a powerful React framework designed to build fast, scalable, and optimized web applications. It simplifies complex tasks like server-side rendering (SSR), static site generation (SSG), and routing. With Next.js, developers can create dynamic, SEO-friendly applications that perform exceptionally well on both the client and server sides. Its built-in features, like API routes and automatic code splitting, make it a go-to choice for modern web development, offering flexibility and performance out of the box.
Redux, on the other hand, is a state management library that helps manage the global state of an application in a predictable and consistent manner. It centralizes the application's state in a single store, making it easier to manage, debug, and scale as the application grows. Redux is especially useful in complex applications where multiple components need to share and update data consistently.
When integrated with Next.js, Redux enhances the framework's ability to handle complex state management across different pages and components, ensuring that data flow remains seamless and predictable. This integration allows developers to build robust applications that are not only fast and SEO-friendly but also maintainable and scalable over time.
Working with Next.js and Redux: Code Example
Setting Up Your Next.js App
- Create a Next.js App: run the following command on your vscode terminal
npx create-next-app@latest my-next-app
cd my-next-app
During this process, you'll be prompted with several configuration options. To keep things simple and follow along easily, I recommend just pressing Enter
at each prompt to accept the default settings. These defaults are optimized for a typical Next.js project and will help us get started quickly without needing to customize anything at this stage
After creating Next.js App, you should have a folder structure that looks like this
To make our Next.js app better organized, we'll use Redux. It's like a central hub for our app's data. We'll install Redux, create a store to hold data, set it up for Next.js, and use it in our app's different parts. This will help keep our code organized and easier to manage.
If you're already familiar with Next.js and Redux, you can skip the setup steps and jump right into integrating them. To help you get started, below I have a link to a pre-configured project that already has Redux set up (link to starter file). This will save you time and allow you to focus on creating slice and the integration process.
By the end of this guide, you'll have a solid understanding of how to integrate Redux into your Next.js app. We'll break down the steps and provide clear explanations along the way.
Install Redux and Redux Toolkit:
run the following command on your vscode terminal to install Redux Toolkit
npm install @reduxjs/toolkit react-redux
Creating a Slice and Configuring Redux
Let's create a counter function that can be used throughout our Next.js app. We'll use Redux to manage the counter's state, so it can be updated and accessed from different parts of the app. This will make our code more organized and easier to understand.
We'll create a "slice" in Redux. Think of a slice as a small piece of our app's state that handles a specific set of actions. In this case, our slice will be responsible for managing the counter's value.
The counter function will have three actions:
Increment: Increases the counter by 1.
Decrement: Decreases the counter by 1.
Increment by Amount: Increases the counter by a specified amount.
By using Redux to manage the counter's state, we can easily update and access the counter value from any component in our app.
// features/counterSlice.js
import { createSlice } from '@reduxjs/toolkit';
export const counterSlice = createSlice({
name: 'counter',
initialState: {
value: 0,
},
reducers: {
increment: (state) => {
state.value += 1;
},
decrement: (state) => {
state.value -= 1;
},
incrementByAmount: (state, action) => {
state.value += action.payload;
},
},
});
export const { increment, decrement, incrementByAmount } = counterSlice.actions;
export default counterSlice.reducer;
Step 2: Configure the Store
To set up our Redux store, we’ll need to configure it to work with our Next.js app. This involves creating a store object and defining the reducers that will handle actions and update the state. Think of the store as a container for our app’s data, and the reducers as the rules that determine how that data changes.
import { configureStore } from '@reduxjs/toolkit';
import counterReducer from './counterSlice';
const store = configureStore({
reducer: {
counter: counterReducer,
},
});
export default store;
Integrating Redux with Next.js: The Issue with SSR in Next.js
In a typical React app, you would wrap the entire app with the Redux Provider
. This will make the store accessible to all of our app’s components. However, doing this directly in Next.js can cause server-side rendering issues because the Redux store is initialized on the server, leading to potential state discrepancies between the server and client.
The Solution: Wrapping with a Custom StoreProvider
Component
To fix the problem of the Redux store being created on the server, we'll create a special component called StoreProvider
. This component will make sure that the Redux store is set up correctly only on the client side, by including "use client";
at the top where it needs to be.
By doing this, we can avoid any issues that might happen because the store is created on the server first. This will help our Next.js app work smoothly and prevent any problems with state management.
// components/StoreProvider.js
"use client";
import React from "react";
import { Provider } from "react-redux";
import store from "../store";
export default function StoreProvider({ children }) {
return <Provider store={store}>{children}</Provider>;
}
Then, in your layout file ( layout.js
) wrap the StoreProvider
around your app's content:
import StoreProvider from "@/StoreProvider";
export default function RootLayout({
children,
}: Readonly<{
children: React.ReactNode;
}>) {
return (
<StoreProvider>
<html lang="en">
<body className={inter.className}>{children}</body>
</html>
</StoreProvider>
);
}
This tells Next.js to wrap all your app's pages with the StoreProvider
component, ensuring the Redux store is initialized correctly on the client side. Once you've set up the StoreProvider
, your layout file should look something like this:
Using Redux to Manage the Counter State in Next.js App
Now at this point, Let's break down how to use Redux to dispatch actions and update the counter state in our Next.js app.
Dispatching Actions:
Think of actions as messages that tell Redux to update the state. In our case, we'll create actions to increment, decrement, and increment the counter by a specific amount.
Example: Dispatching an Action
To use the counter slice to increase the counter value, we'll need to import a special tool called useDispatch
from a library called react-redux
. This tool helps us send messages (called actions) to Redux to update the state.
We'll create a button that, when clicked, will send an "increment" action to Redux. This action will tell Redux to increase the counter value. And because we're using Redux, this change will be reflected everywhere in our app that needs to know about the counter's value.
'use client';
import React from 'react';
import { useDispatch } from 'react-redux';
import { increment } from '../features/counter/counterSlice';
export default function Counter() {
const dispatch = useDispatch();
return (
<div>
<button onClick={() => dispatch(increment())}>
Increment
</button>
</div>
);
}
Using useSelector
to Access State
To read values from the Redux store, you’ll use the useSelector
hook, which allows you to select specific parts of the state.
Example: Accessing State with useSelector
Continuing with the counter example, you can access the current value of the counter like this:
'use client';
import React from 'react';
import { useSelector } from 'react-redux';
export default function CounterValue() {
const count = useSelector((state) => state.counterSlice.value);
return (
<div>
<h1>Current Count: {count}</h1>
</div>
);
}
In this example, useSelector
is used to extract the value
from the counter
slice of the state. Whenever the state changes, this component will automatically re-render with the updated value.
Combining dispatch
and useSelector
Often, you’ll want to both read from and write to the state within the same component. Here’s how you can combine dispatch
and useSelector
:
'use client';
import React from 'react';
import { useSelector, useDispatch } from 'react-redux';
import { increment, decrement } from '../features/counter/counterSlice';
export default function CounterComponent() {
const count = useSelector((state) => state.counter.value);
const dispatch = useDispatch();
return (
<div>
<h1>Count: {count}</h1>
<button onClick={() => dispatch(increment())}>Increment</button>
<button onClick={() => dispatch(decrement())}>Decrement</button>
</div>
);
}
In the above example, the component displays the current count and provides buttons to increment or decrement the value. The state is read with useSelector
, and updated using dispatch
.
Example: Dispatching an Action by Amount
'use client';
import React from 'react';
import { useSelector, useDispatch } from 'react-redux';
import { incrementByAmount } from '../features/counter/counterSlice';
export default function CounterComponent() {
const count = useSelector((state) => state.counter.value);
const dispatch = useDispatch();
return (
<div>
<h1>Count: {count}</h1>
<button onClick={() => dispatch(incrementByAmount(5))}>Increment By Amount</button>
</div>
);
}
Now at this point, you should be well-equipped on how to integrate Redux with your Next.js application, effectively create Redux slices, and dispatch actions to manage your application's state seamlessly.
Additionally, below you can find a repository link to the final project, where you can see how all actions in the counter state are managed, along with styling that enhances the user interface.
Subscribe to my newsletter
Read articles from ayoola lekan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

ayoola lekan
ayoola lekan
Frontend Developer | Article Writer