Understanding Prettier, and how is it different from Linters
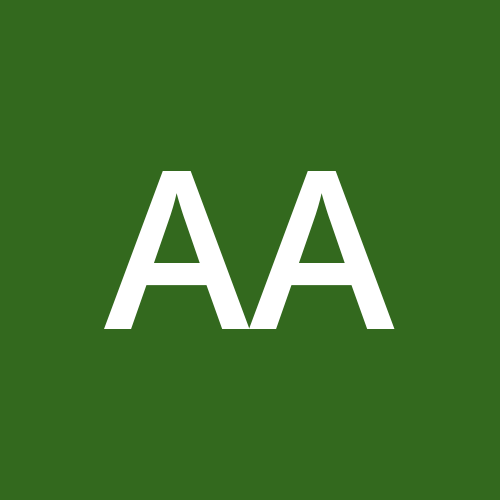
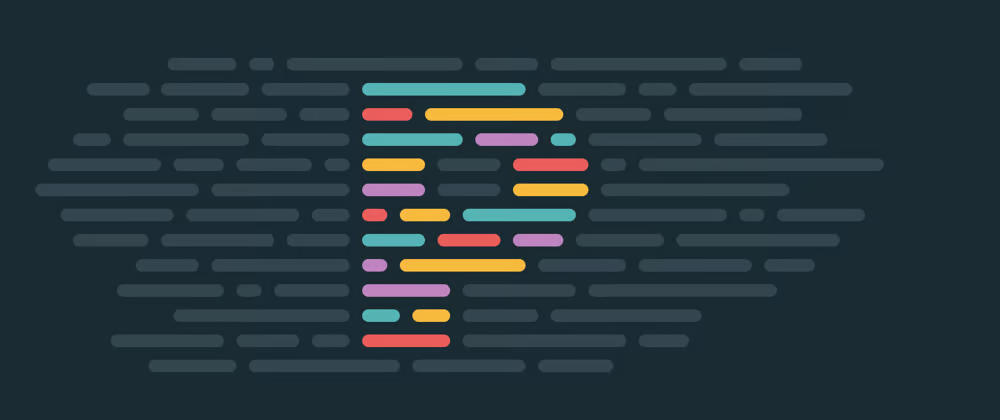
Prettier is an opinionated code formatter that enforces a consistent style by parsing your code and re-printing it with its own rules. It ensures consistent style in your JavaScript, TypeScript, CSS, and HTML code. It's like a personal stylist for your code, ensuring that everything looks neat, tidy, and follows a set of predefined rules. Unlike linters, which analyze your code for potential errors and enforce coding standards, Prettier focuses solely on formatting. This means that while linters can catch bugs and enforce best practices, Prettier ensures that your code looks the same across your entire codebase.
Why Use Prettier?
Consistency: Prettier ensures that your code adheres to a consistent style, making it easier to read and understand, especially when working in a team.
Time-Saving: No more manual formatting! Prettier automatically applies style changes, saving you time and effort.
Reduced Conflict: By enforcing a single style, Prettier helps prevent conflicts during code reviews and merges.
Improved Code Quality: Consistent formatting can improve code readability and maintainability.
Language Support: Prettier supports a wide range of languages and file types.
Integration: Prettier can be integrated with various editors and build tools, making it easy to use in your development workflow.
How is Prettier Different from Linting?
While both Prettier and linters like ESLint are used to improve code quality, they serve different purposes:
Prettier:
Focuses on Formatting: Prettier is solely concerned with the appearance of your code. It ensures that your code follows a consistent style, such as indentation, line length, and spacing.
Opinionated: Prettier has a set of rules that it follows strictly, with limited configuration options. This reduces debates over code style and ensures consistency.
Automatic Fixes: Prettier automatically formats your code, so you don't have to manually fix style issues.
Linters (e.g., ESLint):
Focuses on Code Quality: Linters analyze your code for potential errors, enforce coding standards, and suggest best practices.
Configurable: Linters are highly configurable, allowing you to define your own rules and standards.
Error Detection: Linters can catch potential bugs and issues in your code, such as unused variables, undefined functions, and more.
Setting up Prettier in Your Project
Installation
To get started with Prettier, install it as a development dependency in your project:
npm install --save-dev prettier
Basic Usage
You can format a file using Prettier from the command line:
npx prettier --write yourfile.js
Configuration
Create a .prettierrc
file in your project's root directory. This file specifies Prettier's configuration options. Here's a basic example:
{
"semi": false,
"singleQuote": true,
"tabWidth": 2,
"printWidth": 80,
"trailingComma": "es5"
}
For more configuration options, refer to the Prettier documentation: https://prettier.io/docs/en/
Example: Formatting JavaScript Code
Before:
const greeting ="Hello, world!"
const numbers= [1, 2, 3]
function sayHello(name) {
console.log("Hello, " + name + "!")
}
After:
const greeting = "Hello, world!";
const numbers = [1, 2, 3];
function sayHello(name) {
console.log(`Hello, ${name}!`);
}
Integrating Prettier into Your Workflow
Editor Integration: Most popular code editors have built-in support for Prettier. Enable the Prettier extension or plugin to automatically format your code as you type.
Git Hooks: Use Git Hooks to automatically format your code before committing changes. This ensures that your code is always formatted consistently.
CI/CD: Integrate Prettier into your continuous integration and continuous delivery pipelines to enforce formatting standards and catch formatting issues early.
Integrating with ESLint
You can use Prettier alongside ESLint to handle both formatting and linting. First, install the necessary packages:
npm install --save-dev eslint-config-prettier eslint-plugin-prettier
Then, update your ESLint configuration to include Prettier:
{
"extends": [
"eslint:recommended",
"plugin:prettier/recommended"
],
"plugins": ["prettier"],
"rules": {
"prettier/prettier": "error"
}
}
Editor Integration
Prettier can be integrated with various code editors, such as VSCode, Atom, and Sublime Text. For example, to integrate Prettier with VSCode, install the Prettier extension from the marketplace and add the following settings to your settings.json
:
{
"editor.formatOnSave": true,
"prettier.singleQuote": true,
"prettier.semi": false
}
Conclusion
Prettier is a powerful tool that helps maintain a consistent code style across your project. By automating code formatting, it allows you to focus on writing code rather than worrying about style issues. When used alongside a linter like ESLint, Prettier can significantly improve your development workflow and code quality.
Subscribe to my newsletter
Read articles from Aanchal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
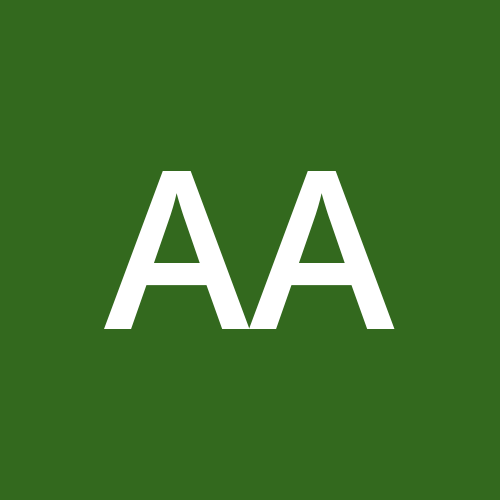