How to Use Merge Sort Algorithm Explained
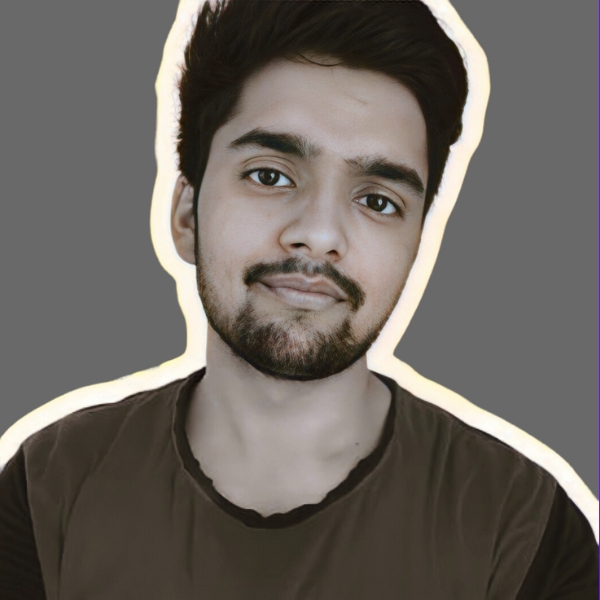
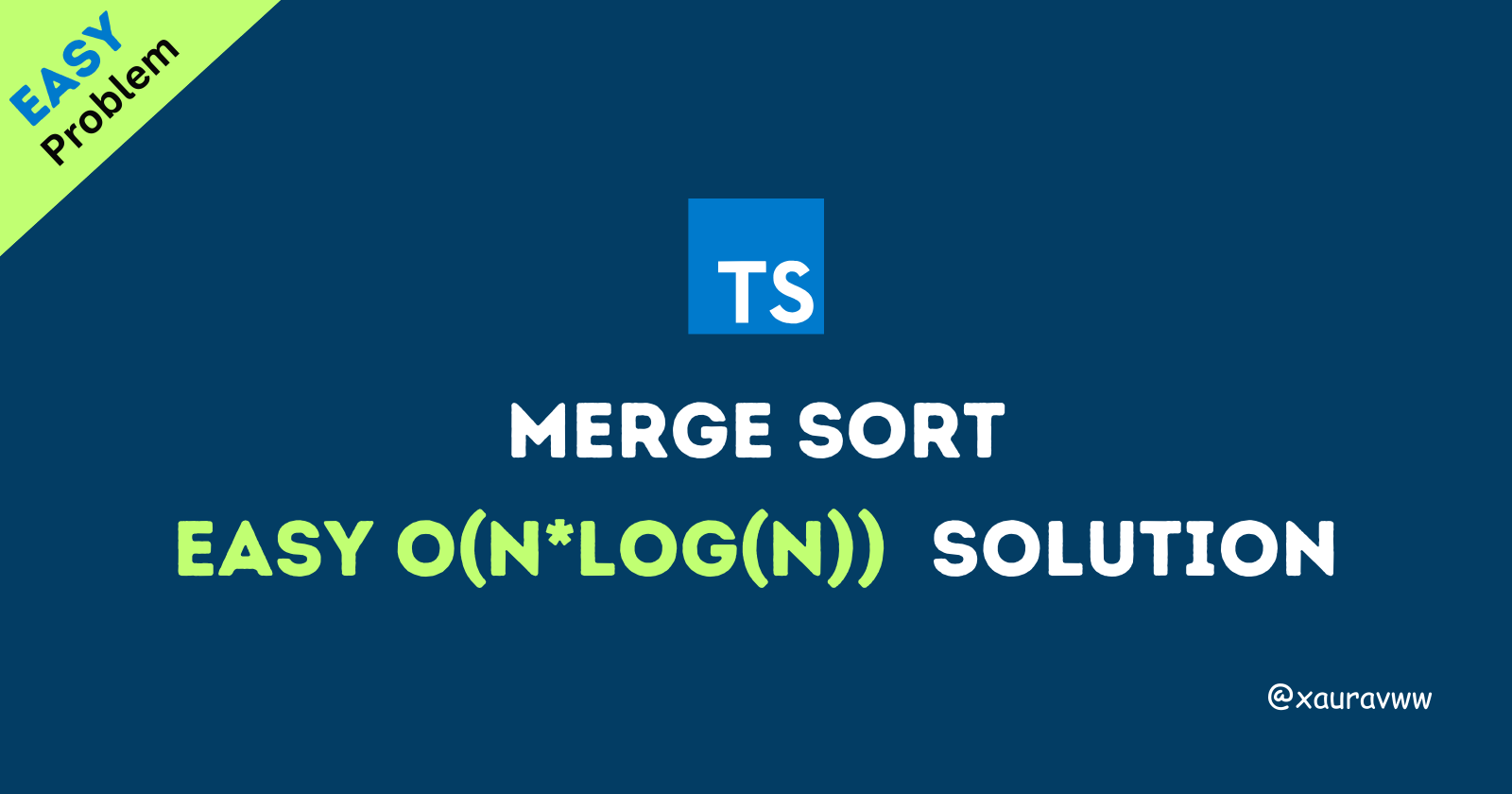
What is Merge Sort?
Merge Sort is a divide and conquer algorithm that splits an array into smaller sub-arrays, sorts those sub-arrays, and then merges them back together in order. It's known for its efficiency, particularly with larger datasets, and has a time complexity of O(n log n).
Merge sort is a stable sorting algorithm. Stability in sorting means that if two elements have equal keys, their relative order is preserved in the sorted output.
Code
//similar to we do merge two sorted arrays problem
// s starting index
// e ending index
// m is middle index
function merge(nums: number[], s: number, m: number, e: number): void {
let arr: number[] = [];
let i = s;
let j = m + 1;
// Compare elements from both halves and merge them in sorted order
while (i <= m && j <= e) {
if (nums[i] <= nums[j]) {
arr.push(nums[i]);
i++;
} else {
arr.push(nums[j]);
j++;
}
}
// If there are remaining elements in the right half, add them
while (j <= e) {
arr.push(nums[j]);
j++;
}
// If there are remaining elements in the left half, add them
while (i <= m) {
arr.push(nums[i]);
i++;
}
// Copy the sorted elements back into the original array
for (let k = 0; k < arr.length; k++) {
nums[s + k] = arr[k];
}
}
function mergeSort(nums: number[], s: number, e: number): void {
// Base case: if the array has only one element, it's already sorted
if (s >= e) {
return;
}
// Find the middle point of the array
let m = Math.floor((s + e) / 2);
// Recursively sort the first and second halves
// this is using 2-branch recursion
mergeSort(nums, s, m);
mergeSort(nums, m + 1, e);
// Merge the sorted halves
merge(nums, s, m, e);
}
function sortArray(nums: number[]): number[] {
mergeSort(nums, 0, nums.length - 1);
return nums;
}
Working
1. The merge
Function
The merge
function is like a helper that combines two groups of numbers into one big group, but in the right order.
Comparing Two Groups: The function starts by looking at the first number in both groups. It picks the smaller number and puts it into a new list. It keeps doing this until it has looked at all the numbers in both groups.
Adding Leftover Numbers: After comparing, there might be some numbers left in one of the groups. The function adds these remaining numbers to the new list.
Putting the Numbers Back: Finally, the function takes this new list (which is now in the right order) and puts it back into the original list where the two groups were.
2. The mergeSort
Function
This function is where the magic happens—it’s the one that actually sorts the list of numbers.
Splitting the List: The function starts by splitting the list into two smaller lists. If a list has only one number, it stops splitting because a single number is already sorted.
Sorting the Small Lists: The function then calls itself to sort each of these smaller lists.
Merging the Small Lists: Once the small lists are sorted, the
merge
function is called to combine them back into a larger list, but now in the correct order.
3. The sortArray
Function
This is the main function that starts the sorting process.
Starting the Sort: It calls the
mergeSort
function on the whole list of numbers.Returning the Sorted List: Once everything is sorted, it gives you back the list of numbers, but now they are in the correct order from smallest to largest.
Comment if I have committed any mistake. Let's connect on my socials. I am always open for new opportunities , if I am free :P
Subscribe to my newsletter
Read articles from Saurav Maheshwari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
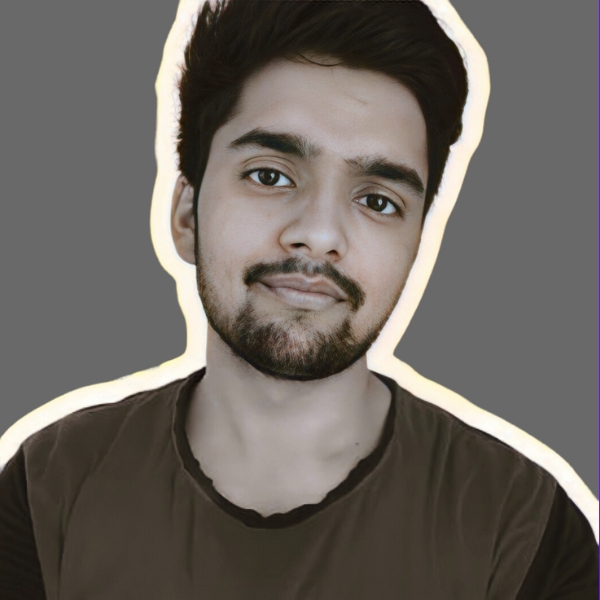