Exploring the Technical Aspects of Stellar and Soroban: A Comprehensive Guide
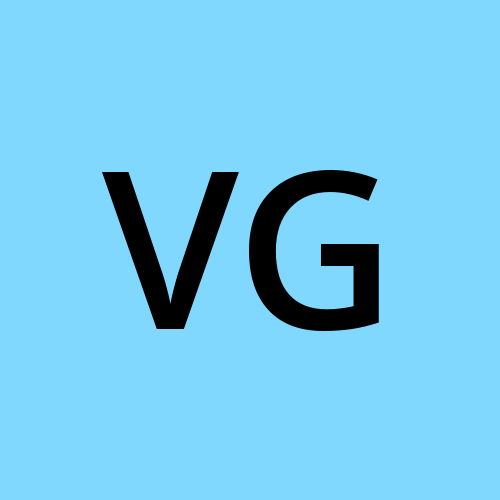
1. Introduction
Stellar: A blockchain platform designed to facilitate fast, secure, and low-cost cross-border transactions. Stellar has gained prominence in the financial sector, especially in regions with limited access to traditional banking services.
Soroban: Stellar's smart contract platform, built to extend the capabilities of the Stellar network. Soroban allows developers to create more complex and customizable decentralized applications (dApps).
Significance: Stellar and Soroban together form a powerful combination in the blockchain ecosystem, offering both financial services and the ability to build sophisticated dApps.
2. Technical Overview
2.1 Stellar's Architecture
Stellar Core:
- The backbone of the Stellar network.
- Responsible for validating transactions and maintaining network integrity.
- Nodes running Stellar Core communicate to achieve consensus on the status of the global ledger.
Ledger and Accounts:
- Global ledger replicated across all network nodes.
- Accounts hold balances in various assets (currencies, tokens).
- Transactions are used to create, modify, or delete accounts.
Stellar Consensus Protocol (SCP):
- A Federated Byzantine Agreement (FBA) protocol.
- Achieves consensus without requiring every node to agree with all others.
- Uses quorum slices (sets of trusted peers) to ensure scalability and security.
2.2 Soroban's Architecture
Soroban Runtime:
- A WebAssembly (Wasm)-based execution environment.
- Enables high-performance and deterministic execution of smart contracts.
- WebAssembly (Wasm) offers fast and secure code execution.
Contract Storage and State:
- Contracts maintain their own state on-chain.
- The state is persistent and accessible across transactions.
Cross-Contract Communication:
- Contracts can interact with each other seamlessly.
- Facilitates the creation of complex applications composed of multiple contracts.
3. Code Snippets
3.1 Creating an Account on Stellar
python
from stellar_sdk import Keypair, Server, TransactionBuilder, Network
Generate a keypair for the new account
new_keypair = Keypair.random()
print(f"Public Key: {new_keypair.public_key}")
print(f"Secret Seed: {new_keypair.secret}")
Fund the new account using a testnet faucet (for development purposes)
server = Server(horizon_url="
https://horizon-testnet.stellar.org
")
response = server.friendbot(new_keypair.public_key).call()
print(f"Account creation response: {response}")
- Purpose:
- Generates a new account on the Stellar testnet.
- Utilizes the stellar_sdk
library to interact with the network.
- Funds the account using a testnet faucet for testing and development.
3.2 Deploying a Smart Contract on Soroban
rust
#![no_std]
use soroban_sdk::{contractimpl, Env};
pub struct HelloWorld;
#[contractimpl]
impl HelloWorld {
pub fn hello(env: Env, name: String) -> String {
let greeting = format!("Hello, {}!", name);
env.log(&greeting);
greeting
}
}
#[cfg(test)]
mod test {
use super::*;
use soroban_sdk::{Env, testutils::Logger};
[test]
fn test_hello() {
let env = Env::default();
let logger = Logger::new(&env);
assert_eq!(HelloWorld::hello(env.clone(), "Soroban".into()), "Hello, Soroban!");
logger.assert_last_log("Hello, Soroban!");
}
}
- Purpose:
- Defines a simple "Hello, World!" smart contract for Soroban.
- Uses Rust programming language.
- Logs and returns a greeting based on the input name.
3.3 Interacting with a Smart Contract on Soroban
python
from stellar_sdk import Server, Keypair, TransactionBuilder, Network, Soroban
Connect to the Stellar testnet
server = Server(horizon_url="
https://horizon-testnet.stellar.org
")
source_keypair = Keypair.from_secret("<Your Secret Seed>")
Create a transaction to invoke the smart contract
tx = (
TransactionBuilder(
source_account=server.load_account(source_keypair.public_key),
network_passphrase=Network.TESTNET_NETWORK_PASSPHRASE,
base_fee=100
)
.add_operation(
Soroban.invoke_contract_operation(
contract_id="<Contract ID>",
method_name="hello",
args=["Stellar"]
)
)
.build()
)
# Sign and submit the transaction
tx.sign(source_keypair)
response = server.submit_transaction(tx)
print(f"Transaction response: {response}")
- Purpose:
- Demonstrates how to invoke a Soroban smart contract from the Stellar network.
- Uses Python and the stellar_sdk
library.
- Creates, signs, and submits a transaction to invoke a contract method.
4. Diagrams or Screenshots
4.1 Diagram
1: Stellar Network Architecture
Visual Representation: A diagram illustrating Stellar's network architecture, including how nodes interact to maintain the global ledger and achieve consensus using SCP.
4.2 Diagram
2: Soroban Smart Contract Workflow
- Visual Representation : A diagram showcasing the workflow of deploying and interacting with smart contracts on Soroban, emphasizing key steps such as contract deployment, method invocation, and state management.
5. Use Cases
5.1 Cross-Border Payments
-Application: Stellar is used to facilitate cross-border payments, especially in underbanked regions.
- Example: IBM's World Wire payment network, built on Stellar, allows financial institutions to clear and settle payments in near real-time.
5.2 Tokenization of Assets
- Application: Soroban enables the tokenization of assets on the Stellar network, allowing various assets (e.g., real estate, commodities) to be traded on-chain.
- Example: Companies can tokenize physical or intellectual assets and manage their transfers on the blockchain.
5.3 Decentralized Finance (DeFi)
-Application : Soroban powers DeFi applications on Stellar, enabling activities like lending, borrowing, and trading without traditional intermediaries.
- Example : Developers can create decentralized lending platforms that operate entirely on Soroban's smart contract infrastructure.
6. Conclusion
- Summary : Stellar and Soroban provide a robust platform for financial transactions and decentralized applications, offering scalability, security, and flexibility.
- Future Potential : As blockchain technology continues to grow, Stellar and Soroban are positioned to drive innovation in various sectors, from cross-border payments to decentralized finance.
-Final Thoughts : The integration of smart contracts with Stellar's payment capabilities through Soroban opens up new possibilities for developers and businesses, enhancing the blockchain ecosystem's overall utility and appeal.
Soroban: A Smart Contract Platform on Stellar
1. Soroban’s Architecture
Soroban is Stellar’s smart contract platform, designed to bring the flexibility and programmability of smart contracts to the Stellar ecosystem. Built as a layer on top of the Stellar network, Soroban integrates seamlessly with Stellar’s existing infrastructure while providing developers with the tools they need to build decentralized applications (dApps).
Soroban’s architecture is modular, allowing developers to deploy smart contracts that can interact with the Stellar network’s core functions, such as asset issuance, payments, and the decentralized exchange. This integration enables developers to create sophisticated financial applications that leverage Stellar’s fast and low-cost transactions.
2. Smart Contract Execution and Security
Smart contracts on Soroban are executed in a secure and deterministic environment, ensuring that the outcome of contract execution is always predictable and consistent. Soroban achieves this through the use of WebAssembly (WASM), a lightweight and efficient runtime that allows smart contracts to be written in multiple programming languages and executed with minimal overhead.
Security is a top priority for Soroban, and the platform includes several features to ensure that smart contracts are safe from vulnerabilities and exploits. For example, Soroban’s execution environment includes built-in protections against common smart contract vulnerabilities, such as reentrancy attacks and integer overflow/underflow.
3. Developer Tools and Ecosystem
Soroban provides a comprehensive set of tools and libraries that make it easy for developers to build, test, and deploy smart contracts. The platform includes a user-friendly development environment with debugging and testing tools, as well as extensive documentation and tutorials to help developers get started.
In addition to these tools, Soroban also benefits from being part of the larger Stellar ecosystem, which includes a wide range of partners, services, and resources. Developers can leverage existing infrastructure, such as Stellar’s decentralized exchange and anchor network, to create innovative dApps that solve real-world problems.
Use Cases: Real-World Applications of Stellar and Soroban
1. Cross-Border Payments with Stellar
Stellar’s primary use case is in the realm of cross-border payments, where its ability to facilitate fast, low-cost transactions across different currencies is particularly valuable. Several major financial institutions and remittance companies have adopted Stellar for this purpose, including IBM’s World Wire platform, which uses Stellar to enable banks to settle international payments quickly and efficiently.
2. Decentralized Finance (DeFi) on Soroban
Soroban’s smart contract capabilities open up new possibilities for decentralized finance (DeFi) applications on the Stellar network. Developers can create dApps that offer services such as lending, borrowing, and asset trading without the need for traditional financial intermediaries. This has the potential to democratize access to financial services, particularly in regions where banking infrastructure is limited.
3. Tokenization of Assets
Both Stellar and Soroban are well-suited for the tokenization of assets, a process that involves creating digital tokens that represent ownership of real-world assets, such as real estate, commodities, or securities. By using Stellar’s fast and low-cost transactions combined with Soroban’s smart contracts, developers can create platforms that allow users to buy, sell, and trade tokenized assets with ease.
Conclusion: The Future of Stellar and Soroban in Blockchain
Stellar and Soroban represent a powerful combination of technologies that are poised to shape the future of blockchain and decentralized finance. With Stellar’s focus on cross-border payments and Soroban’s smart contract capabilities, these platforms offer a robust and scalable solution for a wide range of financial applications.
As the digital economy continues to grow, the need for secure, fast, and efficient financial infrastructure will only become more pressing. Stellar and Soroban are well-positioned to meet these challenges, offering developers and businesses the tools they need to innovate and succeed in this rapidly changing landscape.
Whether you are a developer looking to build the next big dApp or a business seeking to streamline cross-border payments, Stellar and Soroban provide a solid foundation for success in the world of blockchain technology. The future of finance is digital, and with Stellar and Soroban, that future is within reach.
for more details - stellar developer docs => https://developers.stellar.org/docs
Subscribe to my newsletter
Read articles from Vansh Goyal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
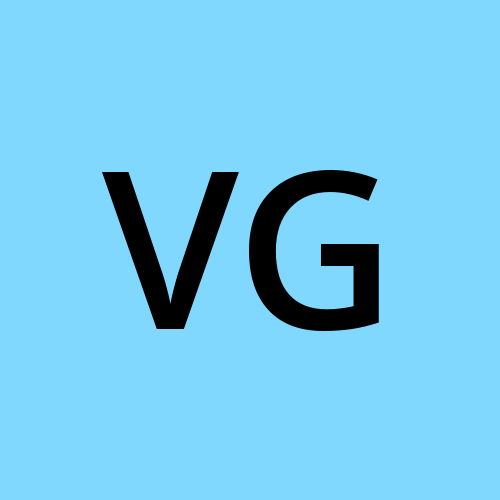