Building a Decentralized Voting Application with Soroban on Stellar
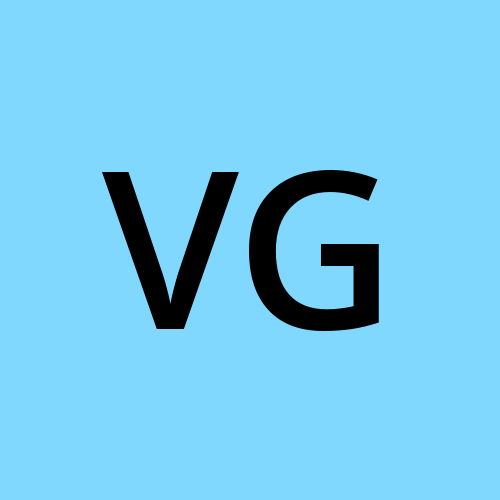
Introduction
In an era where transparency and trust are paramount, blockchain technology offers solutions to some of the most pressing issues in digital voting. Stellar's Soroban, with its robust smart contract capabilities, provides a unique opportunity to build decentralized voting applications. This article will guide you through creating a decentralized voting application on Soroban, showcasing its potential to ensure transparency, security, and fairness in the voting process.
Why Decentralized Voting?
Traditional voting systems are often plagued with issues such as voter fraud, lack of transparency, and the centralization of control. By leveraging blockchain technology, we can build a voting system that is:
Transparent: All votes are recorded on the blockchain, which can be publicly verified.
Secure: Blockchain ensures that once a vote is cast, it cannot be altered.
Decentralized: No single entity has control over the voting process, reducing the risk of manipulation.
Setting Up the Soroban Environment
Before we dive into coding, let's set up the Soroban environment to build and test our decentralized voting application.
Install Rust: First, ensure Rust is installed on your system:
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
Install Soroban CLI: With Rust installed, install the Soroban CLI:
cargo install --locked soroban-cli
Verify Installation: Confirm that the Soroban CLI is installed correctly:
soroban --version
Creating the Voting Contract
Let's start by writing a smart contract that will manage the voting process. Our contract will allow users to create a proposal, vote on it, and fetch the results.
Create a New Soroban Project:
soroban contract new voting_contract cd voting_contract
Define the Contract: Open
src/
lib.rs
and replace its contents with the following code:use soroban_sdk::{contractimpl, Env, Symbol}; pub struct VotingContract; #[contractimpl] impl VotingContract { pub fn create_proposal(env: Env, proposal_name: String) { env.storage().set(Symbol::new(env, "proposal"), &proposal_name); env.storage().set(Symbol::new(env, "yes_votes"), &0u32); env.storage().set(Symbol::new(env, "no_votes"), &0u32); } pub fn vote_yes(env: Env) { let mut yes_votes: u32 = env.storage().get(Symbol::new(env, "yes_votes")).unwrap(); yes_votes += 1; env.storage().set(Symbol::new(env, "yes_votes"), &yes_votes); } pub fn vote_no(env: Env) { let mut no_votes: u32 = env.storage().get(Symbol::new(env, "no_votes")).unwrap(); no_votes += 1; env.storage().set(Symbol::new(env, "no_votes"), &no_votes); } pub fn get_results(env: Env) -> (u32, u32) { let yes_votes: u32 = env.storage().get(Symbol::new(env, "yes_votes")).unwrap(); let no_votes: u32 = env.storage().get(Symbol::new(env, "no_votes")).unwrap(); (yes_votes, no_votes) } }
In this contract:
create_proposal
initializes a new proposal with a name and zero votes.vote_yes
increments the count of yes votes.vote_no
increments the count of no votes.get_results
returns the current number of yes and no votes.
Building the Contract: Compile the contract to generate the WASM file:
soroban build
This will create a compiled contract in the
target/wasm32-unknown-unknown/release/
directory.
Deploying the Contract on Soroban
Now that our contract is ready, let’s deploy it on the Soroban test network.
Deploy the Contract:
soroban contract deploy --wasm target/wasm32-unknown-unknown/release/voting_contract.wasm
This command returns a contract ID, which you'll use for interaction.
Initialize a Proposal: Let’s create a proposal using the contract:
soroban contract invoke --id <contract-id> --fn create_proposal --arg "Upgrade to Soroban"
Replace
<contract-id>
with your actual contract ID. This sets up a new proposal titled "Upgrade to Soroban."
Interacting with the Voting Contract
Once deployed, we can interact with the voting contract to cast votes and check results.
Cast a Vote: You can vote “yes” or “no” using the following commands:
To vote yes:
soroban contract invoke --id <contract-id> --fn vote_yes
To vote no:
soroban contract invoke --id <contract-id> --fn vote_no
Check Results: To see the current voting results:
soroban contract invoke --id <contract-id> --fn get_results
This command will output the number of yes and no votes, giving you real-time visibility into the voting process.
Enhancing the Voting Application
While the basic voting contract is functional, there are several enhancements you can make to create a more sophisticated application:
Voter Authentication: Implement voter registration to ensure that only authorized users can vote.
Vote Weighting: Allow different weights for different voters (e.g., stakeholders vs. regular users).
Proposal Expiry: Introduce a mechanism to close voting after a certain period or when a quorum is reached.
Multi-Choice Voting: Expand beyond binary voting to allow multiple choices.
Conclusion
Soroban on Stellar provides a robust framework for developing decentralized applications like voting systems. By leveraging the transparency, security, and decentralization of blockchain, you can create voting applications that are resistant to fraud and manipulation. The steps outlined in this guide give you a starting point for building and experimenting with Soroban smart contracts, opening up possibilities for innovation in decentralized governance, decision-making, and beyond.
Start building with Soroban today and be a part of the future of decentralized applications on Stellar!
This guide provides practical, hands-on experience in setting up a Soroban environment, writing and deploying a voting contract, and interacting with it. These examples give developers a clear understanding of how to use Soroban for real-world applications, making the learning process more engaging and relevant.
Subscribe to my newsletter
Read articles from Vansh Goyal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
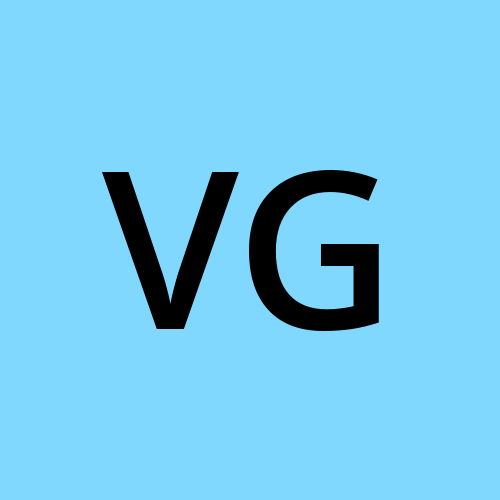