Building a Decentralized Voting Application on Soroban with Python
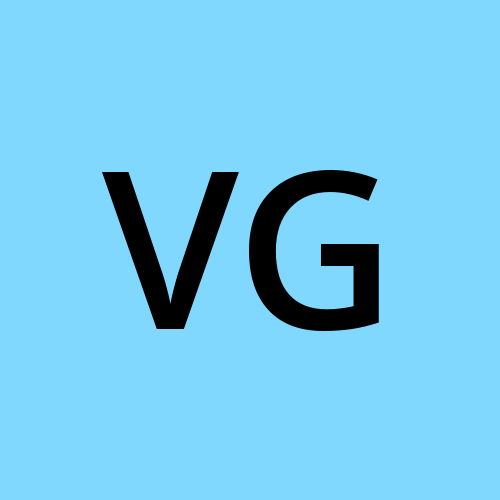
Introduction
Blockchain is increasingly being recognized for its potential to enhance transparency and security across various domains. One exciting application of blockchain is in voting systems, where transparency and immutability are paramount. Stellar's Soroban platform allows developers to build scalable and efficient smart contracts. In this guide, we will walk through building a simple decentralized voting application using Soroban and Python.
Why Use Soroban and Python?
Soroban: Soroban is Stellar's smart contract platform that allows the development of decentralized applications with a focus on scalability and low transaction costs. It integrates seamlessly with the Stellar network, offering a robust environment for smart contracts.
Python: Python is a versatile and popular programming language, known for its simplicity and readability. With a wide range of libraries available for interacting with blockchain networks, Python is an excellent choice for building and interacting with decentralized applications.
Prerequisites
Before you start, ensure you have the following tools installed on your machine:
Python (v3.7 or later)
pip (Python package manager)
Rust (for Soroban development)
Soroban CLI: Install using Cargo (Rust's package manager)
bashCopy codecargo install --locked soroban-cli
Step 1: Setting Up the Python Environment
Create a New Directory: Start by creating a new directory for your project.
bashCopy codemkdir soroban-voting-app-python cd soroban-voting-app-python
Create a Virtual Environment: It's a good practice to use a virtual environment to manage dependencies.
bashCopy codepython3 -m venv venv source venv/bin/activate # On Windows use `venv\Scripts\activate`
Install Dependencies: We will use the
stellar-sdk
for interacting with the Stellar network.bashCopy codepip install stellar-sdk
Step 2: Writing the Soroban Smart Contract
Create the Soroban Project:
bashCopy codesoroban contract new voting_contract cd voting_contract
Write the Voting Contract: In
src/lib.rs
, add the following code to define the voting logic.rustCopy codeuse soroban_sdk::{contractimpl, Env, Symbol}; pub struct VotingContract; #[contractimpl] impl VotingContract { pub fn create_proposal(env: Env, proposal_name: String) { env.storage().set(Symbol::new(env, "proposal"), &proposal_name); env.storage().set(Symbol::new(env, "yes_votes"), &0u32); env.storage().set(Symbol::new(env, "no_votes"), &0u32); } pub fn vote_yes(env: Env) { let mut yes_votes: u32 = env.storage().get(Symbol::new(env, "yes_votes")).unwrap(); yes_votes += 1; env.storage().set(Symbol::new(env, "yes_votes"), &yes_votes); } pub fn vote_no(env: Env) { let mut no_votes: u32 = env.storage().get(Symbol::new(env, "no_votes")).unwrap(); no_votes += 1; env.storage().set(Symbol::new(env, "no_votes"), &no_votes); } pub fn get_results(env: Env) -> (u32, u32) { let yes_votes: u32 = env.storage().get(Symbol::new(env, "yes_votes")).unwrap(); let no_votes: u32 = env.storage().get(Symbol::new(env, "no_votes")).unwrap(); (yes_votes, no_votes) } }
Compile the Contract:
bashCopy codesoroban build
Step 3: Deploying the Contract
Deploy the Contract:
bashCopy codesoroban contract deploy --wasm target/wasm32-unknown-unknown/release/voting_contract.wasm
This command will provide a contract ID that will be used to interact with the contract.
Step 4: Interacting with the Smart Contract using Python
Set Up Interaction Script: In the root directory of your Python project, create a file named
interact.py
.Write the Interaction Code:
pythonCopy codefrom stellar_sdk import Server, Keypair, TransactionBuilder, Network, SorobanServer # Replace with your Horizon server server = Server("https://horizon-testnet.stellar.org") soroban_server = SorobanServer(server) # Replace with your secret key secret_key = "your-secret-key-here" keypair = Keypair.from_secret(secret_key) public_key = keypair.public_key # Replace with your contract ID contract_id = "your-contract-id-here" def create_proposal(proposal_name): account = server.load_account(public_key) transaction = TransactionBuilder( source_account=account, network_passphrase=Network.TESTNET_NETWORK_PASSPHRASE, base_fee=100 ).append_host_function_call_op( contract_id=contract_id, function="create_proposal", parameters=[proposal_name] ).set_timeout(30).build() transaction.sign(keypair) response = server.submit_transaction(transaction) print(f"Proposal created: {response}") def vote_yes(): account = server.load_account(public_key) transaction = TransactionBuilder( source_account=account, network_passphrase=Network.TESTNET_NETWORK_PASSPHRASE, base_fee=100 ).append_host_function_call_op( contract_id=contract_id, function="vote_yes", parameters=[] ).set_timeout(30).build() transaction.sign(keypair) response = server.submit_transaction(transaction) print(f"Voted yes: {response}") def get_results(): results = soroban_server.call_contract( contract_id=contract_id, function="get_results", parameters=[] ) print(f"Current results: {results}") if __name__ == "__main__": create_proposal("Implement Soroban in our Project") vote_yes() get_results()
Run the Script:
Execute the script using Python to create a proposal, vote yes, and retrieve results:
bashCopy codepython interact.py
Enhancements and Next Steps
This guide shows the basic steps to create, deploy, and interact with a decentralized voting contract using Soroban and Python. Here are some enhancements to consider:
User Interface: Integrate the backend with a web interface using Flask or Django for user-friendly interaction.
Authentication: Use Stellar's federated authentication to ensure that only authorized users can vote.
Multiple Proposals: Modify the smart contract to handle and track multiple proposals simultaneously.
Advanced Analytics: Integrate with data visualization tools to show real-time voting results and trends.
Conclusion
Using Soroban with Python provides a powerful and straightforward way to develop decentralized applications. This decentralized voting system can serve as a foundational project for more complex applications, including decentralized finance (DeFi), supply chain tracking, and more. By leveraging Soroban and Python, you are tapping into the future of secure and transparent technology.
This article gives a comprehensive walkthrough for building a decentralized voting application using Soroban and Python, complete with code snippets for creating, deploying, and interacting with the smart contract. It's a practical starting point for Python developers to get hands-on experience with blockchain technology.
More Stellar Docs - https://developers.stellar.org/docs
Subscribe to my newsletter
Read articles from Vansh Goyal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
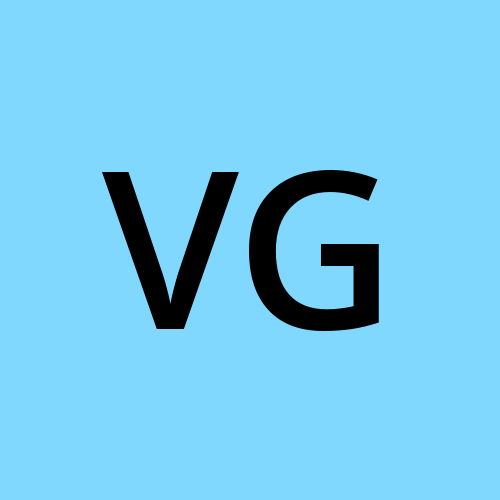