Publishing Your Flutter App: A Comprehensive Guide
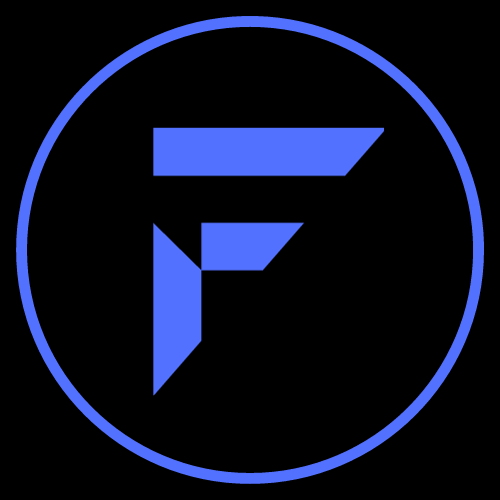
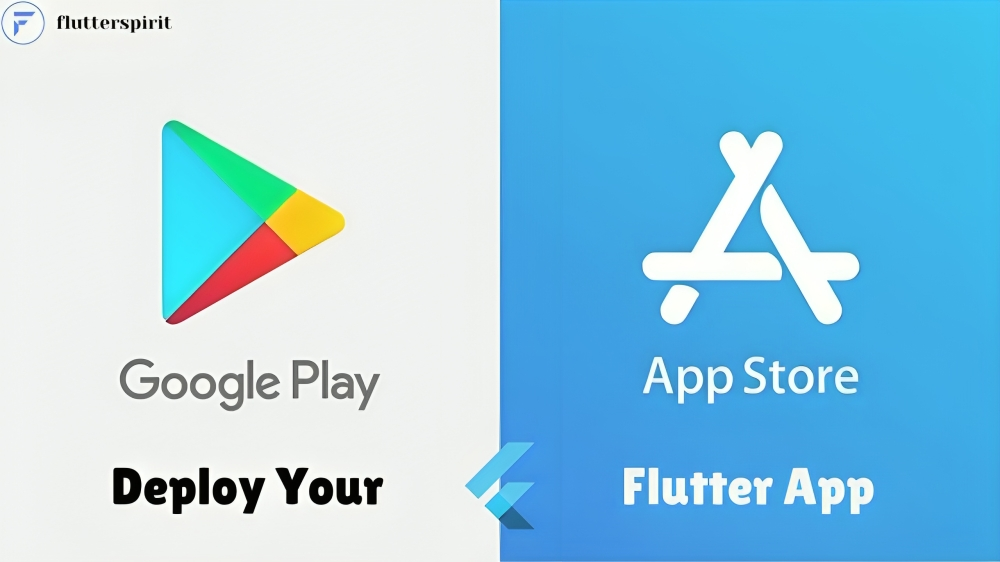
Introduction
Publishing a Flutter app can be an exciting milestone, marking the completion of your hard work. Whether you're aiming to release your app on Google Play, the Apple App Store, or both, the process involves several steps. This guide will walk you through the entire process, from preparing your app for release to successfully publishing it on the respective platforms.
1. Preparing Your Flutter App for Release
Before diving into the publishing process, it's crucial to prepare your app for the production environment. Here are the key steps involved:
1.1 Remove Debugging Code
First, ensure that all debugging code is removed from your app. This includes print()
statements, debugging libraries, and any other code that is not necessary for production.
// Remove any debugging code
print('Debugging information'); // Remove this line
1.2 Minimize Your App Size
Flutter provides tools to help you minimize your app's size, which is essential for a good user experience and to avoid exceeding the size limits set by app stores.
Tree Shaking: Flutter automatically removes unused code (tree shaking) when building a release version.
Using ProGuard (for Android): ProGuard can be used to further reduce your app's size by obfuscating code and removing unused resources.
To enable ProGuard, add the following to your android/app/build.gradle
file:
buildTypes {
release {
// Enable ProGuard to shrink, obfuscate, and optimize the code
minifyEnabled true
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
1.3 Configure App Permissions
Ensure that your app only requests the permissions it truly needs. Excessive permissions can lead to user distrust and potential app store rejection.
Check and update the AndroidManifest.xml
file for Android and the Info.plist
file for iOS to ensure that only necessary permissions are requested.
<!-- AndroidManifest.xml -->
<uses-permission android:name="android.permission.INTERNET" />
<!-- Remove unnecessary permissions -->
<!-- Info.plist -->
<key>NSCameraUsageDescription</key>
<string>We need access to your camera to take photos</string>
<!-- Only include necessary permissions -->
1.4 Set Up App Icons and Launch Screens
Your app icon is the first thing users see, so it needs to be professional and consistent across platforms.
App Icon:
For Android, update the app icon by placing your icon files in the
android/app/src/main/res/mipmap-
directories.For iOS, place your icon files in the
ios/Runner/Assets.xcassets/AppIcon.appiconset/
directory.
Launch Screen:
For Android, configure the launch screen in
android/app/src/main/res/drawable/launch_background.xml
.For iOS, configure the launch screen in the
ios/Runner/Assets.xcassets/LaunchImage.imageset/
directory.
1.5 Versioning
Proper versioning is crucial for managing updates and ensuring users are on the latest version of your app.
In the pubspec.yaml
file, update the version
field:
version: 1.0.0+1
Here, 1.0.0
is the version number, and +1
is the build number.
2. Building the Release Version of Your App
2.1 Setting Up Your Environment for Android
Before you begin the deployment process, ensure your development environment is properly set up.
2.1.1 Install Android Studio
Android Studio is the official IDE for Android development and comes with the Android SDK, which is required for building and deploying Android apps.
Download and Install Android Studio:
Download Android Studio from here.
Install it and set up the Android SDK by following the installation prompts.
2.1.2 Configure the Android SDK
After installing Android Studio, you need to configure the Android SDK:
Open Android Studio and navigate to:
Preferences > Appearance & Behavior > System Settings > Android SDK
.Install SDK Tools: Ensure the following tools are installed:
Android SDK Build-Tools
Android SDK Platform-Tools
Android SDK Command-Line Tools
Set up Emulator (optional): You can set up an Android Emulator to test your app on virtual devices.
2.2 Configuring Your Flutter Project for Android
Before building your Android app for release, several configurations need to be set up.
2.2.1 Set the Application ID
The application ID is a unique identifier for your app on the Google Play Store.
Open
android/app/build.gradle
.Set the
applicationId
:
gradleCopy codeandroid {
defaultConfig {
applicationId "com.yourcompany.yourappname"
}
}
2.2.2 Set Up App Signing
Android apps need to be signed with a private key before they can be released. This key is used to verify the authenticity of the app.
- Generate a Keystore:
bashCopy codekeytool -genkey -v -keystore <your-key-name>.jks -keyalg RSA -keysize 2048 -validity 10000 -alias <your-alias-name>
- Create a
key.properties
file:
propertiesCopy codestorePassword=<your-store-password>
keyPassword=<your-key-password>
keyAlias=<your-alias-name>
storeFile=<path-to-your-keystore.jks>
- Configure
build.gradle
to use this keystore:
gradleCopy codeandroid {
signingConfigs {
release {
keyAlias keystoreProperties['keyAlias']
keyPassword keystoreProperties['keyPassword']
storeFile file(keystoreProperties['storeFile'])
storePassword keystoreProperties['storePassword']
}
}
buildTypes {
release {
signingConfig signingConfigs.release
}
}
}
2.2.3 Configure ProGuard
ProGuard helps optimize your app by shrinking and obfuscating the code. This reduces the APK size and makes reverse engineering more difficult.
- Enable ProGuard in
build.gradle
:
gradleCopy codeandroid {
buildTypes {
release {
minifyEnabled true
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
}
- Customize ProGuard rules in
proguard-rules.pro
: You may need to add rules to keep certain classes or methods from being obfuscated, especially if you're using reflection or native libraries.
2.3 Building the Release APK or AAB
After configuring your project, you can build the release version of your app.
2.3.1 Building an APK
An APK is the package file format used by Android for the distribution and installation of apps.
- Build a release APK:
bashCopy codeflutter build apk --release
- Output: The APK will be located in the
build/app/outputs/flutter-apk/
directory.
2.3.2 Building an AAB
An Android App Bundle (AAB) is a publishing format that includes all your app’s compiled code and resources, but defers APK generation and signing to Google Play.
- Build a release AAB:
bashCopy codeflutter build appbundle --release
- Output: The AAB will be located in the
build/app/outputs/bundle/
directory.
2.4 Testing Your Release Build
Before uploading your app to the Play Store, it's essential to test the release build.
2.4.1 Install the APK on a Device
You can directly install the APK on a physical Android device to test:
bashCopy codeflutter install --release
2.4.2 Test on an Emulator
You can also test the release build on an Android emulator:
bashCopy codeflutter emulators --launch <emulator-id>
flutter install --release
3.1 Uploading to Google Play Store
3.1 Create a Google Play Developer Account
To publish your app, you'll need a Google Play Developer account, which requires a one-time registration fee of $25.
- Register: Sign up at the Google Play Console.
3.2 Create a New App Listing
Go to the Google Play Console and click "Create App."
Fill in the required details:
App name
Default language
Application type (app or game)
Category (choose the appropriate category)
Provide your email and website (optional)
3.3 Prepare Store Listing
Title and Description: Write an engaging title and description for your app.
Screenshots: Upload at least two screenshots for different screen sizes (phone, tablet, etc.).
Feature Graphic: Upload a feature graphic (1024 x 500 pixels).
App Icon: Upload your app icon.
Privacy Policy: Provide a URL to your privacy policy.
3.4 Upload Your APK or AAB
In the "Release" section of the Play Console:
Create a new release under the "Production" track.
Upload your APK or AAB and provide release notes.
3.5 Set Pricing and Distribution
Set the price: Choose whether your app is free or paid.
Select countries: Specify the countries where your app will be available.
Distribution: Select whether your app will be available on Google Play for Android TV, Wear OS, or other platforms.
3.6 Submit for Review
Once everything is set up, click on "Review" and then "Start Rollout to Production." Your app will be reviewed by Google, which can take a few hours to several days.
4. Publishing Your App to the Apple App Store
4.1 Create a Developer Account
To publish on the Apple App Store, you need an Apple Developer account, which costs $99 per year. You can sign up here.
4.2 Prepare Your App Store Listing
Similar to Google Play, you'll need to prepare your App Store listing:
App Name and Description: Write a compelling app name and description.
Screenshots: Upload screenshots for different devices (iPhone, iPad, etc.). Ensure they are high-quality.
App Icon: Upload the same app icon used in your app.
Keywords: Add relevant keywords to help users find your app.
Category: Choose the appropriate category for your app.
Content Rating: Complete the content rating questionnaire.
Privacy Policy: Provide a link to your privacy policy.
4.3 Upload Your App Using Xcode
Once your listing is ready, you can upload your app using Xcode:
Open your Flutter project's iOS directory in Xcode.
Select your project in the Project Navigator.
Go to the "Signing & Capabilities" tab and ensure that your app is properly signed.
Select "Product" > "Archive" from the menu to create an archive of your app.
Once the archive is created, select "Distribute App" and choose the "App Store Connect" option.
4.4 Configure App Store Connect
In App Store Connect, you need to configure your app's metadata, including its version number, build number, and any additional information required by Apple.
4.5 Test Your App with TestFlight
Before releasing your app to the public, you can test it using TestFlight. This allows you to distribute your app to beta testers and receive feedback.
Create a TestFlight build: In App Store Connect, select your uploaded build and enable TestFlight.
Invite testers: Add testers by their email addresses, and they will receive an invitation to download your app via the TestFlight app.
4.6 Submit Your App for Review
Once you're confident that your app is ready for release, you can submit it for review. Apple manually reviews all apps, so the process can take several days.
4.7 Monitor the Review Process
After submitting your app, monitor the review process in App Store Connect. If any issues are found, Apple will provide feedback, which you'll need to address before your app can be published.
5. Post-Release Management
5.1 Monitor App Performance
After your app is published, it's important to monitor its performance. Both Google Play and the Apple
App Store provide analytics tools to track downloads, user ratings, and other key metrics.
5.2 Respond to User Feedback
Engage with your users by responding to reviews and addressing any issues they report. Positive interaction can lead to better ratings and increased downloads.
5.3 Release Updates
Keep your app updated with bug fixes, new features, and improvements. Regular updates show users that the app is actively maintained and can improve your app's visibility in the app stores.
5.4 Marketing Your App
Publishing is just the beginning. To ensure your app reaches its full potential, you'll need to market it effectively. Consider using social media, content marketing, and paid advertising to increase visibility and downloads.
Conclusion
Publishing a Flutter app involves several steps, from preparing the app to releasing it on the Google Play Store and the Apple App Store. By following this comprehensive guide, you can ensure that your app is well-prepared for production and successfully published on both platforms.
Remember, the journey doesn't end with publishing. Continuously monitor your app's performance, respond to user feedback, and release updates to keep your app relevant and successful. Happy coding!
Got an App Idea? Let's Bring Your MVP to Life!
Contact us at Flutter Your Way
Subscribe to my newsletter
Read articles from Aditya Chauhan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
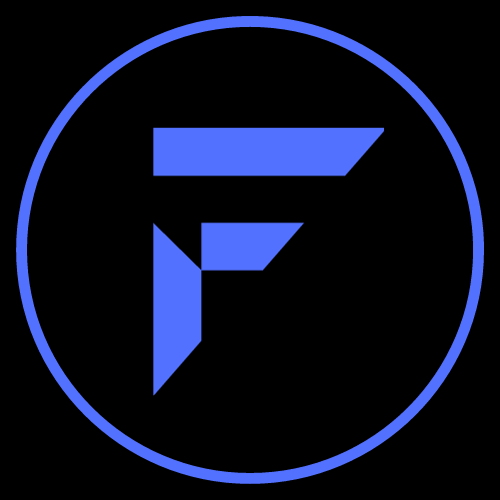
Aditya Chauhan
Aditya Chauhan
I am a highly skilled and motivated designer and programmer, passionate about creating innovative tech solutions that positively impact lives. My expertise spans advanced frameworks and technologies like Flutter, Next.js, Express.js, and React, along with programming languages such as C and Java. I also have strong database management skills, including MySQL, MongoDB, SQLite, Firebase, and Supabase. Having completed my Diploma in Computer Science from GLA University, I continuously expand my skill set and expertise. My diverse project portfolio showcases my grasp of technical concepts and my ability to design and develop high-quality solutions that meet user needs. Notable projects include: Full-Fledged Travel Application: Developed in collaboration with the Ladakh government for tourists, featuring advanced options and a seamless user experience. Real-Time Chatting Application: Designed with impressive UI/UX and extensive features, creating a superior communication platform. Zaepste Social Media App: Enhanced user interaction with AI chatbots for various use cases. FoodMama: A food delivery app with a sleek UI/UX for fast and efficient ordering. These applications are available on both the Play Store and App Store, making them accessible to users worldwide. I have also developed smaller projects like hotel and student management systems, a notes app, and a to-do app using Flutter. Beyond my technical skills, I am an excellent communicator and collaborator, fluent in Hindi and English, which enables me to engage effectively with a diverse audience. My strong leadership skills have been recognized, allowing me to manage accounts and lead teams successfully. In addition to my development work, I am a social media influencer and currently serve as a senior Flutter developer and team lead at Flutter Your Way Agency. I run an Instagram page called "flutterspirit," where I share UI/UX content and tech skill improvement videos, inspiring others to explore technology. My LinkedIn profile has over 3k followers, where I share similar insights, leading to numerous projects and freelance opportunities and i also committed to continuous learning, always seeking new technologies and frameworks to push the boundaries of what is possible. . In summary, I am a talented and motivated designer and programmer with a proven track record of success. My broad range of technical skills and experience makes me an excellent choice for any project or team. I am eager to take on new challenges and continue pushing the boundaries of technology