Create a Python Script to Monitor Website Uptime and Send Alerts
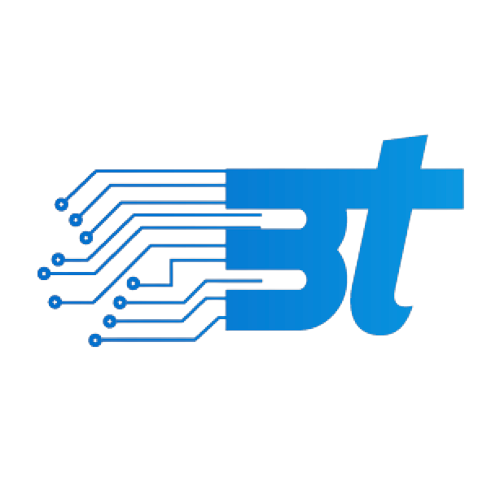
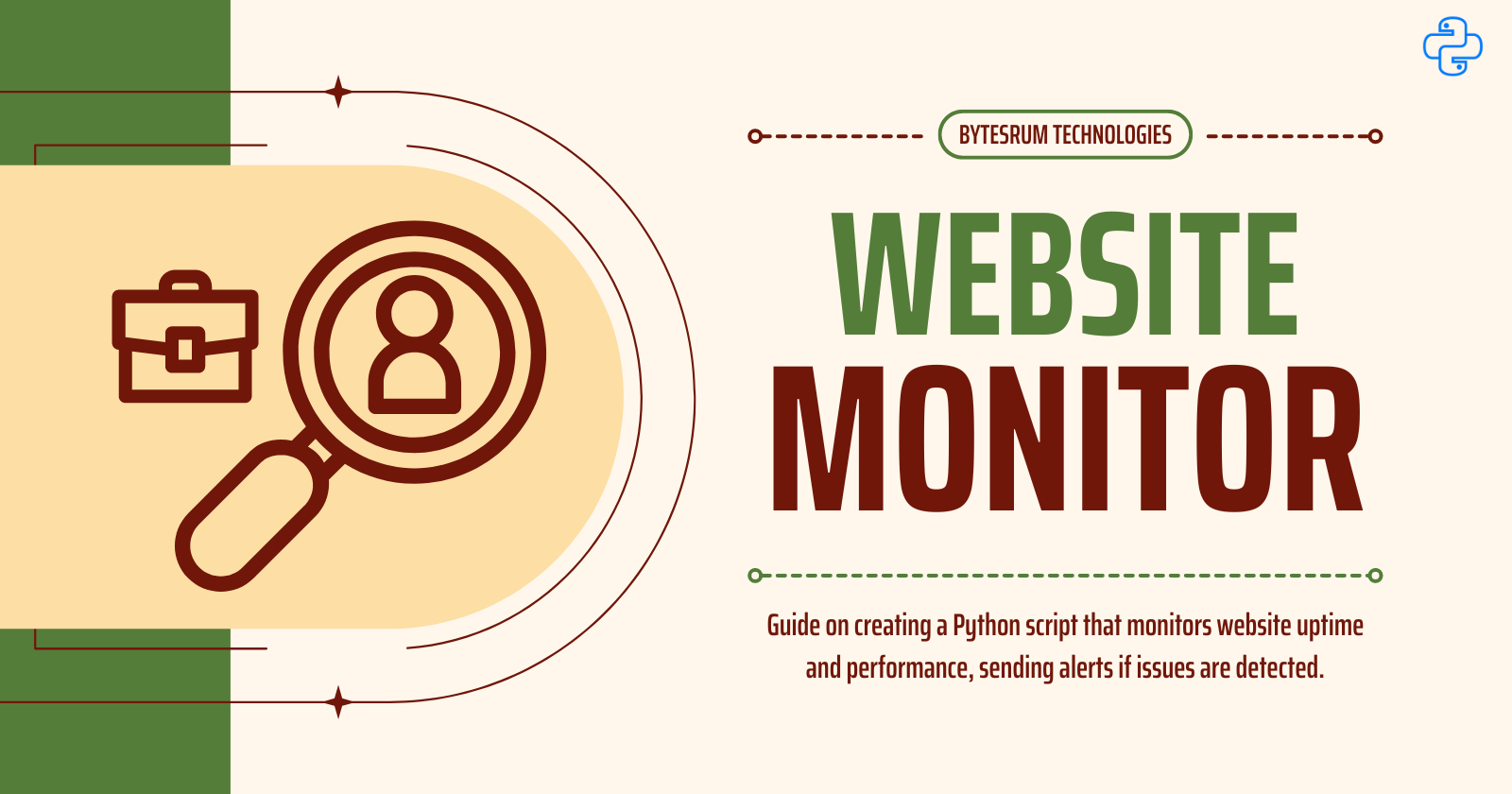
In today's digital landscape, website performance is critical for maintaining user engagement and ensuring a smooth experience. Slow or unresponsive websites can lead to decreased user satisfaction and potential revenue loss. To combat this, you can create a Python tool to monitor website performance and send alerts when issues are detected. This tool will periodically check various performance metrics like response time, uptime, and error rates, and notify you via email or other communication channels if performance falls below acceptable levels.
Key Features of the Python Tool
URL Monitoring: Monitor multiple websites or specific URLs to ensure they are up and running.
Performance Metrics: Measure key performance metrics such as response time, HTTP status codes, and uptime.
Alerts and Notifications: Send alerts via email or messaging services like Slack or SMS when performance issues are detected.
Customizable Check Intervals: Set custom intervals for checking website performance.
Logging and Reporting: Log performance data for historical analysis and generate performance reports.
Let's walk through how to build this tool step-by-step.
Step 1: Setting Up the Environment
First, make sure you have Python installed along with some necessary libraries:
pip install requests smtplib schedule
Step 2: Define the Monitoring Function
We'll create a function to check the performance of a website by sending HTTP requests and recording response times.
import requests
from datetime import datetime
def check_website_performance(url):
try:
# Send a GET request to the website
response = requests.get(url, timeout=10)
# Measure response time
response_time = response.elapsed.total_seconds()
# Check the status code
if response.status_code == 200:
print(f"[{datetime.now()}] {url} is up. Response time: {response_time} seconds.")
else:
print(f"[{datetime.now()}] {url} is down. Status code: {response.status_code}.")
return False, response_time
return True, response_time
except requests.exceptions.RequestException as e:
# Handle exceptions like connection errors, timeouts, etc.
print(f"[{datetime.now()}] Error checking {url}: {e}")
return False, None
Step 3: Set Up Alerts
Let's set up an email alert system that sends notifications if a website is down or slow. You can use an SMTP server to send emails. Here’s a simple example:
import smtplib
from email.mime.text import MIMEText
def send_email_alert(subject, body, to_email):
from_email = 'your-email@example.com'
password = 'your-email-password'
msg = MIMEText(body)
msg['Subject'] = subject
msg['From'] = from_email
msg['To'] = to_email
try:
# Connect to the SMTP server
with smtplib.SMTP('smtp.example.com', 587) as server:
server.starttls() # Upgrade the connection to a secure encrypted SSL/TLS connection
server.login(from_email, password)
server.sendmail(from_email, to_email, msg.as_string())
print("Alert email sent successfully!")
except Exception as e:
print(f"Failed to send email: {e}")
Step 4: Integrate the Monitoring and Alert System
Now, we need to combine the monitoring function with the alert system to create a complete monitoring tool.
def monitor_websites(websites, threshold):
for url in websites:
is_up, response_time = check_website_performance(url)
if not is_up or (response_time is not None and response_time > threshold):
# Prepare email alert
subject = f"Website Performance Alert: {url}"
body = f"Website {url} is down or slow. Response time: {response_time} seconds."
send_email_alert(subject, body, 'recipient-email@example.com')
websites_to_monitor = [
'https://www.example.com',
'https://www.another-example.com'
]
response_time_threshold = 2.0 # in seconds
monitor_websites(websites_to_monitor, response_time_threshold)
Step 5: Automate the Monitoring with a Scheduler
To continuously monitor the websites, we can use the schedule
library to run the monitoring function at regular intervals.
import schedule
import time
# Define the monitoring schedule
schedule.every(5).minutes.do(monitor_websites, websites=websites_to_monitor, threshold=response_time_threshold)
while True:
schedule.run_pending()
time.sleep(1)
Step 6: Run the Python Tool
Save the script and run it in your terminal:
python website_monitor.py
Conclusion
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
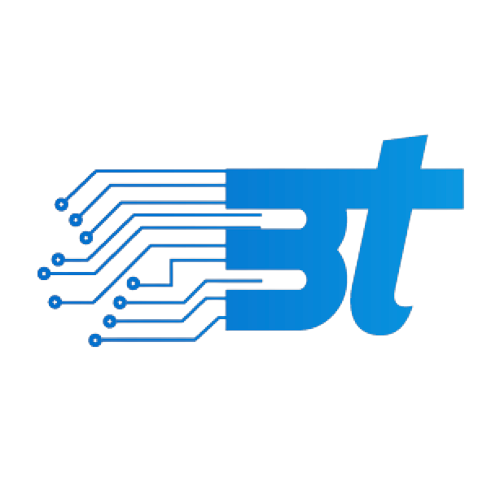
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.