Day 17 Task: Docker Project for DevOps Engineers

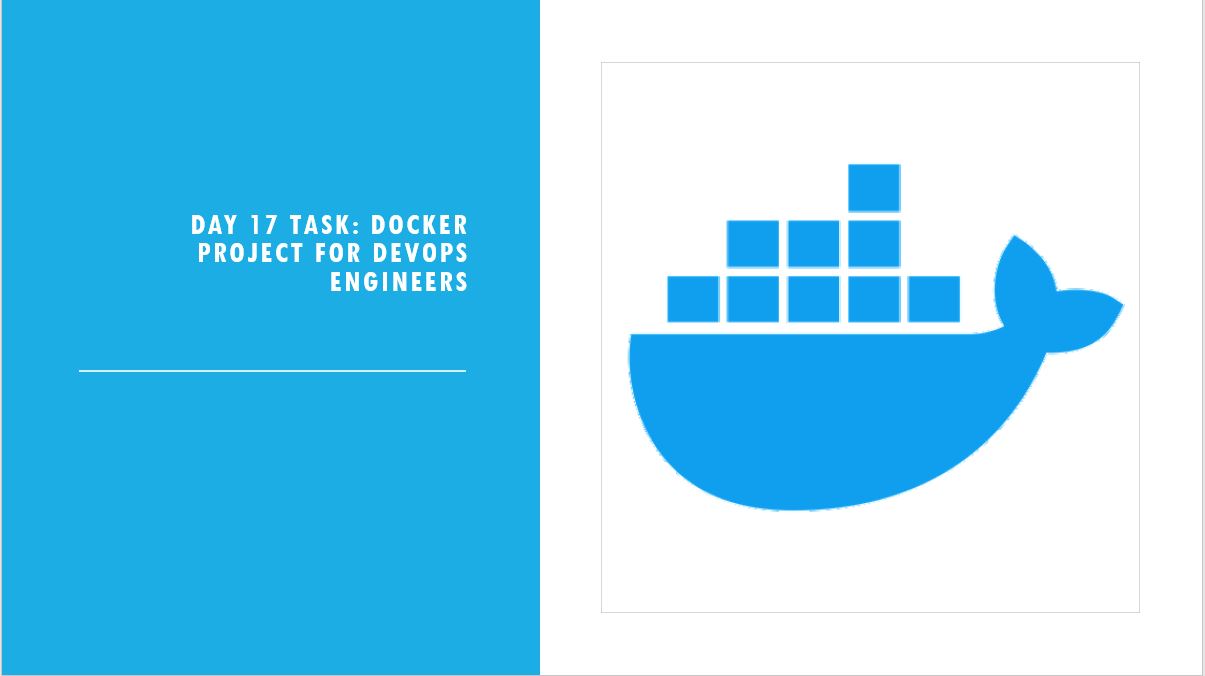
Dockerfile
Docker is a tool that makes it easy to run applications in containers. Containers are like small packages that hold everything an application needs to run. To create these containers, developers use something called a Dockerfile.
A Dockerfile is like a set of instructions for making a container. It tells Docker what base image to use, what commands to run, and what files to include. For example, if you were making a container for a website, the Dockerfile might tell Docker to use an official web server image, copy the files for your website into the container, and start the web server when the container starts.
Dockerfile commands/Instructions
FROM - Represents the base image(OS), which is the command that is executed first before any other commands.
WORKDIR - The WORKDIR is used to set the working directory for any RUN, CMD and COPY instruction that follows it in the Dockerfile. If work directory does not exist, it will be created by default.
COPY - The copy command is used to copy the file/folders to the image while building the image.
RUN - Scripts and commands are run with the RUN instruction. The execution of RUN commands or instructions will take place while you create an image on top of the prior layers (Image).
CMD - The main purpose of the CMD command is to start the process inside the container and it can be overridden.
Stages of Creating Docker Image from Dockerfile
The following are the stages of creating docker image form Dockerfile:
Create a file named Dockerfile.
Add instructions in Dockerfile.
Build Dockerfile to create an image.
Run the image to create a container.
Task
- Create a Dockerfile for a simple web application (e.g. a Node.js or Python app)
# Use an official Python runtime as a parent image
FROM python:3.8-slim
# Set the working directory in the container
WORKDIR /app
# Copy the current directory contents into the container at /app
COPY . /app
# Install any needed packages specified in requirements.txt
RUN pip install --no-cache-dir Werkzeug==2.0.3
# Install any needed packages specified in requirements.txt
RUN pip install --no-cache-dir -r requirement.txt
# Make port 5000 available to the world outside this container
EXPOSE 5000
# Run app.py when the container launches
CMD ["python", "app.py"]
- Build the image using the Dockerfile and run the container.
Docker Image - These are like blueprints for containers. An image contains all the instructions for creating a container. Think of it as a recipe that tells Docker how to build your application’s environment.
Docker Container - Imagine a container as a box that holds everything your application needs to run—like the code, libraries, and system tools. This way, your application can run the same way on any machine, regardless of the environment.
How to build an image in docker -
docker build -t resume-analyser .
docker images
How to run the container in docker -
docker run -d -p 5000:5000 resume-analyser
How to check running container in system -
docker ps -a
Verify that the application is working as expected by accessing it in a web browser
Go the instance details and select security and select security group to add the rules for the port of the flask app
- In security group select inbound rules annd edit it to add the new rules for the port 5000
- After adding rules for the port 5000 copy your private ip address and paste in the web browser and it will run your web application deployed from docker.
After running the ip address we can see the resume analyser application has been run or deployed successfully by docker tool.
Push the image to a public or private repository (e.g. Docker Hub)
Open hub.docker.com (Docker Hub) and create an account on it.
Go to the account setting to generate the Personal access token (PAT) for push local image to public repository.
- Click on generate new token and fill out the required field to create a PAT, make sure you select read,write,delete permission while creating PAT for push your local image to public or private repository.
- Go to your terminal and write command:
docker login
It will ask username and password give the credentials:
- Now tag your image you want to push the public or private repository with the following command:
docker image tag twotier_app:latest vibhutijain12/twotier_app:latest
and check your image tagged or not in docker images
$docker images
REPOSITORY TAG IMAGE ID CREATED SIZE
twotier_app latest 7718ef1f8871 6 hours ago 413MB
vibhutijain12/twotier_app latest 7718ef1f8871 6 hours ago 413MB
python 3.9-slim d8892906392f 5 weeks ago 125MB
mysql 5.7 5107333e08a8 8 months ago 501MB
Image has tagged, now we can push our image to public or private repository.
- Push Image to the docker hub repository:
$docker push vibhutijain12/twotier_app:latest
The push refers to repository [docker.io/vibhutijain12/twotier_app]
787d74f0266b: Pushed
434718541c5f: Pushed
4924633d0372: Pushed
911f3eeb9bd0: Pushed
310d49379ea7: Pushed
dccd55e6ee29: Pushed
d24f9dbb0a3a: Mounted from library/python
8657193c8651: Mounted from library/python
0900caae955e: Mounted from library/python
414698da489a: Mounted from library/python
9853575bc4f9: Mounted from library/python
latest: digest: sha256:c5bee8e16044bb8aa62ce15c36599f778a75a4da679bddc8315752e034703161 size: 2626
- You can check your docker hub account your pushed will be stored there.
Follow for more updates:)
Subscribe to my newsletter
Read articles from Vibhuti Jain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vibhuti Jain
Vibhuti Jain
Hi, I am Vibhuti Jain. A Devops Tools enthusiastic who keens on learning Devops tools and want to contribute my knowledge to build a community and collaborate with them. I have a acquired a knowledge of Linux, Shell Scripting, Python, Git, GitHub, AWS Cloud and ready to learn a lot more new Devops skills which help me build some real life project.