Git for Beginners: A Friendly Guide to Version Control

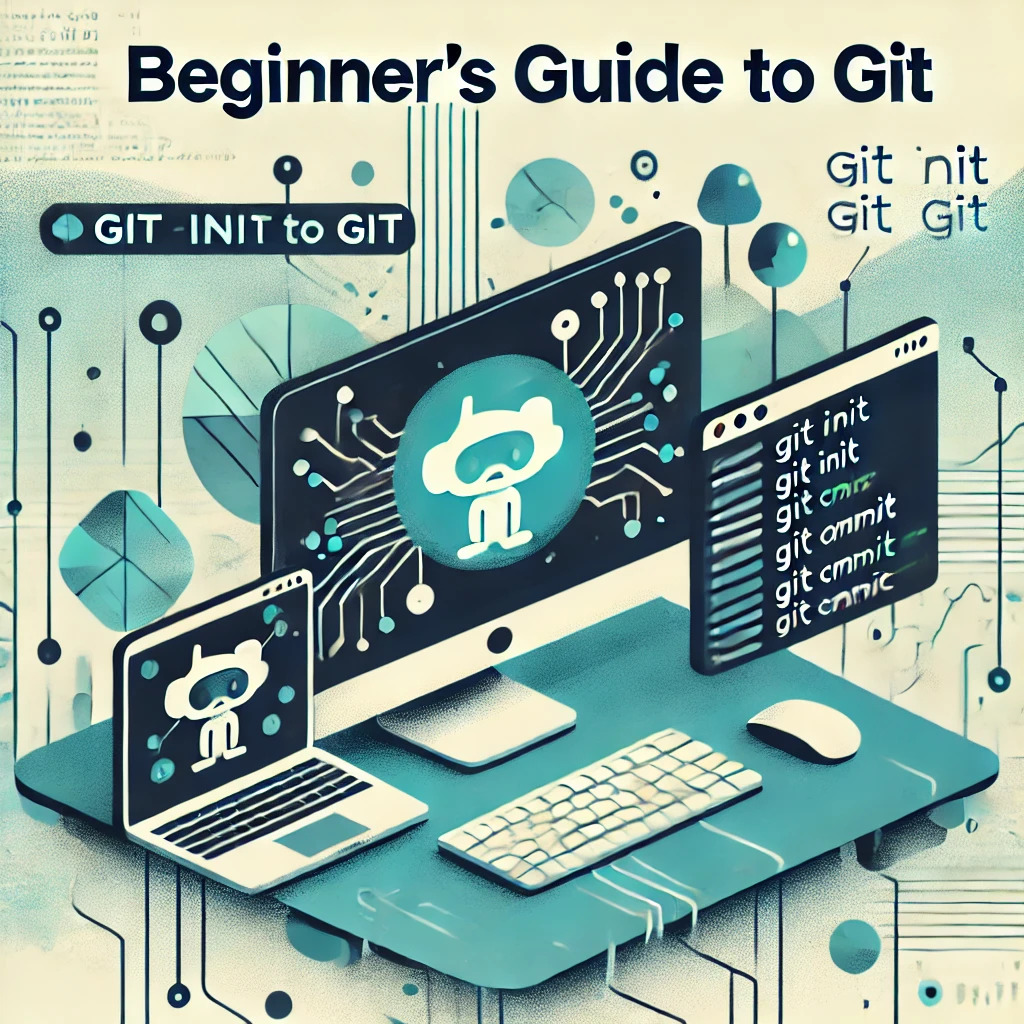
Are you new to programming and keep hearing about this thing called Git? Don't worry! This guide will walk you through the basics of Git, a powerful tool that helps you manage your code and collaborate with others. Let's dive in!
What is Git?
Git is a version control system. Think of it as a time machine for your code. It allows you to save different versions of your project, go back in time if you make a mistake, and work with others without stepping on each other's toes.
Getting Started
Setting Up Your Identity
Before you start using Git, you should tell it who you are. This information is used when you make changes to your project. Here's how to do it:
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
Replace "Your Name"
and "
your.email@example.com
"
with your actual name and email.
Checking Your Settings
To make sure everything is set up correctly, you can use:
git config user.name
git config user.email
This will show you your configured name and email.
To see all your Git configurations, use:
git config --list
Basic Git Commands
Initializing a Git Repository
If you want to initialize Git in your project, navigate to your project directory and use:
git init
Checking the Status of Your Project
git status
This shows you what files have been changed, what's ready to be saved (or "committed" in Git-speak), and what files Git doesn't know about yet.
Saving Your Changes
When you're ready to save your work, you'll use these commands:
git add .
- This tells Git to start tracking all the files you've changed.git commit -m "Your message here"
- This saves your changes with a short description.
Viewing Your History
To see a list of all the changes you've saved, use:
git log
For a more compact view, try:
git log --oneline
Oops! Undoing Changes
Unstage a File
If no commit has been made yet, use:
git rm --cached filename.txt
If a commit is complete and you want to unstage a file:
git restore --staged filename.txt
Undo a Commit
To undo a commit:
Use
git log
to get all the commit IDs, then copy thecommit_id
you want to go back to.Use
git reset <commit_id>
. Boom! You have reverted to your previous code in an un-staged area.
Other Undo Commands
To discard all unstaged changes:
git restore .
To discard changes in a specific file:
git restore filename.txt
To unstage files (undo
git add
):git reset
To undo your last commit but keep the changes staged:
git reset --soft HEAD^
To undo your last commit and unstage the changes:
git reset HEAD^
To undo your last commit and discard all changes:
git reset --hard HEAD^
(Use with caution!)
Comparing Changes
To see the differences between your working directory and the staging area:
git diff
Viewing Commit Details
To see details of a specific commit:
git show commit_id
Working with Branches
Branches let you work on different versions of your project at the same time. Think of them as parallel universes for your code.
To create a new branch:
git checkout -b new-branch-name
To switch between branches:
git checkout branch-name
To see all branches:
git branch -a
To see remote branches:
git branch -r
To delete a branch:
git branch -d branch-name
Stashing: A Temporary Drawer for Your Changes
If you need to switch tasks quickly but aren't ready to commit your changes, you can use Git's "stash" feature:
To stash your changes:
git stash
To include untracked files in the stash:
git stash --include-untracked
To see your list of stashes:
git stash list
To apply your most recent stashed changes:
git stash pop
To apply a specific stash:
git stash pop stash@{number}
To clear all stashes:
git stash clear
Advanced Tips
Reverting Pulls
If you've pulled changes you don't want, you can revert the pull:
git reset --hard HEAD^
Be careful with this command as it discards changes!
Downloading Specific Branches
To download and switch to a specific remote branch:
git checkout -t origin/remote-branch-name
Merging Branches
To merge changes from one branch into another:
- First, switch to the target branch (e.g.,
main
):
git checkout main
- Merge the desired branch:
git merge <branchName>
Cherry-Picking Commits
Suppose you have two branches feature-one and feature-two, and you made a commit to feature-one but it should have been committed to feature-two. You can use cherry-pick to move that commit to feature-two.
Cherry-picking allows you to apply specific commits from one branch to another:
git cherry-pick <commit_id>
Working with Remote Repositories
When collaborating with others or managing your projects, you'll often need to interact with a remote repository, which is a version of your project hosted on the internet or a network. This allows multiple people to work on the same project from different locations.
Cloning a Remote Repository
To get a copy of a remote repository on your local machine, you need to clone it. Cloning creates a local copy of the repository, including all its files, commit history, and branches.
git clone <repository-url>
Replace <repository-url>
with the URL of the remote repository. For example:
git clone https://github.com/user/example-repo.git
Fetching Updates from a Remote Repository
Fetching is the process of downloading the latest changes from the remote repository to your local machine without merging them into your current branch. This allows you to see what others have been working on.
git fetch
After running git fetch
, you'll have the latest commits from the remote repository in your local repository. However, your working directory will remain unchanged until you merge these changes.
To fetch updates from a specific remote branch, use:
git fetch origin <branch-name>
Pulling Changes from a Remote Repository
Pulling is a combination of fetching and merging. When you pull from a remote repository, Git fetches the latest changes and automatically merges them into your current branch.
git pull
This command is handy when you want to update your local branch with the latest changes from the remote branch you are tracking.
To pull changes from a specific branch:
git pull origin <branch-name>
Pushing Changes to a Remote Repository
Once you've made changes to your local repository, you may want to share them with others by pushing them to a remote repository. Pushing updates the remote branch with your local commits.
git push origin <branch-name>
Replace <branch-name>
with the name of the branch you want to push to. For example, to push changes to the main
branch:
git push origin main
If you're pushing to a new branch that doesn't exist on the remote repository, Git will create it for you.
Viewing Remote Branches
To see all remote branches, you can use:
git branch -r
This command will list all branches available on the remote repository.
To see both local and remote branches:
git branch -a
Adding a Remote Repository
If you need to connect your local repository to a new remote repository, you can add it using the git remote add
command:
git remote add origin <repository-url>
Replace <repository-url>
with the URL of your remote repository.
Removing a Remote Repository
If you no longer need to work with a particular remote repository, you can remove it using:
git remote remove <remote-name>
Usually, <remote-name>
is origin
, which is the default name for the remote repository when you clone it.
Conclusion
Congratulations! You now have a comprehensive understanding of Git basics. Remember, Git is a powerful tool with many features, and it's okay to learn them gradually. The more you use Git, the more comfortable you'll become with it.
Happy coding, and may your commits always be meaningful!
Subscribe to my newsletter
Read articles from Sourav Saha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
