Visualizing Data with Seaborn in Python

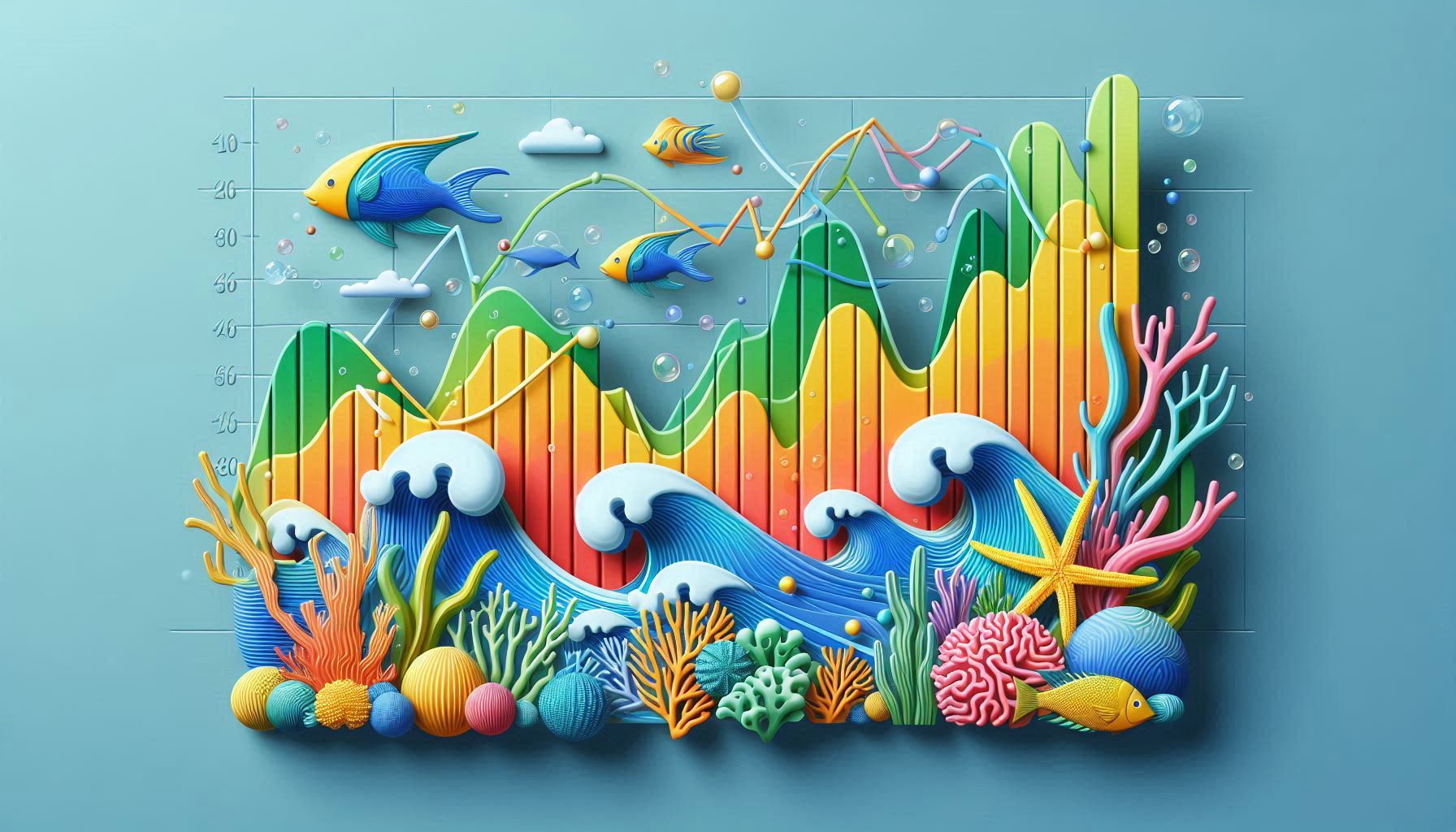
Seaborn is a powerful data visualization library built on top of Matplotlib in Python. It provides a high-level interface for drawing attractive and informative statistical graphics. In this blog post, we will explore some of the most commonly used Seaborn functions with examples.
1. Importing Seaborn
To use Seaborn in your project, you first need to import it. The standard convention is to import Seaborn as sns
:
import seaborn as sns
2. Boxplot
A boxplot displays the distribution of data based on a five-number summary: minimum, first quartile, median, third quartile, and maximum.
sns.boxplot(x='column_name', y='column_name', data=table_name)
Example:
sns.boxplot(x='day', y='total_bill', data=tips)
This will create a boxplot to show the distribution of total bills across different days.
3. lmplot
The lmplot
function is used for scatter plots with an added regression line. This is useful for visualizing the linear relationship between two variables.
sns.lmplot(x='column_name', y='column_name', data=dataframe_name)
Example:
sns.lmplot(x='total_bill', y='tip', data=tips, ci=None)
This will plot a scatter plot with a regression line showing the relationship between total bill and tip. The ci=None
argument removes the confidence interval shade around the line.
4. Scatterplot
A scatter plot is used to show the relationship between two variables.
sns.scatterplot(x='column_name', y='column_name', data=dataframe_name)
Example:
sns.scatterplot(x='total_bill', y='tip', data=tips)
This will create a scatter plot to show the relationship between total bill and tip.
5. regplot
The regplot
function is similar to lmplot
but allows more customization. It is used to plot data and a linear regression model fit.
sns.regplot(x='column_name', y='column_name', data=dataframe_name, label='NameOfPlot')
Example:
sns.regplot(x='total_bill', y='tip', data=tips, label='Bill vs Tip')
This creates a scatter plot with a regression line labeled as "Bill vs Tip".
6. FacetGrid
FacetGrid
is used to create a grid of subplots based on the values of one or more categorical variables.
g = sns.FacetGrid(data=dataframe_name, col='column_name')
g.map(sns.histplot, 'x')
Example:
g = sns.FacetGrid(tips, col='time')
g.map(sns.histplot, 'total_bill')
This will create histograms of total bill amounts for lunch and dinner separately.
7. Heatmap
A heatmap is a data visualization technique that shows the magnitude of a phenomenon as color in two dimensions.
sns.heatmap(series, annot=True, cmap='coolwarm', fmt='.2f')
Example:
sns.heatmap(tips.corr(), annot=True, cmap='coolwarm', fmt='.2f')
This will create a heatmap showing the correlation matrix of the tips dataset with the correlation values annotated.
8. Pairplot
pairplot
creates a matrix of scatter plots for each pair of columns in the dataset, optionally with histograms on the diagonal.
sns.pairplot(df, hue='column_name', diag_kind='kde', kind='scatter')
Example:
sns.pairplot(tips, hue='sex', diag_kind='kde', kind='scatter')
This will create pair plots of all numerical columns in the tips dataset, with the points colored by the sex
column. The diagonal plots will be kernel density estimates.
9. Histplot
The histplot
function is used for creating histograms, which are a way of representing the distribution of data.
sns.histplot(data=series, bins=20, alpha=0.5)
Example:
sns.histplot(data=tips['total_bill'], bins=20, alpha=0.5)
This will create a histogram for the total_bill
column in the tips dataset.
Conclusion
Seaborn is a versatile library that provides a simple interface for creating beautiful visualizations. By using Seaborn, you can easily create plots that help in understanding your data better. With the examples provided, you can start exploring and customizing your own plots in Seaborn. For more detailed information, check out the Seaborn documentation.
Subscribe to my newsletter
Read articles from Emeron Marcelle directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Emeron Marcelle
Emeron Marcelle
As a doctoral scholar in Information Technology, I am deeply immersed in the world of artificial intelligence, with a specific focus on advancing the field. Fueled by a strong passion for Machine Learning and Artificial Intelligence, I am dedicated to acquiring the skills necessary to drive growth and innovation in this dynamic field. With a commitment to continuous learning and a desire to contribute innovative ideas, I am on a path to make meaningful contributions to the ever-evolving landscape of Machine Learning.