"Comprehensive Guide to HTML Tables, iFrames, and Forms: Practical Examples and Best Practices"
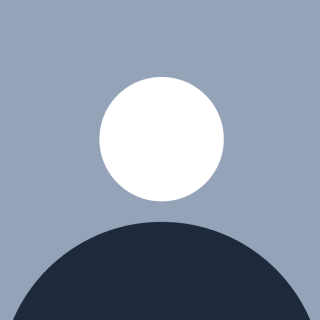
Table of contents
- 1. What is a Table in HTML?
- 2. Table Without Inner Borders
- 3. Assignment: Created School Timetable
- 4. What is an Iframe?
- 5. Assignment 2: Created a Webpage with Iframe and Table
- 6. Assignment 3: Embedded Audio and Video in a Webpage
- 7.Semantics in HTML: Understanding and Applying Meaningful Markup
- Conclusion
1. What is a Table in HTML?
In HTML, a table is a structured way of displaying data in rows and columns. It is created using the <table>
tag, with rows defined by the <tr>
(table row) tag, and columns defined by the <td>
(table data) tag. The <th>
tag is used to define headers within the table, which are often displayed in bold and centered by default. Tables are useful for organizing data that is best understood in a tabular format, such as schedules, pricing plans, or statistical data. For example, a weekly planner or a class schedule can be represented effectively using a table structure. Tables can be styled and customized using CSS to control borders, padding, and alignment, making them versatile for various design needs.
A table consists of several key elements:
<table>
: This tag defines the table itself.<tr>
: Stands for "table row" and is used to create a row within the table.<th>
: Stands for "table header" and is used to define header cells in the table. These cells are bold and centered by default, making them ideal for column headings.<td>
: Stands for "table data" and is used to define standard data cells within the table.
Tables are versatile and can be styled using CSS to enhance their appearance. For example, you can add borders, change colors, and adjust spacing to make the table more visually appealing. Additionally, you can use attributes such as colspan
and rowspan
to merge cells, which can be useful for organizing complex data.
2. Table Without Inner Borders
A table without inner borders in HTML simply means that there are no visible lines separating the rows and columns inside the table. To achieve this, you can either omit the CSS border property or set it to border: none;
for the <table>
, <tr>
, and <td>
elements. This creates a clean, minimalist table where only the data is visible, without any grid lines. However, if you need to add borders later, you can easily style them using CSS by adding border: 1px solid black;
or any other preferred styling. Tables without borders are often used in designs where the focus is on content rather than structure, such as email templates or basic data displays.
For example, in a table without inner borders, you would typically apply the following styles:
border
: Defines the border style, width, and color.border-collapse: collapse;
: Ensures that the table borders are combined into a single border rather than having separate borders for each cell.
This approach helps in creating a more streamlined and visually appealing table, especially when used in assignments such as creating a class timetable where clarity and simplicity are key.
3. Assignment: Created School Timetable
For this assignment, I created a school timetable using various HTML table properties. The table is structured with rows representing days of the week and columns representing time slots. Each cell contains the subject scheduled for that time slot. To enhance readability and aesthetics, I used CSS to add borders, collapse them to avoid double lines, and control the alignment of the content within the cells. The timetable also includes a lunch break row, spanning across multiple columns using the rowspan
attribute. This exercise helped reinforce the use of HTML tables for organizing structured data and the importance of CSS for styling and improving the user interface.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Class Time Table</title>
<style type="text/css">
table,th,td{
border: 1px solid black;
border-collapse: collapse;
}
</style>
</head>
<body>
<h1>CLASS TIME TABLE</h1>
<div style="width: 100%;">
<table>
<tr>
<th>day/hour</th>
<th>8-9</th>
<th>9-10</th>
<th>10-11</th>
<th>11-12</th>
<th>12-1</th>
<th>1-2</th>
<th>2-3</th>
<th>3-4</th>
</tr>
<tr>
<th>Monday</th>
<td>English</td>
<td>Hindi</td>
<td>Science1</td>
<td>Maths</td>
<td rowspan="7">LUNCH</td>
<td>PT</td>
<td>Sanskrit</td>
<td>History</td>
</tr>
<tr>
<th>Tuesday</th>
<td>English</td>
<td>Hindi</td>
<td>Science1</td>
<td>Maths</td>
<td>PT</td>
<td>Sanskrit</td>
<td>History</td>
</tr>
<tr>
<th>Wednesday</th>
<td>English</td>
<td>Marathi</td>
<td>Science1</td>
<td>Sanskrit</td>
<td colspan="2" align="center">Maths</td>
<td>Science2</td>
</tr>
<tr>
<th>Thursday</th>
<td>English</td>
<td>Science2</td>
<td>Marathi</td>
<td>Maths</td>
<td>PT</td>
<td>Sanskrit</td>
<td>Geography</td>
</tr>
<tr>
<th>Friday</th>
<td>English</td>
<td>Science2</td>
<td>Marathi</td>
<td>Maths</td>
<td>PT</td>
<td>Sanskrit</td>
<td>Geography</td>
</tr>
<tr>
<th>Saturday</th>
<td>English</td>
<td>Hindi</td>
<td colspan="2">Science Practical</td>
<td> --- </td>
<td> --- </td>
<td> --- </td>
</tr>
</table>
</div>
</body>
</html>
4. What is an Iframe?
An iframe, or inline frame, is an HTML element that allows you to embed another HTML document within the current one. It is created using the <iframe>
tag and is commonly used to embed external content, such as videos, maps, or other web pages, directly into your webpage. The iframe tag supports various attributes, such as src
(which specifies the URL of the content to embed), height
, and width
to control the size, and frameborder
to manage the presence of borders around the frame. However, simply copying the HTML link of a page won’t work in an iframe. Instead, you need to use the embed code provided by the source, which usually includes additional parameters for controlling the embedded content's behavior.
For instance, when embedding a YouTube video, you use the embed URL format provided by YouTube, which includes the video ID and optional parameters for customizing the player. This ensures the video player is properly embedded and functional within the webpage.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Iframe</title>
</head>
<body>
<h1 align="center">Hitesh Sir</h1>
<iframe src="https://www.youtube.com/embed/XmLOwJHFHf0?si=mg9pjpQXnEJy3mIs" frameborder="3" height="300" width="300" title="html"></iframe>
</body>
</html>
5. Assignment 2: Created a Webpage with Iframe and Table
In this assignment, I created a webpage that incorporates both an iframe and a table. The table's headings represent YouTube video titles, and the data cells contain embedded videos using the <iframe>
tag. This approach demonstrates how tables can be used to organize multimedia content effectively. By embedding videos directly into the table, users can view the content without leaving the page, enhancing the user experience. This assignment reinforced the concept of combining different HTML elements to create a more interactive and functional webpage.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Assignment2</title>
<style type="text/css">
table,th,td{
border: 1px solid black;
border-collapse: collapse;
}
</style>
</head>
<body>
<p>Hitesh Sir's Programming Videos</p>
<div style="width: 100%;">
<table>
<tr>
<th>HTML and VSCode -getting Started</th>
<th>Core Structure of HTML and Mega Tags</th>
<th>Heading,Paragraph and reading docs</th>
</tr>
<tr>
<td > <iframe src="https://www.youtube.com/embed/XmLOwJHFHf0?si=-r4uj1qtc_aEDcCS" frameborder="0"></iframe></td>
<td><iframe src="https://www.youtube.com/embed/v-xdY6VFcio?si=TmCgn7exu6H66jc7" frameborder="0"></iframe></td>
<td><iframe src="https://www.youtube.com/embed/CetKwpx3t6g?si=bTKapMEgW1RtvCtg" frameborder="0"></iframe></td>
</tr>
</table>
</div>
</body>
</html>
6. Assignment 3: Embedded Audio and Video in a Webpage
In this assignment, I embedded both audio and video elements into a webpage. The <audio>
and <video>
tags are used to embed multimedia files directly into HTML documents. These tags support various attributes like controls
(which adds play, pause, and volume controls), autoplay
, and loop
. Embedding multimedia content enhances user engagement and allows for a richer user experience. Additionally, this assignment included creating forms that collect user information, such as name, email, and skills. The form elements used include text inputs, checkboxes, radio buttons, and buttons for submission and resetting. Forms are essential for collecting user input and interacting with the backend of a web application
7.Semantics in HTML: Understanding and Applying Meaningful Markup
What is Semantic HTML?
Semantic HTML refers to the use of HTML tags that are not just used for presentation purposes, but also convey the meaning and structure of the content within them. Unlike non-semantic elements like <div>
and <span>
, which are purely used for layout and styling, semantic elements describe the role of the content they contain. For instance, tags like <header>
, <article>
, <section>
, and <footer>
provide clear information about the type of content or its position in the document.
Using semantic HTML enhances the accessibility of a webpage, improves search engine optimization (SEO), and makes the code easier to read and maintain. It helps search engines and assistive technologies (like screen readers) better understand the content, which can lead to better rankings and a more inclusive user experience.
Why is Semantic HTML Important?
Accessibility: Semantic HTML makes web content more accessible to users with disabilities. Screen readers and other assistive technologies rely on the semantic structure of a webpage to accurately convey its content. For example, a
<nav>
tag signals a navigation section, helping screen reader users easily navigate to the main menu.SEO (Search Engine Optimization): Search engines use the semantic structure of a webpage to better understand its content. By using semantic elements, you give search engines context about the content, which can improve how it is indexed and ranked. For instance, using
<article>
to wrap a blog post informs search engines that the content is a standalone piece of information.Readability and Maintenance: Semantic HTML improves the readability of the code for developers. When you use elements like
<header>
or<footer>
, it's immediately clear what their function is within the document. This clarity is crucial for maintaining and updating code, especially in larger projects with multiple contributors.
Common Semantic HTML Elements
Here are some of the most commonly used semantic HTML elements:
<header>
: Represents the introductory content or a group of navigational links for a section or page. It often contains a logo, title, or navigational elements like a menu.- Example: The
<header>
of a webpage might contain the website’s logo and the main navigation menu.
- Example: The
<nav>
: Represents a section of the page intended for navigation. This could include a list of links to other pages or sections within the same page.- Example: The
<nav>
element is typically used for primary site navigation.
- Example: The
<section>
: Defines a section of content that typically includes a heading. It is used to group related content together.- Example: A
<section>
might be used to group all articles on a particular topic within a webpage.
- Example: A
<article>
: Represents a self-contained composition in a document, page, or site, which is intended to be independently distributable or reusable.- Example: An individual blog post, a news article, or a forum post would be wrapped in an
<article>
tag.
- Example: An individual blog post, a news article, or a forum post would be wrapped in an
<aside>
: Represents content that is tangentially related to the content around it. It is often used for sidebars, pull quotes, or related links.- Example: A sidebar containing related articles or a list of recent posts could be wrapped in an
<aside>
.
- Example: A sidebar containing related articles or a list of recent posts could be wrapped in an
<footer>
: Represents the footer of a section or page. It often contains information about the author, links to related documents, or copyright information.- Example: The
<footer>
element at the bottom of a webpage might include a copyright notice and contact information.
- Example: The
<main>
: Specifies the main content of the document. This content should be unique to the document and not repeated across multiple pages (like a sidebar or footer).- Example: The
<main>
tag would wrap the primary content of a webpage, such as the main article or blog post.
- Example: The
<figure>
and<figcaption>
: The<figure>
tag is used to encapsulate media content, such as images, illustrations, diagrams, or code snippets, while<figcaption>
is used to provide a caption for that content.- Example: An image and its caption can be wrapped in
<figure>
and<figcaption>
respectively to semantically group them together.
- Example: An image and its caption can be wrapped in
Best Practices for Using Semantic HTML
Use Elements Appropriately: Always choose the element that best describes the content. For example, use
<article>
for content that stands alone and<section>
for groups of related content.Keep it Simple: Don’t overuse semantic elements. Use them only where they make sense to describe the structure and content of your document.
Consider Accessibility: Think about how screen readers will interpret your document structure. Proper use of semantic elements will improve the experience for users with disabilities.
Avoid Nesting Non-Semantic Elements: When possible, avoid unnecessary nesting of non-semantic elements like
<div>
and<span>
. Instead, try to use semantic elements that provide context.
Semantic HTML is a fundamental part of modern web development that enhances the accessibility, SEO, and maintainability of a website. By thoughtfully applying semantic elements, you create more meaningful, understandable, and usable web pages for both users and search engines. It’s an essential skill for any web developer, contributing to better user experiences and more effective web content.
Conclusion
Understanding and effectively utilizing HTML's capabilities, including tables, iframes, forms, and semantic elements, is crucial for creating well-structured and user-friendly websites.
Tables are powerful tools for organizing data visually, allowing users to understand complex information at a glance. By properly structuring tables with headers and cells, you ensure that data is accessible and easy to interpret, both for users and search engines.
iFrames enable embedding external content, such as videos or maps, directly into your webpage, enriching the user experience. Using iFrames allows you to incorporate dynamic content seamlessly without disrupting the layout of your page.
Forms are essential for gathering user input, from contact details to feedback. Designing forms that are intuitive and accessible enhances user interaction and can be a vital aspect of any website, from e-commerce to social platforms.
Semantic HTML plays a vital role in improving the accessibility, SEO, and readability of your website. By using tags that describe the content's purpose, you help both users and search engines understand and navigate your site more effectively.
Through these concepts and practical implementations, I can build websites that are not only functional but also accessible, user-friendly, and optimized for performance. As I continue My web development journey, mastering these HTML techniques will set a strong foundation for creating more advanced and dynamic web applications.
Subscribe to my newsletter
Read articles from Rohit Gawande directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Rohit Gawande
Rohit Gawande
🚀 Tech Enthusiast | Full Stack Developer | System Design Explorer 💻 Passionate About Building Scalable Solutions and Sharing Knowledge Hi, I’m Rohit Gawande! 👋I am a Full Stack Java Developer with a deep interest in System Design, Data Structures & Algorithms, and building modern web applications. My goal is to empower developers with practical knowledge, best practices, and insights from real-world experiences. What I’m Currently Doing 🔹 Writing an in-depth System Design Series to help developers master complex design concepts.🔹 Sharing insights and projects from my journey in Full Stack Java Development, DSA in Java (Alpha Plus Course), and Full Stack Web Development.🔹 Exploring advanced Java concepts and modern web technologies. What You Can Expect Here ✨ Detailed technical blogs with examples, diagrams, and real-world use cases.✨ Practical guides on Java, System Design, and Full Stack Development.✨ Community-driven discussions to learn and grow together. Let’s Connect! 🌐 GitHub – Explore my projects and contributions.💼 LinkedIn – Connect for opportunities and collaborations.🏆 LeetCode – Check out my problem-solving journey. 💡 "Learning is a journey, not a destination. Let’s grow together!" Feel free to customize or add more based on your preferences! 😊