Understanding Promises in Node.js
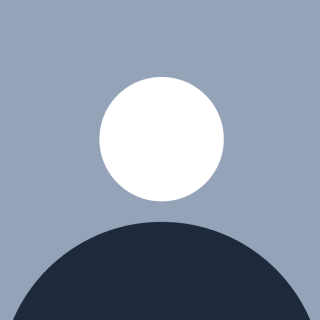
Table of contents
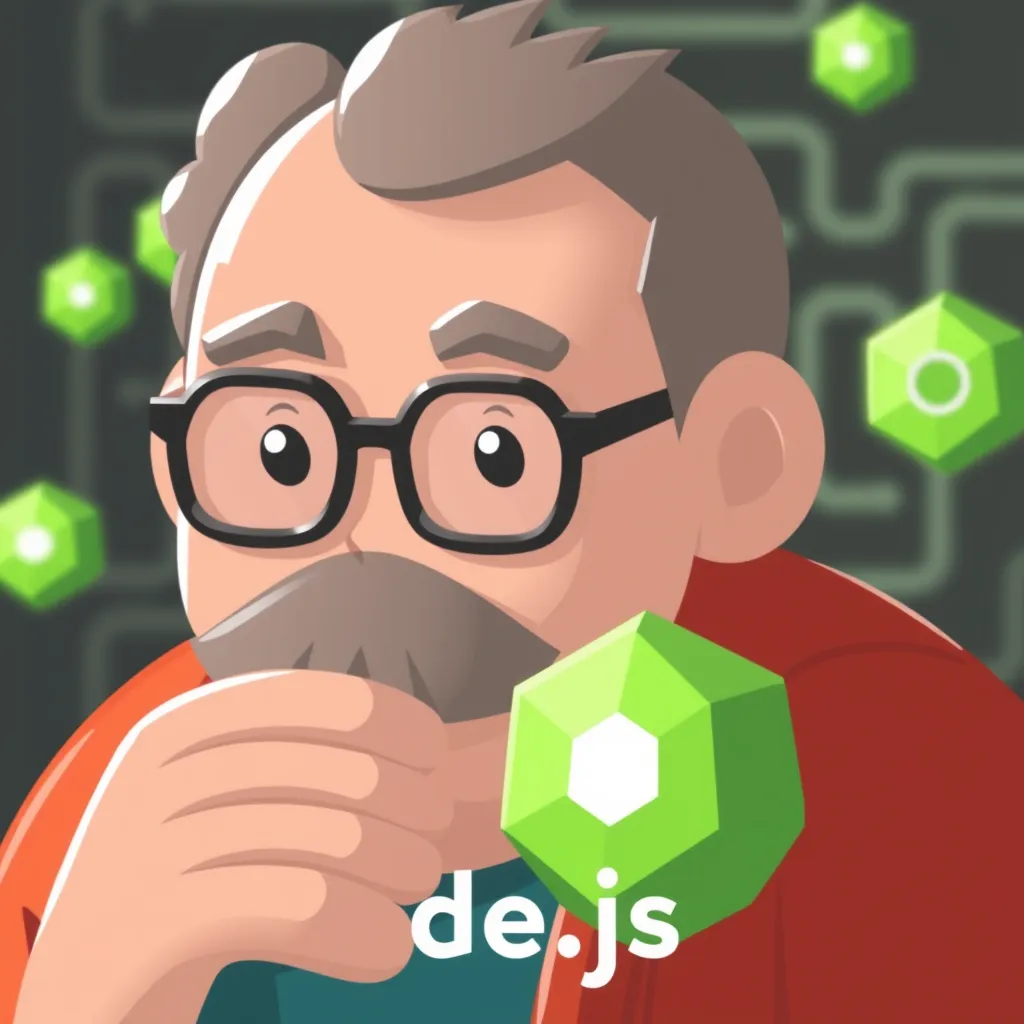
Promises are used to handle asynchronous operations efficiently. In this guide, we will learn about promises and explore their basics, advanced concepts, and best practices. We'll also discuss how to cancel promises and provide a flowchart to illustrate the promise lifecycle.
Introduction of Promise
It is an object that represents the eventual completion (or failure) of an asynchronous operation. Think of it as a guarantee that something will happen—either the operation will succeed, or it will fail. A promise can be in one of three states:
Pending: The initial state, where the operation is still ongoing.
Fulfilled: The operation has been completed successfully, and the promise now has a result.
Rejected: The operation has failed, and the promise now has an error.
Promises have some important methods.
.then()*: It handles the fulfillment of the Promise*
.catch()*: It handles the rejection of the Promise.*
Now We will understand with the help of a basic example. In which we will fetch the user info.
function fetchUserData() {
return new Promise((resolve, reject) => {
console.log("Fetching user data...");
setTimeout(() => {
const success = true; // Simulate a successful response
if (success) {
resolve({ name: "John Doe", age: 30 });
} else {
reject("Failed to fetch user data.");
}
}, 1000); // Simulate a 1-second delay
});
}
fetchUserData()
.then(user => {
console.log("User data fetched:", user);
})
.catch(error => {
console.error(error);
});
Explanation:
fetchUserData()
: This function returns a promise. It simulates fetching data with a 1-second delay.resolve()
: If the data fetch is successful, it resolves the promise with user data.reject()
: If the fetch fails, it rejects the promise with an error message..then()
: Handles the successful resolution of the promise, logging the user data..catch()
: Catches any errors that occur, logging the error message.
Chaining Promise
One of the powerful features of Promises is chaining. You can chain multiple
.then()
methods to handle a sequence of asynchronous operations. Each.then()
returns a new Promise, allowing further chaining.
fetchUserData()
.then(user => {
console.log("User data fetched:", user);
return user.name;
})
.then(userName => {
console.log("User name is:", userName);
})
.catch(error => {
console.error(error);
});
Error Handling in Promises
Handling errors in Promises is straightforward using .catch()
. If an error occurs at any point in the chain, .catch()
will catch it.
function fetchUserDataWithFailure() {
return new Promise((resolve, reject) => {
console.log("Fetching user data...");
setTimeout(() => {
const success = false; // Simulate a failed response
if (success) {
resolve({ name: "John Doe", age: 30 });
} else {
reject("Failed to fetch user data.");
}
}, 1000);
});
}
fetchUserDataWithFailure()
.then(user => {
console.log("User data fetched:", user);
})
.catch(error => {
console.error("Error occurred:", error); // 'Error occurred: Failed to fetch user data.'
});
There are several types of Promise Methods.
Promise.all():
It takes an array of Promises and returns a single Promise. Resolves when each promise in the array is fulfilled; if any promise is rejected, it rejects. Useful when you want to run multiple asynchronous tasks in parallel and wait for all of them to complete.
const promise1 = fetchUserData();
const promise2 = Promise.resolve('Static Data');
Promise.all([promise1, promise2])
.then(results => {
console.log(results); // [{ name: "John Doe", age: 30 }, 'Static Data']
})
.catch(error => {
console.error(error);
});
Promise.race()
:
Similar to Promise.all()
but returns the result of the first promise that resolves or rejects. Useful when you want the fastest result, regardless of the others.
const slowPromise = new Promise(resolve => setTimeout(resolve, 2000, 'Slow Promise'));
const fastPromise = new Promise(resolve => setTimeout(resolve, 1000, 'Fast Promise'));
Promise.race([slowPromise, fastPromise])
.then(result => {
console.log(result); // 'Fast Promise'
});
Promise.allSettled()
:
Waits for all Promises to settle (either resolve or reject) and returns an array of objects representing the outcome of each Promise.
const promise1 = fetchUserDataWithFailure();
const promise2 = Promise.resolve('Success Data');
Promise.allSettled([promise1, promise2])
.then(results => {
console.log(results);
// [{ status: 'rejected', reason: 'Failed to fetch user data.' },
// { status: 'fulfilled', value: 'Success Data' }]
});
Promise.any()
:
Returns the first promise that fulfills, ignoring rejected promises. If all promises are rejected, it returns an AggregateError with all the rejection reasons.
const promise1 = Promise.reject('Error 1');
const promise2 = fetchUserData();
Promise.any([promise1, promise2])
.then(result => {
console.log(result); // { name: "John Doe", age: 30 }
})
.catch(errors => {
console.error(errors);
});
Advanced Concepts
Nesting vs. Chaining Promises: Nesting can occur when you return another Promise within a
.then()
. Chaining is generally preferred for clarity, but nesting can be useful when you need to conditionally execute asynchronous operations.fetchUserData() .then(user => { if (user.age > 25) { return Promise.resolve("User is older than 25"); } else { return "User is 25 or younger"; } }) .then(message => { console.log(message); }) .catch(error => { console.error(error); });
Handling Multiple Promises with Loops: Sometimes you need to handle an array of Promises with a loop or
map
. UsingPromise.all()
withmap
is a common pattern for running operations in parallel.const userIds = [1, 2, 3]; const fetchUser = id => fetch(`https://api.example.com/user/${id}`).then(response => response.json()); Promise.all(userIds.map(id => fetchUser(id))) .then(users => { console.log(users); });
Common Pitfalls
Not handling rejections: Always use .catch() to handle rejections, even if you expect them.
Forgetting to return within.then(): If you don't return a value or Promise, the next .then() will receive undefined.
Overusing nested Promises: While nesting can be useful, overuse can lead to less readable code. Prefer chaining when possible.
Summary:
Promises are a powerful tool for managing asynchronous operations in JavaScript. They provide a more structured way to handle operations compared to callbacks, making your code cleaner and easier to maintain. By mastering Promises and their related methods, you can handle complex asynchronous flows with confidence.
Subscribe to my newsletter
Read articles from Aman jain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Aman jain
Aman jain
I'm a developer who shares advanced insights and expertise through technology-related articles. Passionate about creating innovative solutions and staying up-to-date with the latest tech trends. Let's connect and explore the world of technology together!