☑️Day 1: Python For DevOps

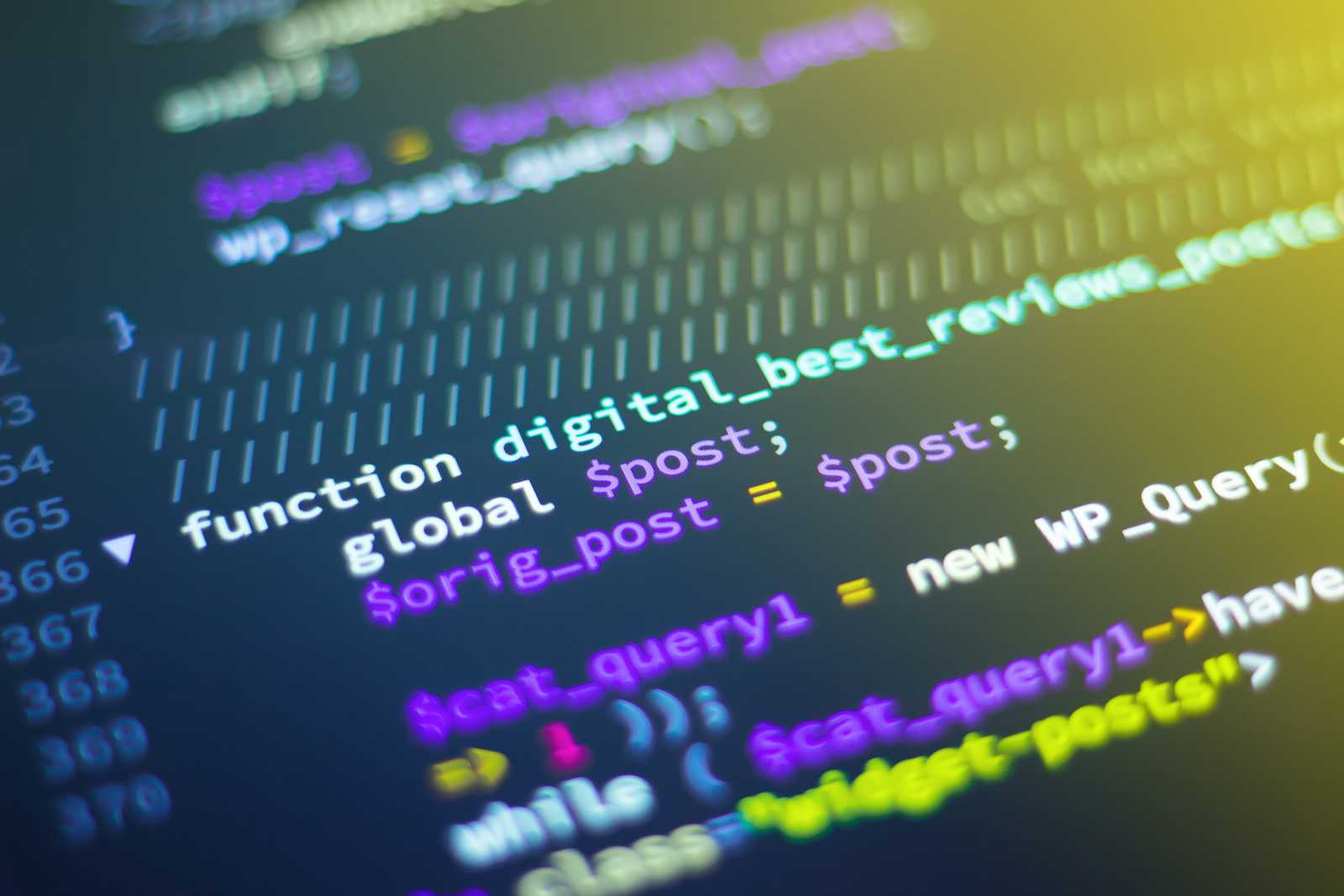
🔹Table Of content :
✅Variables
✅Input Handling
✅Type Checking
✅Type Casting
✅Loops
✅Getting Started with Python Basics
As a DevOps professional, understanding Python is crucial. Python is known for its simplicity and readability, making it an excellent choice for scripting and automation.
Here’s a quick overview of what you should know as you start learning Python:
- Basic Syntax: Python syntax is clean and easy to understand. It uses indentation to define code blocks, which makes it readable.
Loops: Mastering loops is essential for writing scripts that perform repetitive tasks. Python supports for
and while
loops.
✅1. Variables
Variables are used to store data in Python. You can assign a value to a variable using the =
operator.
# Assigning values to variables
name = "John" # String
age = 30 # Integer
height = 5.9 # Float
print(name) # Output: John
print(age) # Output: 30
print(height) # Output: 5.9
✅2. Input Handling
You can take input from the user using the input()
function. By default, input()
returns a string.
# Taking input from the user
user_name = input("Enter your name: ")
user_age = input("Enter your age: ")
print(f"Hello, {user_name}! You are {user_age} years old.")
✅3. Type Checking
To check the type of a variable, you can use the type()
function.
# Checking the type of a variable
variable1 = "Hello"
variable2 = 100
variable3 = 3.14
print(type(variable1)) # Output: <class 'str'>
print(type(variable2)) # Output: <class 'int'>
print(type(variable3)) # Output: <class 'float'>
✅4. Type Casting
Type casting is used to convert one data type into another. Python provides several built-in functions for type casting, such as int()
, float()
, and str()
.
# Type casting example
num_str = "50"
num_int = int(num_str) # Converts string to integer
num_float = float(num_str) # Converts string to float
print(num_int) # Output: 50
print(num_float) # Output: 50.0
# Casting an integer to a string
age = 25
age_str = str(age)
print("Your age is " + age_str) # Output: Your age is 25
✅5. Loops
Loops are used to execute a block of code repeatedly. Python supports two types of loops: for
and while
.
a. For Loop
The for
loop is used to iterate over a sequence (like a list, tuple, dictionary, set, or string).
# For loop example
for i in range(5):
print(f"Iteration {i}")
b. While Loop
The while
loop continues to execute as long as a specified condition is True
.
# While loop example
count = 0
while count < 5:
print(f"Count is: {count}")
count += 1
Stay tuned for more updates on my DevOps journey! 📅
Feel free to reach out to me if you have any questions or need any more help. Let’s connect, learn, and succeed together!
Happy Learning!😊
#90DaysOfDevOps
/
Subscribe to my newsletter
Read articles from Kedar Pattanshetti directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
