Promises in JavaScript

Table of contents
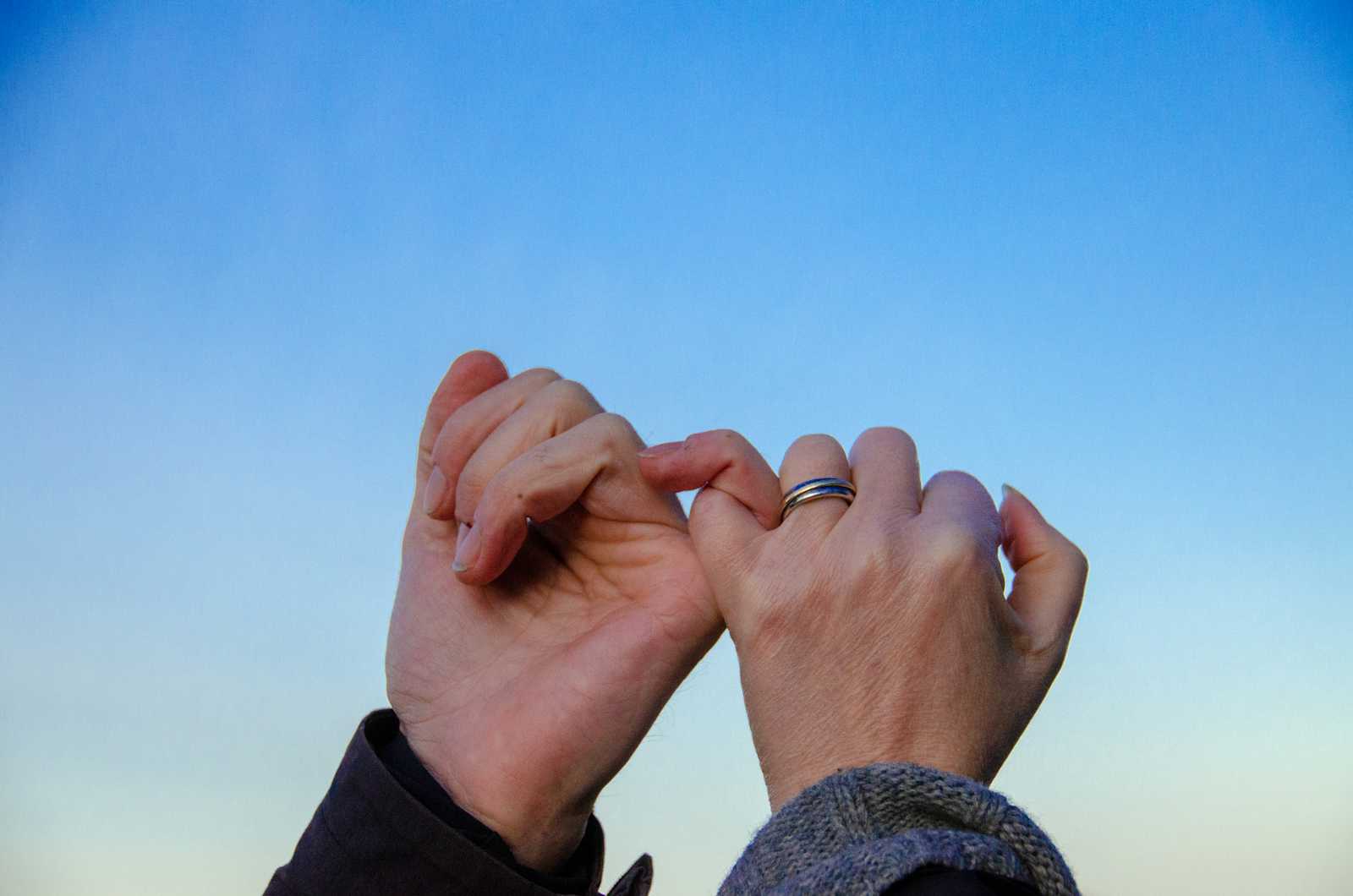
The Promise
object represents the eventual completion (or failure) of an asynchronous operation, and its resulting value.
The methods promise.then()
, promise.catch()
, and promise.finally()
are used to associate further action with a promise that becomes settled.
For example:
const myPromise = new Promise(function (resolve, reject) {
let sampleData = [2, 4, 6, 8];
let randomNumber = Math.floor(Math.random() * (sampleData.length + 1));
if (sampleData[randomNumber]) {
resolve(sampleData[randomNumber]);
} else {
reject('An error occured!');
}
});
myPromise
.then(function (e) {
console.log(e);
})
.catch(function (error) {
throw new Error(error);
})
.finally(function () {
console.log('Promise completed');
});
Methods
These methods are available on Promise.prototype
then
The
.then()
method takes up to two arguments; the first argument is a callback function for the resolved case of the promise, and the second argument is a callback function for the rejected case. Each.then()
returns a newly generated promise object, which can optionally be used for chaining.1
const promise1 = new Promise(function (resolve, reject) {
resolve('Success!');
});
promise1.then(function (value) {
console.log(value);
// expected output: "Success!"
});
catch
A
.catch()
is really just a.then()
without a slot for a callback function for the case when the promise is resolved. It is used to handle rejected promises.2
const promise1 = new Promise((resolve, reject) => {
throw 'An error occured';
});
promise1.catch(function (error) {
console.error(error);
});
// expected output: An error occured
finally
When the promise is settled, i.e either fulfilled or rejected, the specified callback function is executed. This provides a way for code to be run whether the promise was fulfilled successfully or rejected once the Promise has been dealt with.3
function findDataById(id) {
return new Promise(function (resolve, reject) {
let sampleData = [1, 2, 3, 4, 5];
if (sampleData[id]) {
resolve(sampleData[id]);
} else {
reject(new Error('Invalid id'));
}
});
}
findDataById(4)
.then(function (response) {
console.log(response);
})
.catch(function (err) {
console.error(err);
})
.finally(function () {
console.log('Promise completed');
});
then
, MDN. https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Promise/then ↩catch
, MDN. https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Promise/catch ↩finally
, MDN. https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Promise/finally ↩
Credits: Exercism
Subscribe to my newsletter
Read articles from Zeeshan Safdar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Zeeshan Safdar
Zeeshan Safdar
An accomplished front-end developer with a strong background in creating visually stunning and user-friendly websites and web applications. My deep understanding of web development principles and user-centered design allows me to deliver projects that exceed client expectations. I have a proven track record of success in delivering projects on time and to the highest standards, and I am able to work well in a team environment and am always looking for new challenges to take on.