Excel VBA Guide: From Basic to Advanced
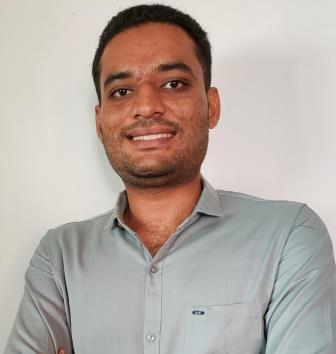
I'll break down each of the topics, explaining their functionalities and providing real-time scenarios to help you understand how these concepts are applied in practice.
1. Basics of VBA
# Introduction to VBA
- What is VBA?
- Functionality: VBA (Visual Basic for Applications) is a programming language integrated into Microsoft Office applications. It allows you to automate tasks, create custom functions, and manage Excel operations through code.
- Real-time Scenario: Automating the generation of monthly sales reports. Instead of manually copying data and applying formulas, you can write a VBA script to handle this in a few clicks.
# VBA Editor (VBE) Overview
- Opening the VBA Editor
- Functionality: The VBA Editor is where you write and manage your VBA code. You can access it by pressing ALT + F11
in Excel.
Real-time Scenario: When you want to create a custom macro, you open the VBA Editor to write and test your code.
- Components of the VBA Editor
- Functionality: The VBA Editor includes various components such as the Project Explorer, Properties Window, and Code Window.
- Real-time Scenario: Navigating through different modules and forms in the Project Explorer to organize your VBA projects effectively.
- Writing and Running Your First Macro
- Functionality: A macro is a sequence of instructions that automate repetitive tasks. You can write it directly in the VBA Editor or record it using Excel’s macro recorder.
- Real-time Scenario: Writing a simple macro to format a report with a specific font, color, and border.
# Macro Recording
- Recording a Macro
- Functionality: Macro recording captures your actions in Excel and converts them into VBA code.
- Real-time Scenario: Recording a macro to automate the process of applying filters and sorting data in a large dataset.
- Understanding the Generated Code
- Functionality: The code generated by the macro recorder can be viewed in the VBA Editor. It’s often a good starting point for learning VBA.
- Real-time Scenario: Analyzing the code generated by a recorded macro to understand how Excel objects like Range
and Selection
work.
- Editing a Recorded Macro
- Functionality: Once recorded, you can modify the VBA code to enhance the macro's functionality.
- Real-time Scenario: Editing a recorded macro to make it dynamic, such as allowing it to handle varying data ranges.
# VBA Syntax and Structure
- Variables, Data Types, and Constants
- Functionality: Variables store data that can change during the execution of the code, while constants hold values that do not change.
- Real-time Scenario: Using variables to store user inputs, such as dates or amounts, which are then used to filter data or perform calculations.
1. Variables
Definition: A variable in VBA is a storage location identified by a name (or an identifier) that holds data which can be modified during the execution of the program. Variables are essential in VBA as they allow you to store data, perform calculations, and control the flow of your code.
Declaration: Before using a variable, you should declare it using the
Dim
statement. Declaring variables is good practice as it helps avoid errors and improves the readability of your code.Syntax:
Dim variableName As DataType
Example:
Dim totalSales As Double Dim customerName As String
Assigning Values: Once a variable is declared, you can assign a value to it using the assignment operator (
=
).Example:
totalSales = 1500.75 customerName = "John Doe"
Scope of Variables: The scope determines where the variable can be accessed within your code. Variables can have different scopes based on where they are declared:
Procedure-level: Declared within a subroutine or function and accessible only within that procedure.
Module-level: Declared at the top of a module and accessible to all procedures within that module.
Global-level: Declared using
Public
at the top of a module and accessible across all modules in the project.
Example:
' Procedure-level variable
Sub CalculateTotal()
Dim totalSales As Double
totalSales = 1500.75
End Sub
' Module-level variable
Dim totalSales As Double
Sub CalculateTotal()
totalSales = 1500.75
End Sub
Sub DisplayTotal()
MsgBox totalSales
End Sub
' Global-level variable
Public totalSales As Double
2. Data Types
Definition: Data types in VBA define the kind of data a variable can hold, such as integers, strings, dates, etc. Choosing the correct data type is important for optimizing memory usage and improving performance.
Common Data Types:
Integer: Stores whole numbers (e.g., 1, 100).
Long: Stores larger whole numbers.
Double: Stores floating-point numbers (e.g., 10.5, 3.14159).
String: Stores text (e.g., "Hello World").
Boolean: Stores
True
orFalse
values.Date: Stores dates and times.
Variant: Can store any type of data (default data type if none is specified).
Examples:
Dim age As Integer
Dim salary As Double
Dim isActive As Boolean
Dim hireDate As Date
Dim employeeName As String
Usage Example:
Sub EmployeeDetails() Dim employeeName As String Dim hireDate As Date Dim salary As Double Dim isActive As Boolean employeeName = "Jane Smith" hireDate = #1/1/2020# salary = 75000.5 isActive = True MsgBox employeeName & " was hired on " & hireDate & " with a salary of " & salary End Sub
3. Constants
Definition: A constant is similar to a variable, but its value cannot be changed once it is assigned. Constants are useful for values that remain the same throughout the execution of the code, such as tax rates, conversion factors, or configuration settings.
Declaration: Constants are declared using the
Const
keyword.Syntax:
Const constantName As DataType = value
Example:
Const PI As Double = 3.14159 Const TAX_RATE As Double = 0.07
Usage Example:
Sub CalculateCircleArea() Const PI As Double = 3.14159 Dim radius As Double Dim area As Double radius = 5 area = PI * radius * radius MsgBox "The area of the circle is " & area End Sub
- Real-time Scenario: Consider you are building a financial model in Excel. You need to apply a constant tax rate across various calculations. Instead of hardcoding the tax rate in multiple places, you define it once as a constant:
Const TAX_RATE As Double = 0.07
Dim revenue As Double
Dim taxAmount As Double
revenue = 100000
taxAmount = revenue * TAX_RATE
MsgBox "The tax amount is " & taxAmount
Variables are dynamic storage locations for data that can change throughout the execution of your code.
Data Types specify the kind of data a variable can hold, such as numbers, text, or dates.
Constants are similar to variables, but their values are fixed and cannot be changed after they are defined.
- Operators (Arithmetic, Comparison, Logical)
- Functionality: Operators allow you to perform calculations, compare values, and make logical decisions in your code.
- Real-time Scenario: Using arithmetic operators to calculate the total sales amount and logical operators to apply conditional formatting based on performance metrics.
1. Arithmetic Operators
Arithmetic operators in VBA are used to perform mathematical calculations. These operators work with numeric data types (e.g., Integer
, Double
).
Basic Arithmetic Operators:
Addition (
+
): Adds two numbers.- Example:
result = 5 + 3
(result = 8)
- Example:
Subtraction (
-
): Subtracts one number from another.- Example:
result = 5 - 3
(result = 2)
- Example:
Multiplication (
*
): Multiplies two numbers.- Example:
result = 5 * 3
(result = 15)
- Example:
Division (
/
): Divides one number by another, resulting in a floating-point number.- Example:
result = 5 / 3
(result = 1.6667)
- Example:
Integer Division (
\
): Divides one number by another, returning an integer result (truncating any remainder).- Example:
result = 5 \ 3
(result = 1)
- Example:
Modulus (
Mod
): Returns the remainder after dividing one number by another.- Example:
result = 5 Mod 3
(result = 2)
- Example:
Exponentiation (
^
): Raises one number to the power of another.- Example:
result = 5 ^ 3
(result = 125)
- Example:
Usage Example:
Sub ArithmeticOperations() Dim num1 As Double Dim num2 As Double Dim result As Double num1 = 10 num2 = 3 result = num1 + num2 ' Addition MsgBox "Addition: " & result result = num1 - num2 ' Subtraction MsgBox "Subtraction: " & result result = num1 * num2 ' Multiplication MsgBox "Multiplication: " & result result = num1 / num2 ' Division MsgBox "Division: " & result result = num1 \ num2 ' Integer Division MsgBox "Integer Division: " & result result = num1 Mod num2 ' Modulus MsgBox "Modulus: " & result result = num1 ^ num2 ' Exponentiation MsgBox "Exponentiation: " & result End Sub
Real-time Scenario: Calculating the total price of an order including tax.
Sub CalculateTotalPrice() Dim price As Double Dim taxRate As Double Dim totalPrice As Double price = 100 taxRate = 0.08 totalPrice = price + (price * taxRate) MsgBox "The total price including tax is: " & totalPrice End Sub
2. Comparison Operators
Comparison operators are used to compare two values. They return a Boolean
value (True
or False
) depending on whether the comparison is true.
Common Comparison Operators:
Equal to (
=
): Checks if two values are equal.- Example:
result = (5 = 3)
(result = False)
- Example:
Not equal to (
<>
): Checks if two values are not equal.- Example:
result = (5 <> 3)
(result = True)
- Example:
Greater than (
>
): Checks if one value is greater than another.- Example:
result = (5 > 3)
(result = True)
- Example:
Less than (
<
): Checks if one value is less than another.- Example:
result = (5 < 3)
(result = False)
- Example:
Greater than or equal to (
>=
): Checks if one value is greater than or equal to another.- Example:
result = (5 >= 3)
(result = True)
- Example:
Less than or equal to (
<=
): Checks if one value is less than or equal to another.- Example:
result = (5 <= 3)
(result = False)
- Example:
Usage Example:
Sub ComparisonOperations() Dim num1 As Double Dim num2 As Double Dim result As Boolean num1 = 10 num2 = 5 result = (num1 = num2) ' Equal to MsgBox "Equal to: " & result result = (num1 <> num2) ' Not equal to MsgBox "Not equal to: " & result result = (num1 > num2) ' Greater than MsgBox "Greater than: " & result result = (num1 < num2) ' Less than MsgBox "Less than: " & result result = (num1 >= num2) ' Greater than or equal to MsgBox "Greater than or equal to: " & result result = (num1 <= num2) ' Less than or equal to MsgBox "Less than or equal to: " & result End Sub
Real-time Scenario: Checking if a user’s input falls within an acceptable range.
Sub ValidateInput() Dim userInput As Integer userInput = InputBox("Enter a number between 1 and 10:") If userInput >= 1 And userInput <= 10 Then MsgBox "Valid input." Else MsgBox "Invalid input. Please enter a number between 1 and 10." End If End Sub
3. Logical Operators
Logical operators are used to combine multiple conditions. They also return Boolean
values and are often used in control structures like If...Then
statements.
Common Logical Operators:
And: Returns
True
if both conditions are true.- Example:
result = (5 > 3 And 8 > 6)
(result = True)
- Example:
Or: Returns
True
if at least one of the conditions is true.- Example:
result = (5 > 3 Or 8 < 6)
(result = True)
- Example:
Not: Reverses the value of the condition.
- Example:
result = Not(5 > 3)
(result = False)
- Example:
Usage Example:
Sub LogicalOperations() Dim age As Integer Dim hasLicense As Boolean Dim canDrive As Boolean age = 18 hasLicense = True canDrive = (age >= 18 And hasLicense) ' Logical AND MsgBox "Can drive: " & canDrive canDrive = (age >= 18 Or hasLicense) ' Logical OR MsgBox "Can drive with either condition true: " & canDrive canDrive = Not(hasLicense) ' Logical NOT MsgBox "Does not have license: " & canDrive End Sub
Real-time Scenario: Determining whether an employee is eligible for a bonus based on performance and attendance.
Sub CheckBonusEligibility() Dim performanceScore As Integer Dim attendanceRate As Double Dim isEligible As Boolean performanceScore = 85 attendanceRate = 0.95 isEligible = (performanceScore > 80 And attendanceRate > 0.9) If isEligible Then MsgBox "The employee is eligible for a bonus." Else MsgBox "The employee is not eligible for a bonus." End If End Sub
Arithmetic Operators: Used for performing mathematical calculations (e.g.,
+
,-
,*
,/
).Comparison Operators: Used to compare values and return
True
orFalse
(e.g.,=
,<>
,>
,<
).Logical Operators: Used to combine multiple conditions and return
True
orFalse
(e.g.,And
,Or
,Not
).
- Commenting Code
- Functionality: Comments are non-executable lines in your code that explain what the code does. They are helpful for documentation and debugging.
- Real-time Scenario: Adding comments to complex parts of your code to help future developers (or yourself) understand its logic.
1. Purpose of Commenting Code
Commenting code in VBA is crucial for writing clear, understandable, and maintainable code. Comments are annotations within the code that explain what the code does, why certain decisions were made, or to provide context for future reference. These comments are ignored by the VBA compiler and do not affect the execution of the program.
2. How to Write Comments
Single-Line Comments:
In VBA, a single-line comment is created by placing an apostrophe (
'
) before the comment text. Everything after the apostrophe on that line is treated as a comment.Syntax:
' This is a single-line comment Dim totalSales As Double ' This variable holds the total sales amount
Commenting Entire Lines:
You can comment out entire lines of code by placing an apostrophe at the beginning of the line. This is useful for temporarily disabling code during debugging or testing.
Example:
'totalSales = price * quantity ' Commented out for testing totalSales = price + taxAmount ' Calculate total sales including tax
Using the
Rem
Keyword:Another way to add comments in VBA is by using the
Rem
keyword. This method is less commonly used but serves the same purpose as an apostrophe.Syntax:
Rem This is a comment using the Rem keyword totalSales = price * quantity Rem Calculating total sales
3. Best Practices for Commenting
Explain the "Why":
Focus on explaining why the code is written a certain way rather than just what it does, as the code itself often makes the "what" clear.
Example:
' Adjusting for tax changes implemented in 2024 taxRate = 0.07
Keep Comments Relevant and Up-to-Date:
Ensure that comments accurately reflect the code. Outdated comments can be misleading and cause confusion.
Example:
' Calculates the total price including the updated tax rate totalPrice = price + (price * taxRate)
Use Comments to Break Down Complex Code:
For complex procedures or functions, use comments to break down each step. This helps others (and your future self) understand the logic.
Example:
' Step 1: Initialize variables Dim totalSales As Double Dim discount As Double ' Step 2: Calculate total sales totalSales = price * quantity ' Step 3: Apply discount if applicable If totalSales > 1000 Then discount = totalSales * 0.1 ' 10% discount for orders over $1000 totalSales = totalSales - discount End If ' Step 4: Output the final total sales MsgBox "The final total sales amount is: " & totalSales
Use Comments to Clarify Non-Obvious Code:
If you write code that is not immediately understandable, add a comment to explain its purpose or the logic behind it.
Example:
' Using a nested loop to check all possible pairs For i = 1 To 10 For j = i + 1 To 10 ' Check if the pair (i, j) satisfies the condition If (array(i) + array(j)) = targetValue Then MsgBox "Pair found: " & i & ", " & j End If Next j Next i
4. Real-Time Scenarios for Commenting
Documenting Business Logic: In a financial model, you might include comments to explain specific business rules or calculations.
' Applying the corporate tax rate as per the 2024 financial guidelines taxAmount = revenue * corporateTaxRate
Debugging Assistance: When troubleshooting, you might comment out specific lines of code or add comments explaining what you've found during debugging.
' Debugging: Checking the value of totalSales before applying the discount Debug.Print "Total Sales before discount: " & totalSales
Team Collaboration: When working in a team, comments help others understand your code, especially when they need to make changes or troubleshoot issues.
' Added by John on 2024-09-01 to handle new discount logic If customerType = "VIP" Then discountRate = 0.15 ' VIP customers get a 15% discount End If
5. Commenting Blocks of Code
While VBA doesn’t have a native way to comment out multiple lines at once (like /* */
in some other languages), you can quickly add or remove comments for multiple lines by selecting them and using a keyboard shortcut in the VBA editor:
Comment Block: Select the lines and press
Ctrl + Shift + C
.Uncomment Block: Select the lines and press
Ctrl + Shift + U
.
Summary
Comments are essential for making your code understandable, maintainable, and easier to debug.
Single-line comments are added using an apostrophe (
'
) or theRem
keyword.Best practices include explaining why the code is written a certain way, keeping comments relevant and up-to-date, breaking down complex code with comments, and clarifying non-obvious logic.
Real-time scenarios for comments include documenting business logic, assisting with debugging, and enhancing team collaboration.
# Control Structures
- If...Then...Else Statements
Functionality: These structures allow you to execute code based on specific conditions.
Real-time Scenario: Writing a script that checks if sales targets are met and applies different actions based on the outcome.
1. Overview
The If...Then...Else
statement is a fundamental control structure in VBA that allows you to execute code conditionally based on whether a specific condition (or set of conditions) is true or false. This control structure helps direct the flow of your program, enabling different outcomes depending on various conditions.
2. Basic Structure
The basic syntax of an If...Then...Else
statement in VBA is as follows:
If condition Then
' Code to execute if the condition is True
Else
' Code to execute if the condition is False
End If
Condition: A Boolean expression (a condition that evaluates to either
True
orFalse
).Then: Indicates the start of the code block that runs if the condition is
True
.Else: An optional clause that specifies the code to run if the condition is
False
.End If: Marks the end of the
If...Then...Else
statement.
3. Examples
Simple Example:
Sub CheckDiscountEligibility()
Dim totalPurchase As Double
totalPurchase = 500
If totalPurchase > 300 Then
MsgBox "You are eligible for a discount!"
Else
MsgBox "You are not eligible for a discount."
End If
End Sub
In this example, if totalPurchase
is greater than 300, the message box will display "You are eligible for a discount!" Otherwise, it will display "You are not eligible for a discount."
4. If...Then...ElseIf...Else Structure
For situations where you need to evaluate multiple conditions, you can use the ElseIf
clause:
If condition1 Then
' Code to execute if condition1 is True
ElseIf condition2 Then
' Code to execute if condition2 is True
ElseIf condition3 Then
' Code to execute if condition3 is True
Else
' Code to execute if none of the above conditions are True
End If
Example:
Sub GradeStudent()
Dim score As Integer
score = 85
If score >= 90 Then
MsgBox "Grade: A"
ElseIf score >= 80 Then
MsgBox "Grade: B"
ElseIf score >= 70 Then
MsgBox "Grade: C"
ElseIf score >= 60 Then
MsgBox "Grade: D"
Else
MsgBox "Grade: F"
End If
End Sub
Here, the student’s score is evaluated, and depending on the score, a grade is assigned. The conditions are checked in order, and the first condition that evaluates to True
triggers the corresponding code block.
5. Nested If Statements
You can also nest If...Then...Else
statements within each other for more complex decision-making:
If condition1 Then
If condition2 Then
' Code to execute if both condition1 and condition2 are True
Else
' Code to execute if condition1 is True but condition2 is False
End If
Else
' Code to execute if condition1 is False
End If
Example:
Sub CheckEligibility()
Dim age As Integer
Dim hasLicense As Boolean
age = 20
hasLicense = True
If age >= 18 Then
If hasLicense Then
MsgBox "You are eligible to drive."
Else
MsgBox "You need a driver's license to drive."
End If
Else
MsgBox "You are not old enough to drive."
End If
End Sub
In this example, the code first checks if the person is old enough to drive. If they are, it then checks if they have a driver’s license before determining eligibility.
6. Single-Line If...Then Statement
For very simple conditions, you can use a single-line If...Then
statement without Else
:
If condition Then statement
Example:
Sub SimpleCheck()
Dim total As Double
total = 150
If total > 100 Then MsgBox "Total is greater than 100"
End Sub
This format is useful for concise checks but should be used sparingly as it can reduce readability.
7. Real-Time Scenario
Scenario: Approving a Loan Application
Let’s say you are writing a VBA macro to approve or reject a loan application based on the applicant’s credit score and income level.
Sub ApproveLoan()
Dim creditScore As Integer
Dim annualIncome As Double
creditScore = 700
annualIncome = 50000
If creditScore >= 650 Then
If annualIncome >= 40000 Then
MsgBox "Loan Approved"
Else
MsgBox "Loan Denied: Insufficient Income"
End If
Else
MsgBox "Loan Denied: Low Credit Score"
End If
End Sub
In this scenario:
If the applicant’s credit score is 650 or higher, the program checks their income.
If the income is also sufficient (e.g., $40,000 or more), the loan is approved.
Otherwise, the loan is denied due to insufficient income or a low credit score.
8. Best Practices
Keep Conditions Simple: Avoid overly complex conditions. If necessary, break them down into smaller parts for clarity.
Indent Code: Properly indent the code within
If...Then...Else
blocks to enhance readability.Consider Readability: When using
ElseIf
, ensure that the order of conditions makes logical sense and is easy to follow.Avoid Deep Nesting: While nesting is sometimes necessary, deep nesting can make the code difficult to follow. Consider using
Select Case
statements or refactoring the logic if the nesting becomes too deep.
Summary
The
If...Then...Else
statement in VBA allows you to execute code based on whether specific conditions are true or false.Basic
If...Then...Else
: Used for simple true/false conditions.ElseIf
: Allows multiple conditions to be checked in sequence.Nested
If
Statements: Used for more complex decision-making where conditions depend on the outcomes of other conditions.Single-Line
If...Then
: Useful for simple, quick checks but should be used sparingly for the sake of readability.
Mastering If...Then...Else
statements is key to controlling the flow of your VBA programs, enabling dynamic and responsive code based on varying conditions.
- Select Case Statement
Functionality: A more readable alternative to multiple If...Then...Else statements when you have several possible conditions to check.
Real-time Scenario: Automating the assignment of grades based on scores where each score range corresponds to a specific grade.
1. Overview
The Select Case
statement in VBA is a control structure used to evaluate a single expression and execute different blocks of code based on the value of that expression. It’s particularly useful when you have multiple possible outcomes for a variable or expression and want to avoid writing a series of If...Then...ElseIf
statements.
2. Basic Structure
The basic syntax of a Select Case
statement is as follows:
Select Case expression
Case value1
' Code to execute if expression equals value1
Case value2
' Code to execute if expression equals value2
Case value3
' Code to execute if expression equals value3
Case Else
' Code to execute if expression does not match any of the above values
End Select
expression: A variable or an expression that is evaluated once and compared against each
Case
.Case value: Specifies a possible value of the expression. The corresponding block of code is executed if the expression matches this value.
Case Else: An optional clause that handles any values not covered by the specified cases. It’s similar to the
Else
clause in anIf...Then...Else
statement.
3. Examples
Basic Example:
Sub DisplayDay()
Dim dayNumber As Integer
dayNumber = 3
Select Case dayNumber
Case 1
MsgBox "Monday"
Case 2
MsgBox "Tuesday"
Case 3
MsgBox "Wednesday"
Case 4
MsgBox "Thursday"
Case 5
MsgBox "Friday"
Case 6
MsgBox "Saturday"
Case 7
MsgBox "Sunday"
Case Else
MsgBox "Invalid day number"
End Select
End Sub
In this example, if dayNumber
is 3
, the program will display "Wednesday" in a message box. If dayNumber
is outside the range of 1 to 7, the Case Else
block will handle it and display "Invalid day number".
4. Handling Multiple Values
You can also handle multiple values within a single Case
by separating them with commas:
Select Case expression
Case value1, value2, value3
' Code to execute if expression equals any of value1, value2, or value3
Case Else
' Code to execute if expression does not match any of the above values
End Select
Example:
Sub DisplaySeason()
Dim monthNumber As Integer
monthNumber = 5
Select Case monthNumber
Case 12, 1, 2
MsgBox "Winter"
Case 3, 4, 5
MsgBox "Spring"
Case 6, 7, 8
MsgBox "Summer"
Case 9, 10, 11
MsgBox "Autumn"
Case Else
MsgBox "Invalid month number"
End Select
End Sub
Here, if monthNumber
is 5
, the program will display "Spring".
5. Using Ranges
VBA’s Select Case
statement allows you to specify ranges of values within a Case
:
Select Case expression
Case Is < value
' Code to execute if expression is less than value
Case value1 To value2
' Code to execute if expression is between value1 and value2 (inclusive)
Case Else
' Code to execute if expression does not match any of the above conditions
End Select
Example:
Sub EvaluateScore()
Dim score As Integer
score = 85
Select Case score
Case Is < 50
MsgBox "Fail"
Case 50 To 69
MsgBox "Pass"
Case 70 To 89
MsgBox "Merit"
Case 90 To 100
MsgBox "Distinction"
Case Else
MsgBox "Invalid score"
End Select
End Sub
In this example, if score
is 85
, the program will display "Merit".
6. Nested Select Case
Like If...Then...Else
statements, you can nest Select Case
statements inside one another for more complex decision-making:
Select Case expression1
Case value1
Select Case expression2
Case valueA
' Code for expression1 = value1 and expression2 = valueA
Case valueB
' Code for expression1 = value1 and expression2 = valueB
End Select
Case value2
' Code for expression1 = value2
End Select
Example:
Sub DetermineCategory()
Dim age As Integer
Dim income As Double
age = 30
income = 45000
Select Case age
Case Is < 18
MsgBox "Minor"
Case 18 To 65
Select Case income
Case Is < 20000
MsgBox "Low income adult"
Case 20000 To 50000
MsgBox "Middle income adult"
Case Is > 50000
MsgBox "High income adult"
End Select
Case Is > 65
MsgBox "Senior"
End Select
End Sub
In this scenario:
The program first checks the person’s age.
If they are an adult (between 18 and 65 years old), it further categorizes them based on income level.
7. Real-Time Scenario
Scenario: Assigning Employee Bonuses
Let’s say you need to write a VBA macro to assign bonuses to employees based on their performance rating. The ratings are on a scale from 1 to 5, with higher ratings earning larger bonuses.
Sub AssignBonus()
Dim performanceRating As Integer
Dim bonusAmount As Double
performanceRating = 4
Select Case performanceRating
Case 1
bonusAmount = 0
Case 2
bonusAmount = 500
Case 3
bonusAmount = 1000
Case 4
bonusAmount = 2000
Case 5
bonusAmount = 3000
Case Else
bonusAmount = 0
MsgBox "Invalid rating"
End Select
MsgBox "The bonus amount is: $" & bonusAmount
End Sub
In this scenario:
The macro assigns a bonus amount based on the employee's performance rating.
If the rating is outside the expected range (1 to 5), the
Case Else
block handles it by setting the bonus to zero and displaying an "Invalid rating" message.
8. Best Practices
Use
Select Case
for Multiple Conditions: Opt forSelect Case
instead ofIf...Then...ElseIf
when you have more than three or four conditions, as it is more readable and maintainable.Handle All Possible Values: Always include a
Case Else
to handle unexpected values, ensuring your code doesn't fail silently.Avoid Overusing Ranges: While ranges are powerful, overusing them can make your
Select Case
statement harder to read. Use them judiciously.Keep It Organized: Ensure that the values and ranges in your
Case
statements are logically ordered for better readability.
Summary
Basic Structure: Consists of an expression and multiple
Case
clauses, with an optionalCase Else
for unmatched cases.Handling Multiple Values and Ranges:
Case
clauses can match single values, multiple values, or ranges of values.Nested
Select Case
: Useful for handling complex scenarios where one decision depends on the outcome of another.Real-Time Scenarios: Commonly used in scenarios like categorizing data, assigning values based on ratings, or responding to different user inputs.
The Select Case
statement is a powerful tool for simplifying complex decision-making processes in your VBA code.
- Looping: For...Next, For Each...Next, Do While, Do Until
Functionality: Loops allow you to repeat actions multiple times, either for a fixed number of iterations (`For...Next`) or until a condition is met (`Do While` or
Do Until
).Real-time Scenario: Automating data entry by looping through rows in a dataset and applying formulas or formatting.
Looping allows you to execute a block of code repeatedly, which is useful for tasks like processing items in a collection, performing repetitive calculations, or iterating through rows in a spreadsheet. VBA provides several types of loops, each suited to different scenarios.
1. For...Next Loop
Purpose: The For...Next
loop is used to execute a block of code a specific number of times. It is typically used when you know in advance how many iterations you need.
Basic Syntax:
For counter = start To end [Step stepValue]
' Code to execute during each iteration
Next counter
counter: A variable that controls the loop, typically an integer.
start: The initial value of the counter.
end: The value at which the loop stops.
Step: An optional parameter that specifies the increment (or decrement) for each iteration. The default is 1.
Example:
Sub PrintNumbers()
Dim i As Integer
For i = 1 To 10
Debug.Print i ' Prints numbers 1 to 10 in the Immediate Window
Next i
End Sub
Real-Time Scenario: Suppose you need to apply a 10% discount to the prices in the first 10 rows of a column in an Excel sheet:
Sub ApplyDiscount()
Dim i As Integer
For i = 1 To 10
Cells(i, 2).Value = Cells(i, 2).Value * 0.9 ' Assuming prices are in column B
Next i
End Sub
2. For Each...Next Loop
Purpose: The For Each...Next
loop is used to iterate through all the elements in a collection or an array. It’s particularly useful when working with collections like worksheets, workbooks, or ranges in Excel.
Basic Syntax:
For Each element In group
' Code to execute during each iteration
Next element
element: A variable that represents each item in the collection during each iteration.
group: The collection or array being iterated over.
Example:
Sub ListSheetNames()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
Debug.Print ws.Name ' Prints the name of each worksheet in the Immediate Window
Next ws
End Sub
Real-Time Scenario: Imagine you need to hide all sheets in a workbook except for the active one:
Sub HideOtherSheets()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
If ws.Name <> ActiveSheet.Name Then
ws.Visible = xlSheetHidden
End If
Next ws
End Sub
3. Do While Loop
Purpose: The Do While
loop is used to execute a block of code as long as a specified condition is True
. It’s useful when you don’t know in advance how many times you’ll need to loop, but you know you want to continue looping while a condition holds true.
Basic Syntax:
Do While condition
' Code to execute while the condition is True
Loop
- condition: A Boolean expression that is evaluated before each iteration. If
True
, the loop continues; ifFalse
, the loop stops.
Example:
Sub CountDown()
Dim i As Integer
i = 10
Do While i > 0
Debug.Print i ' Prints 10 down to 1 in the Immediate Window
i = i - 1
Loop
End Sub
Real-Time Scenario: Suppose you want to find the first empty cell in column A:
Sub FindFirstEmptyCell()
Dim i As Integer
i = 1
Do While Not IsEmpty(Cells(i, 1))
i = i + 1
Loop
MsgBox "First empty cell is in row " & i
End Sub
4. Do Until Loop
Purpose: The Do Until
loop is similar to the Do While
loop but runs until a specified condition becomes True
. The loop continues as long as the condition is False
.
Basic Syntax:
Do Until condition
' Code to execute while the condition is False
Loop
- condition: A Boolean expression that is evaluated before each iteration. The loop continues until this condition is
True
.
Example:
Sub CountUp()
Dim i As Integer
i = 1
Do Until i > 10
Debug.Print i ' Prints 1 to 10 in the Immediate Window
i = i + 1
Loop
End Sub
Real-Time Scenario: Let’s say you want to keep summing values in column B until the sum reaches a threshold of 1000:
Sub SumUntilThreshold()
Dim sumTotal As Double
Dim i As Integer
i = 1
Do Until sumTotal > 1000
sumTotal = sumTotal + Cells(i, 2).Value
i = i + 1
Loop
MsgBox "Reached the threshold after " & (i - 1) & " rows."
End Sub
Summary
For Each...Next: Ideal for iterating over all items in a collection or array.
Do While: Useful for looping as long as a condition is true.
Do Until: Continues looping until a condition becomes true.
Each loop structure is suited to different tasks, and understanding when to use each will make your VBA code more efficient and easier to understand.
2. Intermediate VBA
# Working with Excel Objects
- Understanding Excel Object Model
Functionality: The Excel Object Model is a hierarchy of objects (like Workbook, Worksheet, Range) that you can control using VBA.
Real-time Scenario: Navigating through a workbook programmatically to copy data from one sheet to another or generate summary reports.
- Worksheets, Workbooks, Ranges, and Cells
Functionality: These are the fundamental objects in Excel VBA. You can manipulate them to perform tasks like selecting ranges, copying data, and more.
Real-time Scenario: Writing a script to loop through multiple workbooks, extract data from specific ranges, and compile them into a master sheet.
- Methods and Properties of Excel Objects
Functionality: Properties are attributes of objects (like a cell's value or a sheet's name), while methods are actions that can be performed on objects (like saving a workbook).
Real-time Scenario: Changing the properties of a range to format cells, such as setting the font color based on specific criteria.
# User-Defined Functions (UDFs)
- Creating Custom Functions
Functionality: UDFs are custom functions you can create to perform calculations that Excel doesn’t provide by default.
Real-time Scenario: Creating a UDF to calculate commission based on a complex set of rules that standard Excel functions cannot handle.
- Using UDFs in Excel Sheets
Functionality: Once created, UDFs can be used in Excel just like any other function (e.g.,
=MyCustomFunction(A1, B1)
).Real-time Scenario: Using a UDF in a financial model to calculate the internal rate of return (IRR) with customized parameters.
# Error Handling
- Types of Errors
Functionality: Errors in VBA can be syntax errors, runtime errors, or logical errors. Understanding these helps in writing robust code.
Real-time Scenario: Catching and handling errors that might occur when a user enters invalid data, such as a non-numeric value in a numeric field.
- Error Handling with
On Error
StatementFunctionality: The
On Error
statement allows you to define how your code should react when an error occurs.Real-time Scenario: Implementing error handling in a macro that processes data files, ensuring that the macro skips over problematic files and continues running.
- Debugging Tools (Breakpoints, Watch Window, Immediate Window)
Functionality: These tools help you find and fix errors in your VBA code by allowing you to pause execution, inspect variables, and execute code interactively.
Real-time Scenario: Using breakpoints to step through a macro that isn’t producing the expected results and inspecting variables to find the issue.
# Interacting with Other Applications
- Using VBA to Control Other MS Office Applications
Functionality: VBA can be used to automate tasks across different Office applications, like Word, Outlook, or PowerPoint.
Real-time Scenario: Automating the creation of Word documents with data from Excel, such as generating personalized letters from a mailing list.
- Automating Emails with VBA
Functionality: You can use VBA to automate sending emails from Excel, often through Outlook.
Real-time Scenario: Sending a batch of personalized emails to a list of clients with their respective data attached, such as invoices or reports.
# UserForms
- Creating and Designing UserForms
Functionality: UserForms provide a graphical interface for users to interact with your VBA applications. You can add controls like text boxes, buttons, and drop-down lists.
Real-time Scenario: Creating a UserForm to collect user input, such as order details or survey responses, and process them in Excel.
- Working with Controls (TextBox, ComboBox, ListBox, etc.)
Functionality: Controls are elements on UserForms that allow users to enter or select data.
Real-time Scenario: Using a ComboBox on a UserForm to let users select from a list of products, which then populates other fields like price and stock level.
- Handling Form Events
Functionality: Events are actions like clicking a button or changing a selection that trigger VBA code to run.
Real-time Scenario: Handling the
Click
event of a button to validate and submit data entered in a UserForm.3. Advanced VBA
# Advanced Data Handling
- Arrays (Static and Dynamic)
Functionality: Arrays store multiple values in a single variable. Static arrays have a fixed size, while dynamic arrays can be resized as needed.
Real-time Scenario: Using a dynamic array to store and process data from a large range of cells, such as calculating averages or finding specific values.
- Dictionaries and Collections
Functionality: Dictionaries and collections are advanced data structures that store key-value pairs, allowing for efficient data retrieval.
Real-time Scenario: Storing customer information in a dictionary, where each key is a customer ID and the value is a set of attributes like name and purchase history.
- Working with External Data Sources
Functionality: VBA can connect to and manipulate data from external sources like databases (e.g., Access, SQL) or web services.
Real-time Scenario: Writing a macro to pull sales data from an SQL database, process it in Excel, and generate a summary report.
# Class Modules
- Creating and Using Class Modules
Functionality: Class modules allow you to define custom objects in VBA with properties, methods, and events.
Real-time Scenario: Creating a
Customer
class with properties likeName
,Email
, and methods likeSendEmail
. This allows you to manage customer data more effectively in your code.- Understanding Properties, Methods, and Events
Functionality: Properties store data within an object, methods perform actions, and events respond to specific actions.
Real-time Scenario: Implementing a
Project
class in a project management tool, where properties store project details, methods calculate deadlines, and events notify of overdue tasks.- Implementing Custom Objects
Functionality: Custom objects created through class modules encapsulate data and functionality, making your code more modular and reusable.
Real-time Scenario: Using a custom object to manage inventory items, including tracking stock levels, calculating reorder points, and generating purchase orders.
# File Handling
- Reading from and Writing to Text Files
Functionality: VBA can open, read, write, and close text files, allowing you to process data stored in external files.
Real-time Scenario: Automating the process of importing data from a CSV file into Excel, processing it, and exporting the results to a new text file.
- Working with XML and JSON Files
Functionality: VBA can parse and generate XML and JSON files, which are common formats for data exchange.
Real-time Scenario: Parsing a JSON file containing API data, extracting relevant information, and inserting it into an Excel sheet.
- Automating File Operations
Functionality: VBA can automate tasks like copying, moving, renaming, or deleting files.
Real-time Scenario: Creating a macro to backup important Excel files to a specific folder and clean up old files based on their age.
# Optimizing and Protecting Code
- Improving Code Performance
Functionality: Techniques like avoiding unnecessary calculations, reducing screen updating, and using efficient data structures can make your code run faster.
Real-time Scenario: Optimizing a macro that processes a large dataset by disabling screen updates and using efficient looping structures.
- Code Protection Techniques
Functionality: Protecting your VBA code from unauthorized access or modification using password protection and obfuscation.
Real-time Scenario: Protecting a VBA project in a financial model to prevent users from tampering with critical calculations.
- Best Practices for Writing Clean, Maintainable Code
Functionality: Following best practices like modular coding, commenting, and naming conventions makes your code easier to understand and maintain.
Real-time Scenario: Refactoring a complex macro into smaller, reusable procedures that can be tested and debugged independently.
# API and Advanced Integration
- Calling Windows APIs from VBA
Functionality: You can extend the capabilities of VBA by calling functions from the Windows API to perform tasks that aren’t natively supported in VBA.
Real-time Scenario: Using a Windows API call to display a custom dialog box or interact with the file system in ways that standard VBA functions do not allow.
- Integrating with Web Services
Functionality: VBA can interact with web services via APIs (e.g., REST) to retrieve or send data over the internet.
Real-time Scenario: Writing VBA code to pull real-time financial data from a web service and update an Excel dashboard.
Subscribe to my newsletter
Read articles from Rahul Nadola directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
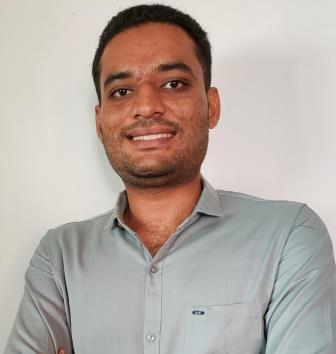
Rahul Nadola
Rahul Nadola
DevOps Enthusiast