Python for Financial Data Analysis Using Custom Indicators and Algorithms
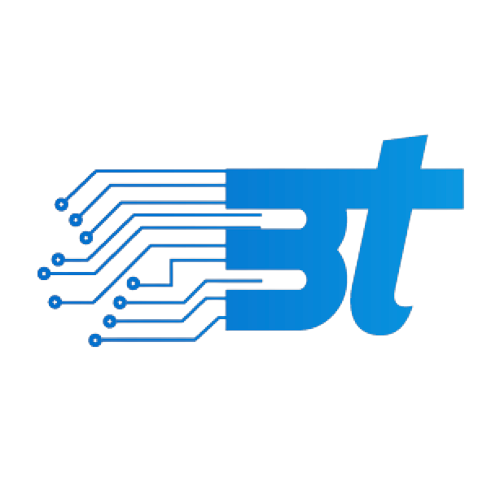
Table of contents
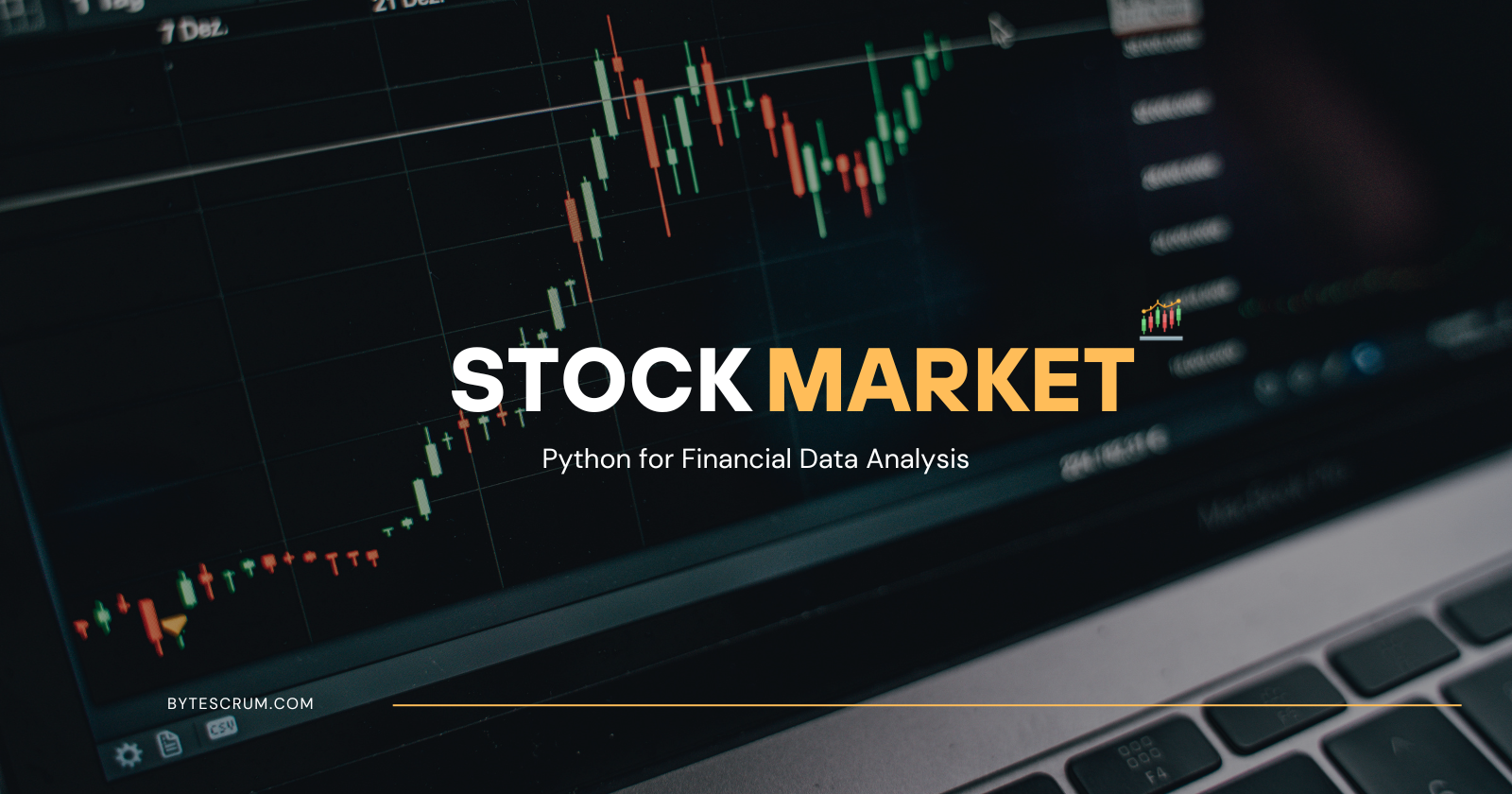
Financial data analysis is a critical component of making informed investment decisions. With Python, a powerful and versatile programming language, you can build your own financial indicators and algorithms to analyze market data and predict future trends. This tutorial will guide you through the process of using Python for financial data analysis, including setting up your environment, fetching market data, creating custom indicators, and implementing basic trading algorithms.
Why Python for Financial Data Analysis?
Python is an excellent choice for financial data analysis due to its simplicity, readability, and extensive ecosystem of libraries tailored for data analysis, visualization, and machine learning. Libraries like Pandas, NumPy, Matplotlib, and TA-Lib make it easier to manipulate data, calculate indicators, and visualize trends.
Getting Started
Step 1: Set Up Your Python Environment
To start, ensure that you have Python installed on your machine along with some essential libraries. You can use pip
to install them:
pip install pandas numpy matplotlib yfinance
Pandas: For data manipulation and analysis.
NumPy: For numerical operations.
Matplotlib: For plotting and visualization.
yfinance: To fetch financial data from Yahoo Finance.
Step 2: Fetch Financial Data
You can use the yfinance
library to easily download historical stock data. Here’s how to fetch daily stock data for a particular company, such as Apple (AAPL):
import yfinance as yf
# Fetch historical data for Apple
ticker = 'AAPL'
data = yf.download(ticker, start='2020-01-01', end='2023-01-01')
# Display the first few rows of data
print(data.head())
Step 3: Calculate Basic Indicators
Indicators are mathematical calculations based on the price, volume, or open interest of a security. They are used to predict future price movements. Let’s calculate some basic indicators like the Moving Average (MA) and Exponential Moving Average (EMA).
# Import necessary libraries
import pandas as pd
# Calculate the 20-day Moving Average
data['MA20'] = data['Close'].rolling(window=20).mean()
# Calculate the 50-day Exponential Moving Average
data['EMA50'] = data['Close'].ewm(span=50, adjust=False).mean()
# Display the first few rows of data with indicators
print(data[['Close', 'MA20', 'EMA50']].head())
Step 4: Plot the Data and Indicators
Visualizing your data along with the indicators can provide insights into market trends and potential buy or sell signals.
import matplotlib.pyplot as plt
plt.figure(figsize=(14, 7))
plt.plot(data['Close'], label='Close Price')
plt.plot(data['MA20'], label='20-Day MA')
plt.plot(data['EMA50'], label='50-Day EMA')
plt.title('AAPL Stock Price with MA and EMA')
plt.xlabel('Date')
plt.ylabel('Price')
plt.legend()
plt.show()
Step 5: Develop Custom Indicators
Let’s develop a custom indicator, such as the Relative Strength Index (RSI), which measures the speed and change of price movements.
def calculate_rsi(data, window):
delta = data['Close'].diff()
gain = (delta.where(delta > 0, 0)).rolling(window=window).mean()
loss = (-delta.where(delta < 0, 0)).rolling(window=window).mean()
rs = gain / loss
rsi = 100 - (100 / (1 + rs))
return rsi
# Add RSI to data
data['RSI'] = calculate_rsi(data, window=14)
# Display the first few rows of data with RSI
print(data[['Close', 'RSI']].head())
Step 6: Implement a Basic Trading Algorithm
Using the calculated indicators, you can implement a basic trading strategy. For example, a simple RSI-based trading algorithm might look like this:
def trading_strategy(data):
buy_signals = []
sell_signals = []
position = False # Track whether we currently have a position
for i in range(len(data)):
if data['RSI'][i] < 30: # Buy signal
if not position:
buy_signals.append(data['Close'][i])
sell_signals.append(float('nan'))
position = True
else:
buy_signals.append(float('nan'))
sell_signals.append(float('nan'))
elif data['RSI'][i] > 70: # Sell signal
if position:
buy_signals.append(float('nan'))
sell_signals.append(data['Close'][i])
position = False
else:
buy_signals.append(float('nan'))
sell_signals.append(float('nan'))
else:
buy_signals.append(float('nan'))
sell_signals.append(float('nan'))
return buy_signals, sell_signals
data['Buy_Signal_Price'], data['Sell_Signal_Price'] = trading_strategy(data)
plt.figure(figsize=(14, 7))
plt.plot(data['Close'], label='Close Price', alpha=0.35)
plt.scatter(data.index, data['Buy_Signal_Price'], label='Buy Signal', marker='^', color='green', lw=3)
plt.scatter(data.index, data['Sell_Signal_Price'], label='Sell Signal', marker='v', color='red', lw=3)
plt.title('AAPL Stock Price with Buy and Sell Signals')
plt.xlabel('Date')
plt.ylabel('Price')
plt.legend()
plt.show()
Step 7: Test and Optimize Your Algorithm
Testing and optimizing your trading algorithm is crucial for real-world applications. You can use techniques like backtesting with historical data, adjusting parameters, or implementing more sophisticated strategies like machine learning models for prediction.
Disclaimer: This script is for educational purposes only. We are not responsible for any financial decisions made based on this script. Always conduct your own research or consult with a professional before making any financial decisions.
Conclusion
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
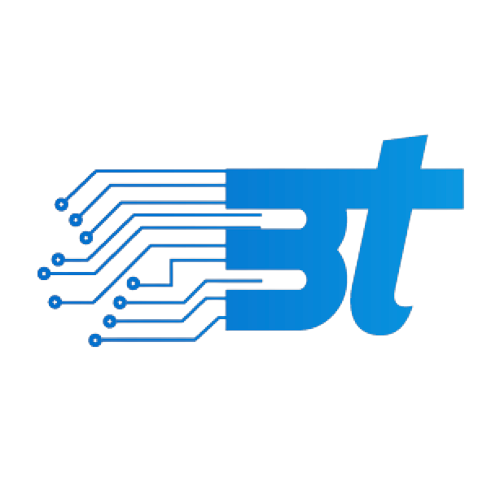
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.