Async vs. Isolates in Flutter: Mastering Parallelism for High-Performance Apps

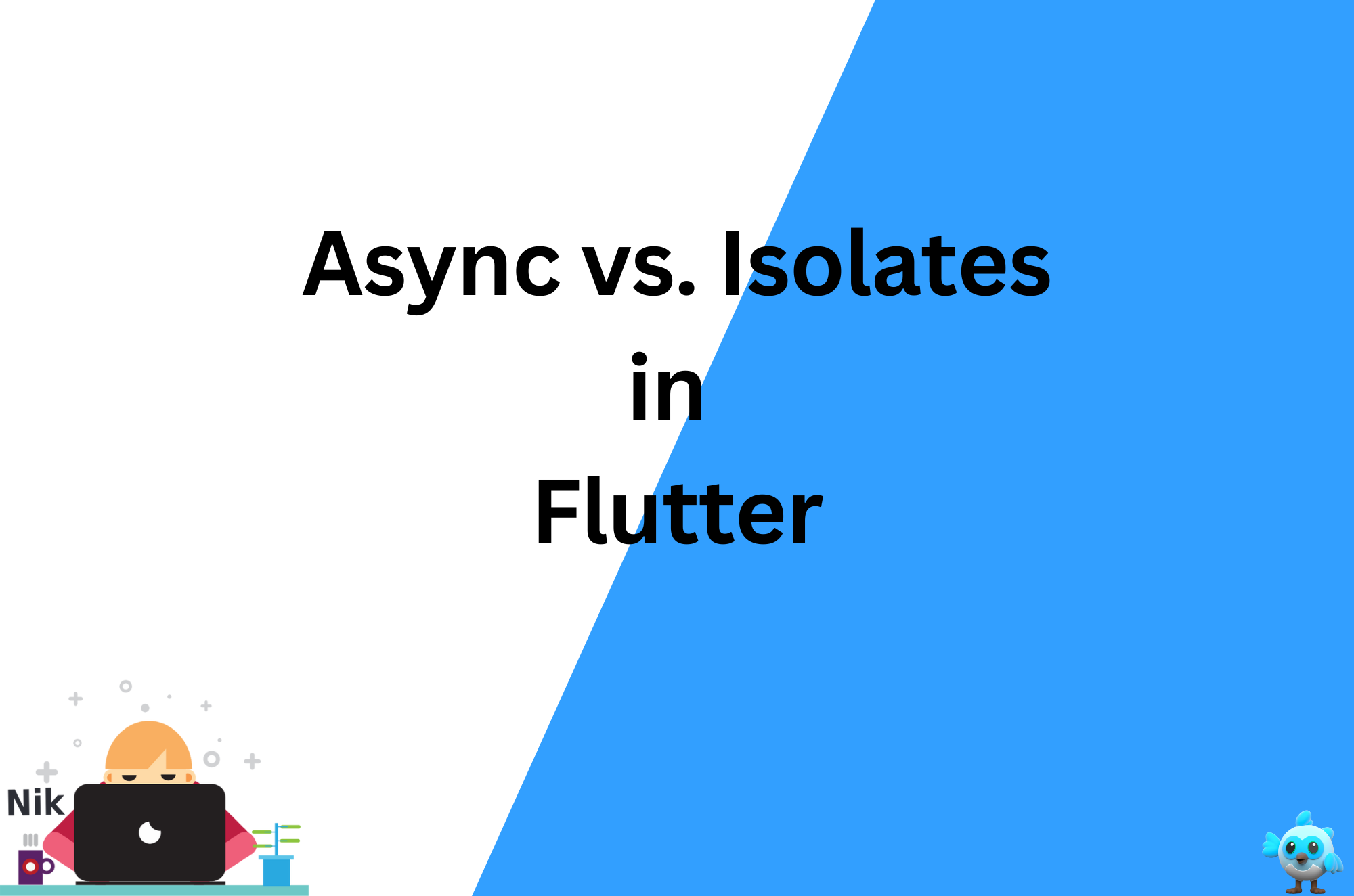
When building high-performance apps with Flutter, one of the key considerations is how to efficiently manage tasks that may take a significant amount of time, such as network requests, file I/O, or complex computations. To keep the app responsive and smooth, Flutter provides two primary mechanisms for handling these tasks: async
and Isolates
. Both are crucial for managing parallelism, but they serve different purposes and are suited to different types of tasks. In this blog, we'll explore the differences between async
and Isolate
, and when to use each in your Flutter apps.
Understanding Parallelism in Flutter
Before diving into async
and Isolate
, it's important to understand how Dart, the language behind Flutter, handles parallelism. Dart runs in a single-threaded environment, meaning it processes one task at a time. To keep the UI responsive, Dart needs to handle long-running tasks in a way that doesn’t block the main thread (also known as the UI thread). This is where async
and Isolate
come into play.
Async: Handling Non-Blocking Operations
async
is a keyword in Dart that allows you to perform non-blocking operations. It’s primarily used for tasks that involve waiting, such as fetching data from an API, reading a file, or waiting for a timer to finish. When you mark a function as async
, it means the function will return a Future
and will not block the thread while waiting for the result. This allows the UI to remain responsive.
Example: Using Async for Network Requests
Future<void> fetchData() async {
final response = await http.get(Uri.parse('https://api.example.com/data'));
if (response.statusCode == 200) {
// Process the data
print('Data fetched successfully');
} else {
throw Exception('Failed to load data');
}
}
In the example above, the fetchData
function is marked as async
, and it uses await
to wait for the network request to complete. The main thread remains free to handle other tasks while the data is being fetched, keeping the UI responsive.
When to Use Async
Use async
for tasks that involve I/O operations, such as:
Fetching data from an API.
Reading or writing files.
Waiting for user input.
Any task where the main delay is waiting for an external process.
Isolate: True Parallelism in Dart
While async
allows for non-blocking operations, it doesn’t provide true parallelism. For compute-intensive tasks that need to run concurrently, Dart provides Isolates
. An Isolate
is a separate thread of execution, with its own memory and event loop. Unlike async
, which runs on the main thread, Isolates
run in their own thread, making them ideal for heavy computations.
Example: Using Isolates for Heavy Computation
import 'dart:isolate';
void heavyComputation(SendPort sendPort) {
int result = 0;
for (int i = 0; i < 1000000000; i++) {
result += i;
}
sendPort.send(result);
}
void startIsolate() async {
final receivePort = ReceivePort();
await Isolate.spawn(heavyComputation, receivePort.sendPort);
receivePort.listen((data) {
print('Result from isolate: $data');
receivePort.close();
});
}
In this example, heavyComputation
is a function that performs a compute-intensive task. By running it in an Isolate
, the main thread remains responsive while the computation is handled in a separate thread. The result is sent back to the main thread using a SendPort
.
When to Use Isolates
Use Isolates
for tasks that require heavy computation, such as:
Processing large datasets.
Performing complex mathematical operations.
Image processing or video encoding.
Any task where the main delay is due to CPU-bound operations.
Async vs. Isolate: Which One to Choose?
Use
async
when the task involves waiting for something external, like I/O operations. It's perfect for tasks that are mostly idle, allowing the main thread to continue executing other code while waiting for a result.Use
Isolate
when the task is CPU-intensive and needs to be run in parallel. This is important for tasks that would otherwise block the main thread and make the app unresponsive.
Conclusion
Understanding when to use async
and when to use Isolates
is key to building efficient, high-performance Flutter apps. While async
is great for handling I/O-bound tasks without blocking the main thread, Isolates
provide true parallelism for compute-intensive tasks. By choosing the right tool for the job, you can ensure your Flutter apps remain responsive and performant, no matter what tasks they need to handle.
Happy coding! 🚀
Subscribe to my newsletter
Read articles from Ravi Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ravi Patel
Ravi Patel
"📱 Passionate Flutter Developer with 6+ years of experience, dedicated to crafting exceptional mobile applications. 🚀 Adept at turning ideas into polished, user-friendly experiences using Flutter's magic. 🎨 Design enthusiast who believes in the power of aesthetics and functionality. 🛠️ Expertise in translating complex requirements into clean, efficient code. 🌟 Committed to staying updated with the latest trends and continuously pushing boundaries. Let's create something extraordinary together!"