How to Build a GUI Calculator with PyQt and Python?
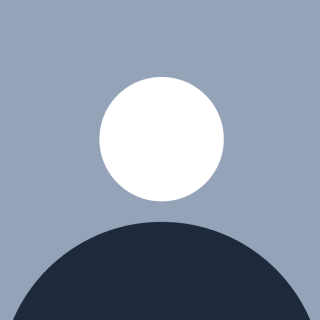
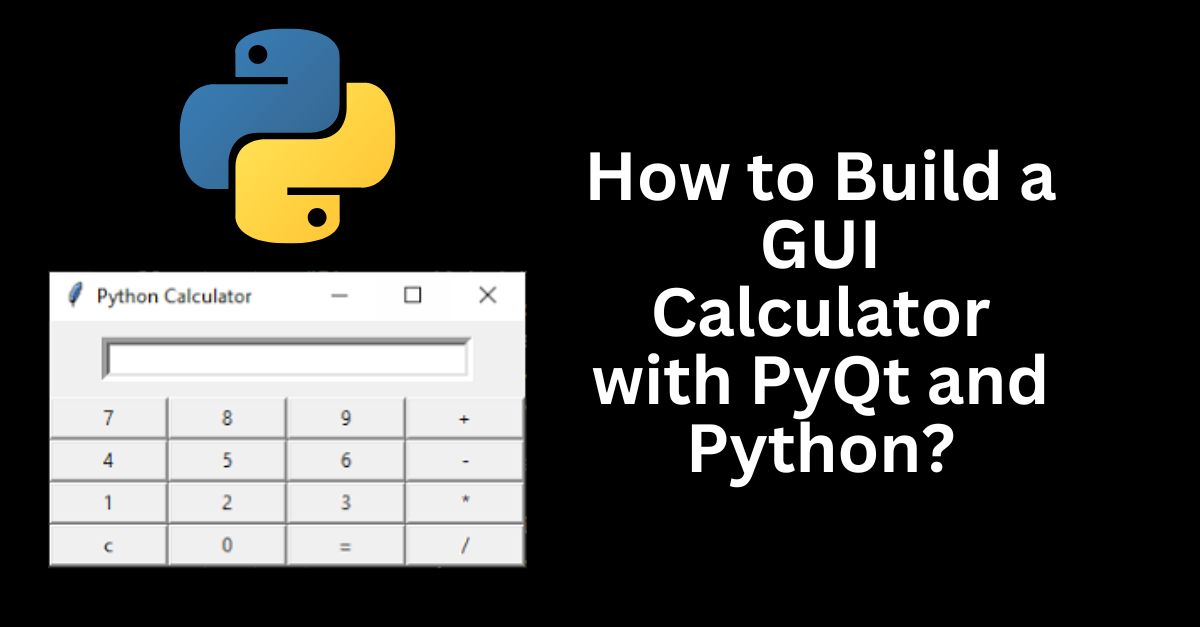
Since Python is one of the most popular languages these days, and since more complex projects include file operations, it will be good to know how we can simulate a text file in python. This guide explores how Python, with its libraries like Fale Process Interface and BytesI/O etc., can help you simulate a text file which comes in extremely useful when performing tests or processing data.
Understanding Why We Need To Simulate Text File in Python
Python text file simulation is a nifty trick to work not only the real files on disk but also other file-like objects without creating actual files. This can be especially beneficial in cases where you need to:
Don't create temporary files (test file operations)
Store Data In-Memory for Fast Performance.
Streamline pipelines in data processing workflows.
Configuration of Python Environment
Get your python environment ready before we move on to code. You'll need:
Python Installed: Ensure to install the latest version of Python on your computer.
Required Libraries: Ensure that you have any required libraries such as io installed on your Python distribution.
Step By Step Guide of Text File Simulation in Python
We will guide a user on how to test the text file by simulating it in Python and implementing StringIO module from the io library of python.
Using StringIO from the io Module
One is the StringIO
class which allows you to create a text file-like object in memory.
```python
from io import StringIO
```
Create an-text file in memory:
If you want to emulate a text file, create a StringIO object and prime it as:
```python
simulated_file = StringIO()
```
Writing Data to the text Feign File
Writing the StringIO
Object The data is written to a file-like object, just as it would with an actual file.
```python
simulated_file. This is not an actual text file. Write("") \n")
simulated_file. write("It is a normal file in memory. \n")
```
Reading Data from the Simulated Text File
You write your data and you can read that from StringIO
object as:
```python
simulated_file. seek (0) # move the cursor back to top wages-1
content = simulated_file. read()
print(content)
Mocking text files has a lot of advantages, esp. useful while testing and working with some sort of data.
Efficiency: In-memory operations are much faster than disk access.
Flexibility, you can test file operations without needing disk storage.
Ease of Use: Makes life easier by managing the temporary files for you.
Common applications to simulated text files
The ability to simulate text files is useful in many places:
Testing File Operations: To test the read, write operation without actually creating files.
Data Processing Pipelines: Simplify data messages between solutions such as ETL systems.
Temporary Data Storage: Save temporary data that doesn't require to save on disk in-memory files.
Testing File Operations
from io import StringIO
def read_file(file):
content = file.read()
return content
# Simulating a file
simulated_file = StringIO("This is a test file content.")
# Testing the function
print(read_file(simulated_file)) # Output: This is a test file content.
Data Processing Pipelines
from io import StringIO
def process_data(file):
lines = file.readlines()
processed_lines = [line.strip().upper() for line in lines]
return processed_lines
# Simulating a file
data = """line one
line two
line three"""
simulated_file = StringIO(data)
# Processing the data
processed_data = process_data(simulated_file)
print(processed_data) # Output: ['LINE ONE', 'LINE TWO', 'LINE THREE']
Temporary Data Storage
from io import StringIO
def temporary_storage():
Simulating a file
simulated_file = StringIO()
simulated_file.write("This data is temporarily stored in memory.\n")
simulated_file.write("No need to create an actual file on disk.")
Moving the cursor to the beginning
simulated_file.seek(0)
Reading the content
return simulated_file.read()
# Using the temporary storage
print(temporary_storage())
Simulating File Uploads
from io import StringIO
def handle_upload(file):
Simulate processing of an uploaded file
content = file.read()
return f"File content: {content}"
# Simulating a file upload
uploaded_file = StringIO("This is the content of the uploaded file.")
print(handle_upload(uploaded_file)) # Output: File content: This is the content of the uploaded file.
Config Parsing
from io import StringIO
import configparser
def parse_config(file):
config = configparser.ConfigParser()
config.read_file(file)
return config
# Simulating a config file
config_data = """
[Settings]
theme = dark
language = en
"""
simulated_config_file = StringIO(config_data)
# Parsing the config
config = parse_config(simulated_config_file)
print(config['Settings']['theme']) # Output: dark
Python Developers for Advanced File Handling Jobs
Next time you need to hire Python developers for projects that involve competent file processing, remember.
Key Skills to Look For
Expertise in Python I/O: A developer should know how to read and write files with the io module.
Data Processing: Experience dealing with data streams, and in-memory data manipulation.
Problem-Solving Abilities: The developer must have the ability to easily debug and optimize file operations.
Where can you even hire trusted Python developers from?
Platforms: Sites such as Techcospace, Upwork and Toptal give you an opportunity to hire professional freelancers.
Conclusion
Regardless of whether you are testing some file operations or working with a data processing kind of issue, knowing how to properly mock files in memory is crucial. This will be key, when you go out and employ Python developers that are competent enough to handle these types of work.
Subscribe to my newsletter
Read articles from TechCospace directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
TechCospace
TechCospace
Techcospace is a top IT & Software outsourcing and Staff Augmentation Company in USA. Hire offshore developers on contract as per your need.