How to Automate Desktop Notifications for Important Events Using Python
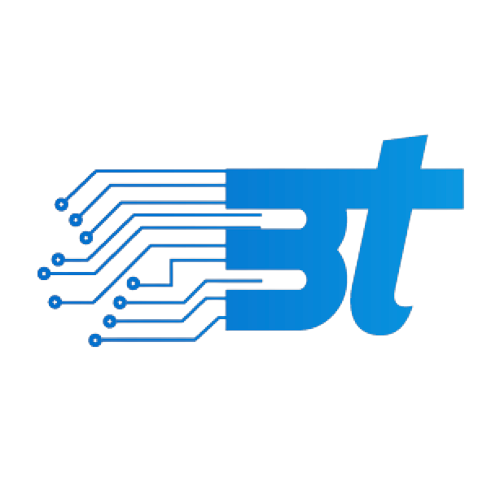
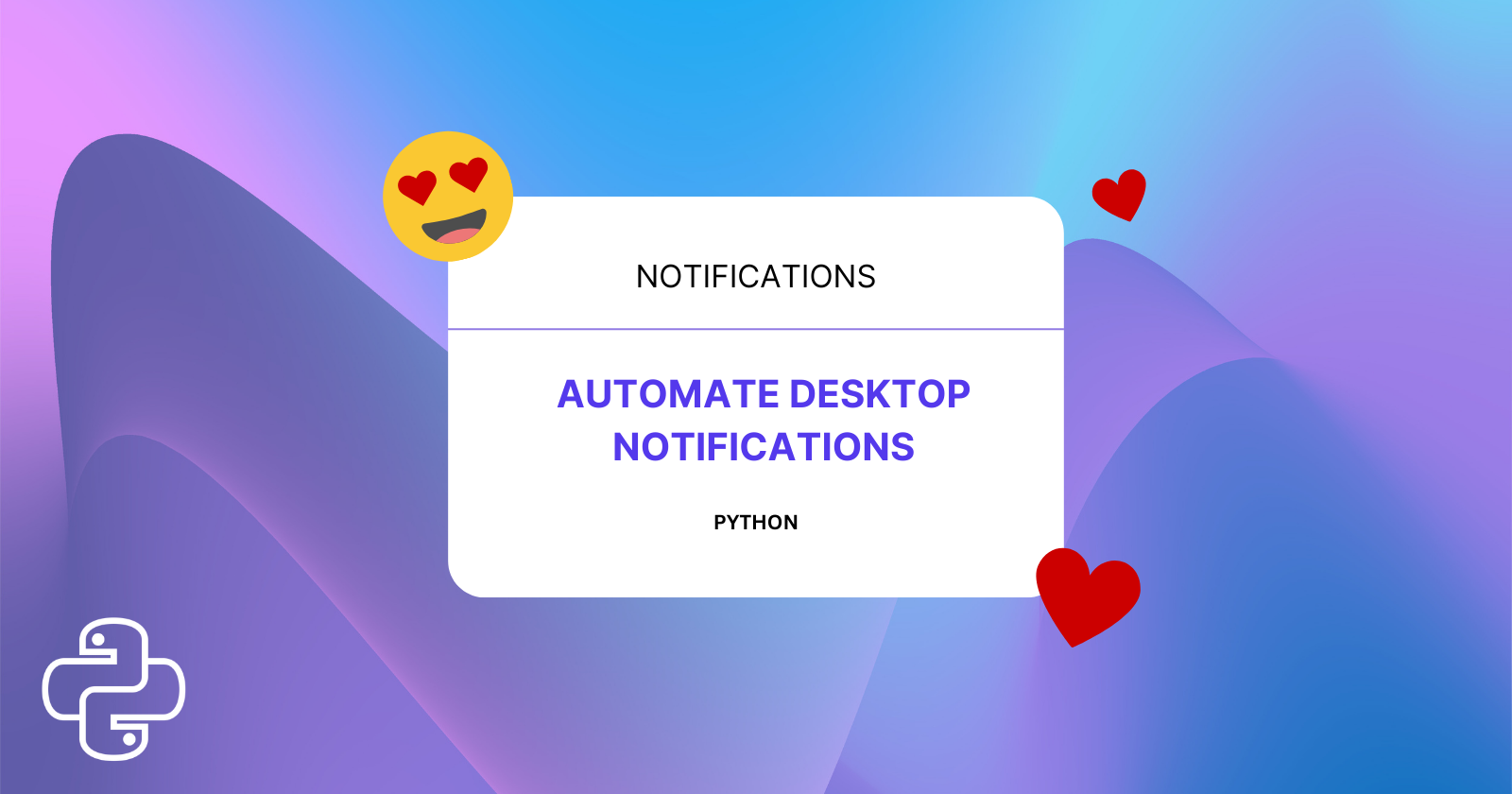
Do you often miss important events or reminders? Automating desktop notifications can be a great way to stay organized and ensure you never miss an important moment again. In this blog, we will guide you through the process of creating a Python script to automate desktop notifications for key events using the plyer
library.
Why Use Desktop Notifications?
Desktop notifications are a handy tool to remind you of critical tasks or events without the need to check your calendar or email constantly. They pop up on your screen, grabbing your attention immediately. This is especially useful for meetings, deadlines, or any other time-sensitive tasks.
What You Will Need
To follow along, you will need:
Python installed on your computer.
A text editor or an IDE (like VSCode or PyCharm).
Basic knowledge of Python programming.
Step 1: Install the Required Libraries
To create desktop notifications in Python, we will use the plyer
library, which is a simple, cross-platform Python library for accessing features commonly found on various platforms.
First, you need to install the plyer
library. Open your terminal or command prompt and run:
pip install plyer pyobjus
Step 2: Write the Python Script
Now, let's write a Python script that will display desktop notifications.
Import Necessary Modules
Start by importing the necessary modules. We need
Notification
fromplyer
andtime
to control the timing of notifications.from plyer import notification import time
Define a Function for Notifications
We'll create a function called
send_notification
that takes in the title, message, and duration of the notification:def send_notification(title, message, duration=5): notification.notify( title=title, message=message, timeout=duration )
Set Up a Schedule for Notifications
Now, let's create a loop to send notifications at regular intervals. This example will send a notification every hour, reminding the user to take a break:
if __name__ == "__main__": while True: send_notification( title="Break Reminder", message="It's time to take a break and stretch!", duration=10 ) # Sleep for an hour (3600 seconds) time.sleep(3600)
This script will display a notification with the title "Break Reminder" and the message "It's time to take a break and stretch!" every hour.
Step 3: Customize Your Notifications
The script above is a basic example. You can customize it further to suit your needs. Here are a few ideas:
Different Notifications for Different Events: Create a dictionary with event names as keys and times as values. Then, check the current time to determine which notification to show.
Integrate with Your Calendar: Use the
google-api-python-client
to integrate with Google Calendar and fetch events dynamically. This way, you can automate notifications for events directly from your calendar.
Step 4: Run Your Script
To run your script:
Save the script in a
.py
file, for example,notification_
script.py
.Open your terminal or command prompt.
Navigate to the directory where your script is saved.
Run the script by typing:
python notification_script.py
Conclusion
Happy coding, and stay notified!
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
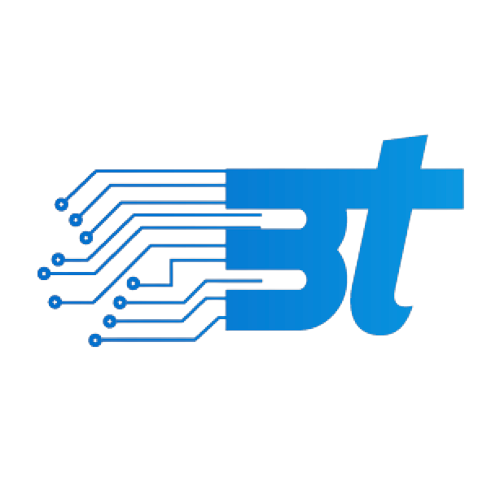
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.