Exploring Python's itertools Module: A Comprehensive Guide
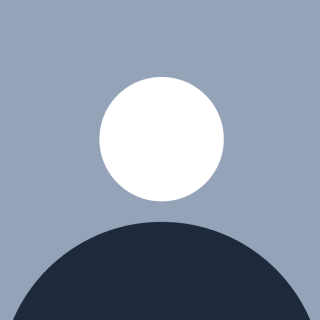
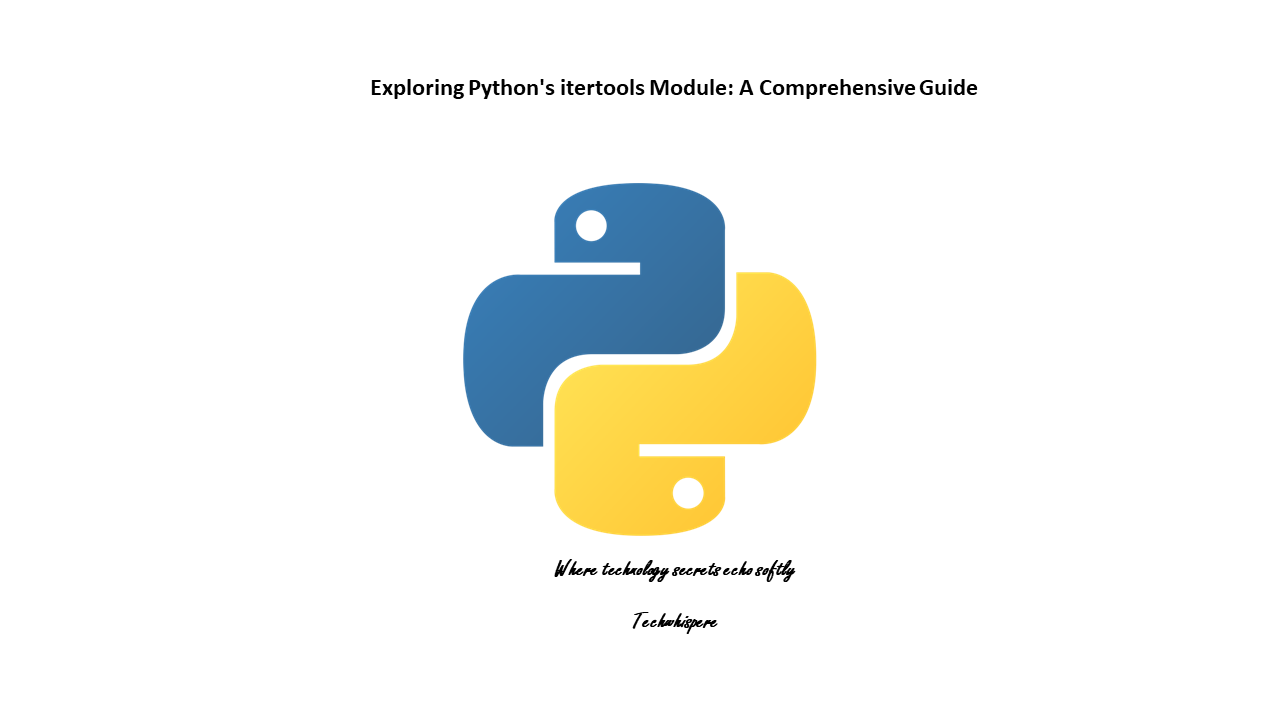
Introduction
Python's itertools
module is a hidden gem in the standard library, offering a collection of fast, memory-efficient tools that handle iterators. These functions are designed to operate on iterators, making them highly useful for handling data streams, generating sequences, and performing combinatorial operations. This blog will dive deep into itertools
, exploring its various functions and how you can leverage them to write more efficient and readable Python code.
The itertools
module in Python is a standard library that provides a collection of tools for creating and working with iterators. Iterators are objects that represent a stream of data and can be iterated (looped) over. The itertools
module allows you to efficiently combine, split, and transform iterators, making it easier to manipulate data.
Using itertools
, you can generate infinite sequences, create Cartesian products, chain multiple iterables together, and much more. The functions in itertools
can be grouped into three main categories:
Infinite Iterators: Generate infinite sequences.
Finite Iterators: Work with finite iterables.
Combinatorial Iterators: Generate combinations, permutations, and Cartesian products.
Infinite Iterators
Infinite iterators are useful when you need to generate a stream of data that could go on indefinitely. These iterators don’t stop on their own, so you must be careful when using them in loops or with functions that consume iterators.
count(start=0, step=1)
The count()
function generates an infinite sequence of numbers, starting from start
and incrementing by step
.
import itertools
for num in itertools.count(10, 2):
print(num)
if num > 20:
break
Output:
10
12
14
16
18
20
22
This function is particularly useful when you need a counter in a loop or when working with iterators that need an index.
cycle(iterable)
The cycle()
function repeatedly cycles through the elements of an iterable indefinitely.
import itertools
colors = ['red', 'green', 'blue']
cycled_colors = itertools.cycle(colors)
for i in range(10):
print(next(cycled_colors))
Output:
red
green
blue
red
green
blue
red
green
blue
red
This is useful for iterating over a sequence in a circular manner.
repeat(object, times=None)
The repeat()
function repeats an object a specified number of times. If times
is not provided, it repeats indefinitely.
import itertools
for item in itertools.repeat('A', 5):
print(item)
Output:
A
A
A
A
A
repeat()
can be useful for creating constant sequences or for testing purposes.
Finite Iterators
Finite iterators operate on finite iterables and transform them in various ways. These are some of the most commonly used tools in itertools
.
chain(*iterables)
The chain()
function takes multiple iterables as arguments and returns a single iterable that yields elements from the first iterable until it’s exhausted, then proceeds to the next iterable, and so on.
import itertools
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
chained = itertools.chain(list1, list2)
print(list(chained))
Output:
[1, 2, 3, 'a', 'b', 'c']
chain()
is useful when you want to iterate over multiple sequences as if they were a single sequence.
compress(data, selectors)
The compress()
function filters elements from data
, returning only those that have a corresponding element in selectors
that evaluates to True
.
import itertools
data = ['a', 'b', 'c', 'd']
selectors = [1, 0, 1, 0]
result = itertools.compress(data, selectors)
print(list(result))
Output:
['a', 'c']
This is particularly useful when you want to filter data based on a set of conditions.
dropwhile(predicate, iterable) and takewhile(predicate, iterable)
dropwhile()
drops elements from the iterable as long as the predicate isTrue
, then returns the rest.takewhile()
returns elements from the iterable as long as the predicate isTrue
, then stops.
import itertools
data = [1, 4, 6, 7, 9, 3, 2]
print(list(itertools.dropwhile(lambda x: x < 7, data))) # [7, 9, 3, 2]
print(list(itertools.takewhile(lambda x: x < 7, data))) # [1, 4, 6]
These functions are useful when you want to split an iterable into two parts based on a condition.
filterfalse(predicate, iterable)
The filterfalse()
function returns elements of the iterable where the predicate is False
.
import itertools
data = [1, 2, 3, 4, 5]
result = itertools.filterfalse(lambda x: x % 2, data)
print(list(result))
Output:
[2, 4]
This is the inverse of the built-in filter()
function.
accumulate(iterable, func=operator.add)
The accumulate()
function returns accumulated sums (or other binary operations) of elements in the iterable.
import itertools
import operator
data = [1, 2, 3, 4, 5]
result = itertools.accumulate(data, operator.mul)
print(list(result))
Output:
[1, 2, 6, 24, 120]
accumulate()
is handy for cumulative operations like running totals, product calculations, etc.
groupby(iterable, key=None)
The groupby()
function groups adjacent elements in an iterable that have the same key. The key is a function that computes a key value for each element.
import itertools
# Group items by even or odd
data = [1, 1, 2, 2, 3, 3, 4, 4]
grouped = itertools.groupby(data, key=lambda x: x % 2 == 0)
for key, group in grouped:
print(key, list(group))
Output:
False [1, 1]
True [2, 2]
False [3, 3]
True [4, 4]
The groupby()
function groups the elements in data
based on whether they are even or odd. The key
function used here checks if each element is even.
Example Use Cases
Generating Cartesian Products
Imagine you need to create all possible combinations of two sets of items, such as shirt sizes and colors.
import itertools
sizes = ['S', 'M', 'L']
colors = ['Red', 'Blue', 'Green']
combinations = itertools.product(sizes, colors)
print(list(combinations))
[('S', 'Red'), ('S', 'Blue'), ('S', 'Green'), ('M', 'Red'), ('M', 'Blue'), ('M', 'Green'), ('L', 'Red'), ('L', 'Blue'), ('L', 'Green')]
Filtering Data Streams
import itertools
data_stream = [0.2, 0.8, 0.9, 0.1, 0.5, 0.4]
filtered_data = itertools.filterfalse(lambda x: x < 0.5, data_stream)
print(list(filtered_data))
[0.8, 0.9, 0.5]
Conclusion
The itertools
module is a treasure trove for Python developers, offering efficient and elegant solutions to common problems involving iteration. Whether you are generating combinations, filtering data, or working with infinite sequences, itertools
provides a rich set of tools that can simplify your code and improve its performance.
By mastering the itertools
module, you can elevate your Python programming skills, making your code more Pythonic and efficient. Start experimenting with these functions today, and you'll quickly see how they can enhance your coding toolkit.
Subscribe to my newsletter
Read articles from Loga Rajeshwaran Karthikeyan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by