Create a Python Bot for Automating Online Transactions
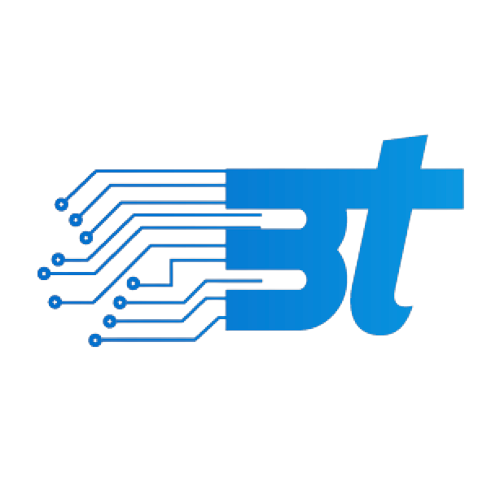
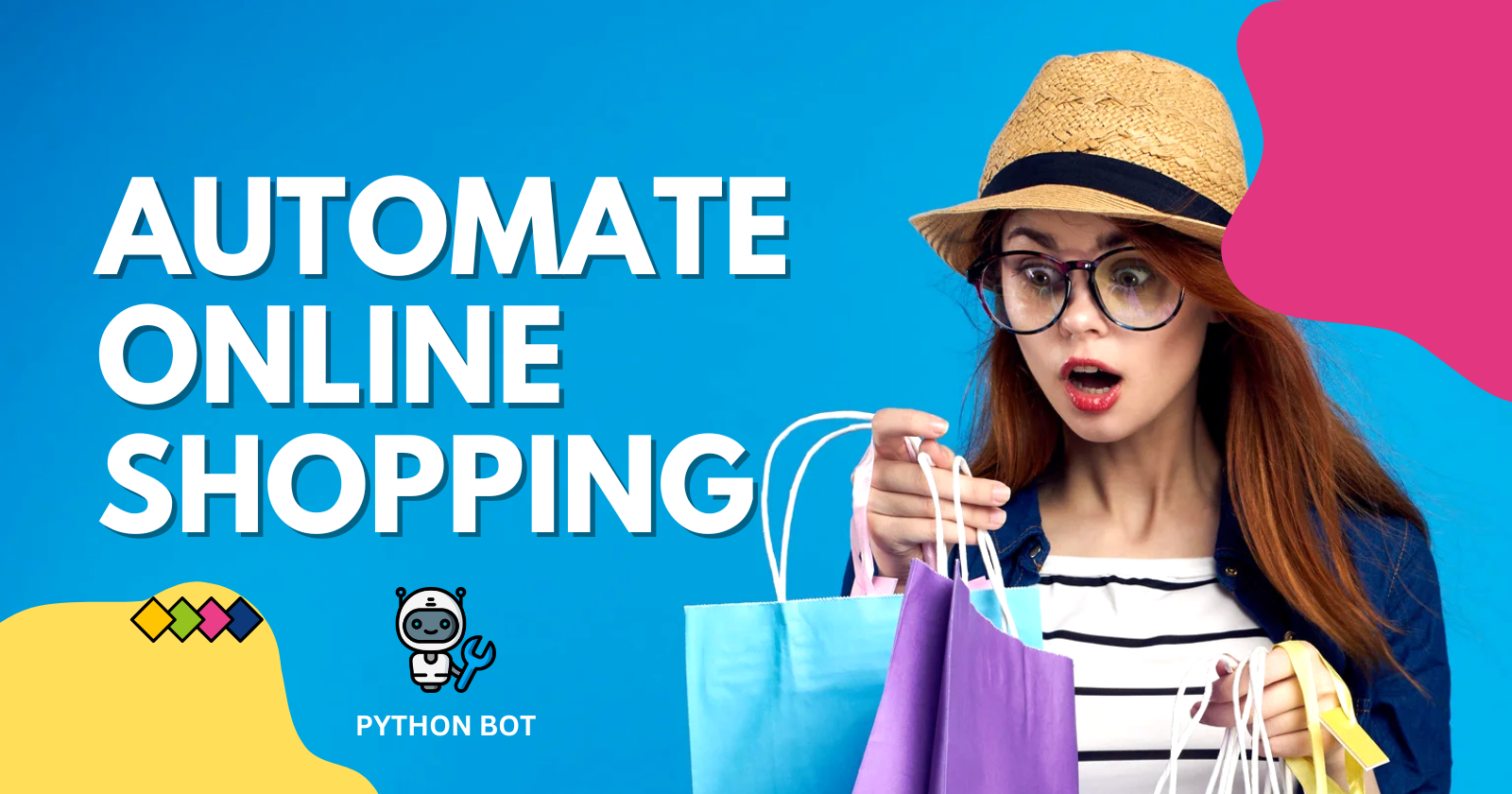
Automating online transactions can be incredibly useful for a variety of purposes, such as managing financial operations, making recurring purchases, or automating trading on financial markets. In this blog post, we will guide you through creating a Python bot that can automate online transactions. We'll cover the basics of web scraping, interacting with websites, and ensuring your bot runs smoothly and securely.
Step-by-Step Guide to Building a Python Transaction Bot
1. Setting Up Your Environment
To begin, you'll need Python installed on your machine, along with some additional libraries for web automation:
pip install selenium requests beautifulsoup4
Selenium: Used for browser automation.
Requests: For making HTTP requests to websites.
BeautifulSoup: For parsing HTML content.
You'll also need a web driver that Selenium can use to control your browser. For Chrome, download the ChromeDriver from here, and make sure it's in your system path.
2. Basic Structure of the Python Bot
Let's start by writing a basic Python script to automate logging into a website.
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
import time
# Initialize the Chrome driver
driver = webdriver.Chrome()
# Open the login page
driver.get("https://example.com/login")
# Locate login elements and log in
username = driver.find_element(By.NAME, "username")
password = driver.find_element(By.NAME, "password")
login_button = driver.find_element(By.NAME, "submit")
username.send_keys("your_username")
password.send_keys("your_password")
login_button.click()
# Wait for the login process to complete
time.sleep(5)
Replace "
https://example.com/login
"
, "username"
, "password"
, and "submit"
with the actual values for the website you want to automate.
3. Navigating the Website and Performing Transactions
Once logged in, you can navigate the website and perform transactions. For example, to automate a purchase, you need to:
Navigate to the Product Page:
# Go to the product page driver.get("https://example.com/product")
Add the Product to the Cart:
# Click the 'Add to Cart' button add_to_cart_button = driver.find_element(By.ID, "add-to-cart") add_to_cart_button.click()
Proceed to Checkout and Complete the Transaction:
# Go to the checkout page driver.get("https://example.com/checkout") # Enter payment information (be careful with sensitive data!) card_number = driver.find_element(By.NAME, "card_number") expiration_date = driver.find_element(By.NAME, "expiration_date") cvv = driver.find_element(By.NAME, "cvv") pay_button = driver.find_element(By.NAME, "pay") card_number.send_keys("1234 5678 9012 3456") expiration_date.send_keys("12/24") cvv.send_keys("123") pay_button.click() # Confirm payment and complete the transaction time.sleep(5) # Wait for transaction to process
Handling Errors and Timeouts
Web automation can be unpredictable due to varying page load times, unexpected pop-ups, or network issues. Here's how you can handle these situations:
Timeouts: Use
WebDriverWait
to wait for elements to load.from selenium.webdriver.common.by import By from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.support import expected_conditions as EC # Wait for the 'Add to Cart' button to appear add_to_cart_button = WebDriverWait(driver, 10).until( EC.presence_of_element_located((By.ID, "add-to-cart")) )
Try-Except Blocks: Catch exceptions and handle them gracefully.
try: pay_button.click() except Exception as e: print(f"Error encountered: {e}") driver.quit() # Exit if there's an error
Security Considerations
Protect Sensitive Information: Never hard-code sensitive information like passwords or credit card details in your scripts. Use environment variables or encrypted vaults.
Use Proxies and Rotate IPs: To avoid being blocked by websites for repetitive actions, use proxies and rotate IP addresses.
Respect Website Terms of Service: Ensure your bot does not violate the terms of service of any website.
Automating and Scheduling the Bot
Use Python's schedule
library to run your bot at specific times:
pip install schedule
Example of scheduling the bot:
import schedule
import time
def job():
print("Starting the transaction bot...")
# Call your bot function here
schedule.every().day.at("10:00").do(job)
while True:
schedule.run_pending()
time.sleep(1)
Conclusion
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
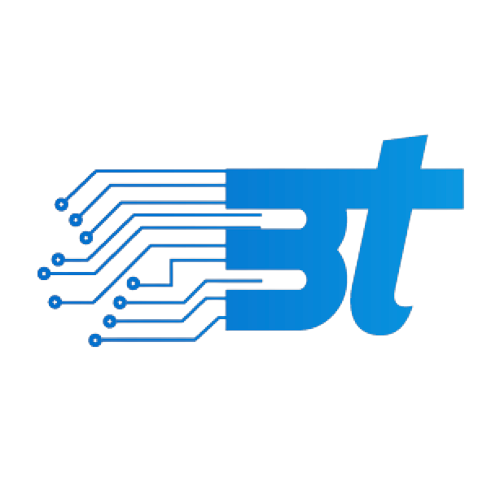
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.