How to rickroll your friends using System calls
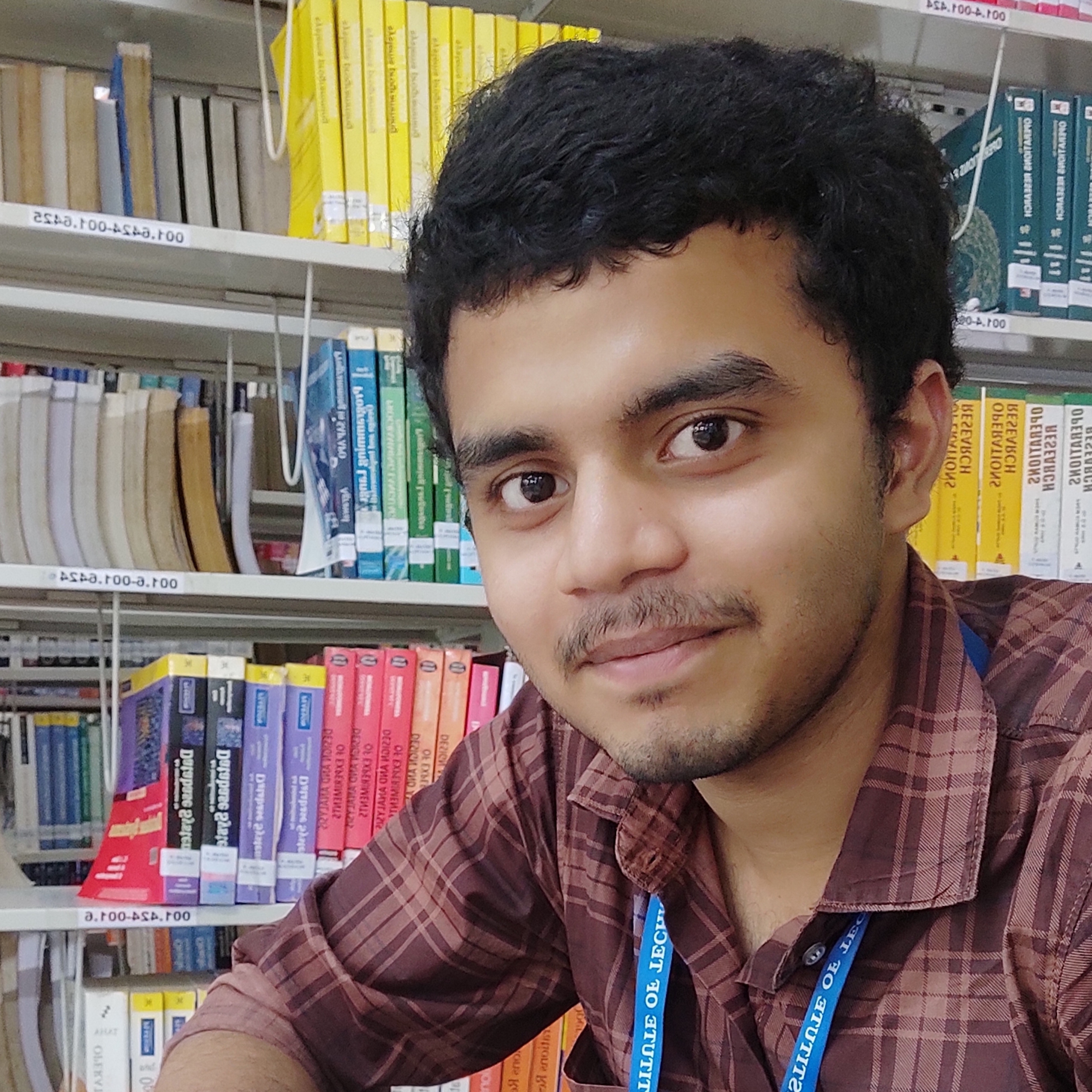
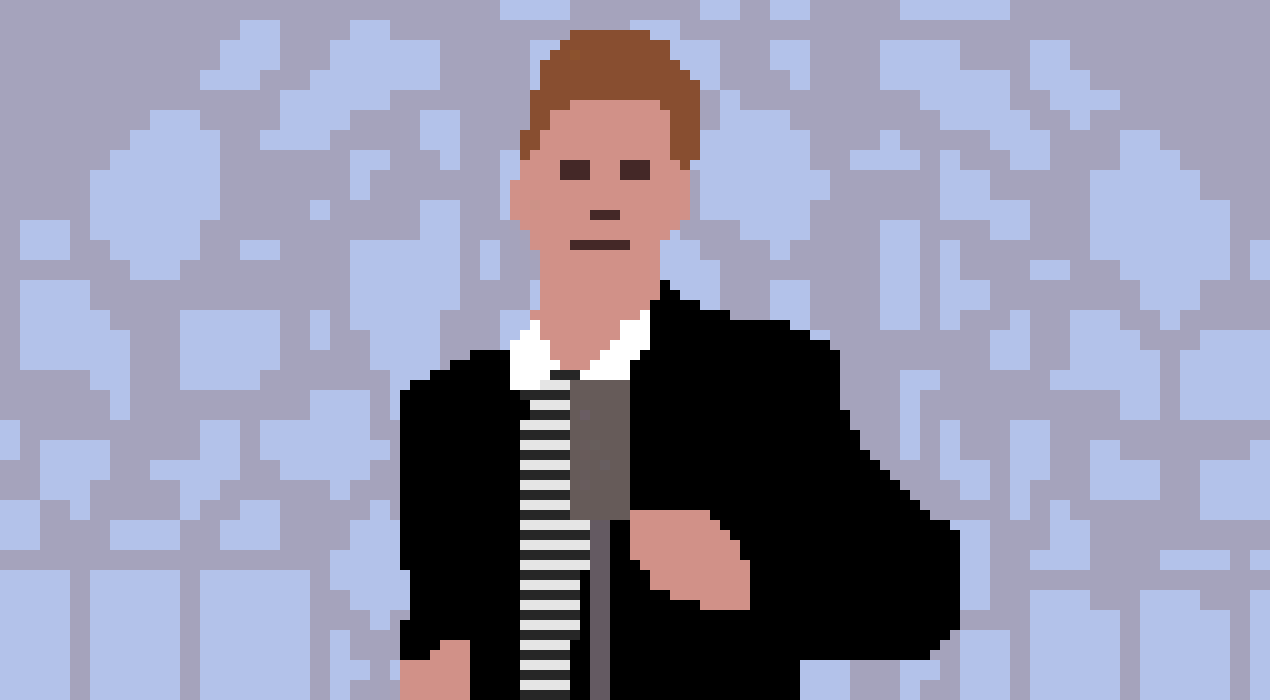
Lets bring back the trend of rickrolling!!.
While being taught about event handlers and execl system calls in my Software Systems course, I had an idea. What if I could make a C executable that opens browser and play Rick Astley's classic "never gonna give you up!".
Now, let us get into it.
for macOS and Unix - based systems
execl() system call
the execl command can be used to run an executable on the hard disk. the binary image of the C executable is now replaced with the application executable path you provide.
The first argument, by convention contains the path of the application being executed, and then there exists a list of arguments.
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
int main() {
char *url = "https://www.youtube.com/watch?v=dQw4w9WgXcQ";
if (execl("/usr/bin/open", "open", "-a", "/Applications/Safari.app", url, NULL) == -1) {
perror("execl");
exit(EXIT_FAILURE);
}
return 0;
}
For Windows systems
start command
The start is a built-in command in Windows that opens a URL in the default browser. The command is then formatted using snprintf() function, so that it can run in a shell.
system() function runs the command in a new shell, from C/C++ programs. Using these three, the following code can be built:-
#include <windows.h>
#include <stdio.h>
int main() {
const char *url = "https://www.youtube.com/watch?v=dQw4w9WgXcQ";
char command[256];
// Prepare the command to open the URL
snprintf(command, sizeof(command), "start %s", url);
// Create a new process to execute the command
if (system(command) == -1) {
perror("Failed to open browser");
return 1;
}
return 0;
}
Now, just obscure the code intent by passing on an executable file to your friends and rick roll them!
Subscribe to my newsletter
Read articles from Peddinti Sriram Bharadwaj directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
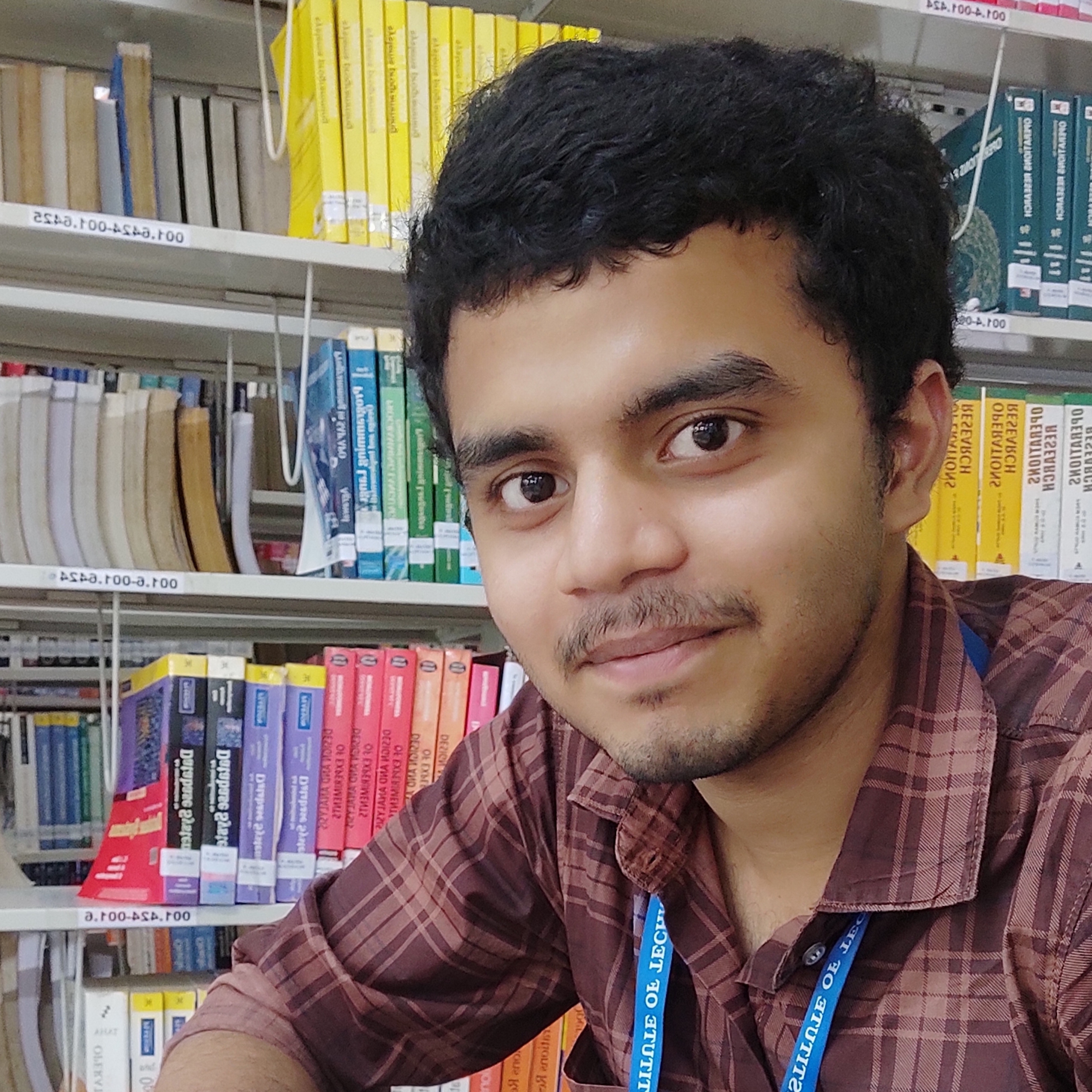
Peddinti Sriram Bharadwaj
Peddinti Sriram Bharadwaj
I am a learner