Creating a Python Bot for Auto-Completing CAPTCHA Challenges
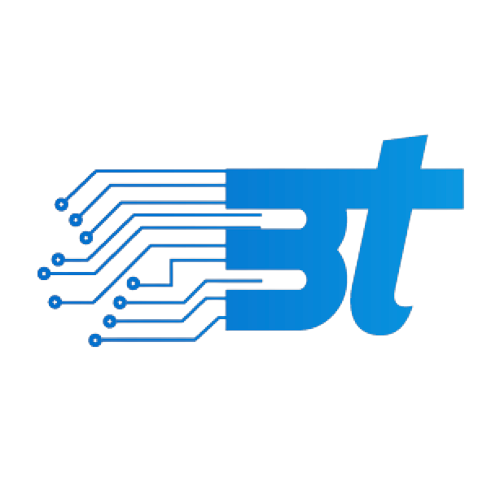
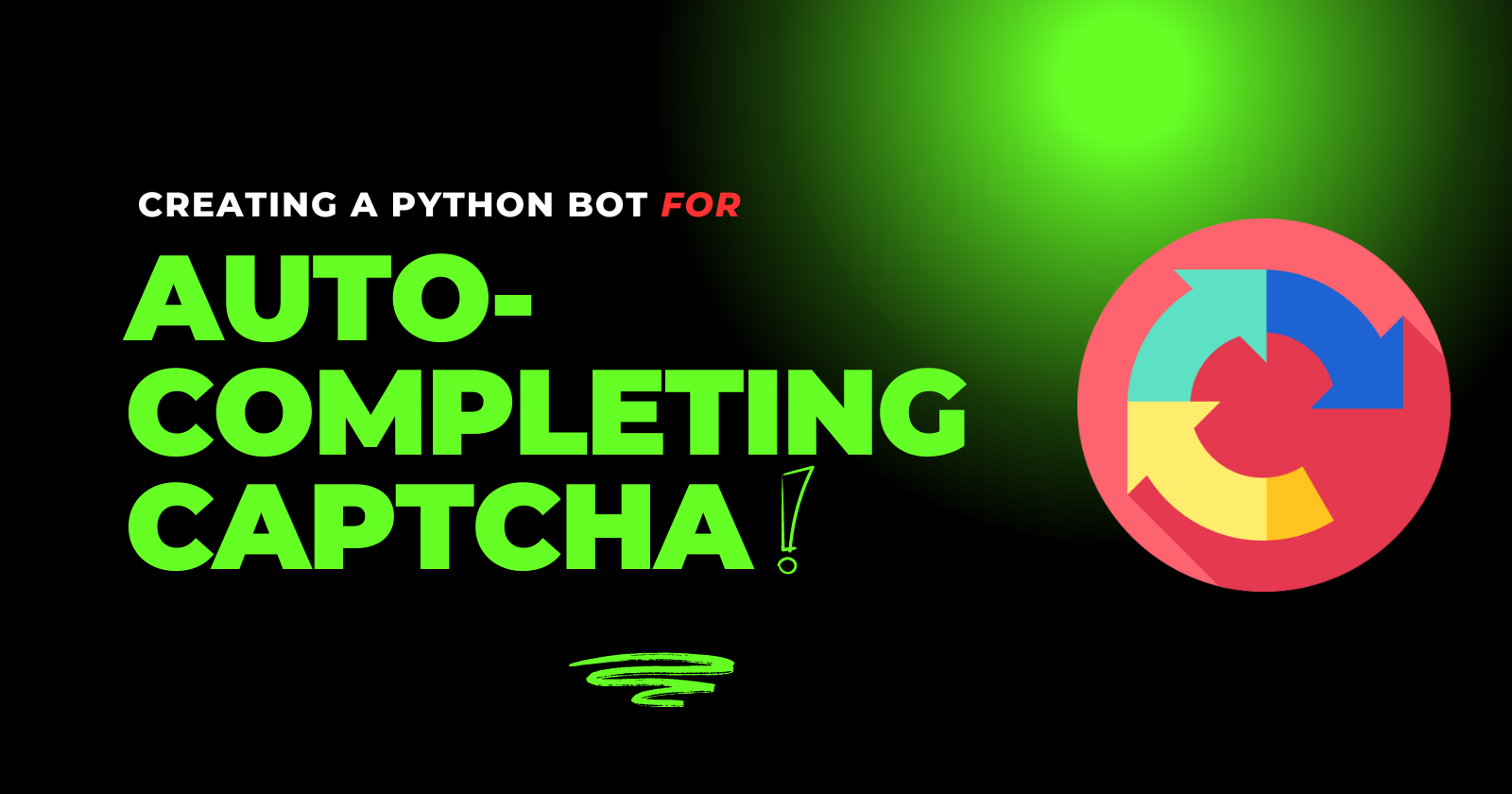
CAPTCHAs (Completely Automated Public Turing test to tell Computers and Humans Apart) are a common security measure used on websites to prevent bots from performing automated tasks. However, in some cases, you might want to build a Python bot capable of solving CAPTCHAs automatically. This blog post will guide you through creating a Python bot for auto-completing CAPTCHA challenges while discussing the ethical considerations and legalities involved.
Disclaimer:
Understanding CAPTCHA Challenges
CAPTCHAs come in various forms:
Text-Based CAPTCHAs: These require users to identify and input distorted text.
Image-Based CAPTCHAs: These ask users to select images based on a given criterion (e.g., "Select all images with traffic lights").
ReCAPTCHA v2 and v3: Google’s CAPTCHA services that use advanced algorithms to differentiate humans from bots.
For this tutorial, we’ll focus on text-based CAPTCHAs since they are simpler and easier to demonstrate.
Tools and Libraries Required
To build a Python bot for solving text-based CAPTCHAs, you will need:
Python: Make sure Python is installed on your system.
requests: For making HTTP requests.
Pillow: A Python Imaging Library (PIL) fork for image processing.
Tesseract OCR: An open-source optical character recognition engine to convert images of text into machine-readable text.
Selenium: For automating web browser interaction.
Installation of Required Libraries
You can install these libraries using pip:
pip install requests Pillow pytesseract selenium
Additionally, install Tesseract OCR:
Windows: Download the installer from Tesseract's official GitHub repository.
macOS: Use Homebrew:
brew install tesseract
.Linux: Install via package manager, e.g.,
sudo apt-get install tesseract-ocr
.
Step-by-Step Guide to Building the CAPTCHA Bot
Step 1: Set Up Selenium WebDriver
Selenium is used to automate browser interaction. Download the WebDriver for your browser (Chrome, Firefox, etc.) and make sure it's in your system's PATH.
Here’s how to set up Selenium WebDriver:
from selenium import webdriver
# Initialize WebDriver (example for Chrome)
driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
Step 2: Access the CAPTCHA Page
Navigate to the page with the CAPTCHA using Selenium:
driver.get("http://example.com/captcha-page")
Step 3: Locate and Download CAPTCHA Image
Use Selenium to find and download the CAPTCHA image:
from PIL import Image
import requests
from io import BytesIO
# Find the CAPTCHA image element
captcha_element = driver.find_element_by_id('captcha_image_id')
# Get the CAPTCHA image source URL
captcha_url = captcha_element.get_attribute('src')
# Download the CAPTCHA image
response = requests.get(captcha_url)
captcha_image = Image.open(BytesIO(response.content))
# Save CAPTCHA image locally (optional)
captcha_image.save("captcha.png")
Step 4: Solve the CAPTCHA Using Tesseract OCR
Tesseract OCR can extract text from the CAPTCHA image. Here’s how to use it:
import pytesseract
# Convert CAPTCHA image to string using Tesseract OCR
captcha_text = pytesseract.image_to_string(captcha_image)
print("Extracted CAPTCHA text:", captcha_text)
Note: For text-based CAPTCHAs, preprocessing the image (e.g., converting to grayscale, increasing contrast) can improve OCR accuracy.
Step 5: Submit the Solved CAPTCHA
Use Selenium to input the extracted CAPTCHA text into the appropriate field and submit the form:
# Locate the CAPTCHA text input field and submit button
captcha_input = driver.find_element_by_id('captcha_input_id')
submit_button = driver.find_element_by_id('submit_button_id')
# Enter the CAPTCHA text
captcha_input.send_keys(captcha_text.strip())
# Click the submit button
submit_button.click()
Step 6: Handle Potential Errors and Improve Accuracy
CAPTCHA solving isn’t foolproof, especially with text distortion or noisy images. Here are a few tips to improve accuracy:
Image Preprocessing: Convert images to grayscale, adjust contrast, or apply noise reduction techniques.
Error Handling: Use a loop to retry CAPTCHA solving if the first attempt fails.
Use More Advanced Machine Learning Models: For complex CAPTCHAs, consider training a neural network model to recognize characters or patterns.
Ethical Considerations and Legal Implications
While it’s technically feasible to create bots that solve CAPTCHAs, it’s crucial to remember the following:
Respect Website Policies: Automating CAPTCHA solving often violates the terms of service of websites, potentially leading to legal consequences.
Ethical Usage: This guide should only be used for educational purposes or personal projects where automation is allowed.
Consider Alternative Approaches: If you’re automating legitimate processes, consider reaching out to website owners for API access or other alternatives.
Conclusion
Happing Coding!
For any software development queries, click here.
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
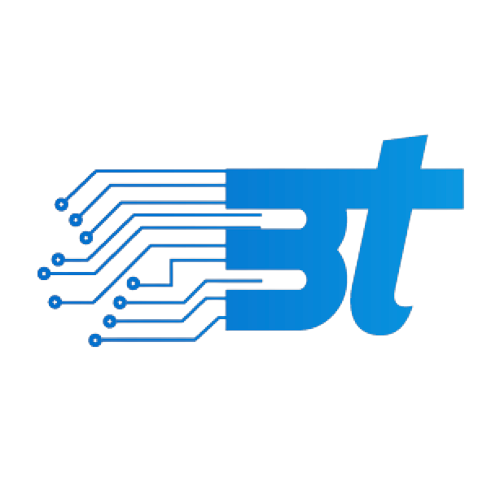
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.