Basic Linux Shell Scripting For DevOps
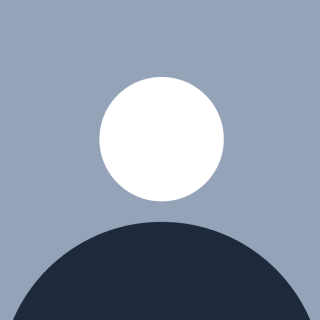
What is Shell Scripting?
Linux shell scripting allows you to automate tasks, manage systems, and streamline development processes. Shell scripts are essentially sequences of commands stored in a file, which can be executed like a program.
Creating and Running a Shell Script:
You need a text editor like: vim, nano, etc. To create shell script.
ubuntu@ip-172-31-23-251:~$ vim hello.sh: this command will open a file of name hello.sh where in which we can write our shell script.
Write the following in a file named hello.sh:
#!/bin/bash
echo "Hello! Devops"
~
~
-- INSERT -- 5,1 All
Shell scripts typically start with a “shebang” (#!
) that tells the system which interpreter to use for the script. press 'i' to insert and write into the file
Press 'esc' and then write column ':wq' . To Save the file and exit the editor.
ubuntu@ip-172-31-23-251:~$ ls
ubuntu@ip-172-31-23-251:~$ vim hello.sh
ubuntu@ip-172-31-23-251:~$ ls
check the permissions of hello.sh by following command
ubuntu@ip-172-31-23-251:~$ ls -l
total 4
-rw-rw-r-- 1 ubuntu ubuntu 34 Sep 3 07:09 hello.sh
hello.sh don't have executable permission give it executable permission by following command
ubuntu@ip-172-31-23-251:~$ chmod +x hello.sh
ubuntu@ip-172-31-23-251:~$ ls -l
total 4 -rwxrwxr-x 1 ubuntu ubuntu 34 Sep 3 07:09 hello.sh
ubuntu@ip-172-31-23-251:~$ ls
hello.sh have given the executable command. Run the script file by following command
ubuntu@ip-172-31-23-251:~$ ./hello.sh
Hello! DevOps
ubuntu@ip-172-31-23-251:~$
Lets Move on to Basics:
Variables:
#!/bin/bash
#'name' is a variable
name="DevOps Engineer"
echo "Hello, $name!"
Input from User:
#!/bin/bash
echo "enter the name:"
read username
echo "You entered $username"
Example: Write a script to create a new user
#!/bin/bash
read -p "Enter username: " username
echo "You entered $username"
sudo useradd -m $username
echo "New user added"
after running the script you can check if user is added or not by this command:
$ cat /etc/passwd
Arguments:
./variables.sh huzefa viewer
variables.sh is argument number 1 donated as $0 in script
huzefa is argument number 2 donated as $1 in script
viewer is argument number 3 donated as $2 in script
Let me show you how these arguments can be used in a shell script:
#!/bin/bash
echo "Print argument number 1: $0"
echo "Print argument number 2: $1"
echo "Print argument number 3: $2"
Conditional Statements (if-else):
Conditional statements are used to make decisions in your script.
#!/bin/bash
if [ -f "/etc/passwd" ]; then
echo "/etc/passwd exists"
else
echo "/etc/passwd does not exist"
fi
Loops (for and while):
For Loop: The for loop is used to iterate over a list of items
Following script will make 5 folder using for loop:
#!/bin/bash
for (( num=1; num<=5; num++ ))
do
mkdir "demo$num"
done
Another example;
#!/bin/bash
for (( num=$2; num<=$3; num++ ))
do
mkdir "$1$num"
done
Following command will run the above script and you can create multiple folders of a specified range
$ ./for_loop.sh day 1 90
While Loop:
The while loop runs as long as the condition is true:
#!/bin/bash
counter=1
while [ $counter -le 5 ]
do
echo "Counter: $counter"
((counter++))
done
Functions in Shell Scripts:
Functions allow you to break down your script into smaller, reusable pieces.
#!/bin/bash
greet() {
echo "Hello, $1!"
}
greet "DevOps Engineer"
Scheduling Scripts with Cron:
To automate script execution, you can use cron
jobs. For example, to run a script every day at 5 AM, edit the crontab:
crontab -e
Add the following line:
0 5 * * * /path/to/your/script.sh
Best Practices for Shell Scripting in DevOps:
Use meaningful names for variables and functions.
Use comments to explain complex sections of your script.
Error handling: Check for errors and handle them gracefully using exit statuses (
$?
).Log actions: Include logging in your scripts for easier debugging and auditing.
Test scripts thoroughly before deploying them in production environments.
Subscribe to my newsletter
Read articles from Huzefa Ahmed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Huzefa Ahmed
Huzefa Ahmed
👋 Hi, I’m Huzefa Ahmed, and I’m starting my DevOps engineering journey! 🚀 I’m passionate about AWS DevOps and aim to land a full-time role in this field. As I dive into cloud technologies, automation, and continuous integration, I’ll share my progress, insights, and challenges. Let’s connect, learn, and grow as a DevOps community! Follow my journey on Hashnode and LinkedIn as I work towards securing a job in DevOps! LinkedIn: https://www.linkedin.com/in/huzefa-ahmed-15720b278/