Create an Android Widget with Ionic Framework

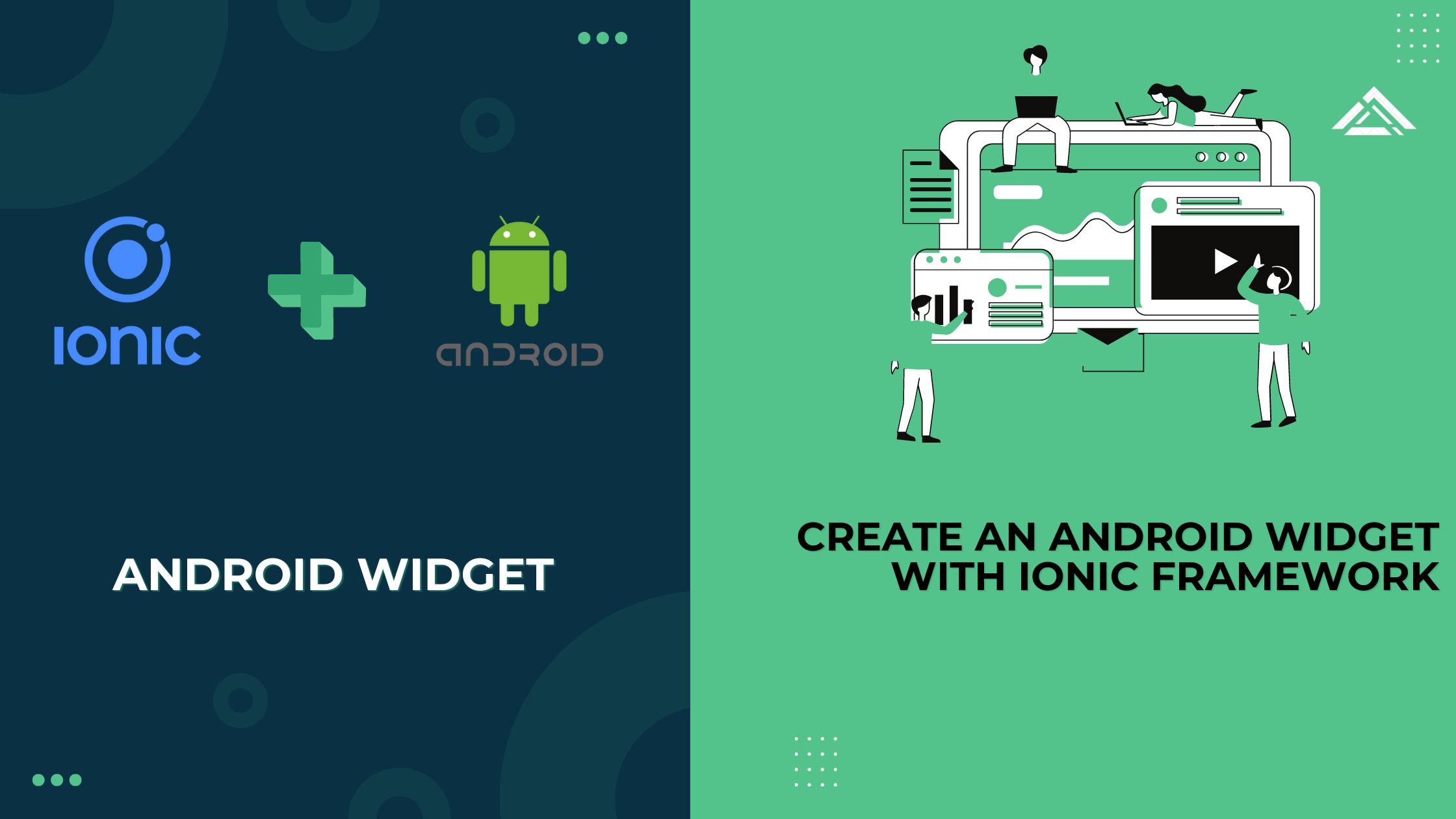
When you're building a mobile app using Ionic, you're essentially creating a web-based app that runs inside a WebView, a kind of browser window embedded in your app. But what if you need to do something that’s not possible with just web technologies, like updating an Android widget? This is where connecting your Ionic app to Android's native capabilities comes in.
Let’s break it down step by step:
1. The Basics: WebView and Native Code
WebView: This is the container that displays your Ionic app. It’s like a mini browser inside your Android app that shows your HTML, CSS, and JavaScript.
Native Code: This is the Android-specific code written in Java or Kotlin that interacts directly with the Android operating system.
Normally, your Ionic app can’t directly talk to Android’s native code because they’re running in different “worlds.” Ionic lives in the WebView, while native Android code runs directly on the device.
2. The Bridge: JavaScript Interface
To connect these two worlds, you create a bridge, a way for your web-based Ionic app to communicate with Android’s native code.
- JavaScript Interface: This is a special mechanism that Android provides, allowing you to expose native methods to the WebView. Basically, you tell Android, “Hey, here’s a function in my native code. I want to be able to call it from my Ionic app.”
In your app, you use a simple JavaScript function like syncCount() in your Ionic app to trigger something in the Android code.
3. Exposing Native Methods to Ionic
In your Android project’s MainActivity.java, you create this bridge. Here’s what happens:
You write a Java method that does something native like updating a widget.
You then expose this method to your WebView using webView.addJavascriptInterface(). This tells Android, “Make this method callable from the Ionic side.”
For example:
webView.addJavascriptInterface(new Object() {
@JavascriptInterface
public void syncCount(int count) {
syncWidgetCount(count);
}
}, "WidgetBridge");
This code means that your Ionic app can now call WidgetBridge.syncCount(count) in JavaScript, and it will trigger the syncCount(int count) method in the native code.
4. Making the Call from Ionic
On the Ionic side, when you want to sync the counter value with the widget, you just call this method:
syncWithWidget() {
if ((window as any).WidgetBridge) {
(window as any).WidgetBridge.syncCount(this.count);
} else {
console.error('WidgetBridge is not available.');
}
}
This JavaScript code checks if the WidgetBridge exists (meaning the bridge is set up correctly), and if it does, it calls the syncCount method, passing the current count value.
5. Updating the Widget
Once the native method is called, it handles the Android-specific logic, such as updating the widget with the new count value. This involves using Android’s AppWidgetManager to tell the widget, “Hey, update yourself with this new data.”
Why This is Powerful
By connecting your Ionic app with Android’s native code, you’re not limited to just what web technologies can do. You can tap into Android’s full potential, like updating a home screen widget.
This bridge is essential for making your Ionic app feel like a fully integrated part of the Android ecosystem, even though it’s built with web technologies. It’s a powerful way to extend the functionality of your app and provide a richer user experience.
Subscribe to my newsletter
Read articles from Uttam Roy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Uttam Roy
Uttam Roy
Hi, I'm Uttam From Bangladesh working as software engineer at Implevista.