Context API
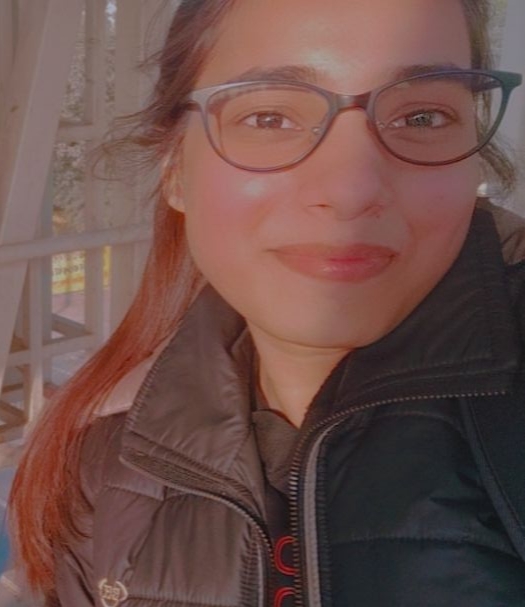
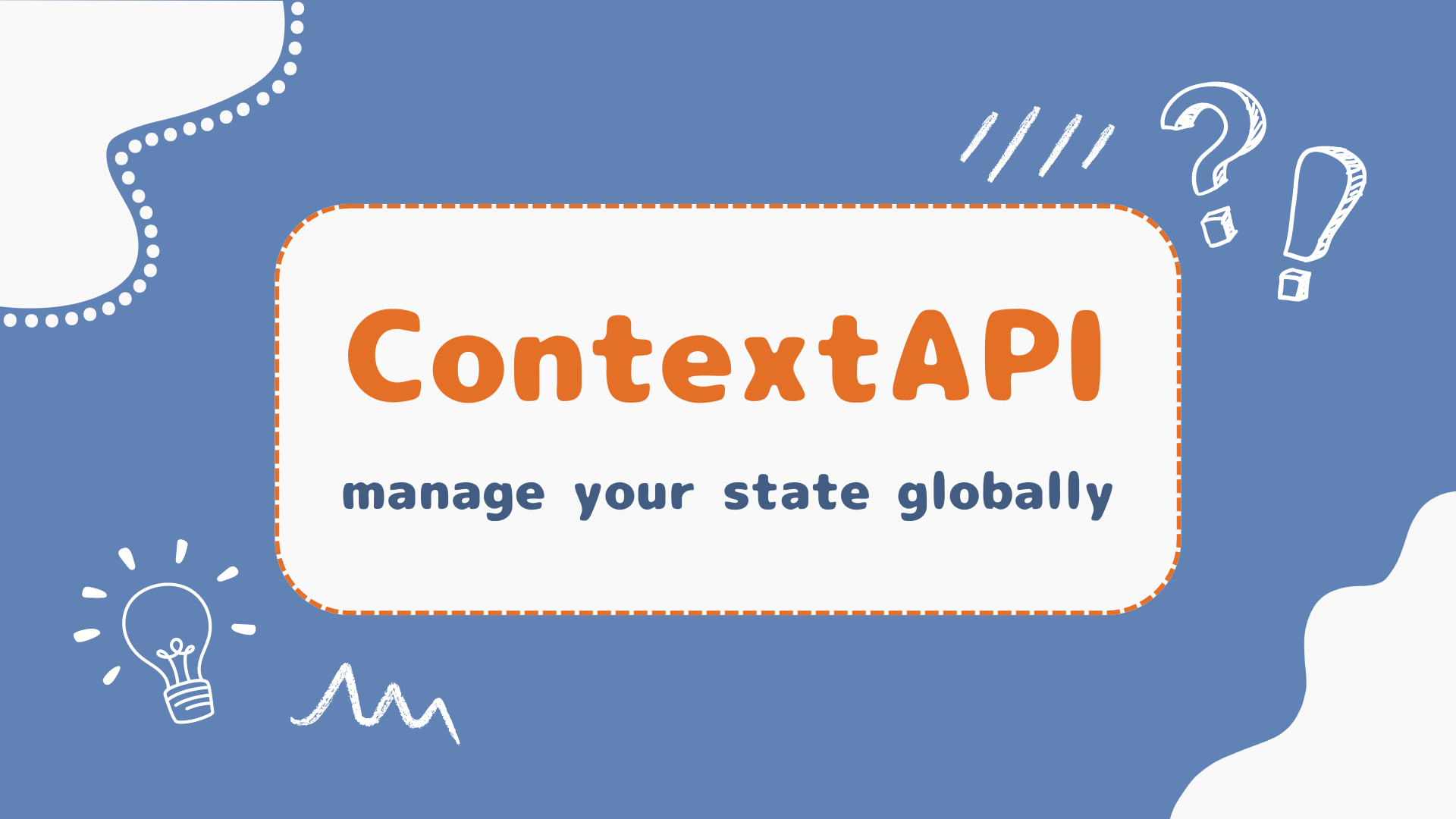
What is the Context API?
The Context API in React provides a streamlined way to share data across the component tree without the need to pass props through every level. This can be especially useful in larger applications where prop drilling becomes cumbersome.
When and Why to Use the Context API
Imagine you have a toolbar component that requires a "theme" prop. In a deeply nested component structure, passing this prop through each level can be tedious and error-prone. The Context API solves this problem by allowing you to pass a value directly to the component that needs it, bypassing the intermediate components.
Context is designed to share data that can be considered "global" for a tree of React components. In simple terms, it saves you from prop drilling and makes your code cleaner and easier to maintain.
React.createContext
const MyContext = React.createContext(defaultVal);
When React renders a component that subscribes to this context object, it will read the context value from the closest matching Provider
above it in the tree. The defaultVal
is only used when a component does not have a matching Provider
in the tree.
Note: Passing undefined
as a Provider
value does not cause consuming components to use the default value.
Context.Provider
<MyContext.Provider value={...}></MyContext.Provider>
The Provider
component accepts a value
prop, which is passed to all consuming components that are descendants of this Provider
. Whenever the value
prop changes, all subscribed consumers will re-render.
useContext
const useMyContext = useContext(MyContext);
The useContext
hook accepts a context object and returns the current context value. It's a straightforward way to access the context in your components.
Accepts: A context object (e.g.,
MyContext
)Returns: The current context value for that object
Note: The argument to useContext
must be the context object itself, not the Provider
or Consumer
.
useContext(MyContext)
✔️useContext(MyContext.Provider)
❌useContext(MyContext.Consumer)
❌
Thank you for reading! 🤗 For a more detailed example of using the Context API, check out my next blog where I walk through a practical implementation step by step.
Subscribe to my newsletter
Read articles from Namrata Jain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
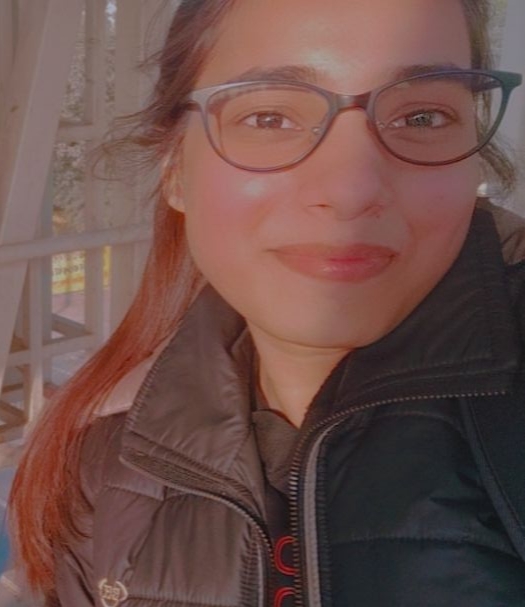
Namrata Jain
Namrata Jain
I am a BCA 22' graduate, a NeoGrad 22', and a software engineer by profession.