Context API
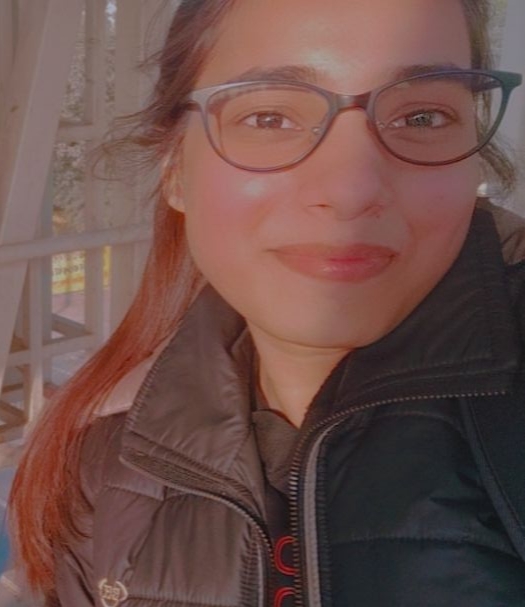
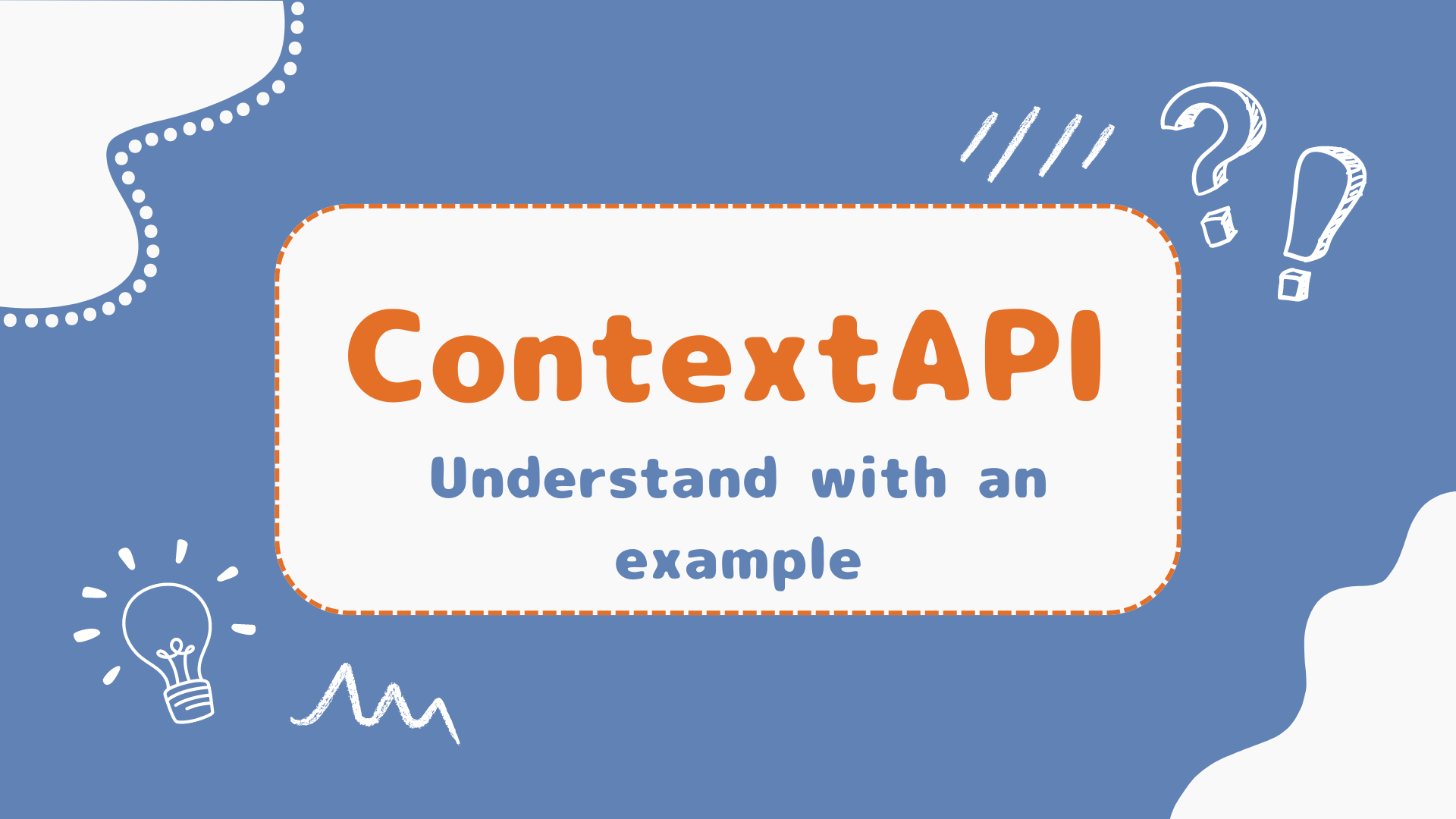
Hello, folks!
In this blog, we'll dive deep into the React Context API with a practical example. But before we explore what the Context API is, let’s first understand why it’s a crucial tool in the React ecosystem.
The Problem: Prop Drilling
React follows a unidirectional data flow where a parent component passes data to its child components via props. This method works seamlessly for small applications. However, as your application scales, you may encounter a scenario where you need to pass a prop through multiple layers of components, even if only a few of them actually need it. This pattern is known as prop drilling.
Prop drilling can make your code more complex, harder to manage, and difficult to debug. It becomes challenging to track where the data is being initialized and where it is ultimately used, leading to potential maintenance issues down the road.
The Solution: Context API
To solve this problem, React introduced the Context API, a powerful feature that allows you to share state across your application without passing props down manually at every level. The Context API is particularly useful when you need to manage global state or pass data that needs to be accessed by multiple components at different nesting levels.
Understanding the Context API
The Context API is built around the createContext
function, which returns a context object. This object is composed of two key elements:
Provider: The
Provider
component supplies the context value to its descendants.Consumer: The
Consumer
component or theuseContext
hook is used to access the context value within its children.
Here’s a simple example to illustrate how the Context API works.
import { createContext } from 'react';
// Create a context with a default value
const CartContext = createContext({ items: 0 });
Implementing the Provider
Imagine you’re building an e-commerce application where multiple components need access to the cart state, such as the number of items in the cart. Managing this state globally can be cumbersome with prop drilling, but it’s straightforward with the Context API.
First, create a CartProvider
component that will provide the cart state to its children.
import { createContext, useState } from 'react';
const CartContext = createContext();
const CartProvider = ({ children }) => {
const [cart, setCart] = useState({ items: 0 });
return (
<CartContext.Provider value={{ cart, setCart }}>
{children}
</CartContext.Provider>
);
};
export { CartProvider, CartContext };
Consuming the Context with useContext
To access the cart state in your components, you can use the useContext
hook, which provides a much cleaner syntax compared to the older Consumer
component.
import { useContext } from 'react';
import { CartContext } from './CartProvider';
const CartComponent = () => {
const { cart, setCart } = useContext(CartContext);
return (
<div>
<h2>Cart Items: {cart.items}</h2>
{/* Logic to update cart items */}
</div>
);
};
Wrapping Your App with the Provider
Finally, you need to wrap your entire application (or the part that needs access to the cart state) with the CartProvider
to ensure that all descendant components can access the provided context.
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
import { CartProvider } from './CartProvider';
ReactDOM.render(
<CartProvider>
<App />
</CartProvider>,
document.getElementById('root')
);
Conclusion
By using the Context API, you can avoid prop drilling and make your state management more efficient and scalable. The Context API is a powerful tool in the React ecosystem, particularly for managing global states like user authentication, theme settings, or, as we’ve seen, shopping carts in an e-commerce app.
Thanks for reading! 🤗
Subscribe to my newsletter
Read articles from Namrata Jain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
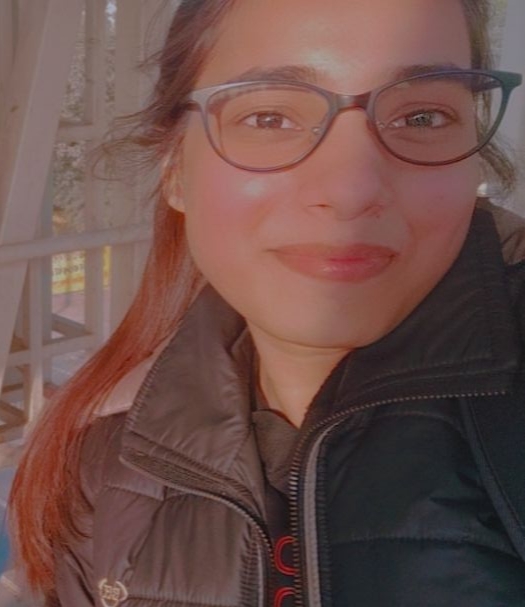
Namrata Jain
Namrata Jain
I am a BCA 22' graduate, a NeoGrad 22', and a software engineer by profession.