Unlock the Web’s Hidden Gems: A Friendly Guide to Web Scraping with Python
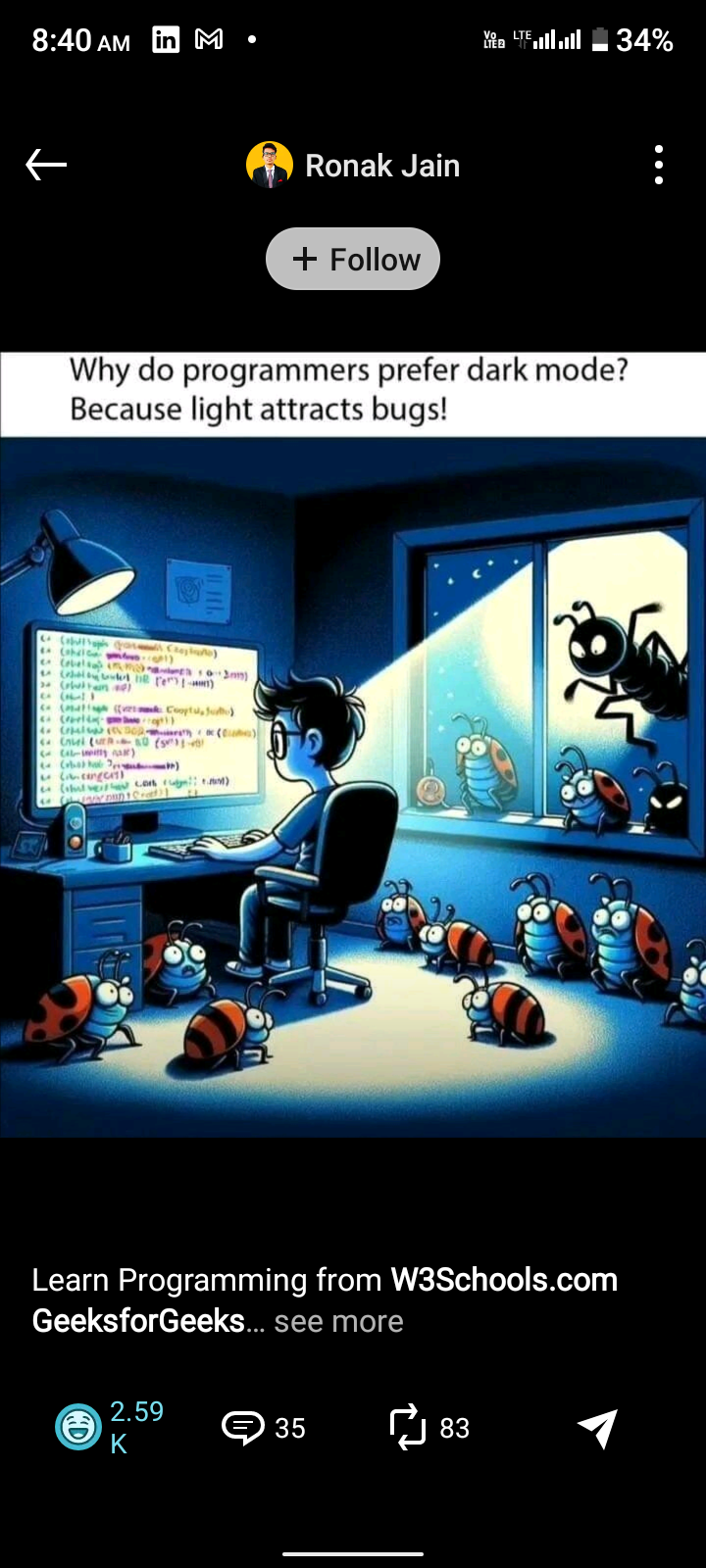
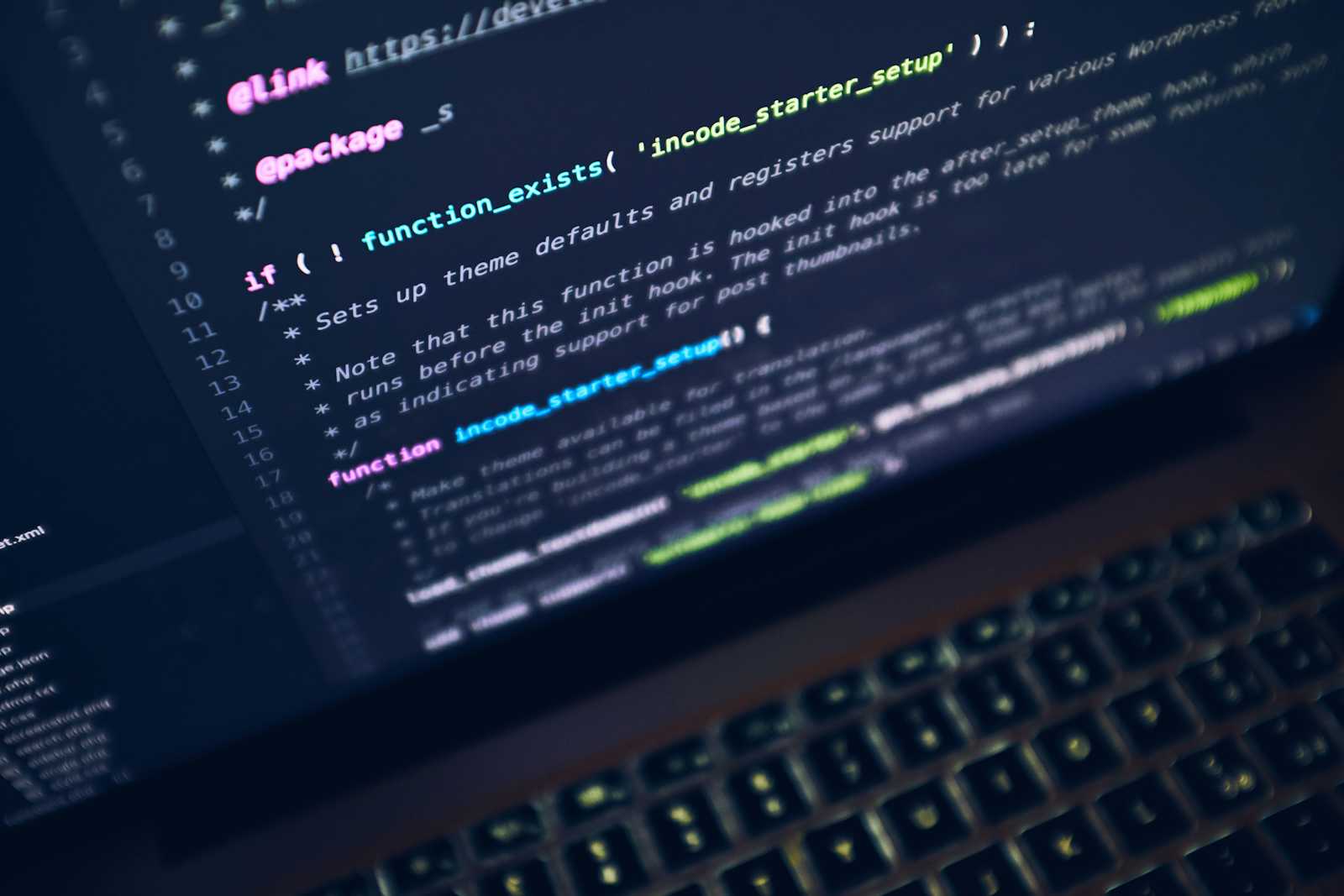
Hey there, fellow explorer of the digital realm! 🌍✨
Ever feel like the internet is one big treasure chest, overflowing with valuable data just waiting to be discovered? Well, today, I’m excited to introduce you to a tool that will turn you into a digital treasure hunter — web scraping with Python! 🐍
Whether you’re a data enthusiast, a curious developer, or someone who’s always wondered how to gather all that amazing information online, this post is for you! Let’s dive into the basics of web scraping and get you started with a simple, yet powerful, Python project.
So, What’s Web Scraping Anyway?
Imagine you had a personal assistant who could visit thousands of websites, grab the exact information you want, and deliver it in a neat little package. Sounds cool. That’s web scraping in a nutshell!
Web scraping is all about automating the process of extracting data from websites, turning the web into your own data playground. From collecting market data and tracking prices to gathering news articles and building datasets for machine learning — web scraping can do it all!
Getting Ready to Scrape: The Tools You’ll Need
To get started with web scraping, you’ll need a few handy tools in Python:
Python: The Swiss Army knife of programming languages, perfect for scraping thanks to its simplicity and vast libraries.
BeautifulSoup: A Python library that helps you easily parse and navigate HTML (the language of the web).
Requests: A library for sending HTTP requests, which we use to fetch the content of web pages.
Install these libraries with a quick run of this command in your terminal:
pip install beautifulsoup4 requests
Your First Scraping Adventure: Step-by-Step
Ready for a little adventure? Let’s scrape a list of top movies from a webpage! Follow these steps to build your very first web scraper:
Step 1: Send a Friendly Hello to the Web Page
First things first, we need to grab the content of the web page we want to scrape. Here, we’ll use the requests
library to fetch the page:
import requests
# The URL of the website you want to scrape
url = "https://www.empireonline.com/movies/features/best-movies-2/"
response = requests.get(url)
webpage_content = response.text
Step 2: Let BeautifulSoup Do the Heavy Lifting
Now that we have the webpage content, let’s hand it over to BeautifulSoup to make sense of it:
from bs4 import BeautifulSoup
# Create a BeautifulSoup object and specify the parser
soup = BeautifulSoup(webpage_content, "html.parser")
Step 3: Hunt for the Data You Want
Time to dig for the gold! We’ll search the HTML content to find the elements containing the movie titles:
# Find all movie title elements
movie_titles = soup.find_all(name="h3", class_="listicleItem_listicle-item__title__BfenH")
# Extract text from each element and store it in a list
movies = [movie.get_text() for movie in movie_titles]
Step 4: Save Your Treasure!
You’ve got the data — now let’s save it for later:
# Reverse the list to keep the order intact (if needed)
movies = movies[::-1]
# Save the movie titles to a text file
with open("movies.txt", mode="w") as file:
for movie in movies:
file.write(f"{movie}\n")
And there you have it! 🎉 You’ve built your first web scraper, and now you have a text file filled with a list of awesome movies, automatically extracted from a website!
Pro Tips for Becoming a Web Scraping Wizard 🧙♂️
Respect the Rules: Always check the website’s
robots.txt
file to understand what you can and cannot scrape.Be Kind to Servers: Avoid sending too many requests at once — add delays with
time.sleep()
to keep things smooth and polite.Prepare for the Unexpected: Use error handling (try-except blocks) to deal with any hiccups along the way.
Why Learn Web Scraping?
Learning to scrape the web opens up a world of possibilities. Want to analyze social media trends? Done. Need to track your favourite products’ prices? Easy. Curious about data science or machine learning? Web scraping is your gateway to building fantastic datasets!
It’s a powerful skill that empowers you to automate repetitive tasks, gather insights, and ultimately, make better data-driven decisions.
Join the Web Scraping Club!
There you go — a friendly introduction to web scraping with Python! Now it’s your turn to try it out. Experiment, explore, and see what amazing data you can uncover. And don’t forget to share your newfound skills with others — there’s a whole community out here cheering you on! 🙌
Feel free to Connect me Here and on Linkedin too. Here is the link
Happy scraping! 🕵️♀️✨
Subscribe to my newsletter
Read articles from Huzaifa Saran directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
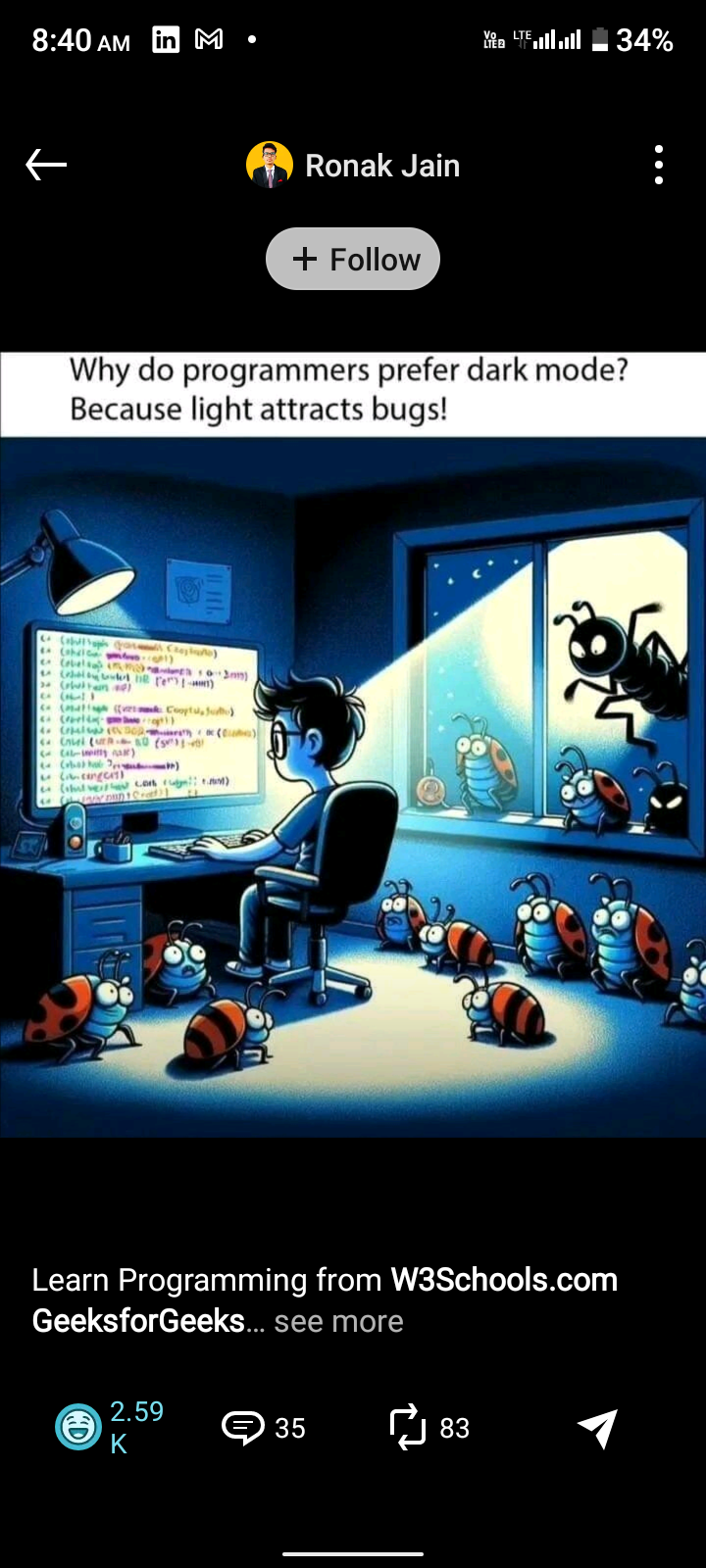
Huzaifa Saran
Huzaifa Saran
Django full stack developer and Emerging software engineer