Why Your Code is Like a Bad Relationship with lots of Red Flags

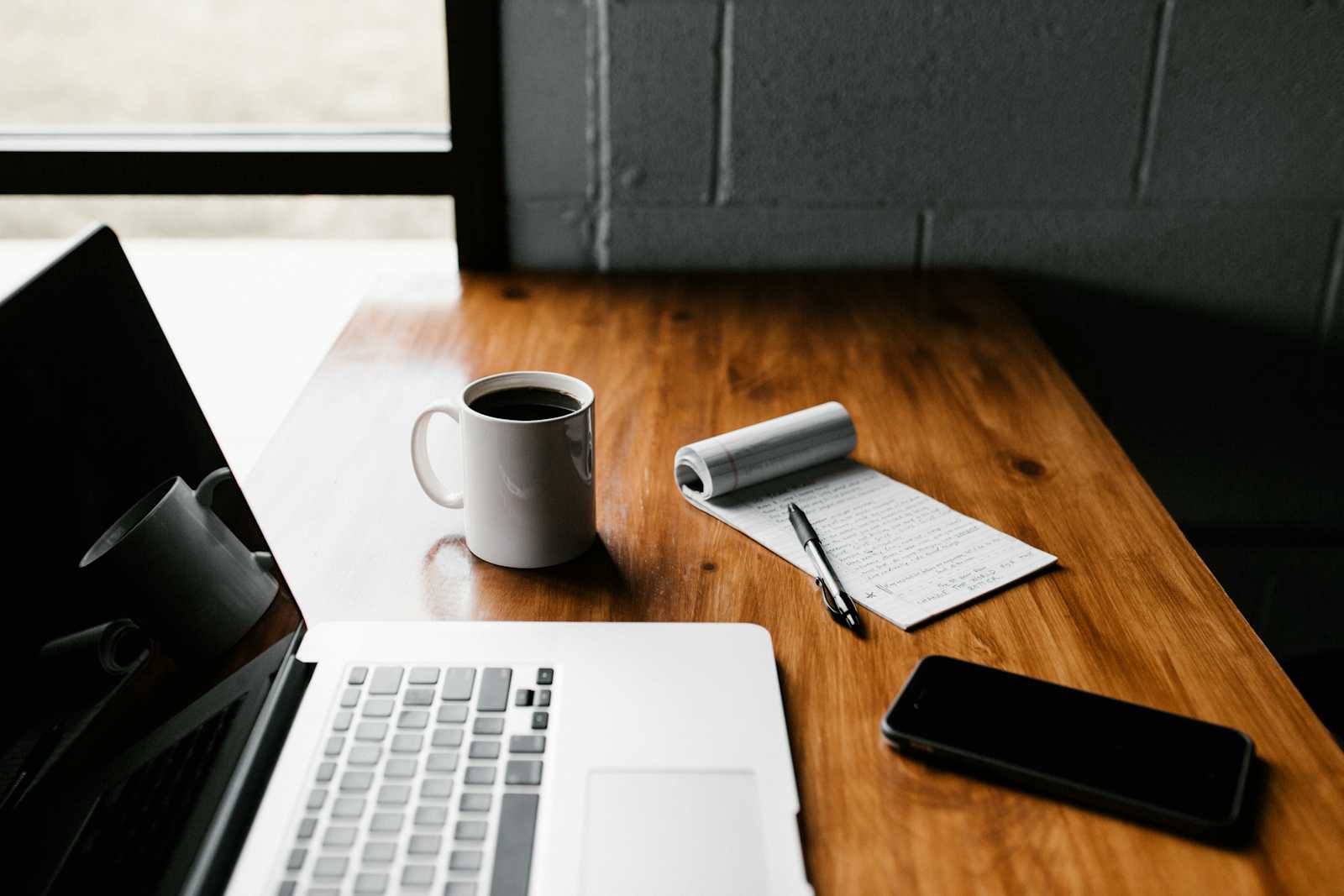
Ever felt like your code is giving you the silent treatment? Or maybe it's being overly clingy, demanding all your attention? ๐ Welcome to the world of toxic code relationships! Today, we're going to explore why your code might be your worst frenemy and how to spot those red flags before you're in too deep.
Disappearing Variables
You know that feeling when someone you're dating suddenly vanishes without a trace? Well, variables can play that game too!
Red Flag #1: Undefined Variables
let mood = "happy";
console.log(mod); // Oops! We meant 'mood', but typed 'mod'
This is like texting the wrong person at 2 AM. Awkward! ๐ฌ
How to Fix It:
Use strict mode in JavaScript (
'use strict';
at the top of your file)Enable "undefined variable" warnings in your code editor
Review your code for typos before running it
"Debugging is like being the detective in a crime movie where you're also the murderer." - Filipe Fortes
Tightly Coupled Code
Does your code refuse to let you hang out with other functions? Time for an intervention!
Red Flag #2: High Coupling
class DoEverything:
def __init__(self):
self.data = []
def add_data(self, item):
self.data.append(item)
def process_data(self):
# Processes data in a very specific way
pass
def display_results(self):
# Displays results in a very specific format
pass
# This class is doing too much! It's the clingy partner of the code world.
This class is like that partner who insists on doing everything together. "No, you can't go to the grocery store alone. I'm coming too!"
How to Fix It:
Break it up! Separate concerns into different classes or functions
Use dependency injection to make your code more flexible
Remember, good code is like a good partner - supportive but not suffocating
Inconsistent Behavior
Ever had code that works fine one minute and throws a fit the next? Classic gaslighting!
Red Flag #3: Race Conditions
let sharedResource = 0;
function incrementResource() {
// Read
let temp = sharedResource;
// Modify
temp = temp + 1;
// Write back
sharedResource = temp;
}
// What if two functions call this at the same time? Chaos ensues!
This is like when your partner says, "I never said that!" but you're pretty sure they did. Maddening!
How to Fix It:
Use locks or semaphores for shared resources
Implement atomic operations where possible
Design your code to be thread-safe from the ground up
Lack of Documentation
Does your code refuse to tell you what it's thinking? Sounds like it has commitment issues!
Red Flag #4: Poor or No Documentation
def mystery_function(x, y, z):
return (x + y) * z if x > y else (x - y) * z
# What does this do? Your guess is as good as mine!
This function is like a partner who says, "Let's just see where this goes" for three years straight. ๐ฉ
How to Fix It:
Write clear, concise comments explaining the 'why', not just the 'what'
Use docstrings to document functions and classes
Keep documentation up-to-date (yes, even when you're "just making a quick change")
"Code is like humor. When you have to explain it, it's bad." - Cory House
Overly Complex Code
Is your code always creating drama? Does it turn a simple task into a three-act opera?
Red Flag #5: Unnecessary Complexity
function isEven(num) {
return !(Boolean(!!(num & 1)));
}
// Meanwhile, in a parallel universe where simplicity exists:
// function isEven(num) {
// return num % 2 === 0;
// }
This is like a partner who turns "What should we have for dinner?" into a philosophical debate about the meaning of life.
How to Fix It:
KISS (Keep It Simple, Silly!)
Refactor regularly
Ask yourself, "Would a five-year-old understand what this function does?" (Okay, maybe a very smart five-year-old)
Inefficient Algorithms
Does your code always take the long way home? It might be suffering from chronic laziness!
Red Flag #6: Poor Performance
def find_duplicate(arr):
for i in range(len(arr)):
for j in range(i+1, len(arr)):
if arr[i] == arr[j]:
return arr[i]
return None
# This function is taking a nap while finding duplicates. Wake up, code!
This algorithm is like a partner who takes two hours to do a ten-minute task. Charming at first, infuriating later.
How to Fix It:
Learn and use appropriate data structures (hash tables are your friends!)
Analyze your algorithm's time and space complexity
Profile your code to find bottlenecks
Memory Leaks
Does your code collect useless junk and never let go? You might be dating a digital hoarder!
Red Flag #7: Memory Leaks
function createButtons() {
let buttons = [];
for (let i = 0; i < 10; i++) {
let button = document.createElement('button');
button.innerHTML = 'Button ' + i;
document.body.appendChild(button);
buttons.push(button);
}
// We're keeping references to all buttons, even if we don't need them anymore
}
This is like a partner who keeps every movie ticket stub, restaurant receipt, and random pebble they've ever encountered. Cute at first, but where are you supposed to put your stuff?
How to Fix It:
Be mindful of object references, especially in languages without garbage collection
Use tools like Chrome DevTools Memory Profiler for JavaScript
Implement proper cleanup in your destroy or dispose methods
Refactoring
Sometimes, the only way forward is a clean break. In the coding world, we call this refactoring.
Steps to a Healthy Code Breakup:
Identify the problems (you're already doing this by reading this article!)
Make a plan for improvement
Take it slow - refactor in small, manageable chunks
Write tests before refactoring to ensure you're not breaking anything
Communicate with your team about the changes you're making
Remember, breaking up with bad code is hard, but staying in a toxic relationship with it is harder!
Wrapping Up
Just like in real relationships, maintaining healthy code takes work. But with attention, care, and maybe a little couples therapy (pair programming, anyone?), you can turn your toxic code relationship into a beautiful partnership.
"Always code as if the guy who ends up maintaining your code will be a violent psychopath who knows where you live." - John Woods
So, the next time your code starts showing these red flags, don't ignore them! Address the issues, have that difficult conversation, and remember: there are plenty of fish in the C. ๐
Now go forth and build healthy, loving relationships... with your code! ๐๐ป
Subscribe to my newsletter
Read articles from Shubham directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
