Next.js Styling: A Guide to Using Modules
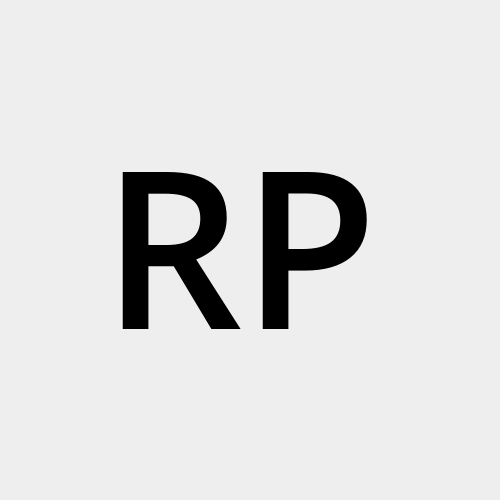
2 min read
Table of contents
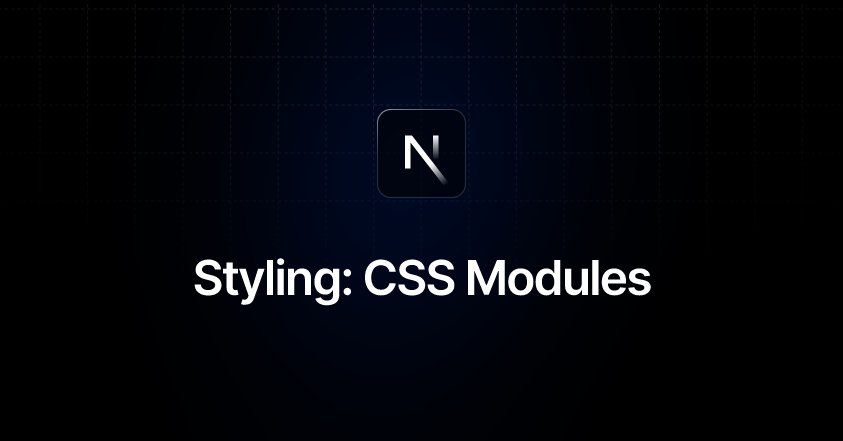
using modules in next js for styling
my_next_project/
├── public/
│ ├── index.html
│ └── favicon.ico
├── src/
│ ├── components/
│ │ └── header.tsx
header-styles.module.css
│ ├── styles/
│ │ └── App.css
├── package.json
├── package-lock.json
├── README.md
└── .gitignore
CSS Modules are only enabled for files with the .module.css
and .module.sass
extensions
Next.js has built-in support for CSS Modules using the .module.css
extension.
CSS Modules locally scope CSS by automatically creating a unique class name. This allows you to use the same class name in different files without worrying about collisions. This behavior makes CSS Modules the ideal way to include component-level CSS.
Make sure that you follow the proper folder structure and create a file named header-styles.module.css.
header-styles.module.css
//header-styles.module.css
.header_logo_nav_div {
display: flex;
flex: 1;
align-items: center;
justify-content: space-between;
}
.header_logo_div {
height: 42px;
width: 125px;
cursor: pointer;
width: fit-content;
}
.header_nav_div {
display: flex;
align-items: center;
justify-content: space-evenly;
gap: 10px;
}
.header_nav_button {
padding: 0.75rem 1rem;
border-radius: 5px;
text-align: center;
cursor: pointer;
}
create a file header.tsx
//header.tsx
import React from "react";
import { HeaderContainer, HeaderTitle, MainHeaderDiv } from "./header.styles";
import Image from "next/image";
import styles from "./header-styles.module.css";
const Header = () => {
return (
<MainHeaderDiv>
<HeaderContainer>
<div className={styles.header_logo_nav_div}>
<div className={styles.header_logo_div}>
<Image
src="https://workablehr.s3.amazonaws.com/uploads/account/logo/299550/logo"
alt="Site Logo"
width={128}
height={42}
/>
</div>
<div className={styles.header_nav_div}>
<div className={styles.header_nav_button}>
<HeaderTitle>Convert</HeaderTitle>
</div>
<div className={styles.header_nav_button}>
<HeaderTitle>Compress</HeaderTitle>
</div>
<div className={styles.header_nav_button}>
<HeaderTitle>All PDF Tools</HeaderTitle>
</div>
<div className={styles.header_nav_button}>
<HeaderTitle>Split</HeaderTitle>
</div>
<div className={styles.header_nav_button}>
<HeaderTitle>Edit</HeaderTitle>
</div>
<div className={styles.header_nav_button}>
<HeaderTitle>Merge</HeaderTitle>
</div>
<div className={styles.header_nav_button}>
<HeaderTitle>Sign</HeaderTitle>
</div>
</div>
</div>
</HeaderContainer>
</MainHeaderDiv>
);
};
export default Header;
For a more structured approach, you can use styled components as well.
0
Subscribe to my newsletter
Read articles from Rushikesh Patil directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
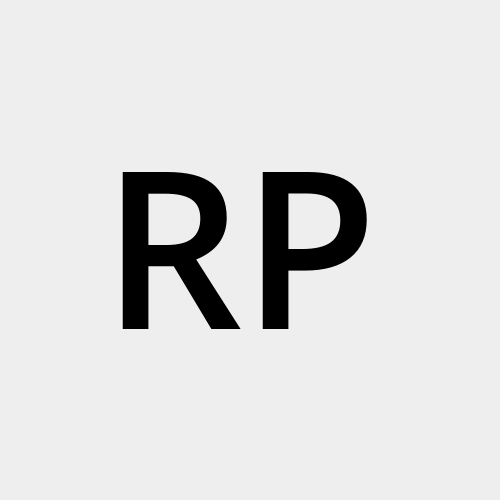