module. export & require
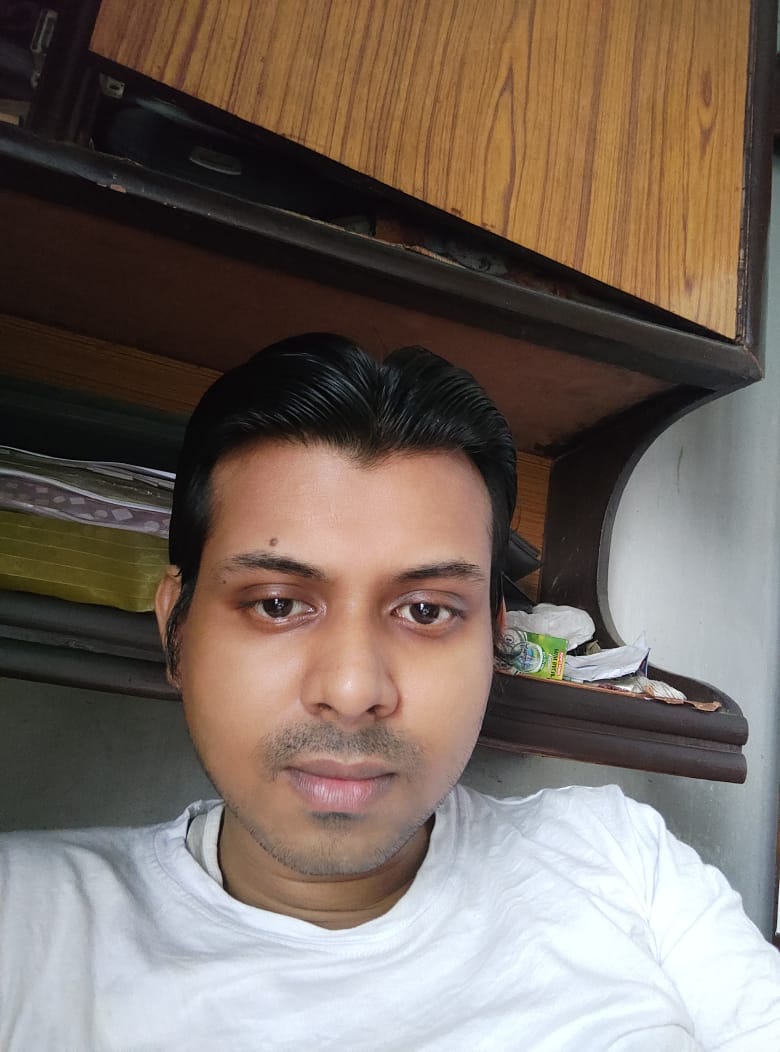
Table of contents

Modules in Node-JS :
Modules
are the collection of java script code which is private to itself and it exists independently.Node.js
modules are powerful tools for organizing, reusing, and sharing code.It is the
central
toNode-JS
development.Modules
can be easily reused across different parts of the application.
Entry Point :
- Whenever we have a
Node-JS
application there is one entry point in our application that entry point isapp.js
.
One Module Into Another :
If there are code in other files along with
app.js
i.e there is another file namednew.js
and we want to execute code of that file along withapp.js
. Herenew.js
will be a separate module.To make two modules work together we need a
require
function.In app.js
require("./new.js");
let a = "Kapil in app.js"
console.log(a)
- In new.js
console.log("Hello Kapil welcome to new file")
- Output :
Hello Kapil welcome to new file
Kapil in app.js
Calculating a Function From One File To Another File :
- There is a file named
sum.js
.
function calculateSum(a, b) {
const sum = a + b;
console.log(sum);
}
This code can be executed into our
app.js
.Whenever we create a separate module after that we write require in
app.js
then the code will run in the separate module but we cannot access the variables, methods and functions of one module into another simply by writingrequire
.Modules protects their variables and functions from leaking, by default the modules are protected. So we cannot access the
calculateSum()
function simply by writingrequire
.For that we have to
export
the functioncalculateSum()
after that we have to import it into ourapp.js
.Code in
sum.js
:
module.exports = {calculateSum};
- Code on
app.js
:
const {calculateSum} = require("./sum.js");
calculateSum(10, 20);
Output:
30
Exporting Multiple Functions/Variables :
We can do this by wrapping it inside an object.
Code in
sum.js
let x = "Hello Sum";
function calculateSum(a, b) {
const sum = a + b;
console.log(sum);
}
module.exports = { x,calculateSum };
- Code in
app.js
const {x, calculateSum} = require("./sum.js");
calculateSum(10, 20);
console.log(x);
Output :
30
Hello Sum
- So now we can export multiple things by wrapping it inside an object.
Common JS Module :
The above pattern of require and export is known as Common-JS Module.
Common-JS is a module system used in Node.js, allowing you to structure and share code. In Common-JS, each file is treated as a module.
Overview :
require
: Used to import modules.module.exports
: Used to export functions, objects, or variables from a module.There is another way of exporting module known as ES Module.
ES Module :
ES Modules (ECMAScript Modules)
are a standardized module system in JavaScript, commonly used in both front-end and modern back-end development (including Node.js). They use import and export statements for defining and using modules.To use ES module we have to write in our
package.json
file :
{
"type": "module"
}
Example :
In sum.js
export let x = "Hello Sum";
export function calculateSum(a, b) {
const sum = a + b;
console.log(sum);
}
- In app.js
import {calculateSum,x} from "./sum.js"
calculateSum(100,200);
console.log(x)
- Output :
300
Hello Sum
Common-JS Module vs ES Module :
Common-JS
andES Modules
are two different module systems used in Java Script.Common-JS :
Uses
require
to import modules.Uses
module.exports
to export modules.By default used in Node-JS.
The Code is run in a
non-strict
mode.Only supports
default exports
.Modules are loaded synchronously.
//Example: // Exporting const add = (a, b) => a + b; module.exports = add; // Importing const add = require('./add'); console.log(add(2, 3)); // Outputs: 5
ES Modules:
Uses
import
to bring in modules.Uses
export
to expose modules.By default used in React, Angular.
The Code is run in a
strict
mode.Supports named
exports
anddefault exports
.Modules
are loaded asynchronously.//Example : // Exporting export const add = (a, b) => a + b; // Importing import { add } from './add.js'; console.log(add(2, 3)); // Outputs: 5
module.exports :
module.exports
is crucial for defining what amodule
exposes to the outside world when it's imported using require. It allows you toexport
single or multiple values from amodule
.
console.log(module.exports)
Output :
{} //Empty Object
Nested Module :
If there are multiple files in a single folder then using a folder as a module.
In other words nested modules in
Node JS
allow for a well-organized, scalable, and maintainable project structure, especially in large applications.Example :
There is a folder named
calculate
. Inside that folder there are file namedindex.js
andmultiply.js
.Common practice is to use
index.js
in a folder to aggregate and export functionalities. This allows importing the whole folder as a module.In
multiply.js :
function calculateMultiply(a,b){ const result = a * b; console.log(result) } module.exports = {calculateMultiply}
function calculateMultiply(a,b){
const result = a * b;
console.log(result)
}
module.exports = {calculateMultiply}
- In
index.js :
const {calculateMultiply} = require("./multiply.js")
module.exports = {calculateMultiply}
Just like multiply.js we can include multiple file and connect them to
index.js
In
app.js
:
const {calculateMultiply} = require("./calculate")
calculateMultiply(20,30)
Output :
600
Importing data from json file :
In data.json
file.
const data = require("./data.json");
console.log(data)
Output :
{ name: 'Kapil Sarkar', city: 'Asansol', country: 'India' }
Core Modules in Node-JS
:
Node-JS
comes with a set of built-in"core modules"
that provide essential functionality for building applications without needing to install external packages.Example :
util
Provides utility functions, including debugging, formatting, and inheritance functions.
const util = require("node:util")
Subscribe to my newsletter
Read articles from Kapil Sarkar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
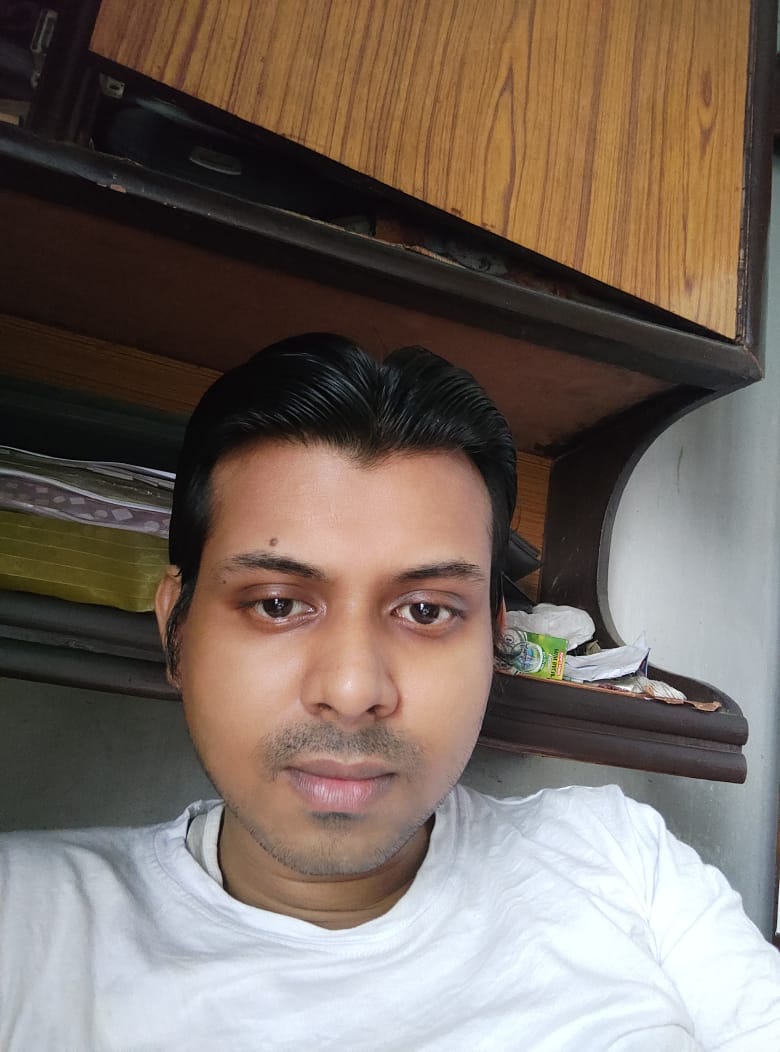
Kapil Sarkar
Kapil Sarkar
I AM A FULL STACK JAVASCRIPT DEVELOPER AND WEB BLOGGER