Creating First Spring Application
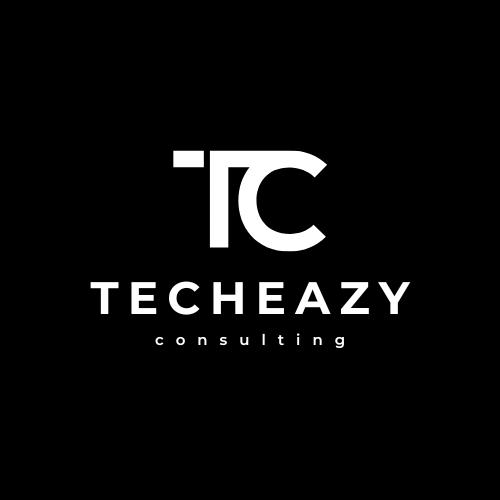
This blog will help you to create your first Spring Application:
Prerequisites:
Java Development Kit (JDK) installed
An IDE like IntelliJ IDEA, Eclipse, or Spring Tool Suite (STS)
Basic understanding of Java
Step 1: Create a New Spring Boot Project
Go to www.spring.io to create a new Spring Boot project.
Configure your project:
Project: Maven
Language: Java
Version: 3.3.2
Name: employee-management-system
Group: com.example
Artifact: employee-management-system
Java Version: 17 (or your installed version)
- Add Dependencies:
Add the following dependency:
Spring Web (for creating RESTful services)
Click Generate to create the project.
Extract the project folder and open it in your favorite IDE.
The project structure should look like this.
Explanation of Project Structure
1. Root Directory (employee-management-system)
- This is the base directory of your project, containing all the essential files and directories.
2. src/main/java
- This directory contains the main application code.
2.1 Base Package (com.example.employee_management_system)
The base package typically follows the naming convention
com.example.employee_management_system
or something similar.EmployeeManagementSystemApplication.java: This is the main class of the Spring Boot application, containing the @SpringBootApplication annotation. This class serves as the entry point to start your application.
2.2 Sub-packages
You should organize your code into different sub-packages to follow best practices and maintain code structure:
controller: Contains all the controllers or REST APIs for handling HTTP requests.
Example:
EmployeeController.java
service: Contains service classes that contain business logic.
Example:
EmployeeService.java
repository: Contains repository interfaces for database interaction, usually extending JpaRepository or CrudRepository.
Example:
EmployeeRepository.java
model or entity: Contains your JPA entity classes, representing the data model of your application.
Example:
Employee.java
config: Contains configuration classes for setting up Spring Beans, Security, etc.
Example:
SecurityConfig.java
3. src/main/resources
This directory contains resources for your application, such as configuration files, static resources, templates, etc.
application.properties or application.yml: This file is used for application configuration, such as database settings, server port, etc.
static/: Contains static resources like CSS, JavaScript, and images.
templates/: Contains templates for web views, typically in Thymeleaf, FreeMarker, etc.
application.properties or application.yml: Configuration files where you can specify environment-specific properties.
4. src/test/java
This directory contains test cases for your application.
You can create test classes with the same package structure as the main code.
5. target/
This directory is generated after building the project and contains the compiled bytecode and other build-related files. This folder will only appear after you build the project.
6. .gitignore
This file specifies which files and directories should be ignored by Git. For example, target/ or .idea/ (for IntelliJ IDEA users).
7. help.md
This file is a basic help document generated automatically by the Spring Initializr. It provides some useful information and quick tips about your project and how to get started with it.
8. mvnw, mvnw.cmd (for Maven) or gradlew, gradlew.bat (for Gradle)
These wrapper scripts allow you to build your project even if you don’t have Maven or Gradle installed on your system. They download the required version of the build tool automatically.
9. pom.xml or build.gradle
pom.xml (for Maven) or build.gradle (for Gradle): These files are used to manage project dependencies, plugins, and build configuration.
Step 2: Create entity Package and Create the Employee Entity
Create the entity Package: Create the entity Package in base package
com.example.employee_management_system
Create the Employee class: Create the Employee class in the entity package.
Define the Employee Entity:
Add attributes like
id
,name
,city
,department
, etc.Define constructors (no-args and all-args), getters and setters, and the toString method.
Step 3: Create controller Package and Create the EmployeeController
Create the controller Package: Create the controller Package in base package
com.example.employee_management_system
Create the EmployeeController class: Create the
EmployeeController
class in the controller package.Define the EmployeeController:
Annotate the class with
@RestController
.Create a simple method to return an Employee object.
Annotate the method with
@GetMapping("/employee")
.
Step 4: Run Your Application
- Run the application:
Go to
EmployeeManagementSystemApplication.java
.Right-click on the file and select Run ''.
- Test the application:
Open your browser or Postman and navigate to http://localhost:8080/employee.
You should see a JSON response with the employee details.
Congratulations! You have successfully created your first Spring Application. Next, learn how to link your Spring Application to GitHub.
Subscribe to my newsletter
Read articles from TechEazy Consulting directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
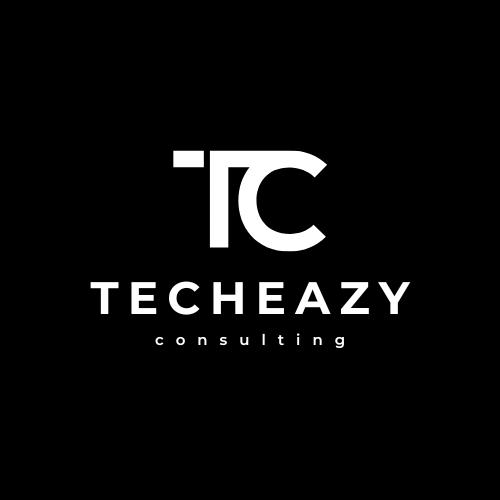