JavaScript Tip: || vs ?? for Default Values 🚀

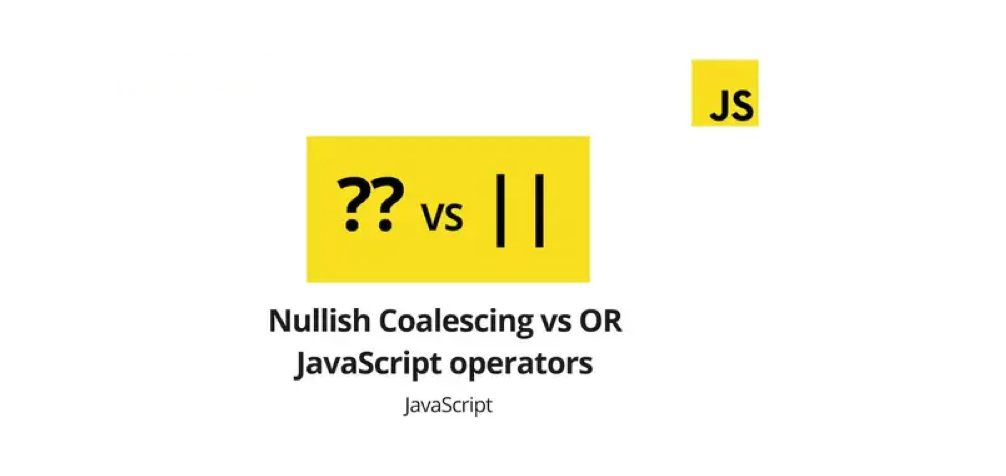
When working with default values in JavaScript, choosing between the logical OR (||
) and nullish coalescing (??
) operators can significantly impact how your data is handled. Here’s a quick guide to help you choose the right one:
🔄 Logical OR (||
)
Behavior: Returns the right-hand operand if the left-hand operand is any falsy value. In JavaScript, falsy values include:
0
(zero)''
(empty string)false
(boolean false)null
(null value)undefined
(undefined value)NaN
(Not-a-Number)
Example:
javascriptCopy codeconst value = 0 || 'default'; // value will be 'default' const anotherValue = null || 'default'; // anotherValue will be 'default'
Use Case: Use
||
when you want to replace any falsy value with a default.
🆗 Nullish Coalescing (??
)
Behavior: Returns the right-hand operand only if the left-hand operand is
null
orundefined
. Other falsy values such as0
,''
,false
,NaN
are preserved.Example:
javascriptCopy codeconst value = 0 ?? 'default'; // value will be 0 const anotherValue = null ?? 'default'; // anotherValue will be 'default'
Use Case: Use
??
when you specifically want to handlenull
orundefined
, while keeping other valid falsy values.
📌 Real-World Example
In cases where you need to preserve specific falsy values like 0
, using ||
might incorrectly replace it with null
. Conversely, ??
will preserve such values, ensuring accurate data handling.
Example:
javascriptCopy codeconst answerData = { judgement: 0 };
// Using || - replaces 0 with null
const judgementWithOr = answerData.judgement || null; // result: null
// Using ?? - preserves 0
const judgementWithNullish = answerData.judgement ?? null; // result: 0
Summary:
Use
||
to handle all falsy values and provide a default.Use
??
to specifically handlenull
orundefined
, preserving other falsy values.
Choosing the right operator ensures your data is handled as intended and avoids unintended transformations. Happy coding! 💻✨
#JavaScript #CodingTips #Programming #WebDevelopment #DeveloperTips
Subscribe to my newsletter
Read articles from Mohamed Zhioua directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mohamed Zhioua
Mohamed Zhioua
Hеllo, I'm Mohamеd Zhioua, a dеdicatеd Full-Stack JavaScript Dеvеlopеr basеd in Tunis, Tunisia 📍. I'm on a mission to shapе thе futurе through codе.