Unlocking the Power of AI: Integrating Spring AI with Google Vertex AI
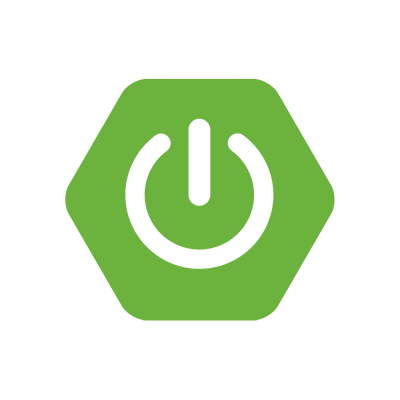
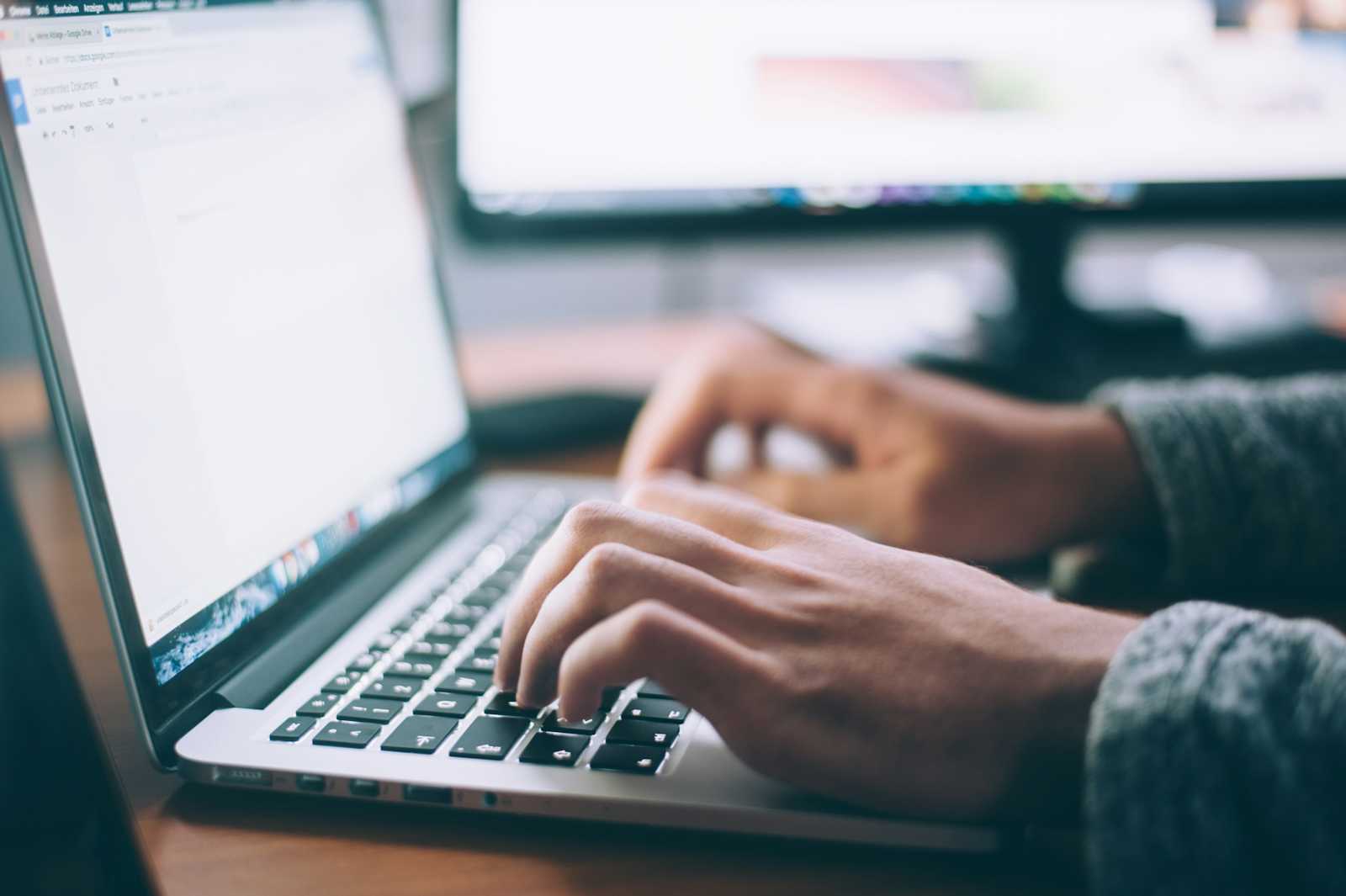
In today's rapidly evolving tech landscape, artificial intelligence (AI) is no longer a futuristic concept but a present reality. For Java developers using the Spring framework, the integration of Spring AI with Google Vertex AI opens up a world of possibilities. In this blog post, we'll explore how to leverage advanced AI capabilities, particularly the Gemini model, in your Spring applications.
Getting Started with Spring AI and Vertex AI
Before we dive into the nitty-gritty, let's set up our development environment to work with Spring AI and Vertex AI.
Step 1: Adding Dependencies
First, you'll need to include the necessary dependencies in your project. If you're using Maven, add the following to your pom.xml
:
<dependency>
<groupId>org.springframework.ai</groupId>
<artifactId>spring-ai-vertex-ai-gemini-spring-boot-starter</artifactId>
</dependency>
For Gradle users, add this to your build.gradle
:
implementation 'org.springframework.ai:spring-ai-vertex-ai-gemini-spring-boot-starter'
Step 2: Configuration
Next, configure your application to connect to Vertex AI. Add these properties to your application.properties
file:
spring.ai.vertex.ai.gemini.project-id=YOUR_PROJECT_ID
spring.ai.vertex.ai.gemini.location=YOUR_LOCATION
spring.ai.vertex.ai.gemini.chat.options.model=vertex-pro-vision
spring.ai.vertex.ai.gemini.chat.options.temperature=0.5
Remember to replace YOUR_PROJECT_ID
and YOUR_LOCATION
with your actual Google Cloud project details.
Step 3: Creating Necessary Beans
Implement the required beans in your Spring application to interact with Vertex AI:
@Bean
public VertexAI vertexAI(GoogleCredentials googleCredentials) throws IOException {
return new VertexAI(YOUR_PROJECT_ID, YOUR_LOCATION, googleCredentials);
}
Harnessing the Power of the Gemini Model
Google's Gemini model is a state-of-the-art AI model that can be leveraged for various tasks. Here's how you can set it up in your Spring application:
@Bean
public GenerativeModel geminiProVision(VertexAI vertexAI) {
GenerationConfig generationConfig = GenerationConfig.newBuilder()
.setMaxOutputTokens(2048)
.setTemperature(0.4F)
.setTopK(32)
.setTopP(1)
.build();
return new GenerativeModel("gemini-1.0-pro-vision-001", generationConfig, vertexAI);
}
This configuration sets up the Gemini model with specific parameters for token generation, temperature, and other factors that influence the model's output.
Securing Your Integration
Security is paramount when working with AI services. Use a service account for authentication:
@Bean
public GoogleCredentials googleCredentials() throws IOException {
return GoogleCredentials.fromStream(new ClassPathResource("path/to/credentials.json").getInputStream());
}
Ensure that your service account has the necessary permissions to access Vertex AI.
Best Practices for Spring AI and Vertex AI Integration
Token Management: Implement a robust strategy for managing access tokens and refresh tokens. This ensures secure and efficient authentication with the Vertex AI services.
Error Handling: Don't forget to include comprehensive error handling in your code. This should cover potential issues like API timeouts or authentication failures.
Testing: Before deploying to production, thoroughly test your integration in a development environment. This step is crucial to ensure all components work seamlessly together.
Conclusion
Integrating Spring AI with Google Vertex AI opens up exciting possibilities for Java developers. By following the steps and best practices outlined in this blog post, you can harness the power of advanced AI models like Gemini in your Spring applications.
Remember, the world of AI is constantly evolving. Stay updated with the latest developments in Spring AI and Vertex AI to make the most of these powerful tools. Happy coding!
Subscribe to my newsletter
Read articles from Nikhil Soman Sahu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
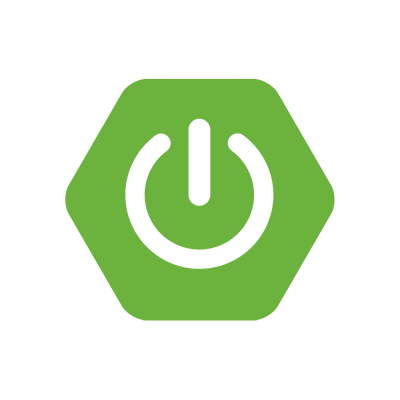
Nikhil Soman Sahu
Nikhil Soman Sahu
Software Developer