Understanding the DOM

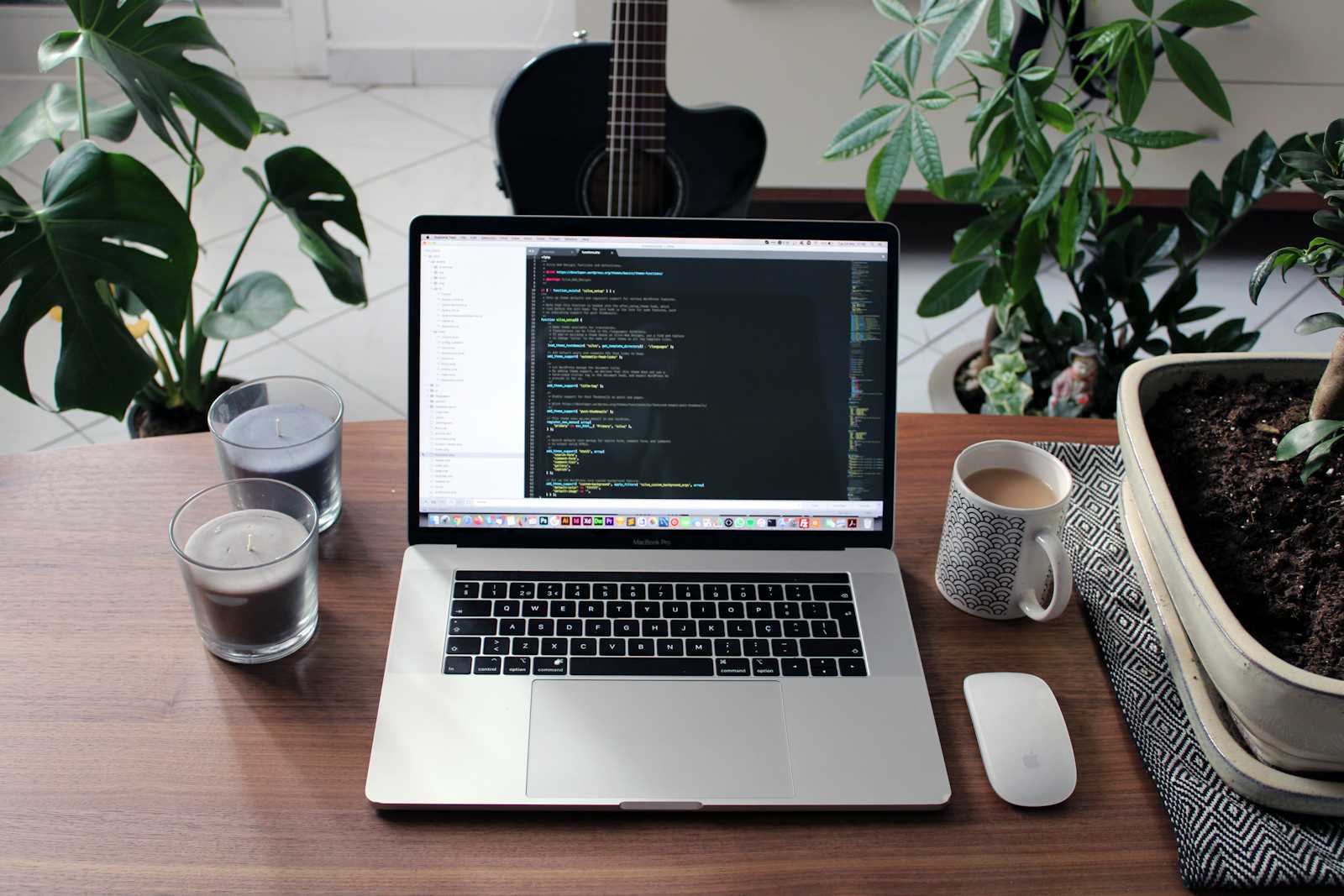
Have you been seeing this acronym DOM and wondered what it means, or do you know what it means but want to learn more about it? Then welcome to another episode of Ridwan’s Nugget.
DOM (Document Object Model) is like a bridge that connects HTML to the programming languages used in manipulating web pages, such as JavaScript. It is important to understand how the DOM works because it is necessary for creating dynamic and interactive web applications.
The DOM is like a tree-like structure that represents the HTML content of a web page. Every part of the HTML, including the elements, attributes, and texts in the HTML, is a NODE in this tree. The browser will automatically create the DOM when it loads the page, making it easier for the developer to interact with the document structure, style, and content through programming languages.
Nodes and Elements
Just in case you felt lost above, these examples will explain what I meant by Nodes better.
Element Nodes: These are the HTML tags present in the document. For example,
<div>
,<p>
,<h1>
,<span>
, etc.Text Nodes: These are the texts that are present inside the elements. For example,
<p>Hello World!</p>
Attribute Nodes: These are the attributes that represent the elements. For example,
<p class="greetings">Hello World!</p>
,<p id="greetings">Hello World!</p>
. Here, theid
andclass
are the attributes.
How to Manipulate the DOM Using JavaScript
There are several methods to interact with and manipulate the DOM using JavaScript, but I will provide you with the common ones.
Selecting Elements You would first need to select the element you want to interact with or manipulate and make it available in the programming language you intend to use. Here we are using JavaScript. Below are ways you can select your elements:
getElementById()
: This allows you to select an element by its id attribute:
const element = document.getElementById('myId');
//Replace the "myId" with your own id
getElementByClassName()
: This allows you to select an element by its class attribute:
const element = document.getElementsByClassName('myClass');
//Replace the "myClass" with your own class
querySelectorAll()
: This allows you to select all elements that match a CSS selector:
const elements = document.querySelectorAll('div.myClass');
//Replace the "myClass" with your own class
Modifying Elements you might be wondering what comes after selecting the elements, what else can we do with them? We have many things we can do with our elements after selecting them, and below are some examples:
- Changing Contents: After selecting our elements, we can easily change the content of the element, which can be helpful when creating dynamic web applications. Below are examples:
element.textContent = 'New content';
element.innerHTML = '<span>New HTML content</span>';
- Changing Attributes: This is fun to me as a React developer because I use it every day, and it is really helpful. This is how you can change the attribute of an element using JavaScript:
element.setAttribute('class', 'newClass');
element.id = 'newId';
Changing Styles: Just like CSS, we can also use JavaScript to change our style, and this is possible using the DOM. Below is an example:
element.style.color = 'red'; element.style.display = 'none';
Creating and Inserting Elements
Creating New Elements:
const newDiv = document.createElement('div'); newDiv.textContent = 'Hello, World!';
Appending Elements:
document.body.appendChild(newDiv);
Inserting Elements Before Another Element:
const referenceNode = document.querySelector('.myClass'); document.body.insertBefore(newDiv, referenceNode);
Removing Elements
element.remove();
Event Handling
The DOM gives the developer the opportunity to allow the web applications to listen and respond to users' interactions through event handling. Events can be attached to DOM elements to trigger JavaScript functions:
element.addEventListener('click', function() {
alert('Element clicked!');
});
Mastering DOM manipulation is essential for any frontend developer because it gives you the chance to create an interactive web experience by making your web content dynamic by altering the content, structure, and style of web pages.
If you enjoy this once again. Follow me for more educative episodes of Ridwan’s nugget.
Happy coding!!
Subscribe to my newsletter
Read articles from Ridwan Tajudeen directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ridwan Tajudeen
Ridwan Tajudeen
I am a knowledgeable frontend dev and writer. Adept at writing clear and concise technical articles.