Understanding JavaScript Event Propagation: A Chill Dive into the Bubbling World
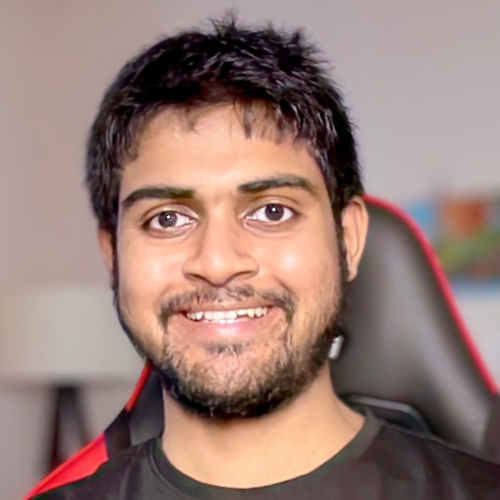
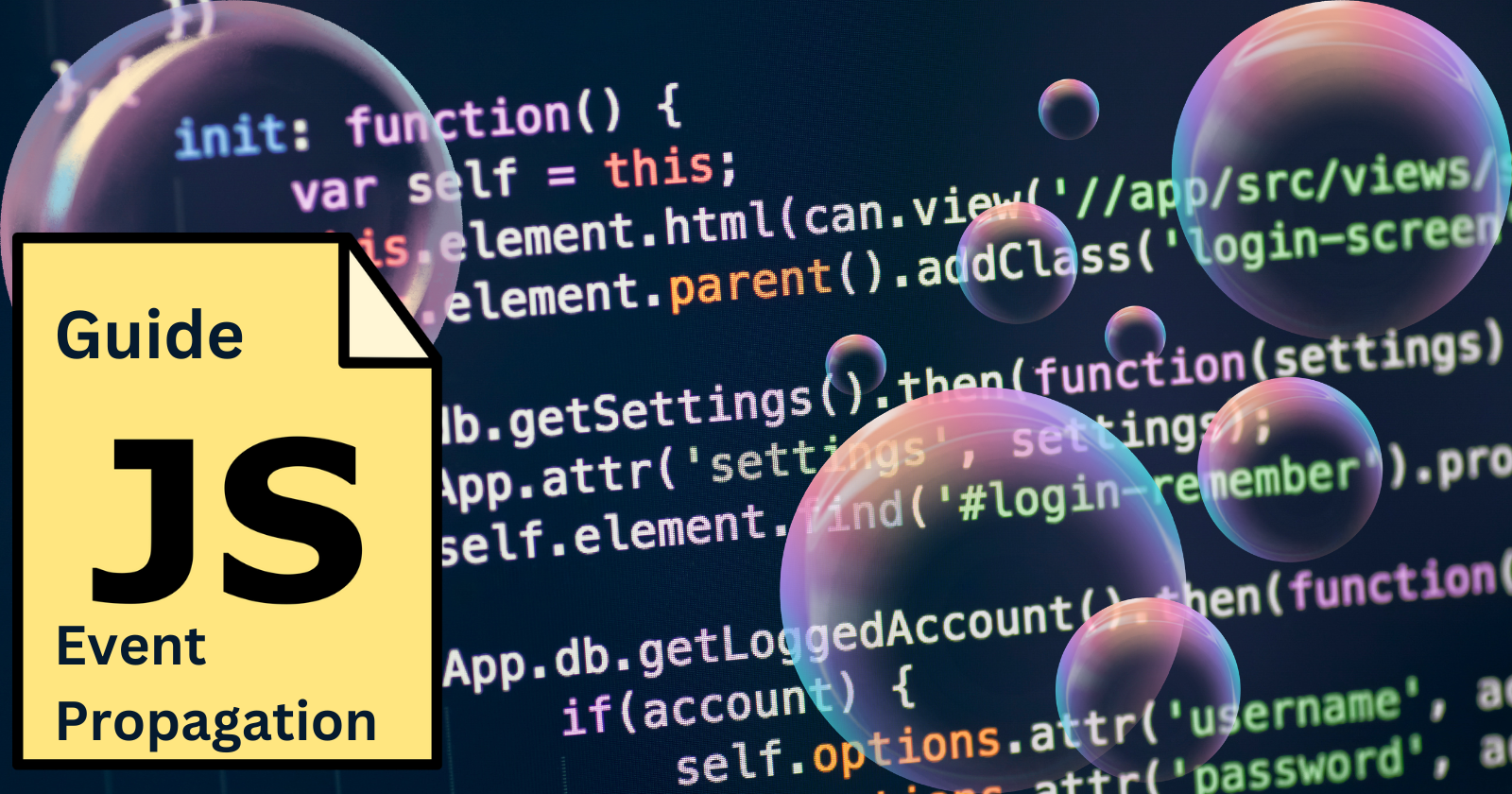
Hey there, fellow coders! 🌊 Ever found yourself puzzled by why a click on a tiny button also triggers something in its parent element? You're not alone! Welcome to the world of JavaScript event propagation, where events bubble up, and things get a little... unpredictable. But don't worry—I'm here to break it down in a way that’s as smooth as your favorite playlist on a lazy afternoon. 🎶
What is Event Propagation?
Imagine this: you're at a party (the DOM), and you tap someone on the shoulder (an element). But somehow, everyone between you and the DJ (the top-level element) thinks you tapped them too. That's event propagation in a nutshell!
In JavaScript, when you interact with an element (like clicking a button), the event doesn’t just stay there. It has two main phases: capturing and bubbling.
Capturing Phase: The event starts from the top (think of it as the bouncer at the door) and trickles down to the element you actually clicked.
Bubbling Phase: After the event hits your target, it starts bubbling up—like a good fizzy drink—back through the parent elements all the way to the top.
Why Does This Matter?
Let’s say you have a list item (li
) inside an unordered list (ul
), which is inside a div
. You click the li
, but surprise! The ul
and div
also catch wind of that click, and any event handlers attached to them will fire too. Sometimes that’s cool, but other times, it’s like trying to chill in a coffee shop only to be bombarded by loud conversations from the next table over.
Here’s a basic example to show how it works:
<div id="parent-div">
<ul>
<li>Click me!</li>
</ul>
</div>
// Adding event listeners
document.getElementById('parent-div').addEventListener('click', function() {
console.log('Div clicked!');
});
document.querySelector('ul').addEventListener('click', function() {
console.log('UL clicked!');
});
document.querySelector('li').addEventListener('click', function() {
console.log('LI clicked!');
});
If you click on the li
, you’ll see this in your console:
LI clicked!
UL clicked!
Div clicked!
The event starts at the li
, bubbles up to the ul
, and then to the div
. It’s like a domino effect, but with clicks!
Controlling the Party: Stopping the Propagation
Sometimes, you don’t want the party to spread. Maybe you want the li
to be the only element that reacts. This is where stopPropagation()
comes in—a true lifesaver for those moments when you just need a little peace.
Let’s update our example:
document.querySelector('li').addEventListener('click', function(event) {
console.log('LI clicked!');
event.stopPropagation(); // The party stops here!
});
Now, when you click on the li
, the console will only log:
LI clicked!
No more uninvited guests (aka ul
and div
) crashing the event!
The Big Chill: When to Use What
Bubbling: It’s perfect for when you want events to naturally flow up the DOM. For instance, a delete button inside a list item can trigger a delete function for the entire item, which is super handy!
stopPropagation(): Use it when you need to contain the event. Maybe you have a modal with buttons inside it, and you don’t want the background elements reacting when you click something inside the modal.
Wrapping It Up
And there you have it—event propagation explained with all the chill vibes you need. 🛋️ Whether you’re dealing with bubbling chaos or trying to keep things contained, understanding how events move through the DOM will make your coding life a whole lot smoother.
So next time you find yourself wondering why clicking one thing affects another, just remember: it’s all part of the party that is JavaScript event propagation. And now you know how to be the DJ, controlling the flow just the way you like it. 🎧
Happy coding, and keep it chill! ✌️
Subscribe to my newsletter
Read articles from Junaid Bin Jaman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
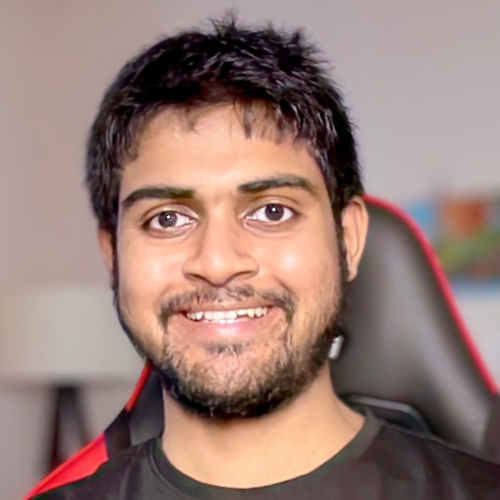
Junaid Bin Jaman
Junaid Bin Jaman
Hello! I'm a software developer with over 6 years of experience, specializing in React and WordPress plugin development. My passion lies in crafting seamless, user-friendly web applications that not only meet but exceed client expectations. I thrive on solving complex problems and am always eager to embrace new challenges. Whether it's building robust WordPress plugins or dynamic React applications, I bring a blend of creativity and technical expertise to every project.