Handling Vectors and Factors in R: Avoiding the Factor-Variable Trap
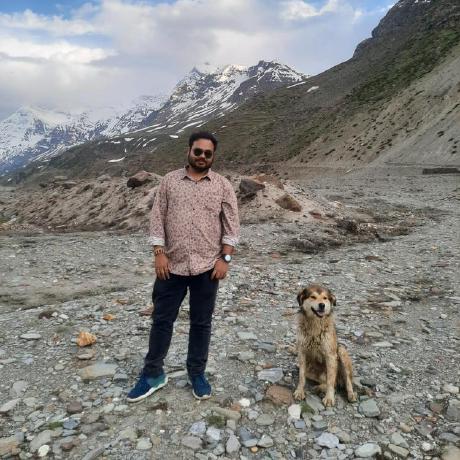

R is a versatile language designed for statistical computing and data analysis, offering powerful tools for managing different data types like vectors and factors. However, these tools can sometimes lead to common pitfalls, such as the factor-variable trap. This blog will guide you through effectively managing vectors and factors in R, helping you avoid these potential issues.
Understanding Vectors in R
What are Vectors?
In R, vectors are one-dimensional arrays that store elements of the same type. They are fundamental to R programming, enabling efficient data storage and manipulation. There are several types of vectors in R:
Atomic Vectors: The simplest type of vector, where all elements must be of the same type. You can create atomic vectors using the
c()
function.# Creating a numeric vector numeric_vector <- c(1, 2, 3, 4, 5) print(numeric_vector) print(class(numeric_vector)) # Check the class print(typeof(numeric_vector)) # Check the type
Expected Output:
[1] 1 2 3 4 5 [1] "numeric" [1] "double"
Lists: Unlike atomic vectors, lists can hold elements of different types. They are created using the
list()
function.# Creating a list with mixed types mixed_list <- list(1, "a", TRUE) print(mixed_list) print(class(mixed_list)) # Check the class print(typeof(mixed_list)) # Check the type
Expected Output:
[[1]] [1] 1 [[2]] [1] "a" [[3]] [1] TRUE [1] "list" [1] "list"
Matrices and Arrays: Matrices are two-dimensional arrays created from vectors by specifying a
dim
attribute.# Creating a matrix matrix_example <- matrix(1:6, nrow = 2) print(matrix_example) print(class(matrix_example)) # Check the class print(typeof(matrix_example)) # Check the type
Expected Output:
[,1] [,2] [,3] [1,] 1 3 5 [2,] 2 4 6 [1] "matrix" "array" [1] "integer"
Working with Vectors
One of R's strengths is its support for vectorized operations, allowing you to perform operations on entire vectors simultaneously, which is both time-efficient and concise.
# Vectorized addition
result <- numeric_vector + 1
print(result)
print(class(result)) # Check the class
print(typeof(result)) # Check the type
Expected Output:
[1] 2 3 4 5 6
[1] "numeric"
[1] "double"
Indexing and Subsetting
You can easily access and manipulate vector elements using indexing or logical conditions.
# Accessing the first element
first_element <- numeric_vector[1]
print(first_element)
print(class(first_element)) # Check the class
print(typeof(first_element)) # Check the type
Expected Output:
[1] 1
[1] "numeric"
[1] "double"
# Logical indexing to find elements greater than 2
greater_than_two <- numeric_vector[numeric_vector > 2]
print(greater_than_two)
print(class(greater_than_two)) # Check the class
print(typeof(greater_than_two)) # Check the type
Expected Output:
[1] 3 4 5
[1] "numeric"
[1] "double"
Working with Factors in R
What are Factors?
Factors in R represent categorical data. They store data as integers with corresponding labels, making them efficient for statistical analysis. Factors are particularly useful in regression models and other statistical contexts.
# Creating a factor
gender <- factor(c("male", "female", "female", "male"))
print(gender)
print(class(gender)) # Check the class
print(typeof(gender)) # Check the type
Expected Output:
[1] male female female male
Levels: female male
[1] "factor"
[1] "integer"
Levels and Ordering of Factors
Factors come with levels that define the possible categories. For ordinal data, you can create ordered factors that have a meaningful sequence.
# Creating an ordered factor
education <- factor(c("high school", "bachelor", "master"),
levels = c("high school", "bachelor", "master"),
ordered = TRUE)
print(education)
print(class(education)) # Check the class
print(typeof(education)) # Check the type
Expected Output:
[1] high school bachelor master
Levels: high school < bachelor < master
[1] "ordered" "factor"
[1] "integer"
Creating and Modifying Factors
Factors can be created using the factor()
function. You can also modify factor levels to suit your analysis needs.
# Changing factor levels
gender <- factor(c("male", "female", "female", "male"))
gender <- relevel(gender, ref = "female")
print(gender)
print(class(gender)) # Check the class
print(typeof(gender)) # Check the type
Expected Output:
[1] male female female male
Levels: female male
[1] "factor"
[1] "integer"
Avoiding the Factor-Variable Trap
What is the Factor-Variable Trap?
The factor-variable trap, also known as the dummy variable trap, can occur in regression analysis when all levels of a factor are included, leading to multicollinearity. To prevent this, R automatically drops one level—known as the reference level.
How to Relevel Factors
To control which level is treated as the reference in your analysis, you can relevel factors.
# Original factor
education <- factor(c("high school", "bachelor", "master", "bachelor"))
# Changing the reference level
education <- relevel(education, ref = "master")
print(education)
print(class(education)) # Check the class
print(typeof(education)) # Check the type
Expected Output:
[1] high school bachelor master bachelor
Levels: master high school bachelor
[1] "factor"
[1] "integer"
Practical Example: Regression with Factors
Let’s see how to avoid the factor-variable trap in a regression model:
# Sample data frame
data <- data.frame(
gender = factor(c("male", "female", "female", "male")),
score = c(85, 90, 95, 80)
)
# Fitting a linear model
model <- lm(score ~ gender, data = data)
summary(model)
Expected Output:
Call:
lm(formula = score ~ gender, data = data)
Residuals:
1 2 3 4
0.0000 2.5000 -2.5000 0.0000
Coefficients:
Estimate Std. Error t value Pr(>|t|)
(Intercept) 82.500 1.250 66.00 0.00964 **
genderfemale 7.500 1.768 4.24 0.14754
---
Signif. codes:
Visualizing Factors
Using ggplot2
, you can easily visualize the impact of factors on your data.
# Load ggplot2
library(ggplot2)
# Plotting the data
ggplot(data, aes(x = gender, y = score)) +
geom_boxplot() +
labs(title = "Score by Gender", x = "Gender", y = "Score")
Expected Output:
- A boxplot showing the distribution of scores by gender, with one box for each gender level.
Best Practices for Working with Vectors and Factors
Avoid Automatic Conversion: When importing data, use
stringsAsFactors = FALSE
in functions likeread.csv()
to prevent R from automatically converting strings to factors.# Reading data without converting strings to factors df <- read.csv("data.csv", stringsAsFactors = FALSE) print(class(df$column_name)) # Check the class of a column print(typeof(df$column_name)) # Check the type of a column
Expected Output:
[1] "character" [1] "character"
Check Factor Levels: Always check the levels of your factors using
levels()
to ensure they align with your expectations.# Checking factor levels print(levels(gender))
Expected Output:
[1] "female" "male"
Leverage
dplyr
for Factor Manipulation: Thedplyr
package provides powerful tools for manipulating factors, including functions likemutate()
andrecode()
.
# Recode factors using dplyr
library(dplyr)
df <- data.frame(gender = factor(c("male", "female", "female", "male")))
df <- df %>% mutate(gender = recode(gender, male = "M", female = "F"))
print(df$gender)
print(class(df$gender)) # Check the class
print(typeof(df$gender)) # Check the type
Expected Output:
[1] M F F M
Levels: F M
[1] "factor"
[1] "integer"
Handling vectors and factors in R can be straightforward if you understand the underlying concepts and avoid common pitfalls like the factor-variable trap. By following these best practices, you can ensure your data analysis is both accurate and efficient.
2024 | Indraneel Chakraborty
Subscribe to my newsletter
Read articles from Indraneel Chakraborty directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
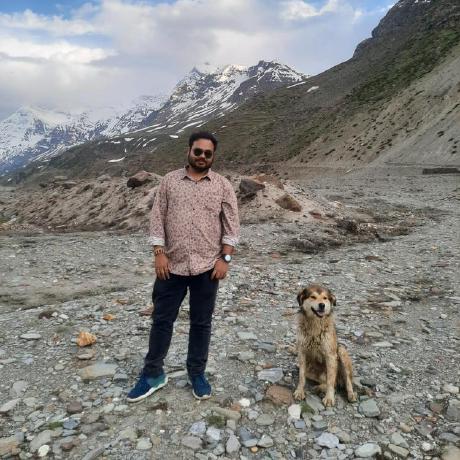
Indraneel Chakraborty
Indraneel Chakraborty
I am Indraneel Chakraborty - a recovering Bioinformatician in love with Technology, Data Science and DevOps. Solving problems (not limited to Bioinformatics) with code-first, data-centric approaches on cloud architecture is my primary focus. Currently, I'm working with Elucidata as a Bioinformatics Engineer, helping teams to scale up using advanced workflow management systems like Nextflow and cloud based solutions to effectively manage technological resources, thereby cutting costs and time taken in providing ML ready biomedical data. Other than these, I am also involved in development of webapps using R-Shiny (R programming) and Streamlit (Python). Apart from my full time job, I also volunteer as an application creator at Streamlit, open source lesson maintainer at The Carpentries, technical reviewer at Packt Publications, Community member at Data Science Festival London and beta tester at Coursera. Found my profile interesting? Lets talk!