Understanding VueJS ( ref: Reactive Reference ) A Comprehensive Guide

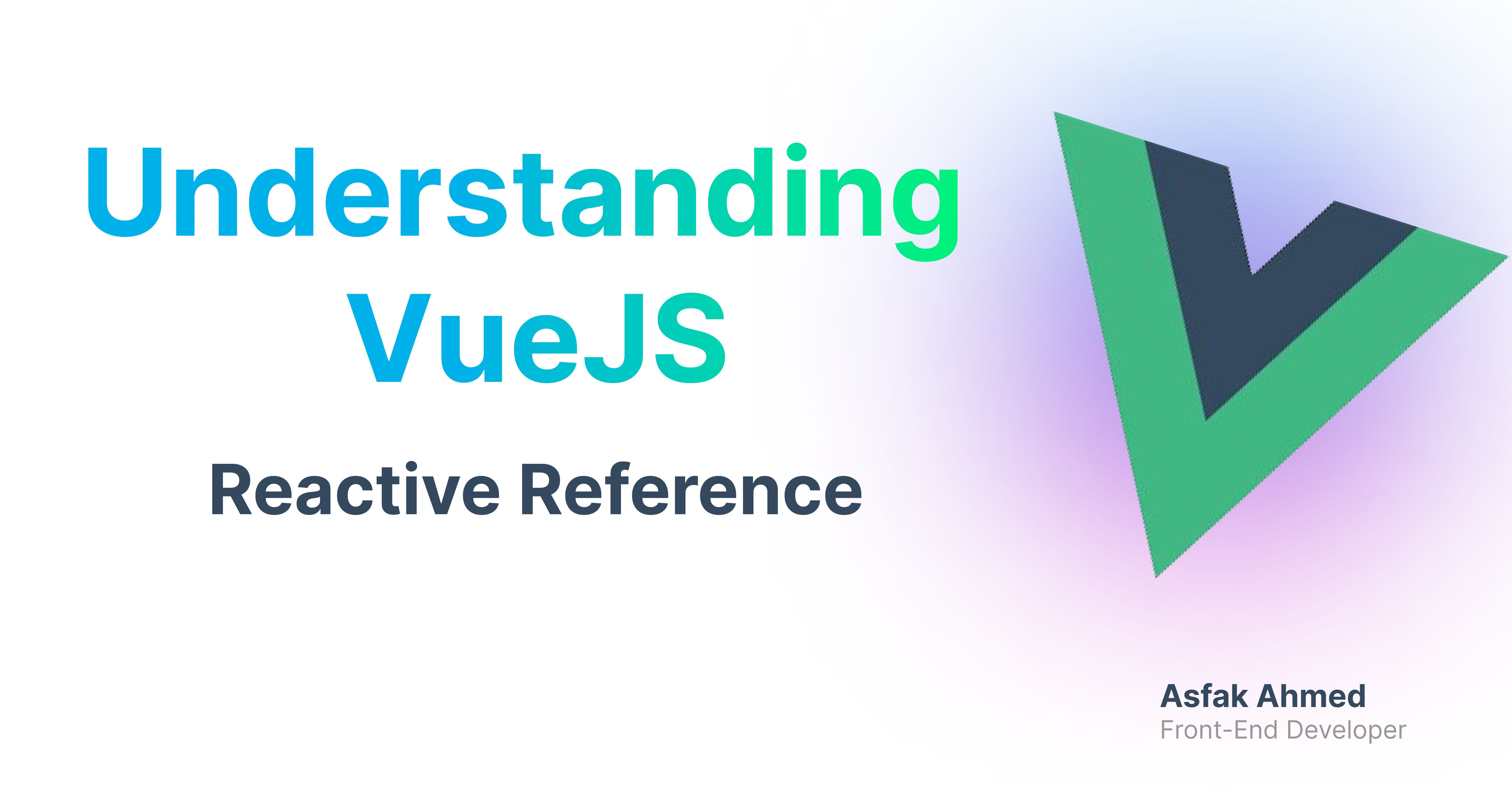
Vue.js is one of the most popular frameworks for building user interfaces, and one of its powerful features is the ref
function, which is part of the Vue 3 Composition API. The ref
function allows developers to create reactive references to primitive values, complex objects, or DOM elements. In this article, we'll dive deep into the concept, its usage, and how it helps build dynamic and responsive applications.
What is ref
in Vue.js?
The ref
function is used to create a reactive reference to a value. In Vue.js, reactivity is a core feature that allows the UI to update when the underlying data changes automatically. ref
is a way to wrap a value in a reactive container, enabling Vue to track changes and update the DOM accordingly.
Why Use ref
?
Reactivity for Primitive Values: While Vue's
reactive
function is great for objects and arrays, it doesn't work with primitive values (e.g., numbers, strings, booleans).ref
allows you to create a reactive reference to primitive values, making it easier to manage the state.Access to DOM Elements: When working with templates, you might need direct access to DOM elements. The
ref
function allows you to reference DOM elements directly, enabling you to manipulate them as needed.Simple API for Reactivity:
ref
provides a straightforward way to create reactive data. It encapsulates the value and exposes a.value
property to access or update it.
When to Use ref
?
Handling Primitive Data: When you need to work with primitive data types reactively,
ref
is the go-to solution.DOM Manipulation: If you need to interact with or manipulate a DOM element directly,
ref
provides a way to reference that element.Tracking Single Values: If you need to track a single value that may change over time, such as a counter or a toggle state,
ref
is ideal.
How Does ref
Work?
The ref
function returns a reactive object that contains the original value. This object has a single property, .value
which holds the wrapped value. When the value changes, Vue's reactivity system detects the change and updates the DOM automatically.
Basic Example of ref
Let's start with a simple example of using ref
to create a reactive counter:
<template>
<div>
<p>Count: {{ count.value }}</p>
<button @click="increment">Increment</button>
</div>
</template>
<script setup>
import { ref } from 'vue';
const count = ref(0);
const increment = () => {
count.value++;
};
</script>
Explanation:
Creating a
ref
:const count = ref(0);
creates a reactive reference to the initial value0
.Accessing the Value:
{{ count.value }}
is used in the template to access the current value ofcount
.Updating the Value:
count.value++;
increments the value, and the DOM updates automatically.
Using ref
with Objects
Although ref
is primarily used for primitive values, you can also use it to create reactive references to objects. This is particularly useful when you want to track a single object but don't want the entire object to be reactive.
<template>
<div>
<p>{{ user.value.name }}</p>
<button @click="changeName">Change Name</button>
</div>
</template>
<script setup>
import { ref } from 'vue';
const user = ref({
name: 'John Doe',
age: 30
});
const changeName = () => {
user.value.name = 'Jane Doe';
};
</script>
Explanation:
Creating a
ref
:const user = ref({...});
wraps theuser
object in a reactive reference.Accessing the Value:
{{
user.value.name
}}
allows access to thename
property of theuser
object.Updating the Value:
user.value.name
= 'Jane Doe';
changes the name, and Vue updates the DOM accordingly.
Using ref
DOM Elements
In Vue, ref
can also be used to get direct access to DOM elements. This is particularly useful when you need to perform operations that require direct interaction with the DOM.
<template>
<div>
<input ref="inputRef" type="text" placeholder="Type something..." />
<button @click="focusInput">Focus Input</button>
</div>
</template>
<script setup>
import { ref } from 'vue';
const inputRef = ref(null);
const focusInput = () => {
inputRef.value.focus();
};
</script>
Explanation:
Creating a
ref
:const inputRef = ref(null);
creates a reference to the input element, which will be populated when the element is rendered.Accessing the Element:
inputRef.value
holds the DOM element, allowing you to call native DOM methods likefocus()
.
Key Points to Remember
Reactive Wrapper: The
ref
function wraps a value and makes it reactive. It automatically updates the DOM when the value changes..value
Property: Always access and modify the value inside aref
using the.value
property.Primitives and Objects:
ref
can be used with both primitive values and objects, although for complex objects,reactive
might be a better choice.DOM Manipulation:
ref
is a powerful tool for accessing and manipulating DOM elements directly from your Vue components.
Advantages of Using ref
Simplicity:
ref
provides a straightforward way to manage reactive state without the complexity of Vuex or other state management libraries.Fine-Grained Control: You get precise control over what part of your state is reactive, helping you optimize performance and avoid unnecessary re-renders.
Flexibility:
ref
can be used for a wide range of purposes, from managing simple counters to interacting with the DOM.
The ref
function is an essential part of the Vue 3 Composition API, providing a simple yet powerful way to create reactive references to data and DOM elements. Whether you're managing state or interacting with the DOM, ref
offers a flexible solution that enhances the reactivity system in Vue.js.
Subscribe to my newsletter
Read articles from Asfak Ahmed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Asfak Ahmed
Asfak Ahmed
I'm Asfak Ahmed, a Front-End Developer with 2+ years of experience, currently enhancing user experiences at Doplac CRM. As the founder of ZenUI Library, I focus on creating intuitive, user-centric interfaces. My passion lies in blending creativity with technology to craft impactful digital solutions.