Mastering Asynchronous JavaScript: Real-World Applications of Promise.allSettled vs. Promiss.all
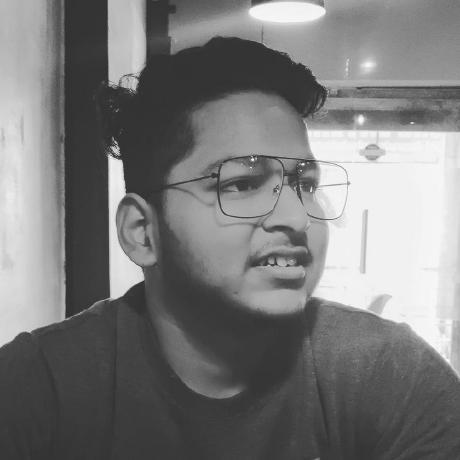
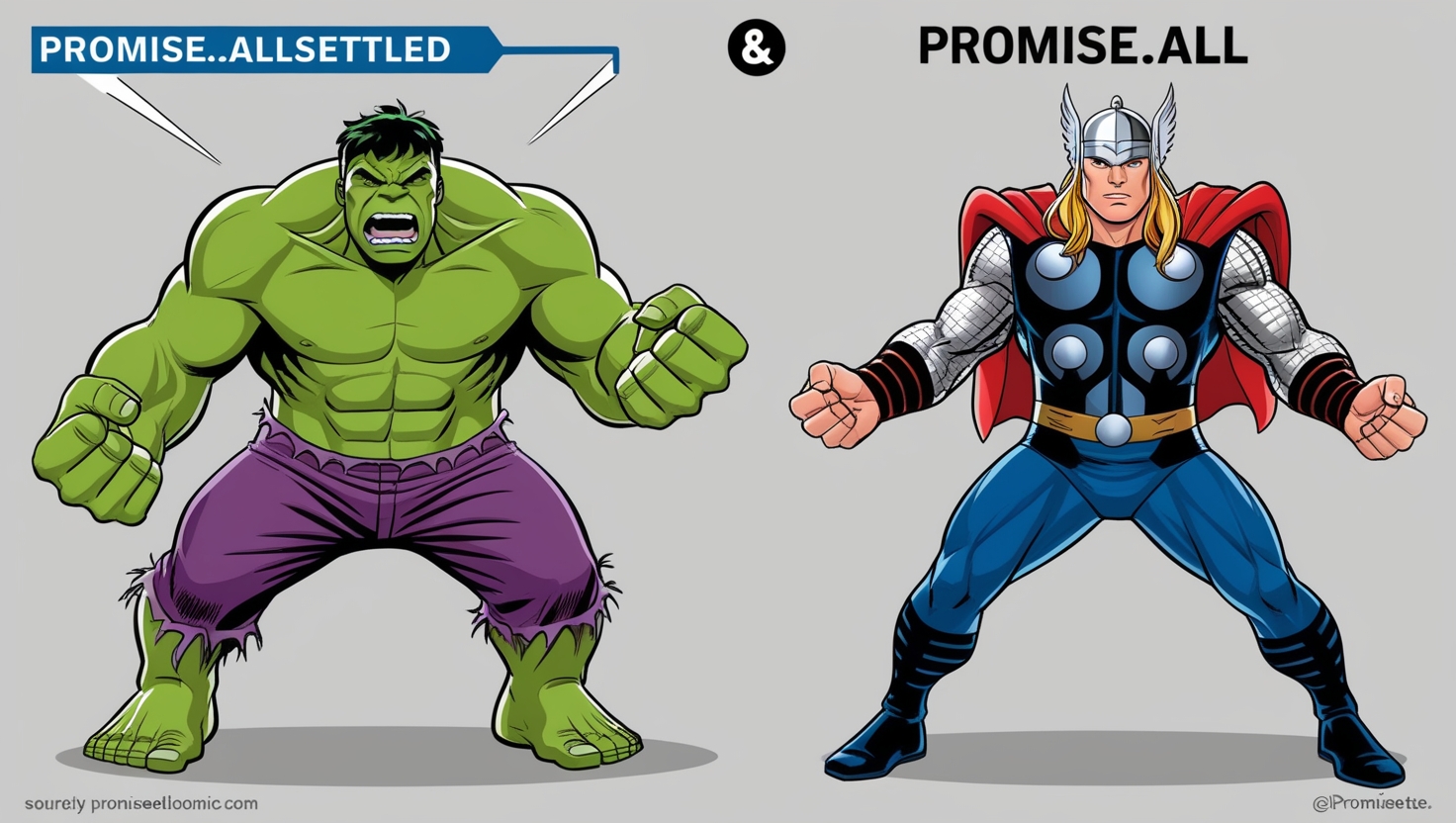
async/await:
Basically async/await is a syntactical sugar build on top of promises, It was introduce in ES2017 . It provides more readability as compare to promises. It provides a way to write a Asynchronous code in synchronous style.
Let's take a real-world example of implementing fetch Api in both way using async/await and promise.
Example (using promise) :
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error('Error:', error);
});
Example (using async/await):
async function fetchData() {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
}
fetchData();
If you see the both example carefully you can see that in promise style we also handle the error using .catch() . But int async/await we did not handle any error.
Let see How we can handle the error
try catch method
.catch method
// using try and catch
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error:', error);
}
}
fetchData();
// using .catch method
async function fetchData() {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
}
fetchData().catch((err)=>console.log(err));
async and await both are the Keywords , Without async we can not use await.
async:
async keyword is use to declare the function as Asynchronous.
Indicates function may contain Asynchronous task using promises.
Return a promise automatically.
await:
Only use within async function.
Pauses the execution of the function until the promise it's used with resolves.
Returns the resolved value of the promise.
Imagine you're cooking a meal. You put a pot of water on the stove to boil. While you wait for the water to boil, you can't do anything else with the pot until it's ready.
In JavaScript, await
is like a "wait" command. When you use await
before an asynchronous operation (like fetching data from a server or waiting for a timeout), it tells JavaScript to "wait here until this operation finishes."
Guess the Output:
const timer1 = new Promise((resolve, reject) => {
setTimeout(() => {
resolve("timer1");
}, 5000);
});
const timer2 = new Promise((resolve, reject) => {
setTimeout(() => {
resolve("timer2");
}, 3000);
});
const fun = async () => {
const p1 = await timer1;
console.log(p1);
const p2 = await timer2;
console.log(p2);
};
fun();
Output will be timer1 then immediately timer2 (not waiting for extra 3 sec. because timer1 execution takes 5s to execute and under this period timer2 is executed in 3 sec but not return or print any thing as already mention await keyword hold the execution at that line).
Promise.all and Promise.allSettled
Let's understand with practical use case , If you are making an dashboard and you have to call multiple Api in single page, Then how will you achieve that
First thing comes in mind , Make an async function and in that call your all Api. You can do that but if any Api gives an error then your code will throw an error or else you have to handle using try and catch or .catch method for all the Api call. Now here you can use Promise.all and Promise.allSettled according to your usecase.
Promise.all
const fetchApi = (url) => fetch(url).then((res) => res.json());
const fetchData = async () => {
const apis = [
"https://dummyjson.com/products",
"https://dummyjso.com/product/1",
"https://dummyjson.com/products/search?q=phone",
];
const data = await Promise.all(apis.map((api) => fetchApi(api)));
console.log(data);
};
fetchData();
It return the array of Promise when it resolve.
Output:
[
{
products: [
[Object], [Object], [Object],
[Object], [Object], [Object],
],
total: 194,
skip: 0,
limit: 30
},
{
id: 1,
title: 'Essence Mascara Lash Princess',
description: 'The Essence Mascara Lash Princess is a popular mascara known for its volumizing and lengthening effects. Achieve dramatic lashes with this long-lasting and cruelty-free formula.',
category: 'beauty',
price: 9.99,
images: [
'https://cdn.dummyjson.com/products/images/beauty/Essence%20Mascara%20Lash%20Princess/1.png'
],
thumbnail: 'https://cdn.dummyjson.com/products/images/beauty/Essence%20Mascara%20Lash%20Princess/thumbnail.png'
},
{
products: [
[Object], [Object], [Object],
[Object], [Object], [Object],
[Object]
],
total: 23,
skip: 0,
limit: 23
}
]
If you will not use Promise.all then it return the array of promise as pending.
const fetchApi = (url) => fetch(url).then((res) => res.json());
const fetchData = async () => {
const apis = [
"https://dummyjson.com/products",
"https://dummyjso.com/product/1",
"https://dummyjson.com/products/search?q=phone",
];
const data = await apis.map((api) => fetchApi(api));
console.log(data);
};
fetchData();
output:-
[ Promise{ <pending> }, Promise{ <pending> }, Promise{ <pending> }]
🔴 But if any of the Api is not giving the response the it throws error.
Promise.allSettled:
const fetchApi = (url) => fetch(url).then((res) => res.json());
const fetchData = async () => {
const apis = [
"https://dummyjson.com/products",
"https://dummyjso.com/product/1",
"https://dummyjson.com/products/search?q=phone",
];
const data = await Promise.allSettled(apis.map((api) => fetchApi(api)));
console.log(data);
};
fetchData();
Output : -
[
{
status: 'fulfilled',
value: { products: [Array], total: 194, skip: 0, limit: 30 }
},
{
status: 'fulfilled',
value: {
id: 1,
title: 'Essence Mascara Lash Princess',
description: 'The Essence Mascara Lash Princess is a popular mascara known for its volumizing and lengthening effects. Achieve dramatic lashes with this long-lasting and cruelty-free formula.',
category: 'beauty',
}
},
{
status: 'fulfilled',
value: { products: [Array], total: 23, skip: 0, limit: 23 }
}
]
It returns an array of objects, where each object represents the result of a corresponding promise. Each object has two properties:
status
: This property indicates whether the promise was fulfilled or rejected. Its value can be either"fulfilled"
or"rejected"
.value
: If the promise was fulfilled, this property contains the resolved value.reason
: If the promise was rejected, this property contains the rejection reason.
🔴 now In this case if any Api is not working, In this condition also your code give output for remaining all other Api and with status rejected for all Api which gives error.
When to Use Which Approach?
Use
Promise.allSettled
when:Independent Tasks: You need to handle multiple tasks in parallel that don’t depend on each other, and you want to process all results, even if some fail.
Performance Optimization: You want to execute multiple tasks in parallel, especially when you don’t need to stop the execution if one task fails.
Use Promise.all
when:
- Dependent Tasks: Tasks are dependent on each other, and the failure of one task should prevent the execution of subsequent tasks.
Conclusion:
Both Promise.all and Promise.allSettled offer Powerful way to handle asynchronous operations in JavaScript. Promise.allSettled is excellent for handlind multiple independent task in parallel. ensuring that all result are processed. on the other hand, Promise.all is provide a way to handle all the dependent task, stopping execution upon failure.
Understanding when to use each approach allows you to write more efficient, maintainable, and reliable code.
Subscribe to my newsletter
Read articles from shivam kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
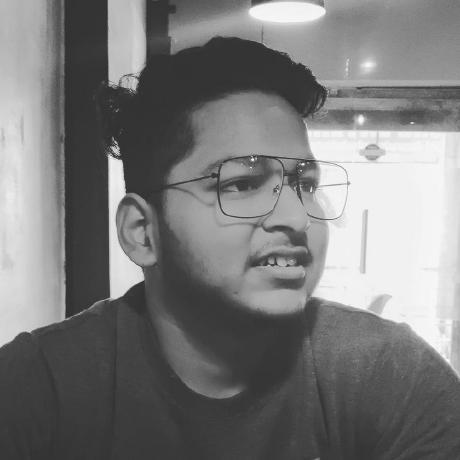