Exploring Python's Built-in enumerate() Function: An Underappreciated Gem

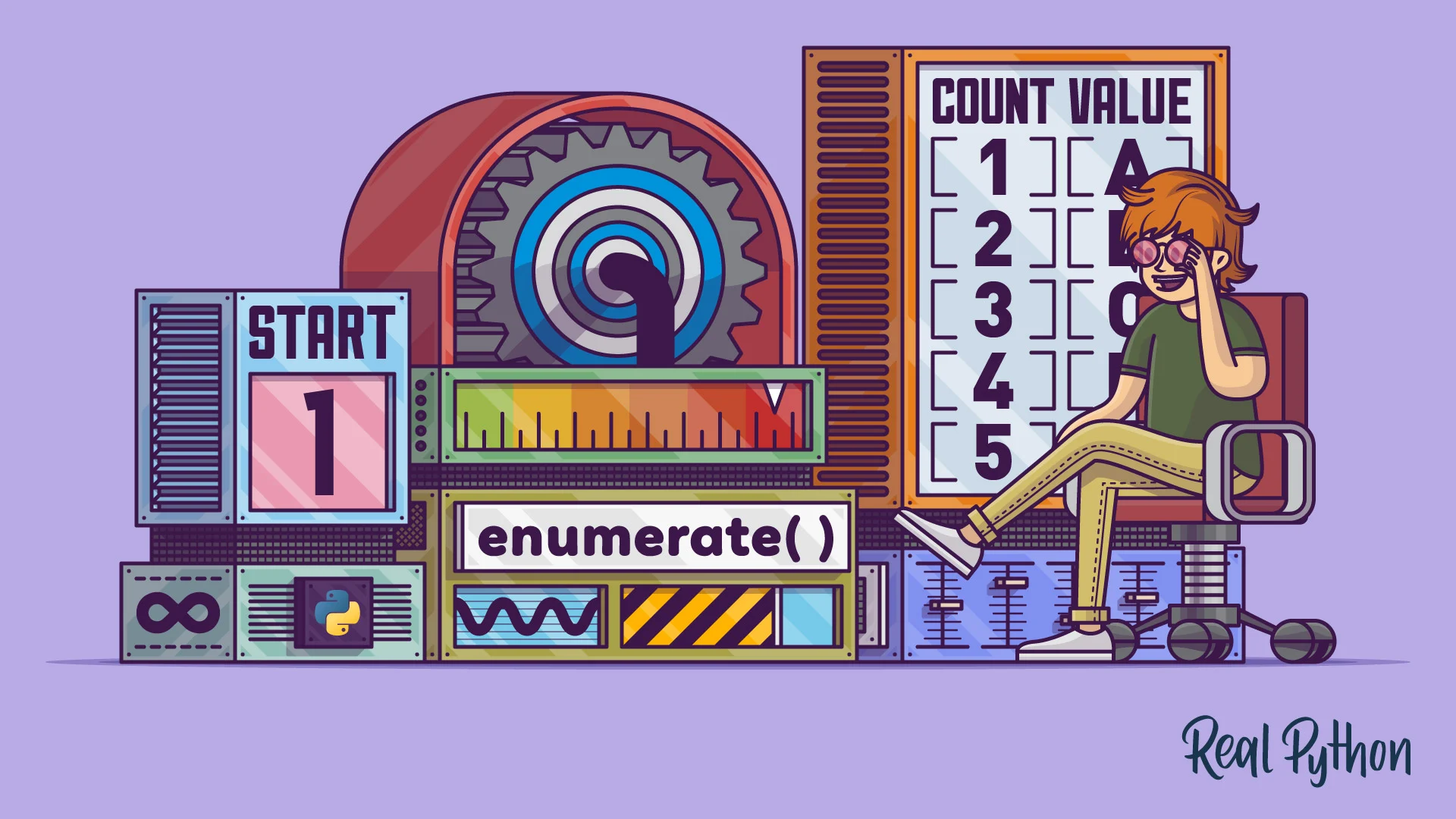
Python is known for its simplicity and readability, and part of that comes from its rich set of built-in functions. While many Python developers are familiar with functions like map()
, filter()
, or zip()
, there’s one that often flies under the radar: enumerate()
. This function, though basic, can significantly improve the clarity and efficiency of your code when iterating over sequences. In this blog, we'll explore what enumerate()
does, why you should use it, and some practical examples to illustrate its utility.
What is enumerate()
?
The enumerate()
function adds a counter to an iterable and returns it as an enumerate
object, which can then be used directly in for loops or converted into a list of tuples. Each tuple contains a pair of the index and the corresponding element from the original iterable.
Syntax:
iterable: The sequence you want to iterate over (e.g., a list, tuple, string).
start: The starting index of the counter (default is
0
).
Why Use enumerate()
?
1. Clarity and Readability
- When you need both the index and the value of items in an iterable, using
enumerate()
is more readable and less error-prone than managing a separate counter variable manually.
2. Less Code, Fewer Bugs
- By avoiding the manual increment of a counter, you reduce the chances of off-by-one errors and other common mistakes.
3. Pythonic Code
- Using
enumerate()
aligns with Python’s philosophy of writing clean, readable, and efficient code. It’s a more "Pythonic" way to iterate over sequences.
Basic Usage of enumerate()
Let’s start with a simple example to see enumerate()
in action:
In this example, enumerate()
returns both the index and the corresponding fruit in each iteration, making it easy to access both without additional code.
Custom Starting Index
Sometimes, you might want to start counting from a different number. The enumerate()
function allows you to specify the starting index:
Here, the index starts at 1
instead of 0
, which can be useful in scenarios where you want your count to be more user-friendly.
Practical Examples of enumerate()
1. Processing Lines in a File
When reading a file line by line,
enumerate()
can help you keep track of line numbers:2. Updating Elements in a List
If you need to update elements in a list while iterating,
enumerate()
is invaluable:
The enumerate()
function might seem like a small utility, but it can significantly streamline your loops, making your code cleaner and less error-prone. Whether you're processing lists, tuples, or even files, enumerate()
helps you keep track of indexes effortlessly, reducing the need for manual counters and enhancing your code's readability.
So next time you find yourself needing an index in a loop, remember enumerate()
—the underappreciated gem in Python’s treasure chest of built-in functions.
Subscribe to my newsletter
Read articles from Shrey Dikshant directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Shrey Dikshant
Shrey Dikshant
Aspiring data scientist with a strong foundation in adaptive quality techniques. Gained valuable experience through internships at YT Views, focusing on operation handling. Proficient in Python and passionate about data visualization, aiming to turn complex data into actionable insights.