Understanding the V8 Architecture: The Heart of Modern JavaScript
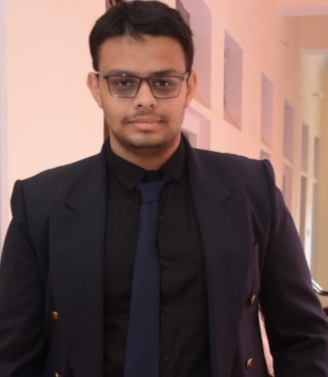
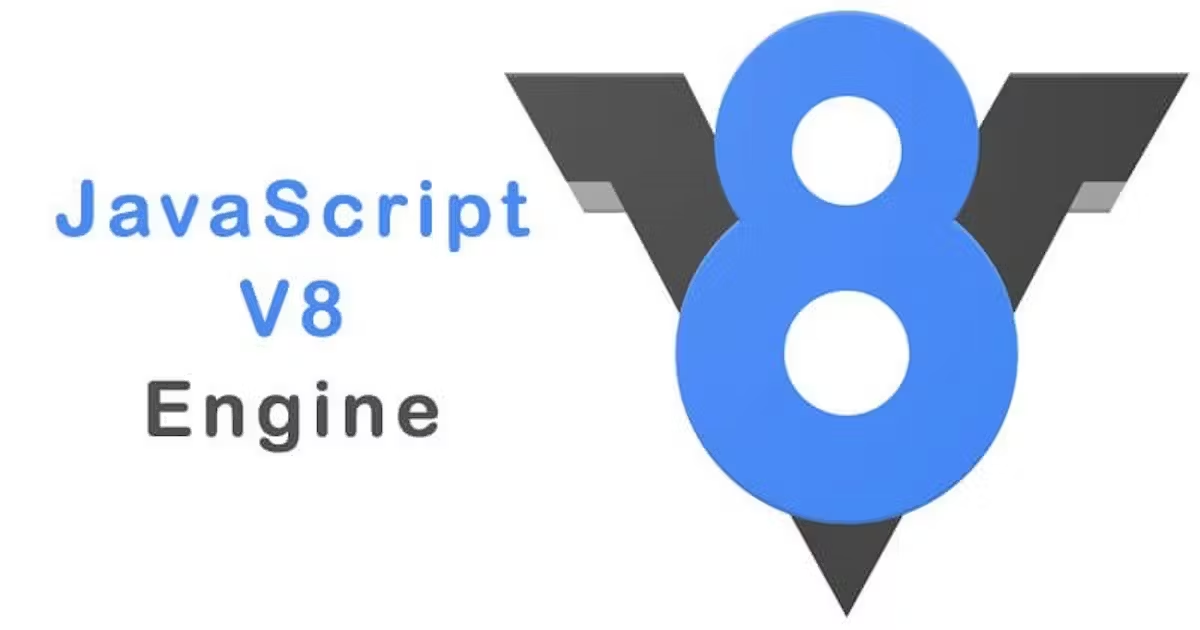
When I first started working with JavaScript in-depth, especially within the context of Node.js, I quickly realized the importance of understanding the underlying engine that powers it: V8. Developed by Google, V8 is an open-source JavaScript engine that not only powers Chrome but also Node.js, making it a key component in both client-side and server-side JavaScript applications.
My journey into exploring V8's architecture opened my eyes to how much optimization and engineering goes into making JavaScript fast and efficient. In this blog, I'll share my insights into V8's architecture, its components, and how they work together to execute JavaScript code efficiently.
What is V8?
V8 is a high-performance JavaScript and WebAssembly engine developed by Google. It is written in C++ and designed to execute JavaScript code in the Chrome browser and Node.js environment. V8 compiles JavaScript directly to native machine code, allowing for extremely fast execution.
Key Components of the V8 Architecture
Understanding V8's architecture involves looking at several critical components and stages that it uses to execute JavaScript code efficiently. Here’s a breakdown of how V8 operates under the hood:
Parser: The first stage in the V8 engine is parsing. When V8 receives JavaScript code, it passes it through a parser, which converts the source code into an Abstract Syntax Tree (AST). The AST is a tree representation of the code, detailing its structure and the relationships between various elements. This step is crucial because it transforms human-readable JavaScript code into a format that the engine can process.
Ignition Interpreter: Once the AST is generated, V8 uses a component called Ignition to interpret the code. Ignition is a bytecode interpreter that converts the AST into bytecode, which is a lower-level, intermediate representation of the JavaScript code. Ignition then begins executing this bytecode. This approach allows V8 to start running the code quickly without needing to compile it into machine code first. Ignition focuses on executing code as efficiently as possible while gathering information that can help with further optimizations.
TurboFan Compiler: As the code executes, V8 monitors and analyzes the execution patterns. If it identifies code that is frequently executed (hot code), it triggers a just-in-time (JIT) compilation process using a component called TurboFan. TurboFan compiles the hot bytecode into highly optimized machine code that can run directly on the CPU. This compilation process leverages the type feedback gathered during interpretation to make intelligent decisions about optimizations, ensuring the generated machine code is fast and efficient.
Garbage Collector: Memory management is a critical part of any programming language runtime, and V8 handles this using a garbage collector known as Orinoco. V8’s garbage collector uses a generational approach, dividing objects into two generations: young and old. Young objects are those that have been recently allocated, and old objects are those that have survived several garbage collection cycles. By focusing on collecting and cleaning up young objects more frequently, V8 minimizes the impact of garbage collection on performance. The garbage collector runs in the background, ensuring that memory is reclaimed efficiently without significantly impacting the execution of JavaScript code.
Optimized Code Deoptimization: One of the fascinating features of V8 is its ability to deoptimize code. If V8's assumptions about the code change (for example, if the type of a variable changes), it can revert the optimized machine code back to less optimized bytecode. This process is known as deoptimization, and it ensures that V8 can adapt to changing conditions in the code, maintaining both correctness and performance.
How V8 Executes JavaScript
Here’s a simplified flow of how V8 handles JavaScript code:
Parsing: JavaScript source code is parsed into an AST.
Bytecode Generation: Ignition converts the AST into bytecode.
Bytecode Execution: Ignition executes the bytecode, gathering type feedback.
JIT Compilation: TurboFan compiles hot code paths into optimized machine code.
Execution of Machine Code: The optimized machine code is executed directly by the CPU.
Garbage Collection: Orinoco reclaims memory no longer in use.
Deoptimization: If type assumptions change, the engine deoptimizes code back to bytecode.
My Experience with V8's Architecture
Learning about V8's architecture has profoundly influenced how I write and optimize JavaScript code. Here are a few key takeaways from my experience:
Writing Performance-Oriented Code: Understanding that V8 optimizes hot code means that I now pay more attention to writing efficient loops and critical code paths. I try to avoid code patterns that can lead to frequent deoptimization.
Memory Management Awareness: Knowing how V8’s garbage collector works has made me more mindful of memory allocation. I aim to reduce unnecessary object creation and consider the lifecycle of objects to minimize the workload on the garbage collector.
Leveraging the Power of Modern JavaScript: V8 is optimized for modern JavaScript features. Utilizing ES6 and beyond not only makes the code more expressive but also allows V8 to optimize it better. I embrace features like arrow functions, template literals, and destructuring to write cleaner, more efficient code.
Debugging with the V8 Inspector: V8’s architecture provides powerful debugging tools like the V8 Inspector, which can be accessed via Chrome DevTools. By using these tools, I can inspect memory usage, understand garbage collection patterns, and optimize performance-critical sections of my applications.
Conclusion: The Power of V8
V8’s architecture is a marvel of modern engineering, enabling JavaScript to be a powerful, performant language for both client-side and server-side applications. By understanding the inner workings of V8, developers can write more efficient JavaScript code, leading to faster applications and a better user experience.
For me, learning about V8’s architecture has been a game-changer, shaping how I approach JavaScript development. It has provided me with the insights needed to write code that not only works but works efficiently. The next time you run your JavaScript code, remember the incredible V8 engine that’s working behind the scenes to make everything happen seamlessly.
Subscribe to my newsletter
Read articles from SANKALP HARITASH directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
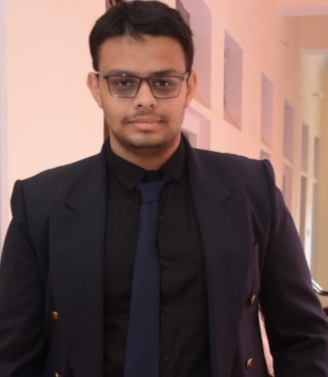
SANKALP HARITASH
SANKALP HARITASH
Hey 👋🏻, I am , a Software Engineer from India. I am interested in, write about, and develop (open source) software solutions for and with JavaScript, ReactJs. 📬 Get in touch Twitter: https://x.com/SankalpHaritash Blog: https://sankalp-haritash.hashnode.dev/ LinkedIn: https://www.linkedin.com/in/sankalp-haritash/ GitHub: https://github.com/SankalpHaritash21 📧 Sign up for my newsletter: https://sankalp-haritash.hashnode.dev/newsletter