How to Create a React Native Video Call App

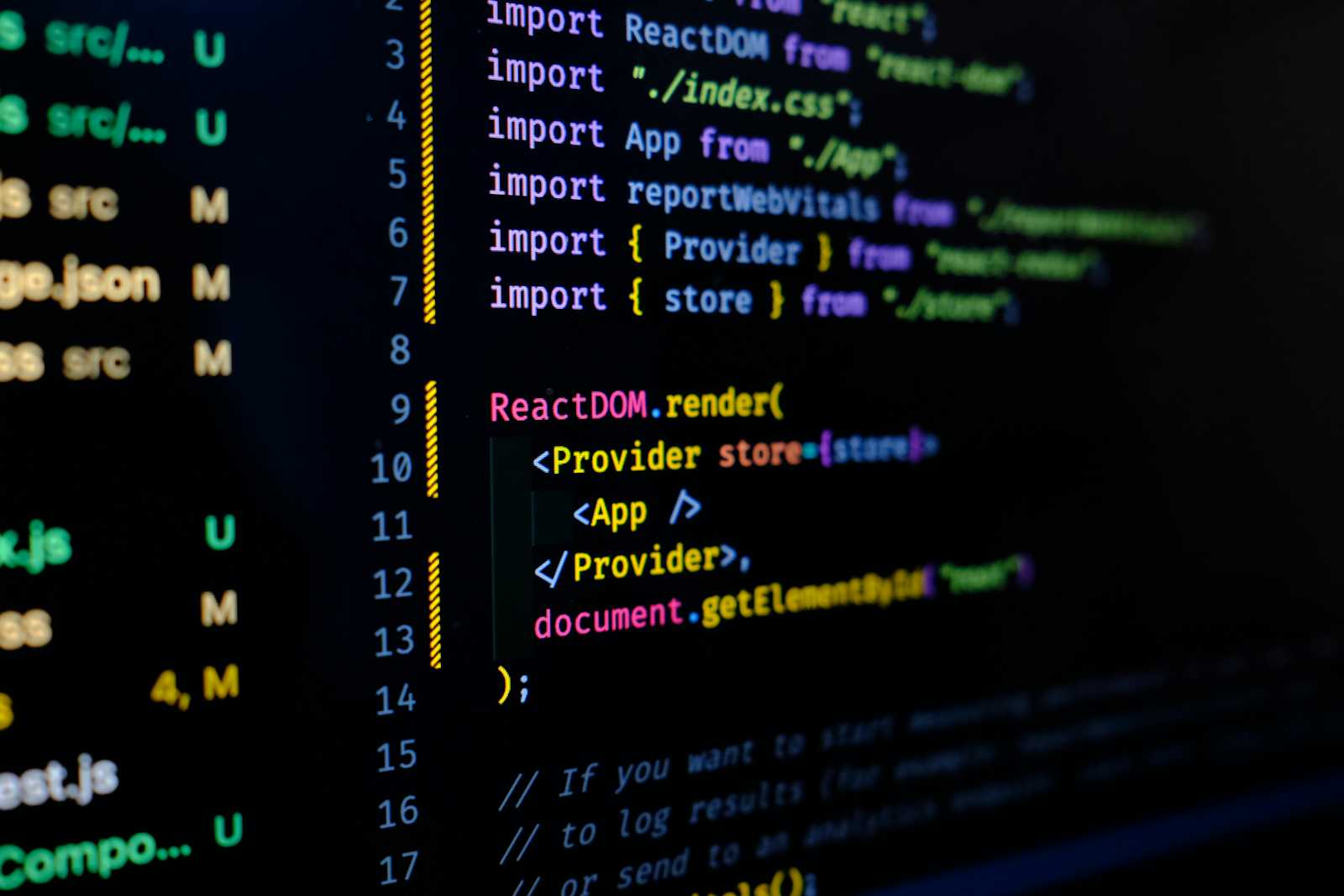
Creating a React Native video call app is an important skill in today's tech world. Whether you're building for work, education, or social use, real-time communication is key. This guide will show you how to make a video call app step by step using React Native.
We'll go through everything you need, from setting up your development tools to adding features like video streaming and user login. Even if you're new to mobile app development, you'll find simple instructions and useful tips to help you build a working and easy-to-use video call app. Let's start building a smooth communication tool for your users.
How to Build a React Native Video Call App
Building a React Native video call app might seem tough, but with the right tools, it’s actually quite simple. In this section, we’ll show you how to do it using ZEGOCLOUD Call Kit, which makes adding video call features to your app much easier.
ZEGOCLOUD is a platform designed to help developers add real-time communication features like voice and video calls to their apps. It offers prebuilt UI Kits that are easy to use, as well as custom kits, SDKs, and APIs for those who need more control and customisation. With ZEGOCLOUD, you can quickly integrate video calls into your app without starting from scratch.
Before we begin, let’s make sure you have everything you need:
Sign up for a ZEGOCLOUD developer account.
Get your app details from the ZEGOCLOUD dashboard.
Basic knowledge of React and JavaScript is needed, as we’ll be using these for the app.
Install NodeJS to help manage your development environment and dependencies.
Once you have these essentials, you’re ready to start building your React Native video call app using ZEGOCLOUD Call Kit. In the next sections, we’ll guide you step by step through the setup and integration, keeping everything clear and easy to follow.
1. Install Required Packages
To begin, you need to install the necessary packages in your project. Follow these steps:
Run the following command in your project root directory to install the ZEGOCLOUD package:
yarn add @zegocloud/zego-uikit-prebuilt-call-rn
Next, install other dependencies to ensure the ZEGOCLOUD package works correctly:
yarn add @zegocloud/zego-uikit-rn react-delegate-component @react-navigation/native @react-navigation/native-stack react-native-screens react-native-safe-area-context react-native-sound react-native-encrypted-storage zego-express-engine-reactnative@3.14.5 zego-zim-react-native@2.16.0 zego-zpns-react-native@2.6.6 zego-callkit-react-native@1.0.6 react-native-keep-awake@4.0.0 react-native-device-info
Note: If your React Native version is below 0.60, you might need additional steps, such as manually linking some dependencies.
2. Configure React Navigation
To enable navigation between multiple screens, configure React Navigation as follows:
Install the required navigation packages:
yarn add @react-navigation/native @react-navigation/native-stack
Integrate the
ZegoCallInvitationDialog
Component. Place theZegoCallInvitationDialog
component at the top level of yourNavigationContainer
. This dialog handles call invitations and should be present throughout your app.import { NavigationContainer } from '@react-navigation/native'; import { createNativeStackNavigator } from '@react-navigation/native-stack'; import ZegoUIKitPrebuiltCallService, { ZegoCallInvitationDialog, ZegoUIKitPrebuiltCallWaitingScreen, ZegoUIKitPrebuiltCallInCallScreen } from '@zegocloud/zego-uikit-prebuilt-call-rn'; const Stack = createNativeStackNavigator(); function App() { return ( <NavigationContainer> <ZegoCallInvitationDialog /> <Stack.Navigator initialRouteName="HomeScreen"> <Stack.Screen name="ZegoUIKitPrebuiltCallWaitingScreen" component={ZegoUIKitPrebuiltCallWaitingScreen} options={{ headerShown: false }} /> <Stack.Screen name="ZegoUIKitPrebuiltCallInCallScreen" component={ZegoUIKitPrebuiltCallInCallScreen} options={{ headerShown: false }} /> {/* Other Screens */} </Stack.Navigator> </NavigationContainer> ); } export default App;
Ensure that the screen names ZegoUIKitPrebuiltCallWaitingScreen
and ZegoUIKitPrebuiltCallInCallScreen
are not changed as they are required for the prebuilt call UI to function correctly.
3. Initialize Call Invitation Service
The next step is to initialize the call invitation service in your app. This should be done after the user logs in.
Import the necessary ZEGOCLOUD modules and initialize the service:
import { AppRegistry } from 'react-native'; import App from './App'; import { name as appName } from './app.json'; import ZegoUIKitPrebuiltCallService from '@zegocloud/zego-uikit-prebuilt-call-rn'; import as ZIM from 'zego-zim-react-native'; import as ZPNs from 'zego-zpns-react-native'; ZegoUIKitPrebuiltCallService.useSystemCallingUI([ZIM, ZPNs]); AppRegistry.registerComponent(appName, () => App);
After the user logs in, call the
ZegoUIKitPrebuiltCallService.init
method to initialize the call service:const onUserLogin = async (userID, userName) => { return ZegoUIKitPrebuiltCallService.init( yourAppID, // Replace with your App ID from ZEGOCLOUD console yourAppSign, // Replace with your App Sign from ZEGOCLOUD console userID, // Unique identifier for the user userName, // User's display name [ZIM, ZPNs], { ringtoneConfig: { incomingCallFileName: 'zego_incoming.mp3', outgoingCallFileName: 'zego_outgoing.mp3', }, androidNotificationConfig: { channelID: "ZegoUIKit", channelName: "ZegoUIKit", }, } ); }; const onUserLogout = async () => { return ZegoUIKitPrebuiltCallService.uninit(); };
4. Add Call Invitation Buttons
You can now add buttons to send call invitations. Add the ZegoSendCallInvitationButton
component. Configure the call invitation button with invitee details and call type (audio or video):
<ZegoSendCallInvitationButton
invitees={[{ userID: inviteeID, userName: inviteeName }]} // Replace with actual invitee info
isVideoCall={true} // true for video call, false for audio call
resourceID={"zego_call"} // Replace with the resource ID from ZEGOCLOUD console
/>
Note: resourceID is used to specify the ringtone for offline call invitations. It must match the Push Resource ID configured in the ZEGOCLOUD Admin Console.
Here is a complete example integrating all the steps:
import React, { useState, useEffect } from 'react';
import { View, Text, TextInput, Button, StyleSheet } from 'react-native';
import { NavigationContainer, useNavigation } from '@react-navigation/native';
import { createNativeStackNavigator } from '@react-navigation/native-stack';
import ZegoUIKitPrebuiltCallService, { ZegoCallInvitationDialog, ZegoUIKitPrebuiltCallWaitingScreen, ZegoUIKitPrebuiltCallInCallScreen, ZegoSendCallInvitationButton } from '@zegocloud/zego-uikit-prebuilt-call-rn';
const Stack = createNativeStackNavigator();
function App() {
return (
<NavigationContainer>
<ZegoCallInvitationDialog />
<Stack.Navigator initialRouteName="HomeScreen">
<Stack.Screen name="LoginScreen" component={LoginScreen} />
<Stack.Screen name="HomeScreen" component={HomeScreen} />
<Stack.Screen name="ZegoUIKitPrebuiltCallWaitingScreen" component={ZegoUIKitPrebuiltCallWaitingScreen} options={{ headerShown: false }} />
<Stack.Screen name="ZegoUIKitPrebuiltCallInCallScreen" component={ZegoUIKitPrebuiltCallInCallScreen} options={{ headerShown: false }} />
</Stack.Navigator>
</NavigationContainer>
);
}
function LoginScreen({ navigation }) {
const [userID, setUserID] = useState('');
const [userName, setUserName] = useState('');
const loginHandler = () => {
onUserLogin(userID, userName).then(() => {
navigation.navigate('HomeScreen', { userID });
});
};
return (
<View style={styles.container}>
<TextInput placeholder="User ID" value={userID} onChangeText={setUserID} />
<TextInput placeholder="User Name" value={userName} onChangeText={setUserName} />
<Button title="Login" onPress={loginHandler} />
</View>
);
}
function HomeScreen({ route }) {
const [invitees, setInvitees] = useState([]);
return (
<View style={styles.container}>
<TextInput placeholder="Invitee IDs" onChangeText={(text) => setInvitees(text.split(','))} />
<ZegoSendCallInvitationButton invitees={invitees.map(id => ({ userID: id, userName: user_${id} }))} isVideoCall={true} resourceID="zego_call" />
<ZegoSendCallInvitationButton invitees={invitees.map(id => ({ userID: id, userName: user_${id} }))} isVideoCall={false} resourceID="zego_call" />
</View>
);
}
export default App;
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
});
5. Android Configuration
If you're developing for Android, additional setup is required. Follow the steps below to configure your video call app for Android:
- Set up a Firebase project.
- Add your FCM certificate to the ZEGOCLOUD console and create a resource ID.
- Request permission for the system alert window (required for floating call notifications):
ZegoUIKitPrebuiltCallService.requestSystemAlertWindow({
message: 'We need your consent for the following permissions to use the offline call function properly',
allow: 'Allow',
deny: 'Deny',
});
Prevent code obfuscation by adding the following lines to your
proguard-rules.pro
file:-keep class .zego. { ; } -keep class *.**.zego_zpns.** { *; }
Add the Firebase Messaging dependency in your
android/app/build.gradle
:implementation 'com.google.firebase:firebase-messaging:21.1.0'
6. iOS Configuration
Follow these steps to configure you iOS video call app just like we did for Android:
Create a VoIP services certificate in the Apple Developer Center and add it to the ZEGOCLOUD console.
Add the necessary permissions in
my_project/ios/my_project/Info.plist
:<key>NSCameraUsageDescription</key> <string>We need to use the camera</string> <key>NSMicrophoneUsageDescription</key> <string>We need to use the microphone</string>
Enable Push Notifications and Background Modes capabilities in Xcode.
8. Run and Test
Finally, run your app to ensure everything works as expected. Make sure to test both video and audio calls:
Run on iOS:
yarn ios
Run on Android:
yarn android
Conusion
Creating a React Native video call app is a valuable skill, especially with the growing need for real-time communication in work, education, and social settings. This guide walked you through the process, from setting up your development tools to adding essential features like video streaming and user login.
Using tools like ZEGOCLOUD Call Kit makes the process straightforward, even for beginners. By following these steps, you can build a functional and user-friendly video call app that meets the needs of your users. Start coding today and bring seamless communication to your app!
Subscribe to my newsletter
Read articles from samuel chigozie directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
