GraphQL in Microsoft Fabric

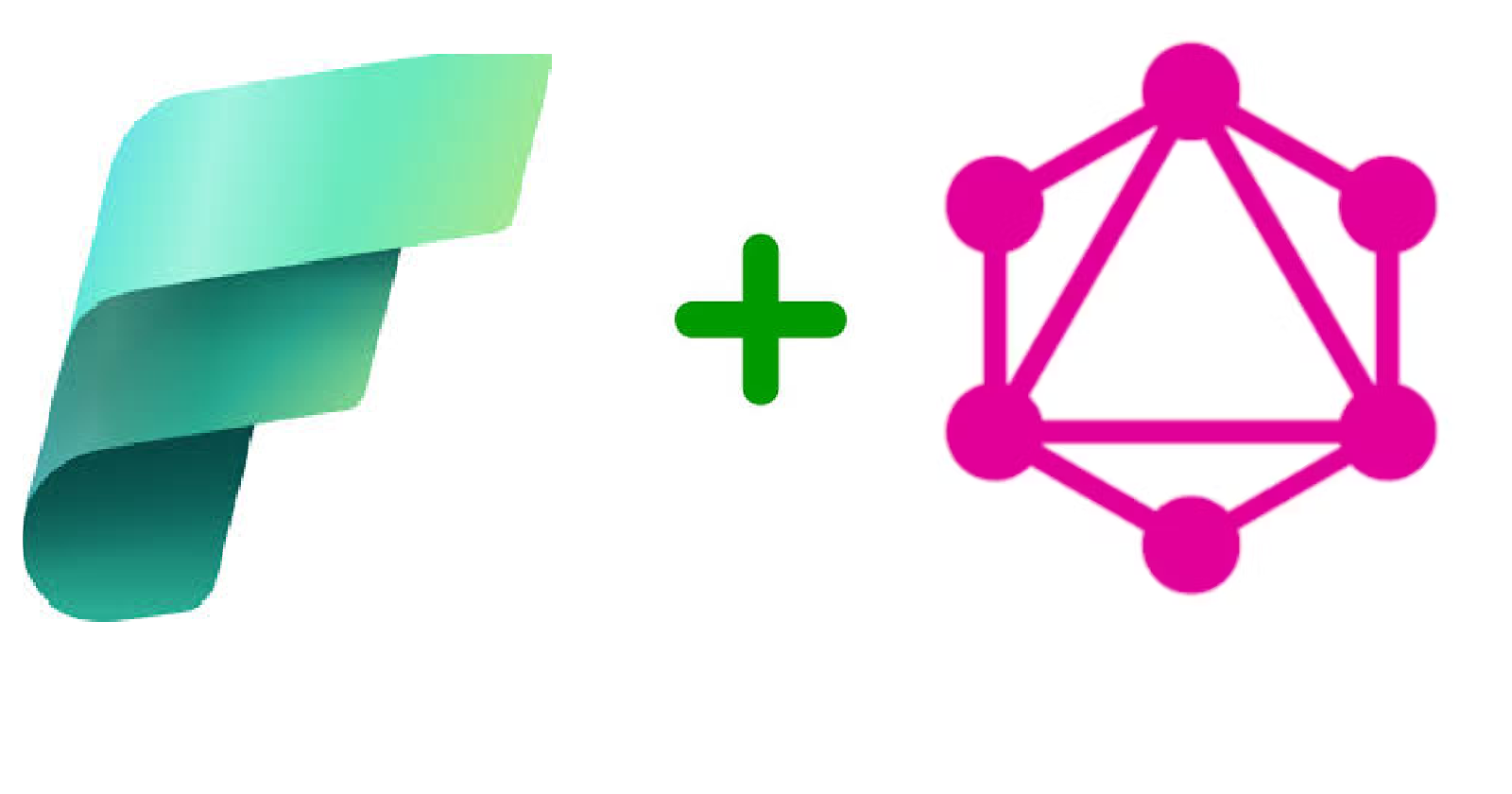
Some days ago, I had penned down an article on integrating GraphQL with Azure Functions. This let me to wonder if Microsoft Fabric offers any options for integrating with GraphQL .Thankfully it does. At the time of writing this article this feature is in preview status..so expect further enhancement in the future .
I explored further wondering if its possible to expose lakehouse data to an application’s data layer, so I created an C# console application to test it out.
The Setup
I used the following data that had five columns from one of the lakehouse tables.
I then created a GraphQL endpoint on Fabric for the above data .Creating a GraphQL on your lakehouse data is pretty straightforward. You have to set name and the datasource for your GraphQL endpoint and that's all. Once completed, the landing page of your Fabric GraphQL would look like this
On the left sidebar you'll find the columns of the underlying data and on the right, there's a query window where you can query the data through GraphQL endpoint.
What you see in the screenshot below is a simple GraphQL query to return the Value
and MetricDate
columns from the data source.
Also there is a Copy endpoint option. This option gives you the end point link of the GraphQL service of the workspace where its created.
The format is :https://api.fabric.microsoft.com/v1/workspaces/{workspaceid}/graphqlapis/{graphqlid}/graphql
The link will be required to be referenced in the console application.
Here is an example of a GraphQL query in Fabric with a filter parameter.
You can find a list of major filter parameters used in GraphQL here.
App Registration
Login to https://entra.microsoft.com to register your application. I registered a new application under the name Fabric GraphQL Calls
.For more detailed step by step process on how to register a new app, you can refer to one of my earlier article on Fabric REST API's https://www.azureguru.net/microsoft-fabric-rest-apis
To set permissions for the registered app, under the API permissions
select Delegated permissions
for Power BI
service.
and set GraphQL.Execute.All
option
C# Implementation
Create a new console application and ensure you have the following Nuget
references installed
using Microsoft.Identity.Client;
using GraphQL.Client.Http;
using GraphQL.Client.Serializer.Newtonsoft;
Declare a bunch of static variables
private const string ClientId = "This is the Application ID from Entra App Registration";
private const string Authority = "https://login.microsoftonline.com/organizations";
private const string GraphQLUri = "Fabric GraphQL endpoint URI";
private static string[] scopes = new string[] { "https://analysis.windows.net/powerbi/api/Item.Execute.All" };
In the Main
method of Progarm.cs
of the console application add the following code. This code is derived from the similar code that was used in my other article on Fabric REST API's. Only difference being that here we use GraphQLHttpClient
static async System.Threading.Tasks.Task Main(string[] args)
{
try
{
PublicClientApplicationBuilder PublicClientAppBuilder =
PublicClientApplicationBuilder.Create(ClientId)
.WithAuthority(Authority)
.WithRedirectUri("http://localhost");
IPublicClientApplication PublicClientApplication = PublicClientAppBuilder.Build();
AuthenticationResult result = await PublicClientApplication.AcquireTokenInteractive(scopes)
.ExecuteAsync()
.ConfigureAwait(false);
var graphQLClient = new GraphQLHttpClient(GraphQLUri, new NewtonsoftJsonSerializer());
graphQLClient.HttpClient.DefaultRequestHeaders.Authorization = new System.Net.Http.Headers.AuthenticationHeaderValue("Bearer", result.AccessToken);
HttpResponseMessage response = await graphQLClient.HttpClient.GetAsync(GraphQLUri);
graphQLClient.Options.IsValidResponseToDeserialize = response => response.IsSuccessStatusCode;
var query = new GraphQLHttpRequest
{
Query = @"query {
demo_datas
{
items {
Value,
MetricDate
}
}
}
"
};
Console.WriteLine((await graphQLClient.SendQueryAsync<dynamic>(query)).Data.ToString());
}
catch (Exception ex)
{
Console.WriteLine($"Error: {ex.Message}");
}
}
Executing the application outputs the lakehouse data in the JSON
format.
Closing Notes
I personally think that GraphQL feature in Fabric is a cool and fantastic feature. It offers a powerful and flexible way to query and interact with your data in lakehouse making it easier to access data on an application and help to create robust data-driven applications backed up by the Fabric ecosystem.
Thanks for reading !!!
Subscribe to my newsletter
Read articles from Sachin Nandanwar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
