Simplifying Task Scheduling in .NET Core with CronScheduler.AspNetCore and Generative AI

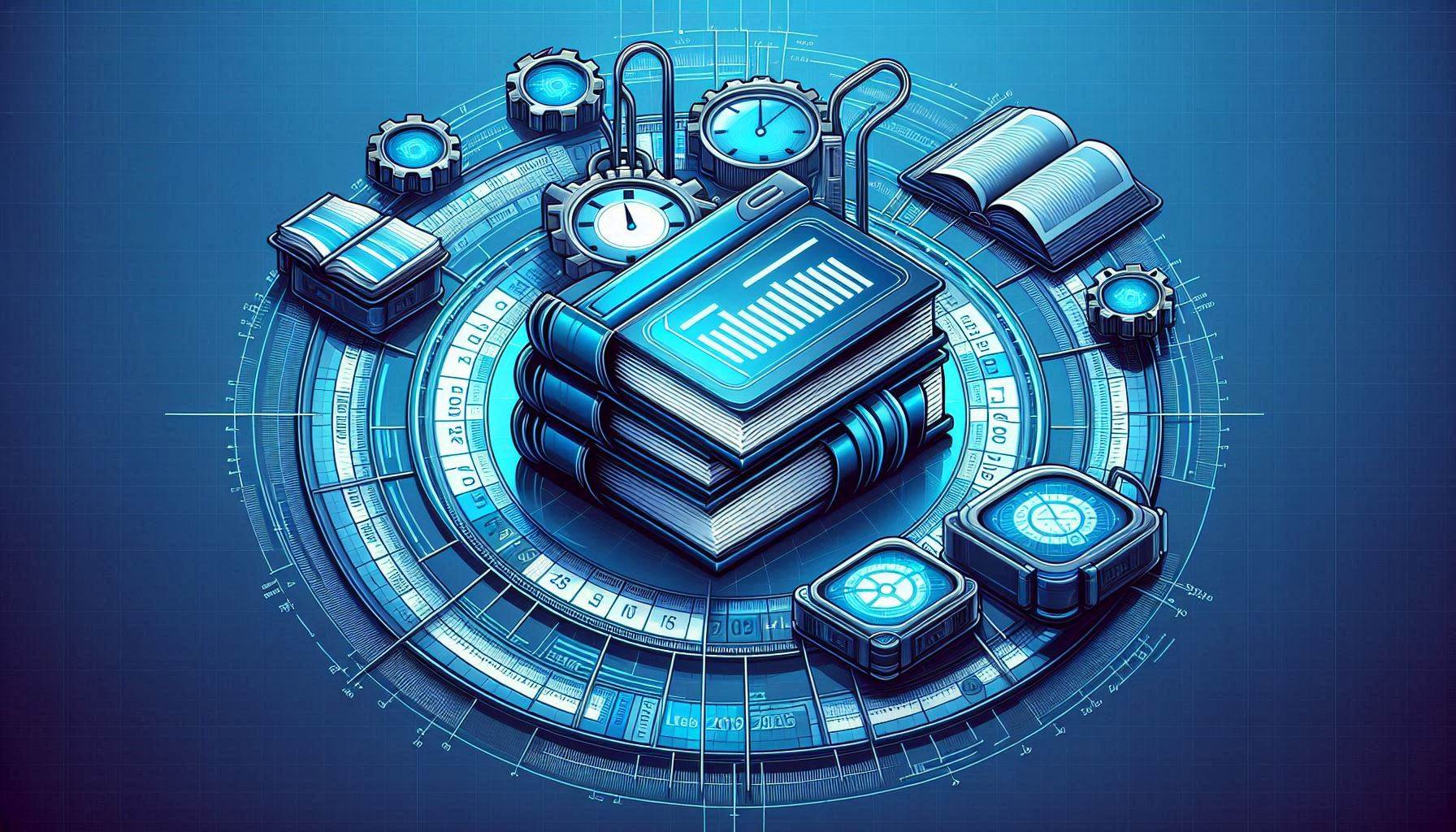
In the world of software development, efficient task scheduling is crucial for building scalable and maintainable applications. If you’re working with .NET Core, you might have encountered the complexities of existing scheduling libraries like Quartz. Enter CronScheduler.AspNetCore (https://github.com/kdcllc/CronScheduler.AspNetCore), a lightweight and easy-to-use library designed to simplify your scheduling needs.
What is CronScheduler.AspNetCore?
CronScheduler.AspNetCore is a library specifically designed for .NET Core applications, whether you’re using IHost or IWebHost. It adheres to the KISS (Keep It Simple, Stupid) principle, making it a straightforward alternative to more complex schedulers.
Key Features
Lightweight and Easy-to-Use: Unlike other scheduling libraries, CronScheduler.AspNetCore is designed to be lightweight, ensuring that it doesn’t add unnecessary overhead to your application.
Cron Syntax: The library uses cron syntax for scheduling tasks, making it familiar and easy to use for those who have worked with cron jobs before.
Async Initialization: With the IStartupJob feature, you can initialize critical processes asynchronously before the host starts, ensuring your application is ready to go even in complex environments like Kubernetes.
Flexible Hosting: Whether you’re hosting your application in AspNetCore or using a generic IHost, CronScheduler.AspNetCore has you covered.
Integrating Generative AI
Generative AI, a branch of artificial intelligence capable of creating new content such as text, images, and code, can further enhance the capabilities of CronScheduler.AspNetCore. By leveraging generative AI, you can automate the creation of complex scheduling configurations, generate dynamic task parameters, and even predict optimal scheduling times based on historical data.
Example Use Case: Dynamic Task Generation
Imagine you need to schedule tasks that vary based on user behavior or external data. Generative AI can analyze patterns and generate appropriate cron expressions dynamically. Here’s a conceptual example:
C#
public class DynamicCronJob : ICronJob
{
private readonly IGenerativeAIService _aiService;
public DynamicCronJob(IGenerativeAIService aiService)
{
_aiService = aiService;
}
public async Task ExecuteAsync(CancellationToken cancellationToken)
{
var cronExpression = await _aiService.GenerateCronExpressionAsync();
// Schedule the task based on the generated cron expression
}
}
public class Startup
{
public void ConfigureServices(IServiceCollection services)
{
services.AddSingleton<IGenerativeAIService, GenerativeAIService>();
services.AddCronScheduler(config =>
{
config.AddJob<DynamicCronJob>("*/5 * * * * *"); // Initial placeholder
});
}
}
In this example, DynamicCronJob
uses a generative AI service to create cron expressions dynamically, allowing for more flexible and adaptive scheduling.
Getting Started
To get started with CronScheduler.AspNetCore, you need to install the appropriate package. For AspNetCore hosting, use the following command:
dotnet add package CronScheduler.AspNetCore
For IHost hosting, use:
dotnet add package CronScheduler.Extensions
Conclusion
CronScheduler.AspNetCore is a powerful yet simple tool for managing scheduled tasks in .NET Core applications. Its lightweight nature and ease of use make it an excellent choice for developers looking to streamline their task scheduling processes. By integrating generative AI, you can take your scheduling capabilities to the next level, making your applications more adaptive and intelligent.
Give it a try and simplify your scheduling today!
Subscribe to my newsletter
Read articles from King David Consulting LLC directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

King David Consulting LLC
King David Consulting LLC
A highly skilled Cloud Solutions Architect with 20+ years of experience in software application development across diverse industries. Offering expertise in Cloud Computing and Artificial Intelligence. Passionate about designing and implementing innovative cloud-based solutions, migrating applications to the cloud, and integrating third-party platforms. Dedicated to collaborating with other developers and contributing to open-source projects to enhance software application functionality and performance