The Ultimate Cheat Sheet for Linux and Git

Table of contents
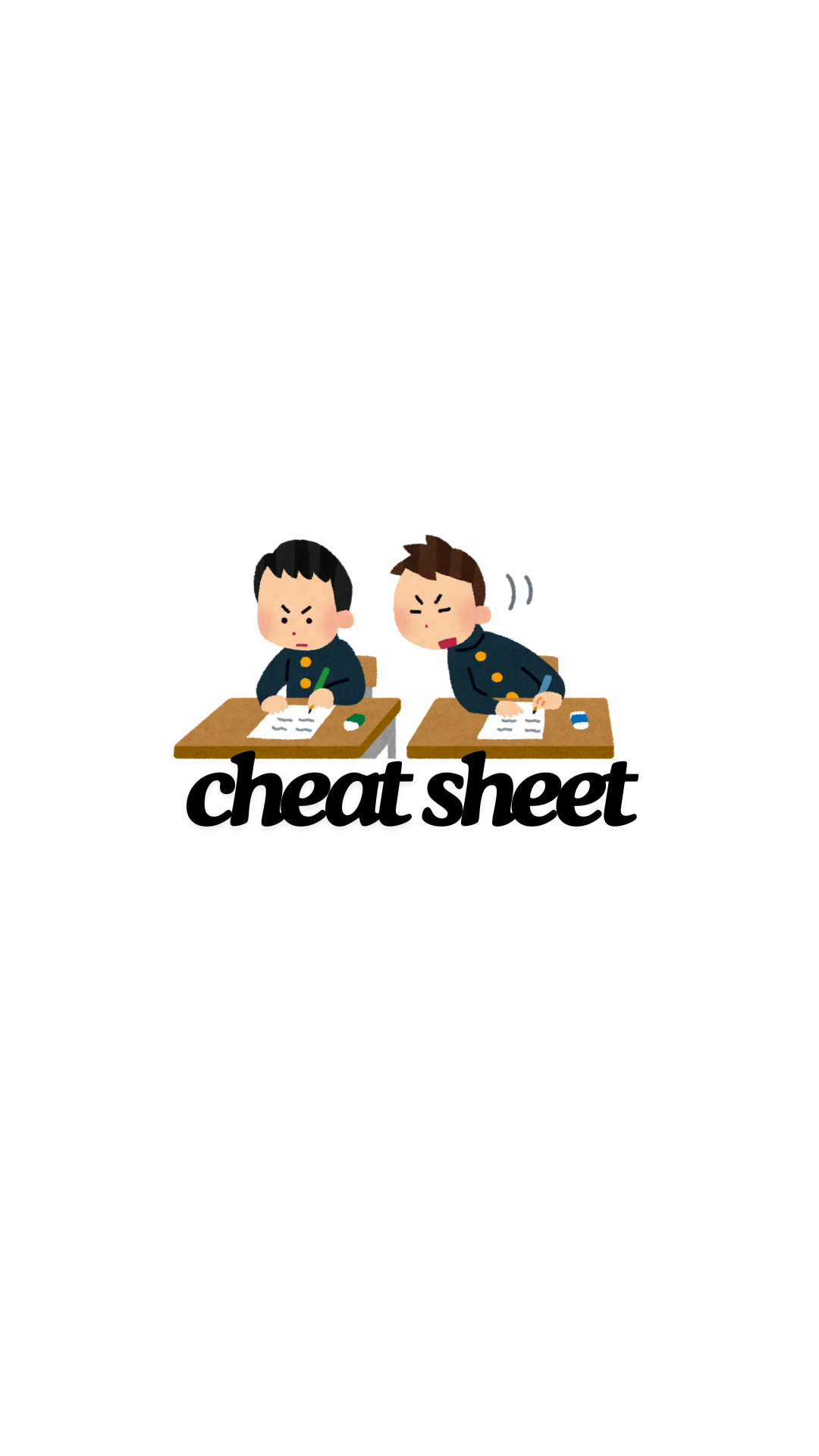
As DevOps engineers, mastering Linux and Git-GitHub is critical for efficient code management and deployment. With a multitude of commands and features at your disposal, having a well-organized cheat-sheet can significantly streamline your workflow. Below, I've compiled a detailed cheat-sheet with essential Linux commands, Git commands, and GitHub tips to enhance your DevOps toolkit.
Linux Commands
File & Directory Management
ls
– List directory contents.
Example:ls /home/user
lists all files and folders in the/home/user
directory.cd [directory]
– Change to a specified directory.
Example:cd /var/log
navigates to the/var/log
directory.pwd
– Print the current working directory.
Example:pwd
might output/home/user/projects
.mkdir [directory]
– Create a new directory.
Example:mkdir new_project
creates a directory namednew_project
.rmdir [directory]
– Remove an empty directory.
Example:rmdir old_folder
deletes the emptyold_folder
.rm [file]
– Remove a file.
Example:rm file.txt
deletesfile.txt
.cp [source] [destination]
– Copy files or directories.
Example:cp file.txt /backup/
copiesfile.txt
to the/backup
directory.mv [source] [destination]
– Move or rename files or directories.
Example:mv file.txt /archive/
movesfile.txt
to the/archive
directory.touch [file]
– Create an empty file or update its timestamp.
Example:touch newfile.txt
createsnewfile.txt
if it doesn't exist, or updates its timestamp.
File Viewing & Editing
cat [file]
– Display the content of a file.
Example:cat
README.md
shows the content ofREADME.md
.less [file]
– View file content page by page.
Example:less largefile.log
allows you to scroll throughlargefile.log
.head [file]
– Display the first 10 lines of a file.
Example:head log.txt
shows the top 10 lines oflog.txt
.tail [file]
– Display the last 10 lines of a file.
Example:tail error.log
shows the bottom 10 lines oferror.log
.nano [file]
– Edit a file using the Nano text editor.
Example:nano config.json
opensconfig.json
in Nano.vim [file]
– Edit a file using the Vim text editor.
Example:vim
script.sh
opensscript.sh
in Vim.
Permissions & Ownership
chmod [permissions] [file]
– Change file permissions.
Example:chmod 755
script.sh
sets permissions torwxr-xr-x
forscript.sh
.chown [owner]:[group] [file]
– Change file owner and group.
Example:chown user:admin file.txt
changes the owner touser
and the group toadmin
.ls -l
– List files with detailed information, including permissions and ownership.
Example:ls -l
displays file details in the current directory.
System Monitoring & Management
top
– Display active processes.
Example:top
shows real-time process information.ps aux
– List all running processes.
Example:ps aux
lists all processes with detailed information.df -h
– Show disk space usage.
Example:df -h
displays disk usage in a human-readable format.du -sh [directory]
– Show disk usage of a directory.
Example:du -sh /var
displays the size of the/var
directory.free -h
– Display memory usage.
Example:free -h
shows memory usage in a human-readable format.uptime
– Show system uptime and load averages.
Example:uptime
provides the system's uptime and load averages over the last 1, 5, and 15 minutes.
Git Commands
Basic Commands
git init
– Initialize a new Git repository.
Example:git init
sets up a new Git repository in the current directory.git clone [url]
– Clone a repository from a URL.
Example:git clone
https://github.com/user/repo.git
clones the repository from the given URL.git add [file]
– Add a file to the staging area.
Example:git add index.html
stagesindex.html
for commit.git commit -m "[message]"
– Commit changes with a message.
Example:git commit -m "Add new feature"
commits staged changes with the message "Add new feature".git status
– Show the status of changes.
Example:git status
displays which files are staged, unstaged, or untracked.git log
– Display commit history.
Example:git log
shows a list of recent commits.
Branching & Merging
git branch
– List all branches.
Example:git branch
lists all branches in the repository.git branch [branch_name]
– Create a new branch.
Example:git branch feature-branch
creates a new branch namedfeature-branch
.git checkout [branch_name]
– Switch to a specified branch.
Example:git checkout feature-branch
switches tofeature-branch
.git merge [branch_name]
– Merge a branch into the current branch.
Example:git merge feature-branch
mergesfeature-branch
into the current branch.git rebase [branch_name]
– Rebase the current branch onto another branch.
Example:git rebase main
rebases the current branch on top ofmain
.
Stashing
git stash
– Temporarily save changes without committing.
Example:git stash
saves your local changes and reverts to the last commit.git stash pop
– Apply stashed changes and remove them from the stash.
Example:git stash pop
re-applies the most recent stash and removes it.git stash list
– List all stashed changes.
Example:git stash list
shows all stashed changes.git stash drop
– Remove a specific stash.
Example:git stash drop stash@{0}
removes the specified stash.git stash clear
– Remove all stashes.
Example:git stash clear
deletes all stashed changes.
Cherry-Picking
git cherry-pick [commit_hash]
– Apply a specific commit from another branch.
Example:git cherry-pick 1a2b3c4d
applies the commit with hash1a2b3c4d
to the current branch.
Conflict Resolution
git status
– Show files with conflicts.
Example:git status
identifies files that have conflicts during a merge.git diff
– Show differences between conflicting versions.
Example:git diff
shows the changes that need to be resolved.git add [file]
– Stage resolved files for commit.
Example:git add resolved_file.txt
stages the resolved file for the next commit.
GitHub Tips
Repository Management
Create a Repository: Click "New" on GitHub and follow the setup prompts.
Fork a Repository: Click "Fork" at the top-right of the repository page to create a personal copy.
Clone a Repository: Use
git clone [url]
to copy a repository locally.
Example:git clone
https://github.com/user/repo.git
clones the repository.
Collaboration
Pull Requests: Create a pull request to propose and review changes before merging.
Issues: Track bugs, tasks, and feature requests using GitHub Issues.
Wiki: Use the GitHub Wiki for project documentation.
Branch Protection
- Enable Branch Protection: Configure settings to prevent force-pushes and require pull request reviews before merging.
GitHub Actions
- Set Up CI/CD: Create workflows in
.github/workflows
to automate build, test, and deployment processes.
Subscribe to my newsletter
Read articles from Namdev Pratap directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Namdev Pratap
Namdev Pratap
Come along with me on a public learning journey into AWS Cloud and DevOps, designed specifically for those without a technical background. I'll be documenting each step in straightforward, easy-to-understand language to help others make a smooth transition into DevOps. Together, we'll delve into continuous integration, deployment, and automation, breaking down complex concepts into manageable, actionable insights.