Bitcoin Smart Contracts: Handling Errors

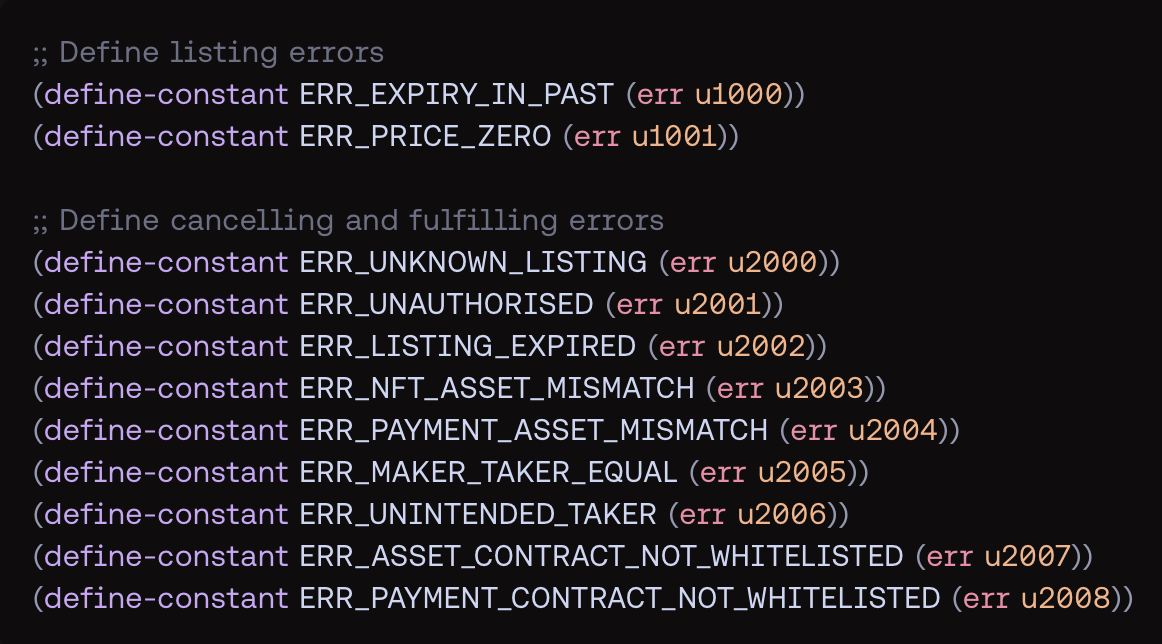
When you're developing smart contracts, especially on the Bitcoin blockchain using Clarity, handling errors early on is an important step. It’s like building the foundation of a house—you want to make sure it’s strong and stable. Errors aren't just bugs; they’re an opportunity to define the logic and ensure your contract behaves in a predictable, secure way.
Let’s take a gander. 🤓
Handling Errors Early
Think about this: when you're coding a smart contract, you're dealing with immutable transactions on the blockchain. Once a transaction is recorded, there’s no going back. This means that even a small mistake can lead to funds being locked up, assets misplaced, or worse—security vulnerabilities that could be exploited.
We can't afford mistakes.
By defining and handling errors early in the development process, you:
Ensure predictable outcomes.
Enhance security.
Improve code readability.
Clarity’s Error Handling Approach
Clarity emphasizes readability and predictability.🧐 Errors are explicitly defined and can be handled gracefully. Unlike Solidity, which uses exceptions, Clarity provides explicit error values, making the code easier to audit and reason about.
Let's see how it works.
Coding Errors in Clarity
Step 1: Define The Errors
The first thing you should do is define constants for your potential errors. By defining constants, you create reusable error codes that make the logic clear.
For instance, in the NFT marketplace example in Hiro Docs:
;; Define listing errors
(define-constant ERR_EXPIRY_IN_PAST (err u1000))
(define-constant ERR_PRICE_ZERO (err u1001))
;; Define cancelling and fulfilling errors
(define-constant ERR_UNKNOWN_LISTING (err u2000))
(define-constant ERR_UNAUTHORISED (err u2001))
(define-constant ERR_LISTING_EXPIRED (err u2002))
(define-constant ERR_NFT_ASSET_MISMATCH (err u2003))
(define-constant ERR_PAYMENT_ASSET_MISMATCH (err u2004))
(define-constant ERR_MAKER_TAKER_EQUAL (err u2005))
(define-constant ERR_UNINTENDED_TAKER (err u2006))
(define-constant ERR_ASSET_CONTRACT_NOT_WHITELISTED (err u2007))
(define-constant ERR_PAYMENT_CONTRACT_NOT_WHITELISTED (err u2008))
Each of these error codes clearly represents a specific failure condition, such as ERR_EXPIRY_IN_PAST
for an invalid expiration date or ERR_UNAUTHORISED
for access violations. By using constants, your error messages become declarative, meaning that anyone reading your code can instantly know what went wrong without deciphering ambiguous conditions.
Step 2: Return Errors Early
Clarity encourages a functional programming approach where errors are returned early and explicitly. Let's imagine you’re listing an NFT, and the price is set to zero. Instead of continuing down a path that will lead to a failure later, catch it at the start:
(define-public (list-nft (price uint) (expiry uint))
(begin
(if (is-eq price u0)
(err ERR_PRICE_ZERO) ;; Catch zero price error early
(if (< expiry (block-height))
(err ERR_EXPIRY_IN_PAST) ;; Ensure expiry is in the future
(ok "NFT listed successfully")))))
This early error return pattern ensures that your smart contract doesn’t waste computational resources or perform irreversible actions based on invalid data. More importantly, it prevents users from interacting with faulty logic.
Step 3: Use Errors for Security
Security is paramount. By properly defining and handling errors, you prevent a contract from being manipulated in unintended ways. Imagine a situation where someone tries to cancel an NFT listing they don’t own. You can do something like this:
(define-public (cancel-listing (listing-id uint) (owner principal))
(let ((listing-owner (unwrap! (map-get? listings {id: listing-id})
ERR_UNKNOWN_LISTING)))
(if (is-eq listing-owner owner)
(begin
;; proceed with cancellation
(ok "Listing cancelled"))
(err ERR_UNAUTHORISED))))
In this case, the ERR_UNKNOWN_LISTING
error ensures we don’t attempt to cancel a non-existent listing, and ERR_UNAUTHORISED
prevents unauthorized users from canceling listings they don’t own. 'Cause, yeah, that would be bad. 😅
Step 4: Testing and Debugging
Once you’ve defined and integrated your errors, testing becomes a lot easier. Since Clarity is a decidable language, every possible execution path can be simulated and tested. We can specifically test that the right errors are thrown under the right conditions:
(define-test (test-listing-errors)
(begin
;; Test price zero error
(asserts! (is-err (list-nft u0 u100)) (err ERR_PRICE_ZERO))
;; Test expiry in past error
(asserts! (is-err (list-nft u100 (block-height - 1))) (err ERR_EXPIRY_IN_PAST))))
By doing this, we’re ensuring that the contract not only performs its intended function but also handles all potential errors gracefully. And that’s 🔥
Conclusion
When building on a blockchain, we can’t leave error handling to the end. It’s an essential part of our design, ensuring security, clarity, and predictability from the ground up. As you begin your journey with Clarity, define your errors early. Handle them properly. And you’ll have the foundation necessary to build something great. 😎
Subscribe to my newsletter
Read articles from DAMIAN ROBINSON directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
